
- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
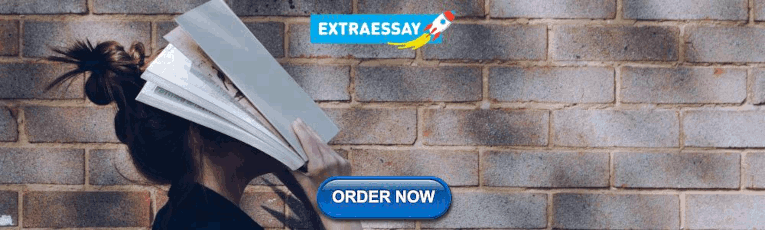
Pointer to an Array in C
An array name is a constant pointer to the first element of the array. Therefore, in this declaration,
balance is a pointer to &balance[0] , which is the address of the first element of the array.
In this code, we have a pointer ptr that points to the address of the first element of an integer array called balance .
In all the three cases, you get the same output −
If you fetch the value stored at the address that ptr points to, that is *ptr , then it will return 1 .
Array Names as Constant Pointers
It is legal to use array names as constant pointers and vice versa. Therefore, *(balance + 4) is a legitimate way of accessing the data at balance[4] .
Once you store the address of the first element in " ptr ", you can access the array elements using *ptr , *(ptr + 1) , *(ptr + 2) , and so on.
The following example demonstrates all the concepts discussed above −
When you run this code, it will produce the following output −
In the above example, ptr is a pointer that can store the address of a variable of double type. Once we have the address in ptr , *ptr will give us the value available at the address stored in ptr .
To Continue Learning Please Login
C Programming/Pointers and arrays
A pointer is a value that designates the address (i.e., the location in memory), of some value. Pointers are variables that hold a memory location.
There are four fundamental things you need to know about pointers:
- How to declare them (with the address operator ' & ': int *pointer = &variable; )
- How to assign to them ( pointer = NULL; )
- How to reference the value to which the pointer points (known as dereferencing , by using the dereferencing operator ' * ': value = *pointer; )
- How they relate to arrays (the vast majority of arrays in C are simple lists, also called "1 dimensional arrays", but we will briefly cover multi-dimensional arrays with some pointers in a later chapter ).
Pointers can reference any data type, even functions. We'll also discuss the relationship of pointers with text strings and the more advanced concept of function pointers.
- 1 Declaring pointers
- 2 Assigning values to pointers
- 3 Pointer dereferencing
- 4 Pointers and Arrays
- 5 Pointers in Function Arguments
- 6 Pointers and Text Strings
- 7.1 Practical use of function pointers in C
- 8 Examples of pointer constructs
- 10 External Links
Declaring pointers [ edit | edit source ]
Consider the following snippet of code which declares two pointers:
Lines 1-4 define a structure . Line 8 declares a variable that points to an int , and line 9 declares a variable that points to something with structure MyStruct. So to declare a variable as something that points to some type, rather than contains some type, the asterisk ( * ) is placed before the variable name.
In the following, line 1 declares var1 as a pointer to a long and var2 as a long and not a pointer to a long. In line 2, p3 is declared as a pointer to a pointer to an int.
Pointer types are often used as parameters to function calls. The following shows how to declare a function which uses a pointer as an argument. Since C passes function arguments by value, in order to allow a function to modify a value from the calling routine, a pointer to the value must be passed. Pointers to structures are also used as function arguments even when nothing in the struct will be modified in the function. This is done to avoid copying the complete contents of the structure onto the stack. More about pointers as function arguments later.
Assigning values to pointers [ edit | edit source ]
So far we've discussed how to declare pointers. The process of assigning values to pointers is next. To assign the address of a variable to a pointer, the & or 'address of' operator is used.
Here, pPointer will now reference myInt and pKeyboard will reference dvorak.
Pointers can also be assigned to reference dynamically allocated memory. The malloc() and calloc() functions are often used to do this.
The malloc function returns a pointer to dynamically allocated memory (or NULL if unsuccessful). The size of this memory will be appropriately sized to contain the MyStruct structure.
The following is an example showing one pointer being assigned to another and of a pointer being assigned a return value from a function.
When returning a pointer from a function, do not return a pointer that points to a value that is local to the function or that is a pointer to a function argument. Pointers to local variables become invalid when the function exits. In the above function, the value returned points to a static variable. Returning a pointer to dynamically allocated memory is also valid.
Pointer dereferencing [ edit | edit source ]
To access a value to which a pointer points, the * operator is used. Another operator, the -> operator is used in conjunction with pointers to structures. Here's a short example.
The expression bb->m_aNumber is entirely equivalent to (*bb).m_aNumber . They both access the m_aNumber element of the structure pointed to by bb . There is one more way of dereferencing a pointer, which will be discussed in the following section.
When dereferencing a pointer that points to an invalid memory location, an error often occurs which results in the program terminating. The error is often reported as a segmentation error. A common cause of this is failure to initialize a pointer before trying to dereference it.
C is known for giving you just enough rope to hang yourself, and pointer dereferencing is a prime example. You are quite free to write code that accesses memory outside that which you have explicitly requested from the system. And many times, that memory may appear as available to your program due to the vagaries of system memory allocation. However, even if 99 executions allow your program to run without fault, that 100th execution may be the time when your "memory pilfering" is caught by the system and the program fails. Be careful to ensure that your pointer offsets are within the bounds of allocated memory!
The declaration void *somePointer; is used to declare a pointer of some nonspecified type. You can assign a value to a void pointer, but you must cast the variable to point to some specified type before you can dereference it. Pointer arithmetic is also not valid with void * pointers.
Pointers and Arrays [ edit | edit source ]
Up to now, we've carefully been avoiding discussing arrays in the context of pointers. The interaction of pointers and arrays can be confusing but here are two fundamental statements about it:
- A variable declared as an array of some type acts as a pointer to that type. When used by itself, it points to the first element of the array.
- A pointer can be indexed like an array name.
The first case often is seen to occur when an array is passed as an argument to a function. The function declares the parameter as a pointer, but the actual argument may be the name of an array. The second case often occurs when accessing dynamically allocated memory.
Let's look at examples of each. In the following code, the call to calloc() effectively allocates an array of struct MyStruct items.
Pointers and array names can pretty much be used interchangeably; however, there are exceptions. You cannot assign a new pointer value to an array name. The array name will always point to the first element of the array. In the function returnSameIfAnyEquals , you could however assign a new value to workingArray, as it is just a pointer to the first element of workingArray. It is also valid for a function to return a pointer to one of the array elements from an array passed as an argument to a function. A function should never return a pointer to a local variable, even though the compiler will probably not complain.
When declaring parameters to functions, declaring an array variable without a size is equivalent to declaring a pointer. Often this is done to emphasize the fact that the pointer variable will be used in a manner equivalent to an array.
Now we're ready to discuss pointer arithmetic. You can add and subtract integer values to/from pointers. If myArray is declared to be some type of array, the expression *(myArray+j) , where j is an integer, is equivalent to myArray[j] . For instance, in the above example where we had the expression secondArray[i].otherNumber , we could have written that as (*(secondArray+i)).otherNumber or more simply (secondArray+i)->otherNumber .
Note that for addition and subtraction of integers and pointers, the value of the pointer is not adjusted by the integer amount, but is adjusted by the amount multiplied by the size of the type to which the pointer refers in bytes. (For example, pointer + x can be thought of as pointer + (x * sizeof(*type)) .)
One pointer may also be subtracted from another, provided they point to elements of the same array (or the position just beyond the end of the array). If you have a pointer that points to an element of an array, the index of the element is the result when the array name is subtracted from the pointer. Here's an example.
You may be wondering how pointers and multidimensional arrays interact. Let's look at this a bit in detail. Suppose A is declared as a two dimensional array of floats ( float A[D1][D2]; ) and that pf is declared a pointer to a float. If pf is initialized to point to A[0][0], then *(pf+1) is equivalent to A[0][1] and *(pf+D2) is equivalent to A[1][0]. The elements of the array are stored in row-major order.
Let's look at a slightly different problem. We want to have a two dimensional array, but we don't need to have all the rows the same length. What we do is declare an array of pointers. The second line below declares A as an array of pointers. Each pointer points to a float. Here's some applicable code:
We also note here something curious about array indexing. Suppose myArray is an array and i is an integer value. The expression myArray[i] is equivalent to i[myArray] . The first is equivalent to *(myArray+i) , and the second is equivalent to *(i+myArray) . These turn out to be the same, since the addition is commutative.
Pointers can be used with pre-increment or post-decrement, which is sometimes done within a loop, as in the following example. The increment and decrement applies to the pointer, not to the object to which the pointer refers. In other words, *pArray++ is equivalent to *(pArray++) .
Pointers in Function Arguments [ edit | edit source ]
Often we need to invoke a function with an argument that is itself a pointer. In many instances, the variable is itself a parameter for the current function and may be a pointer to some type of structure. The ampersand ( & ) character is not needed in this circumstance to obtain a pointer value, as the variable is itself a pointer. In the example below, the variable pStruct , a pointer, is a parameter to function FunctTwo , and is passed as an argument to FunctOne .
The second parameter to FunctOne is an int. Since in function FunctTwo , mValue is a pointer to an int, the pointer must first be dereferenced using the * operator, hence the second argument in the call is *mValue . The third parameter to function FunctOne is a pointer to a long. Since pAA is itself a pointer to a long, no ampersand is needed when it is used as the third argument to the function.
Pointers and Text Strings [ edit | edit source ]
Historically, text strings in C have been implemented as arrays of characters, with the last byte in the string being a zero, or the null character '\0'. Most C implementations come with a standard library of functions for manipulating strings. Many of the more commonly used functions expect the strings to be null terminated strings of characters. To use these functions requires the inclusion of the standard C header file "string.h".
A statically declared, initialized string would look similar to the following:
The variable myFormat can be viewed as an array of 21 characters. There is an implied null character ('\0') tacked on to the end of the string after the 'd' as the 21st item in the array. You can also initialize the individual characters of the array as follows:
An initialized array of strings would typically be done as follows:
The initialization of an especially long string can be split across lines of source code as follows.
The library functions that are used with strings are discussed in a later chapter.
Pointers to Functions [ edit | edit source ]
C also allows you to create pointers to functions. Pointers to functions syntax can get rather messy. As an example of this, consider the following functions:
Declaring a typedef to a function pointer generally clarifies the code. Here's an example that uses a function pointer, and a void * pointer to implement what's known as a callback. The DoSomethingNice function invokes a caller supplied function TalkJive with caller data. Note that DoSomethingNice really doesn't know anything about what dataPointer refers to.
Some versions of C may not require an ampersand preceding the TalkJive argument in the DoSomethingNice call. Some implementations may require specifically casting the argument to the MyFunctionType type, even though the function signature exacly matches that of the typedef.
Function pointers can be useful for implementing a form of polymorphism in C. First one declares a structure having as elements function pointers for the various operations to that can be specified polymorphically. A second base object structure containing a pointer to the previous structure is also declared. A class is defined by extending the second structure with the data specific for the class, and static variable of the type of the first structure, containing the addresses of the functions that are associated with the class. This type of polymorphism is used in the standard library when file I/O functions are called.
A similar mechanism can also be used for implementing a state machine in C. A structure is defined which contains function pointers for handling events that may occur within state, and for functions to be invoked upon entry to and exit from the state. An instance of this structure corresponds to a state. Each state is initialized with pointers to functions appropriate for the state. The current state of the state machine is in effect a pointer to one of these states. Changing the value of the current state pointer effectively changes the current state. When some event occurs, the appropriate function is called through a function pointer in the current state.
Practical use of function pointers in C [ edit | edit source ]
Function pointers are mainly used to reduce the complexity of switch statement. Example with switch statement:
Without using a switch statement:
Function pointers may be used to create a struct member function:
Use to implement this pointer (following code must be placed in library).
Examples of pointer constructs [ edit | edit source ]
Below are some example constructs which may aid in creating your pointer.
sizeof [ edit | edit source ]
The sizeof operator is often used to refer to the size of a static array declared earlier in the same function.
To find the end of an array (example from wikipedia:Buffer overflow ):
To iterate over every element of an array, use
Note that the sizeof operator only works on things defined earlier in the same function. The compiler replaces it with some fixed constant number. In this case, the buffer was declared as an array of 10 char's earlier in the same function, and the compiler replaces sizeof(buffer) with the number 10 at compile time (equivalent to us hard-coding 10 into the code in place of sizeof(buffer) ). The information about the length of buffer is not actually stored anywhere in memory (unless we keep track of it separately) and cannot be programmatically obtained at run time from the array/pointer itself.
Often a function needs to know the size of an array it was given -- an array defined in some other function. For example,
Unfortunately, (in C and C++) the length of the array cannot be obtained from an array passed in at run time, because (as mentioned above) the size of an array is not stored anywhere. The compiler always replaces sizeof with a constant. This sum() routine needs to handle more than just one constant length of an array.
There are some common ways to work around this fact:
- Write the function to require, for each array parameter, a "length" parameter (which has type "size_t"). (Typically we use sizeof at the point where this function is called).
- Use of a convention, such as a null-terminated string to mark the end of the array.
- Instead of passing raw arrays, pass a structure that includes the length of the array (such as ".length") as well as the array (or a pointer to the first element); similar to the string or vector classes in C++.
It's worth mentioning that sizeof operator has two variations: sizeof ( type ) (for instance: sizeof (int) or sizeof (struct some_structure) ) and sizeof expression (for instance: sizeof some_variable.some_field or sizeof 1 ).
External Links [ edit | edit source ]
- "Common Pointer Pitfalls" by Dave Marshall
- Book:C Programming
Navigation menu
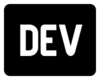
DEV Community

Posted on Oct 29, 2023
How C-Pointers Works: A Step-by-Step Beginner's Tutorial
In this comprehensive C Pointers tutorial, my primary goal is to guide you through the fundamentals of C pointers from the ground up. By the end of this tutorial, you will have gained an in-depth understanding of the following fundamental topics:
- What is a Pointer?
- How Data is Stored in Memory?
- Storing Memory Addresses using Pointers
Accessing Data through Pointers
- Pointer Arithmetic
- Pointer to Pointer (Double Pointers)
- Passing Pointers as Function Arguments
Arrays of Pointers
Null pointers, prerequisite:.
To grasp pointers effectively, you should be comfortable with basic C programming concepts, including variables, data types, functions, loops, and conditional statements. This familiarity with C programming forms the foundation for understanding how pointers work within the language. Once you have a solid grasp of these fundamental concepts, you can confidently delve into the intricacies of C pointers.
What is a pointer?
A pointer serves as a reference that holds the memory location of another variable. This memory address allows us to access the value stored at that location in the memory. You can think of a pointer as a way to reference or point to the location where data is stored in your computer's memory
Pointers can be a challenging concept for beginners to grasp, but in this tutorial, I'll explain them using real-life analogies to make the concept clearer. However, Before delving into pointers and their workings, it's important to understand the concept of a memory address.
A memory address is a unique identifier that points to a specific location in a computer's memory. Think of it like a street address for data stored in your computer's RAM (Random Access Memory). Just as a street address tells you where a particular house is located in the physical world, a memory address tells the computer where a specific piece of information or data is stored in its memory.
Take a look at the image below for a better understanding:

In this illustration, each block represents one byte of memory. It's important to note that every byte of memory has a unique address. To make it easier to understand, I've represented the addresses in decimal notation, but computers actually store these addresses using hexadecimal values. Hexadecimal is a base-16 numbering system commonly used in computing to represent memory addresses and other low-level data. It's essential to be aware of this representation when working with memory-related concepts in computer programming
How data is stored in the memory:
Every piece of data in your computer, whether it's a number, a character, or a program instruction, is stored at a specific memory address. The amount of space reserved for each data type can vary, and it is typically measured in bytes (where 1 byte equals 8 bits, with each bit representing either 0 or 1). The specific sizes of data types also depend on the computer architecture you are using. For instance, on most 64-bit Linux machines, you'll find the following typical sizes for common data types: char = 1 byte int = 4 bytes float = 4 bytes double = 8 bytes These sizes define how much memory each data type occupies and are crucial for memory management and efficient data representation in computer systems.
You can use the sizeof operator to determine the size of data types on your computer. example:
In this example: sizeof(char) returns the size of the char data type in bytes. sizeof(int) returns the size of the int data type in bytes. sizeof(float) returns the size of the float data type in bytes. sizeof(double) returns the size of the double data type in bytes. When you run this code, it will print the sizes of these data types on your specific computer, allowing you to see the actual sizes used by your system.
When you declare a variable, the computer allocates a specific amount of memory space corresponding to the chosen data type. For instance, when you declare a variable of type char, the computer reserves 1 byte of memory because the size of the 'char' data type is conventionally 1 byte.

In this example, we declare a variable n of type char without assigning it a specific value. The memory address allocated for the n variable is 106 . This address, 106 , is where the computer will store the char variable n, but since we haven't assigned it a value yet, the content of this memory location may initially contain an unpredictable or uninitialized value.
When we assign the value 'C' to the variable n, the character 'C' is stored in the memory location associated with the variable n. When we assign the value 'C' to the variable n, the character 'C' is stored in the memory location associated with the variable n.

As mentioned earlier, a byte can only store numerical values. When we store the letter 'C' in a byte, the byte actually holds the ASCII code for 'C,' which is 67. In computer memory, characters are represented using their corresponding ASCII codes. So, in memory, the character 'C' is stored as the numerical value 67. Here's how it looks in memory

Since integers are typically stored within four bytes of memory, let's consider the same example with an int variable. In this scenario, the memory structure would appear as follows:

In this example, the memory address where the variable t is stored is 121. An int variable like “t” typically uses four consecutive memory addresses, such as 121, 122, 123, and 124. The starting address, in this case, 121, represents the location of the first byte of the int, and the subsequent addresses sequentially represent the following bytes that collectively store the complete int value.
If you want to know the memory address of a variable in a program, you can use the 'address of' unary operator, often denoted as the '&' operator. This operator allows you to access the specific memory location where a variable is stored.
When you run the following program on your computer: It will provide you with specific memory addresses for the variables c and n. However, each time you rerun the program, it might allocate new memory addresses for these variables. It's important to understand that while you can determine the memory address of a variable using the & operator, the exact memory location where a variable is stored is typically managed by the system and the compiler. As a programmer, you cannot directly control or assign a specific memory location for a variable. Instead, memory allocation and management are tasks handled by the system and the compiler.
Storing memory address using pointers
As mentioned earlier, a pointer is a variable that stores the memory address of another variable. This memory address allows us to access the value stored at that location in memory. You can think of a pointer as a way to reference or point to the location where data is stored in your computer's memory.
Now, let's begin by declaring and initializing pointers. This step is essential because it sets up the pointer to hold a specific memory address, enabling us to interact with the data stored at that location.
Declaring Pointers: To declare a pointer, you specify the data type it points to, followed by an asterisk (*), and then the pointer's name. For example:
Here, we've declared a pointer named ptr that can point to integers.

The size of pointers on 64-bit systems is usually 8 bytes (64 bits). To determine the pointer size on your system, you can use the sizeof operator:
Initializing Pointers: Once you've declared a pointer, you typically initialize it with the memory address it should point to. Once again, To obtain the memory address of a variable, you can employ the address-of operator (&). For instance:
In this program:
We declare an integer variable x and initialize it with the value 10. This line creates a variable x in memory and assigns the value 10 to it.

We declare an integer pointer ptr using the int *ptr syntax. This line tells the compiler that ptr will be used to store the memory address of an integer variable.

We initialize the pointer ptr with the memory address of the variable x . This is achieved with the line ptr = &x; . The & operator retrieves the memory address of x, and this address is stored in the pointer ptr .

Dereferencing Pointers: To access the data that a pointer is pointing to, you need to dereference the pointer. Dereferencing a pointer means accessing the value stored at the memory address that the pointer points to. In C, you can think of pointers as variables that store memory addresses rather than actual values. To get the actual value (data) stored at that memory address, you need to dereference the pointer.
Dereferencing is done using the asterisk (*) operator. Here's an example:
It looks like this in the memory: int x = 10; variable 'x' stores the value 10:

int *ptr = &x; Now, the pointer 'ptr' point to the address of 'x':

int value = *ptr; Dereference 'ptr' to get the value stored at the address it points to:

Reading and Modifying Data: Pointers allow you to not only read but also modify data indirectly:
Note: The asterisk is a versatile symbol with different meanings depending on where it's used in your C program, for example: Declaration: When used during variable declaration, the asterisk (*) indicates that a variable is a pointer to a specific data type. For example: int *ptr; declares 'ptr' as a pointer to an integer.
Dereferencing: Inside your code, the asterisk (*) in front of a pointer variable is used to access the value stored at the memory address pointed to by the pointer. For example: int value = *ptr; retrieves the value at the address 'ptr' points to.
Pointer Arithmetic:
Pointer arithmetic is the practice of performing mathematical operations on pointers in C. This allows you to navigate through arrays, structures, and dynamically allocated memory. You can increment or decrement pointers, add or subtract integers from them, and compare them. It's a powerful tool for efficient data manipulation, but it should be used carefully to avoid memory-related issues.
Incrementing a Pointer:
Now, this program is how it looks in the memory: int arr[4] = {10, 20, 30, 40};

This behavior is a key aspect of pointer arithmetic. When you add an integer to a pointer, it moves to the memory location of the element at the specified index, allowing you to efficiently access and manipulate elements within the array. It's worth noting that you can use pointer arithmetic to access elements in any position within the array, making it a powerful technique for working with arrays of data. Now, let's print the memory addresses of the elements in the array from our previous program.
If you observe the last two digits of the first address is 40, and the second one is 44. You might be wondering why it's not 40 and 41. This is because we're working with an integer array, and in most systems, the size of an int data type is 4 bytes. Therefore, the addresses are incremented in steps of 4. The first address shows 40, the second 44, and the third one 48
Decrementing a Pointer Decrement (--) a pointer variable, which makes it point to the previous element in an array. For example, ptr-- moves it to the previous one. For example:
Explanation:
We have an integer array arr with 5 elements, and we initialize a pointer ptr to point to the fourth element (value 40) using &arr[3].
Then, we decrement the pointer ptr by one with the statement ptr--. This moves the pointer to the previous memory location, which now points to the third element (value 30).
Finally, we print the value pointed to by the decremented pointer using *ptr, which gives us the value 30.
In this program, we demonstrate how decrementing a pointer moves it to the previous memory location in the array, allowing you to access and manipulate the previous element.
Pointer to pointer
Pointers to pointers, or double pointers, are variables that store the address of another pointer. In essence, they add another level of indirection. These are commonly used when you need to modify the pointer itself or work with multi-dimensional arrays.
To declare and initialize a pointer to a pointer, you need to add an extra asterisk (*) compared to a regular pointer. Let's go through an example:
In this example, ptr2 is a pointer to a pointer. It points to the memory location where the address of x is stored (which is ptr1 ).

The below program will show you how to print the value of x through pointer to pointer
In this program, we first explain that it prints the value of x using a regular variable, a pointer, and a pointer to a pointer. We then print the memory addresses of x , ptr1 , and ptr2 .
Passing Pointers as Function Arguments:
In C, you can pass pointers as function arguments. This allows you to manipulate the original data directly, as opposed to working with a copy of the data, as you would with regular variables. Here's how it works:
How to Declare and Define Functions that Take Pointer Arguments: In your function declaration and definition, you specify that you're passing a pointer by using the * operator after the data type. For example:
In the above function, we declare ptr as a pointer to an integer. This means it can store the memory address of an integer variable.
Why Would You Pass Pointers to Functions?
Passing pointers to functions allows you to:
- Modify the original data directly within the function.
- Avoid making a copy of the data, which can be more memory-efficient.
- Share data between different parts of your program efficiently.
This concept is especially important when working with large data structures or when you need to return multiple values from a function.
Call by Value vs. Call by Reference:
Understanding how data is passed to functions is crucial when working with pointers. there are two common ways that data can be passed to functions: call by value and call by reference.
Call by Value:
When you pass data by value, a copy of the original data is created inside the function. Any modifications to this copy do not affect the original data outside of the function. This is the default behavior for most data types when you don't use pointers.
Call by Reference (Using Pointers):
When you pass data by reference, you're actually passing a pointer to the original data's memory location. This means any changes made within the function will directly affect the original data outside the function. This is achieved by passing pointers as function arguments, making it call by reference. Using pointers as function arguments allows you to achieve call by reference behavior, which is particularly useful when you want to modify the original data inside a function and have those changes reflected outside the function.
Let's dive into some code examples to illustrate how pointers work as function arguments. We'll start with a simple example to demonstrate passing a pointer to a function and modifying the original data.
Consider this example:
In this code, we define a function modifyValue that takes a pointer to an integer. We pass the address of the variable num to this function, and it doubles the value stored in num directly.
This is a simple demonstration of passing a pointer to modify a variable's value. Pointers allow you to work with the original data efficiently.
An array of pointers is essentially an array where each element is a pointer. These pointers can point to different data types (int, char, etc.), providing flexibility and efficiency in managing memory.
How to Declare an Array of Pointers? To declare an array of pointers, you specify the type of data the pointers will point to, followed by square brackets to indicate it's an array, and then the variable name. For example:
Initializing an Array of Pointers You can initialize an array of pointers to each element to point to a specific value, For example:
How to Access Elements Through an Array of Pointers? To access elements through an array of pointers, you can use the pointer notation. For example:
This program demonstrates how to access and print the values pointed to by the pointers in the array.
A NULL pointer is a pointer that lacks a reference to a valid memory location. It's typically used to indicate that a pointer doesn't have a specific memory address assigned, often serving as a placeholder or default value for pointers.
Here's a code example that demonstrates the use of a NULL pointer:
In this example, we declare a pointer ptr and explicitly initialize it with the value NULL. We then use an if statement to check if the pointer is NULL. Since it is, the program will print "The pointer is NULL." This illustrates how NULL pointers are commonly used to check if a pointer has been initialized or assigned a valid memory address.
conclusion:
You've embarked on a comprehensive journey through the intricacies of C pointers. You've learned how pointers store memory addresses, enable data access, facilitate pointer arithmetic, and how they can be used with arrays and functions. Additionally, you've explored the significance of NULL pointers.
By completing this tutorial, you've equipped yourself with a robust understanding of pointers in C. You can now confidently navigate memory, manipulate data efficiently, and harness the power of pointers in your programming projects. These skills will be invaluable as you advance in your coding endeavors. Congratulations on your accomplishment, and keep coding with confidence!
Reference: C - Pointers - Tutorials Point
Pointers in C: A One-Stop Solution for Using C Pointers - simplilearn
Top comments (3)
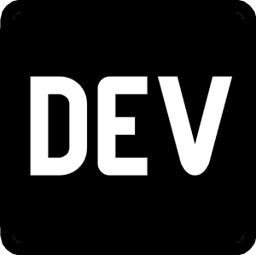
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Jan 7, 2024
Love your way to write articles, could you add an article for, .o files, .h files, lists and makefile? Thank you in advance!

- Joined Nov 4, 2023
Great post. Thank you so much for this.

- Email [email protected]
- Joined Jul 7, 2023
Thank you for your kind words! I'm thrilled to hear that you enjoyed the article. Your feedback means a lot to me. If you have any questions or if there's a specific topic you'd like to see in future posts, feel free to let me know. Thanks again for your support
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

SMTPGet Review- Analyzing Client Reviews, Ratings, and User Experience
Otis Milburnn - May 14

Carregando dados com Apache HOP & Postgres
DeadPunnk - May 9
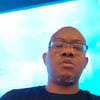
HOW TO UTILIZE MICROSOFT AZURE ACTIVE DIRECTORY TO MANAGE CLOUD-BASED IDENTITIES.
Oziegbe Pierre Okukpon - May 9
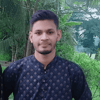
Scop in Javascript.
ABU SAID - May 10
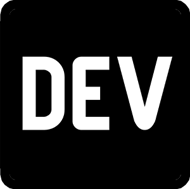
We're a place where coders share, stay up-to-date and grow their careers.
C Programming Tutorial
- Pointers and 1-D arrays
Last updated on July 27, 2020
In C, the elements of an array are stored in contiguous memory locations. For example: if we have the following array.
Then, this is how elements are stored in the array.
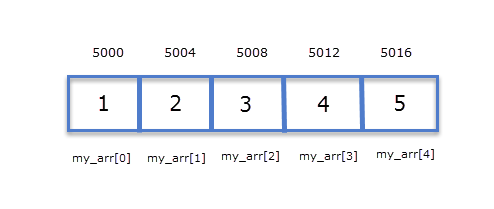
Here the first element is at address 5000 , since each integer takes 4 bytes the next element is at 5004 and so on.
In C, pointers and arrays are very closely related. We can access the elements of the array using a pointer. Behind the scenes compiler also access elements of the array using pointer notation rather than subscript notation because accessing elements using pointer is very efficient as compared to subscript notation. The most important thing to remember about the array is this:
The name of the array is a constant pointer that points to the address of the first element of the array or the base address of the array.
We can use subscript notation (i.e using square brackets) to find the address of the elements of the array. For example:
here &my_arr[0] points to the address of the first element of the array. Since the name of the array is a constant pointer that points to the first element of the array, my_arr and &my_arr[0] represent the same address. &my_arr[1] points to the address of the second element. Similarly &my_arr[2] points to the address of the third element and so on.
Note: my_arr is of type (int *) or pointer to int .
The following program demonstrates that the elements of an array are stored in contiguous memory locations.
Expected Output:
Note: Memory address may differ every time you run the program.
Using pointers to access elements and address of elements in an array #
We know that name of the array is a constant pointer to the first element. Consider the following snippet:
Here arr is a pointer to the first element. But, What is the base type of pointer arr ? If your answer is a pointer to int or (int *) . Well, Done ;).
In this case, arr points to the address of an integer number i.e address of integer 1 . So the base type of arr is a pointer to int or (int*) .
Let's take some more examples:
What is the type of pointer arr ?.
Here arr points to the address of the first element which is a character. So the type of arr is a pointer to char or (char *) .
here arr is a pointer of type pointer to double or (double *) .
Note: These concepts are the building blocks for the upcoming chapters, so don't skip them. If it is still confusing to you, go through it one more time.
Now you can easily access values and address of elements using pointer arithmetic. Suppose my_arr is an array of 5 integers.
Here my_arr is a constant pointer of base type pointer to int or (int *) and according to pointer arithmetic when an integer is added to a pointer we get the address of the next element of same base type. So In the above example my_arr points to the address of the first element, my_arr+1 points to the address of the second element, my_arr + 2 points to the address of the third element and so on. Therefore we can conclude that:
my_arr is same as &my_arr[0] my_arr + 1 is same as &my_arr[1] my_arr + 2 is same as &my_arr[2] my_arr + 3 is same as &my_arr[3] my_arr + 4 is same as &my_arr[4]
In general (my_arr + i) is same as writing &my_arr[i] .
Now we know how to get the address of each element of the array, by using indirection operator ( * ) we can get the value at the address. If we dereference my_arr then we get the first element of the array i.e *my_arr . Similarly, *(my_arr + 1) will return the second element of the array and so on.
*(my_arr) is same as my_arr[0] *(my_arr + 1) is same as my_arr[1] *(my_arr + 2) is same as my_arr[2] *(my_arr + 3) is same as my_arr[3] *(my_arr + 4) is same as my_arr[4]
In general *(my_arr+i) is same as writing my_arr[i] .
The following program prints value and address of array elements using pointer notation.
Assigning 1-D array to a Pointer variable #
Yes, you can assign a 1-D array to a pointer variable. Consider the following example:
Now you can use pointer p to access address and value of each element in the array. It is important to note that assignment of a 1-D array to a pointer to int is possible because my_arr and p are of the same base type i.e pointer to int . In general (p+i) denotes the address of the ith element and *(p+i) denotes the value of the ith element.
There are some differences between the name of the array (i.e my_arr ) and pointer variable (i.e p ). The name of the array is a constant pointer hence you can't alter it to point to some other memory location. You can’t assign some other address to it nor you can apply increment/decrement operator like you do in a pointer variable.
However, p is an ordinary pointer variable, so you can apply pointer arithmetic and even assign a new address to it.
The following program demonstrates how you can access values as address of elements of a 1-D array by assigning it to a pointer variable.
Load Comments
- Intro to C Programming
- Installing Code Blocks
- Creating and Running The First C Program
- Basic Elements of a C Program
- Keywords and Identifiers
- Data Types in C
- Constants in C
- Variables in C
- Input and Output in C
- Formatted Input and Output in C
- Arithmetic Operators in C
- Operator Precedence and Associativity in C
- Assignment Operator in C
- Increment and Decrement Operators in C
- Relational Operators in C
- Logical Operators in C
- Conditional Operator, Comma operator and sizeof() operator in C
- Implicit Type Conversion in C
- Explicit Type Conversion in C
- if-else statements in C
- The while loop in C
- The do while loop in C
- The for loop in C
- The Infinite Loop in C
- The break and continue statement in C
- The Switch statement in C
- Function basics in C
- The return statement in C
- Actual and Formal arguments in C
- Local, Global and Static variables in C
- Recursive Function in C
- One dimensional Array in C
- One Dimensional Array and Function in C
- Two Dimensional Array in C
- Pointer Basics in C
- Pointer Arithmetic in C
- Pointers and 2-D arrays
- Call by Value and Call by Reference in C
- Returning more than one value from function in C
- Returning a Pointer from a Function in C
- Passing 1-D Array to a Function in C
- Passing 2-D Array to a Function in C
- Array of Pointers in C
- Void Pointers in C
- The malloc() Function in C
- The calloc() Function in C
- The realloc() Function in C
- String Basics in C
- The strlen() Function in C
- The strcmp() Function in C
- The strcpy() Function in C
- The strcat() Function in C
- Character Array and Character Pointer in C
- Array of Strings in C
- Array of Pointers to Strings in C
- The sprintf() Function in C
- The sscanf() Function in C
- Structure Basics in C
- Array of Structures in C
- Array as Member of Structure in C
- Nested Structures in C
- Pointer to a Structure in C
- Pointers as Structure Member in C
- Structures and Functions in C
- Union Basics in C
- typedef statement in C
- Basics of File Handling in C
- fputc() Function in C
- fgetc() Function in C
- fputs() Function in C
- fgets() Function in C
- fprintf() Function in C
- fscanf() Function in C
- fwrite() Function in C
- fread() Function in C
Recent Posts
- Machine Learning Experts You Should Be Following Online
- 4 Ways to Prepare for the AP Computer Science A Exam
- Finance Assignment Online Help for the Busy and Tired Students: Get Help from Experts
- Top 9 Machine Learning Algorithms for Data Scientists
- Data Science Learning Path or Steps to become a data scientist Final
- Enable Edit Button in Shutter In Linux Mint 19 and Ubuntu 18.04
- Python 3 time module
- Pygments Tutorial
- How to use Virtualenv?
- Installing MySQL (Windows, Linux and Mac)
- What is if __name__ == '__main__' in Python ?
- Installing GoAccess (A Real-time web log analyzer)
- Installing Isso
- Overview of C
- Features of C
- Install C Compiler/IDE
- My First C program
- Compile and Run C program
- Understand Compilation Process
- C Syntax Rules
- Keywords and Identifier
- Understanding Datatypes
- Using Datatypes (Examples)
- What are Variables?
- What are Literals?
- Constant value Variables - const
- C Input / Output
- Operators in C Language
- Decision Making
- Switch Statement
- String and Character array
- Storage classes
C Functions
- Introduction to Functions
- Types of Functions and Recursion
- Types of Function calls
- Passing Array to function
C Structures
- All about Structures
- Pointers concept
- Declaring and initializing pointer
- Pointer to Pointer
Pointer to Array
Pointer to Structure
Pointer Arithmetic
- Pointer with Functions
C File/Error
- File Input / Output
- Error Handling
- Dynamic memory allocation
- Command line argument
- 100+ C Programs with explanation and output
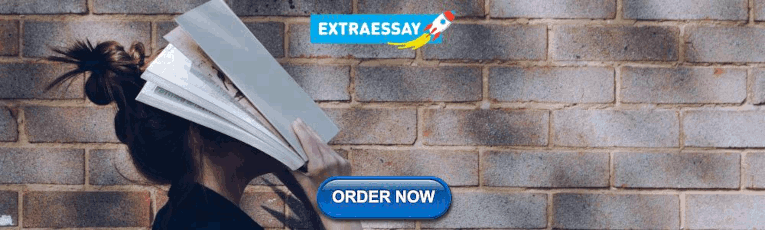
Pointer and Arrays in C
Before you start with Pointer and Arrays in C, learn about these topics in prior:
Pointer in C
When an array in C language is declared, compiler allocates sufficient memory to contain all its elements. Its base address is also allocated by the compiler.
Declare an array arr ,
Suppose the base address of arr is 1000 and each integer requires two bytes, the five elements will be stored as follows:
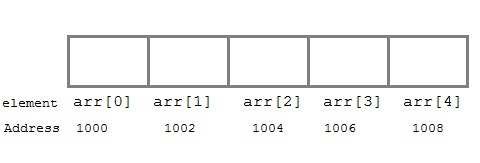
Variable arr will give the base address, which is a constant pointer pointing to arr[0] . Hence arr contains the address of arr[0] i.e 1000 .
arr has two purpose -
- It is the name of the array
- It acts as a pointer pointing towards the first element in the array.
arr is equal to &arr[0] by default
For better understanding of the declaration and initialization of the pointer - click here. and refer to the program for its implementation.
- You cannot decrement a pointer once incremented. p-- won't work.
Use a pointer to an array , and then use that pointer to access the array elements. For example,
is same as:
Pointer to Multidimensional Array
Let's see how to make a pointer point to a multidimensional array. In a[i][j] , a will give the base address of this array, even a + 0 + 0 will also give the base address, that is the address of a[0][0] element.
Pointer and Character strings
Pointer is used to create strings. Pointer variables of char type are treated as string.
The above code creates a string and stores its address in the pointer variable str . The pointer str now points to the first character of the string "Hello".
- The string created using char pointer can be assigned a value at runtime .
- The content of the string can be printed using printf() and puts() .
- str is a pointer to the string and also name of the string. Therefore we do not need to use indirection operator * .
Array of Pointers
Pointers are very helpful in handling character arrays with rows of varying lengths.
In the second approach memory wastage is more, hence it is preferred to use pointer in such cases.
Suggested Tutorials:
Pointers with Function
Pointer to Array Program
- ← Prev
- Next →
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
C Multidimensional Arrays
Pass arrays to a function in C
C Programming Pointers
Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
- C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Find Largest Element in an Array
- Calculate Average Using Arrays
- Access Array Elements Using Pointer
- Add Two Matrices Using Multi-dimensional Arrays
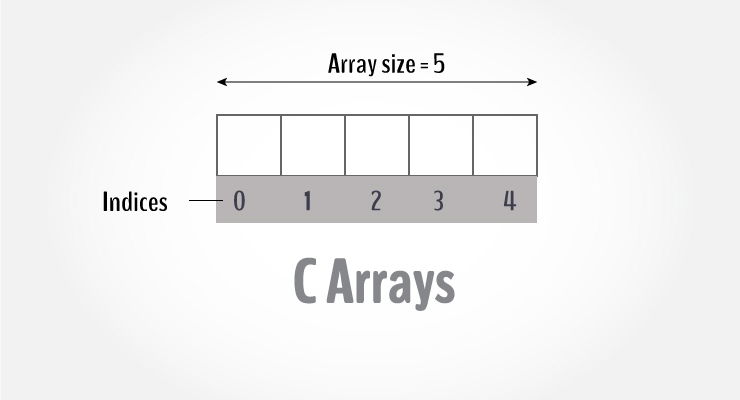
An array is a variable that can store multiple values. For example, if you want to store 100 integers, you can create an array for it.
How to declare an array?
For example,
Here, we declared an array, mark , of floating-point type. And its size is 5. Meaning, it can hold 5 floating-point values.
It's important to note that the size and type of an array cannot be changed once it is declared.
Access Array Elements
You can access elements of an array by indices.
Suppose you declared an array mark as above. The first element is mark[0] , the second element is mark[1] and so on.
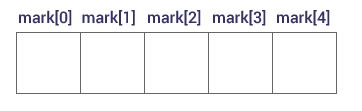
Few keynotes :
- Arrays have 0 as the first index, not 1. In this example, mark[0] is the first element.
- If the size of an array is n , to access the last element, the n-1 index is used. In this example, mark[4]
- Suppose the starting address of mark[0] is 2120d . Then, the address of the mark[1] will be 2124d . Similarly, the address of mark[2] will be 2128d and so on. This is because the size of a float is 4 bytes.
How to initialize an array?
It is possible to initialize an array during declaration. For example,
You can also initialize an array like this.
Here, we haven't specified the size. However, the compiler knows its size is 5 as we are initializing it with 5 elements.
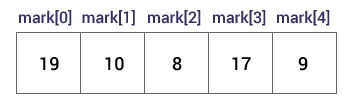
Change Value of Array elements
Input and output array elements.
Here's how you can take input from the user and store it in an array element.
Here's how you can print an individual element of an array.
Example 1: Array Input/Output
Here, we have used a for loop to take 5 inputs from the user and store them in an array. Then, using another for loop, these elements are displayed on the screen.
Example 2: Calculate Average
Here, we have computed the average of n numbers entered by the user.
Access elements out of its bound!
Suppose you declared an array of 10 elements. Let's say,
You can access the array elements from testArray[0] to testArray[9] .
Now let's say if you try to access testArray[12] . The element is not available. This may cause unexpected output (undefined behavior). Sometimes you might get an error and some other time your program may run correctly.
Hence, you should never access elements of an array outside of its bound.
Multidimensional arrays
In this tutorial, you learned about arrays. These arrays are called one-dimensional arrays.
In the next tutorial, you will learn about multidimensional arrays (array of an array) .
Table of Contents
- C Arrays (Introduction)
- Declaring an Array
- Access array elements
- Initializing an array
- Change Value of Array Elements
- Array Input/Output
- Example: Calculate Average
- Array Elements Out of its Bound
Video: C Arrays
Sorry about that.
Related Tutorials
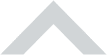
Fill out the form in 2 simple steps below:
Your contact information:, desired license type:.

Your message has been sent. We will email you at
If you haven't received our response, please do the following: check your Spam/Junk folder and click the "Not Spam" button for our message. This way, you won't miss messages from our team in the future.

Error on verge of extinction, or why I put if (x = 42) in Red List of C & C++ bugs
If we ask a programmer what bugs are the most common in C and C++ code, they'll name a null pointer dereference, undefined behavior, array overrun, and other typical error patterns. They may name an accidental assignment in condition as well. However, let's see if this error is common today.
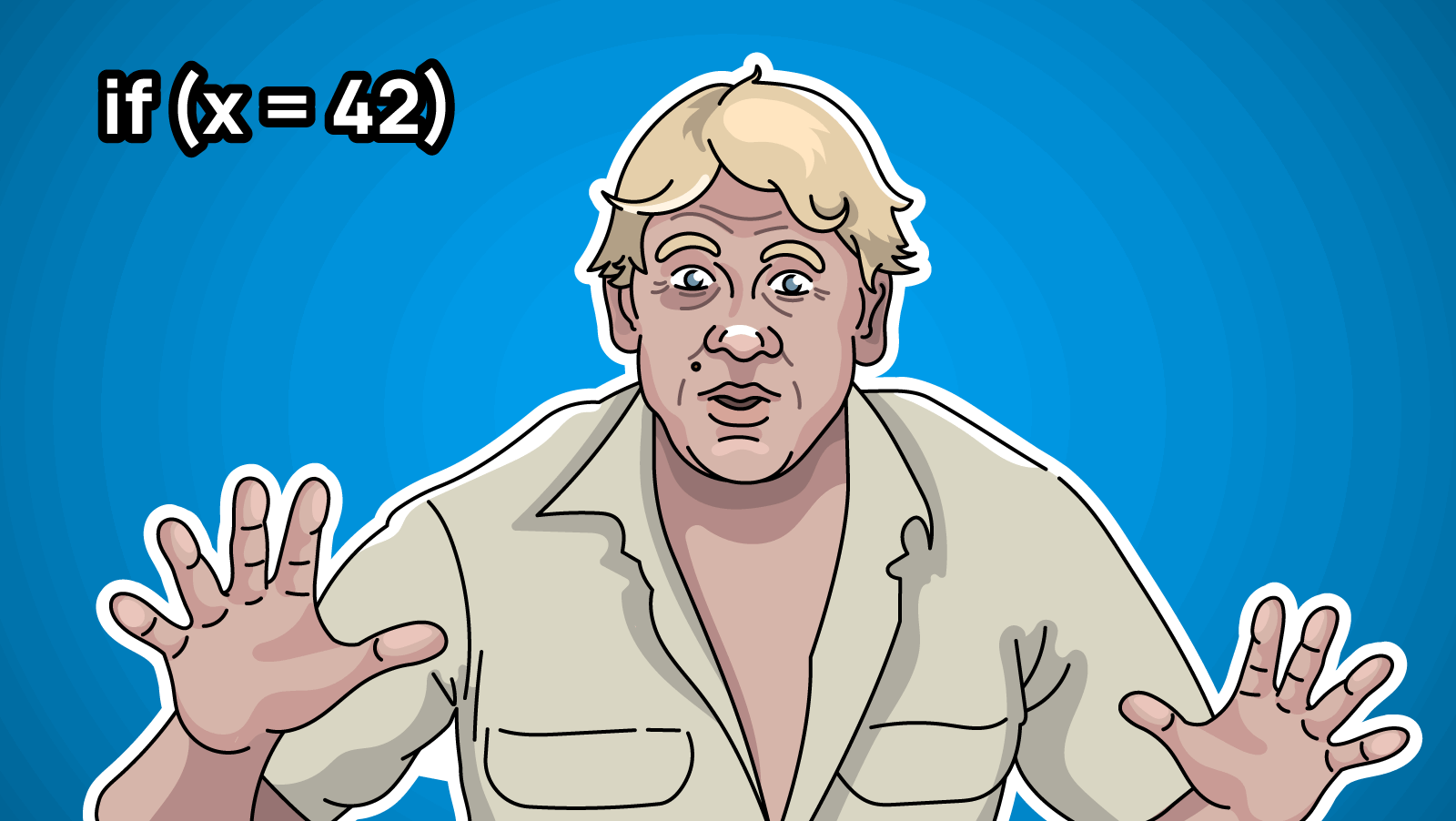
C and C++ use the = symbol for the assignment operator and == for the comparison operator. That's why we may make typos when we write = instead of == and get compliable but incorrectly operating code.
These errors look like this:
There are two unpleasant things going on at once:
- The condition is always true or false, depending on what is assigned to the variable.
- The correct value has been lost. If the abcd variable is used elsewhere, its value will be invalid (corrupted).
These errors are simple yet common and notorious among programmers. Although devs don't film movies about bugs, they make memes about them.
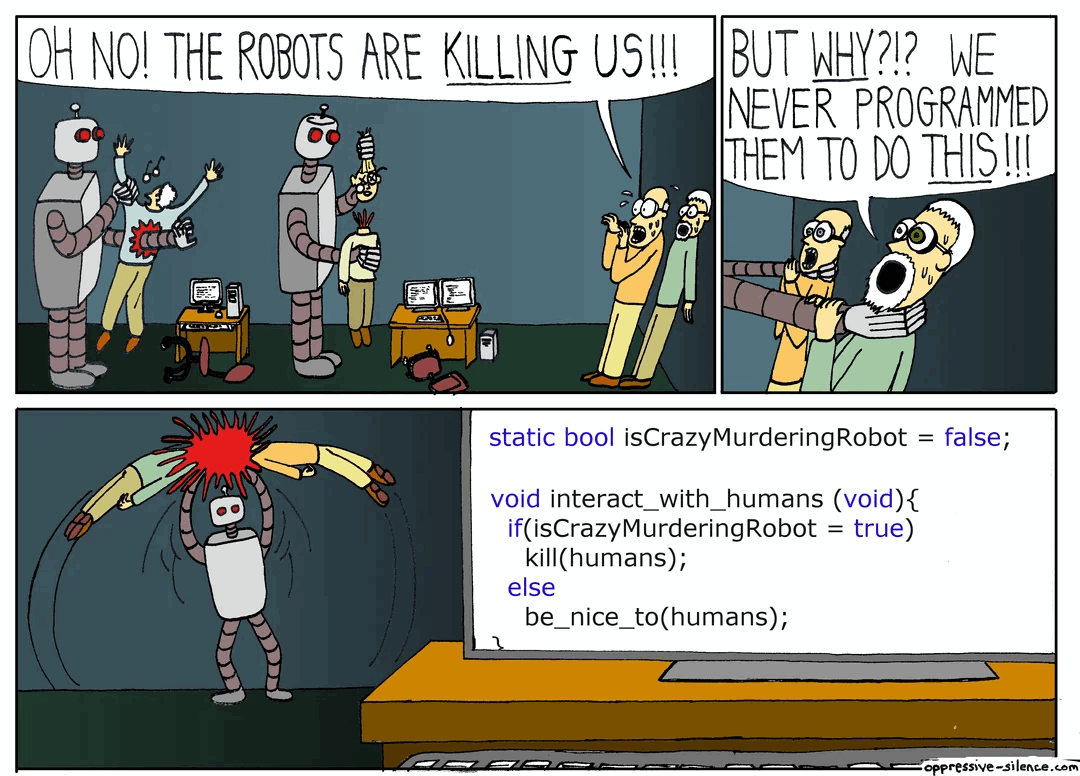
The bugs look like they're from these memes, though. For example, I've found the following code in the AMT SDK project:
Because of this bug, developers invented the Yoda notation : a programming style where the constant is placed on the left side of the comparison operator.
This style was meant to prevent a typo. If a programmer writes = instead of == , the code won't compile.
However, this notation hasn't caught on in real cases. At least, I've seen it very rarely in open-source projects.
Btw, I don't like this coding style too. First, it makes the code a bit more complicated: you look at the constant and only then realize what the code is about and what is checked.
Second, the coding style won't help if two variables are compared with each other:
In my opinion, it's better to declare all variables that are further used read-only as const . This reduces the likelihood that typos or failed refactoring will cause accidental modification.
Sorry, I got a little distracted. In the beginning, I talked about the rarity of such bugs and the Red List. Let's get back to the main topic of the article.
Once I was preparing for a conference talk, where I've discussed how expectations to find errors in code align with reality. To prove it, I researched our collection of bugs found over several years of writing articles about open-source project checking.
Sometimes, expectations match reality. For example, programmers suppose that there are many bugs in C and C++ code related to null pointer dereference and array overrun. Indeed, such bugs are common.
Sometimes, expectations don't match reality. A programmer is very likely to say something about division by zero, but there are few such errors in reality. At least if we compare it to many other bugs.
Of course, I couldn't help but look at assignment errors in conditions. It seems like there should be a mountain of them.
But, surprise, surprise, that such errors are quite rare in open-source projects! We only found 14 cases . Fun fact, but the last error we wrote out was in 2016 !
It's amazing how high expectations are, and there are no errors!
Let's dig deeper into the statistics again. With the V559 diagnostic rule, the PVS-Studio analyzer detects errors related to an assignment in a condition. The rule was introduced in PVS-Studio 4.12 on February 7, 2011.
For some time, the analyzer found these errors, but gradually they became fewer and fewer. The last one we found was in 2016. Although we still write many articles about checking open-source projects, it has been eight years since we last saw errors like that.
I think this is an amazing and nice example of how some kinds of errors go extinct due to development of programmers' tools. I don't think that such errors have died off because everyone is aware of them, and now developers write code more carefully. As our experience shows, typos are still as common as they're 10 years ago.
So, it looks like the tools affect it. At first, static analyzers detected such errors. Then the compilers caught it up and started to detect it too. Generally, there are no compilers that will ignore an assignment in a condition. If we write such code now, and we'll see warnings all around: an IDE highlights the code, compilers and static analyzers issue warnings. We just can't help but see the error. Gorgeous!
Of course, I'm not saying that there are no programs with these bugs at all. We encounter such programs, but they're very rare. In addition, in my experience, we haven't seen them in a long time when checking different projects.
By the way, doesn't it mean that static analyzers will become unnecessary as compilers issue more and more warnings? No, of course not. The analyzer development doesn't stand still, either. They are working on to detect more and more complex and intricate errors. Analyzers comprises such technologies as interprocedural and intermodular analysis, data-flow analysis, symbolic execution, taint analysis, and so on. In addition, they can afford to run longer and use more memory than compilers. This means we can go deeper and analyze more complex data.
We may say that static analyzers set the direction of compilers development in terms of detecting bugs. We've even noticed how diagnostic rules from PVS-Studio move into compilers. Our team is proud that PVS-Studio is a source of inspiration for new things coming out. Let good diagnostic rules influence the tools. This way, there will be fewer errors. Meanwhile, we'll write more rules. After all, someone has to find bugs in compilers :)
Here's an example of the typo in the LLVM code — the PVS-Studio analyzer issued the following warning: V547 Expression 'FirstSemiPos == std::string::npos' is always false. UnicodeNameMappingGenerator.cpp 46.
If you wish, you can look at other bugs in compilers .
Finally, let's look back at the assignment in the condition:
What if the code author really wants to assign and check at the same time?
The first option: if we write in C++ and we need the variable only inside the body of the if/while instruction, we can declare it directly in the condition.
Now it's obvious, that there's a variable declaration with initialization and subsequent check.
The second option: almost all developers of compilers and code analyzers have an unspoken agreement that additional parentheses are a sign that everything is OK.
If a programmer has written the code like this, it almost sounds like, "I know what I am doing, this code is correct". In this case, compilers and analyzers don't issue the warning.
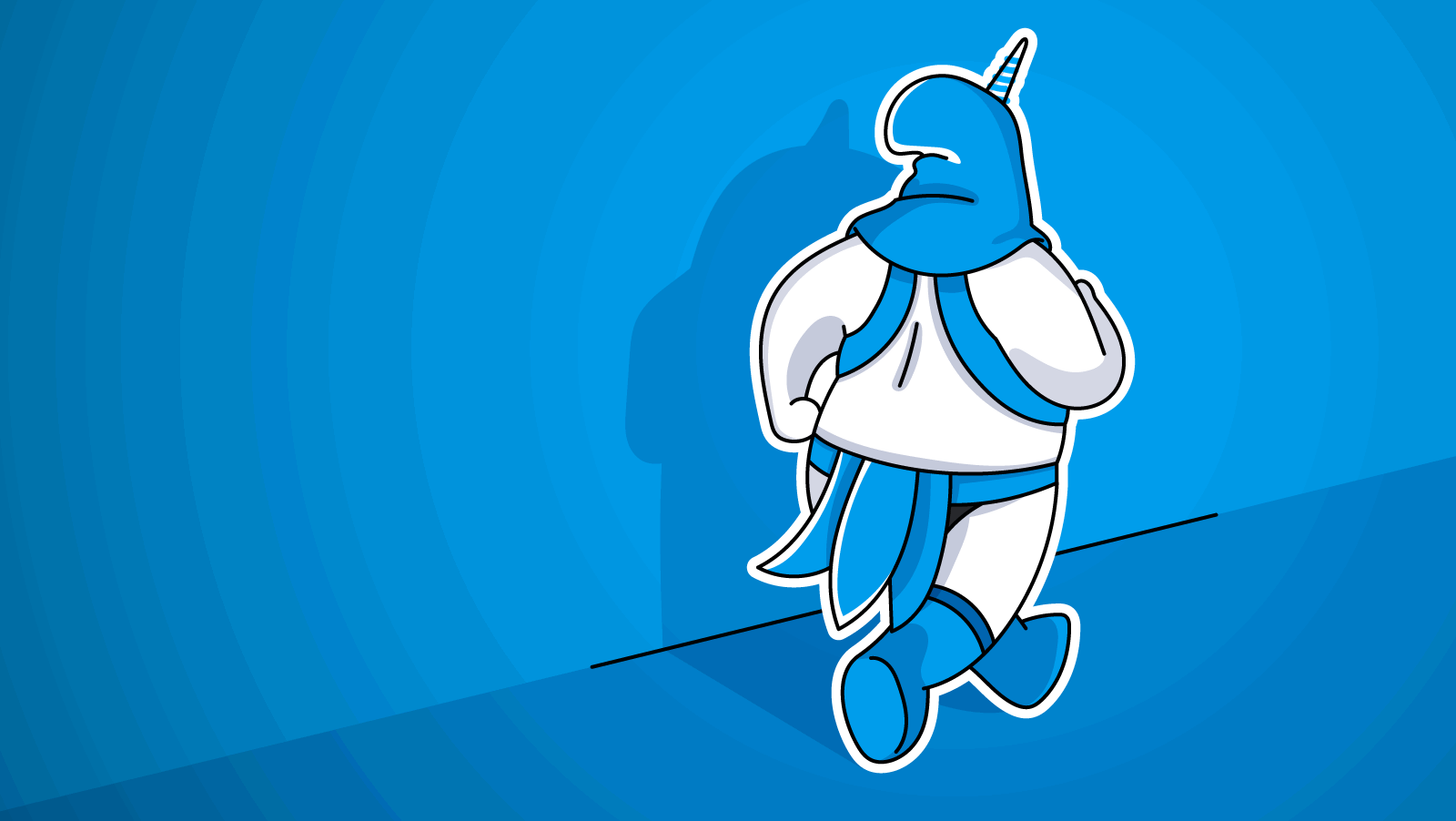
Thank you for your attention. The Skeletor Unicorn will be back soon with some new, mind-boggling information.
We can email you a selection of our best articles once a month
Posts: articles
Date: May 29 2024
Author: Alexey Smolskas
Date: May 23 2024
Author: Andrey Karpov
Date: May 16 2024
Date: May 14 2024
Author: Taras Shevchenko
Date: May 07 2024
Get notifications about comments to this article
You subscribed to the comments
Comments ( 0 )
Want to try pvs‑studio for free.
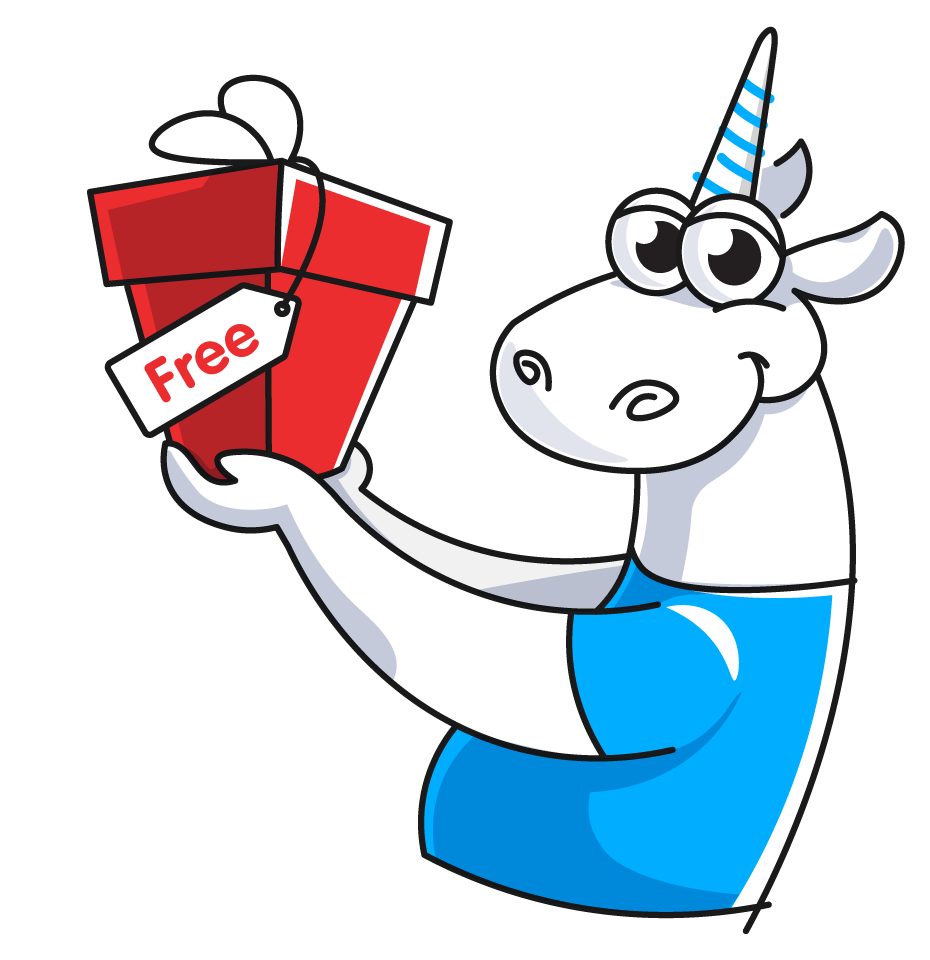
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Pointer to an Array in C++
- Pointer vs Array in C
- Pointers vs Array in C++
- How to Sort an Array in C++?
- How to Initialize an Array in C++?
- Pointer to Arrays in Objective-C
- Array of Pointers to Strings in C++
- Array of Pointers to Strings in C
- array::size() in C++ STL
- How to Find the Length of an Array in C++?
- How to Declare an Array in C++?
- How to Find Largest Number in an Array in C++?
- Arrays and Strings in C++
- How to Resize an Array of Strings in C++?
- void Pointer in C++
- Reference to Array in C++
- 'this' pointer in C++
- Data type of a Pointer in C++
- Pointer to an Array | Array Pointer
- Array of Pointers in C
Pointers in C++ are variables that store the address of another variable while arrays are the data structure that stores the data in contiguous memory locations. In C++, we can manipulate arrays by using pointers to them. These kinds of pointers that point to the arrays are called array pointers or pointers to arrays.
In this article, we will discuss what is a pointer to an array, how to create it and what are its applications in C++.
Before we start discussing pointers to arrays, let’s first recall what are pointer and Arrays:
What are Arrays?
An array is a collection of elements of similar data types that are stored in contiguous memory locations. The elements are of the same data type. The name of the array points to the memory address of its first element.
The syntax to declare an array in C++ is given below:
What are Pointers?
Pointers are variables that store the memory addresses of other variables or any data structures in C++.
The syntax to declare a pointer is given below:
Pointers to an Array in C++
Pointers to an array is the pointer that points to the array. It is the pointer to the first element of the pointer instead of the whole array but we can access the whole array using pointer arithmetic.
Syntax to Declare Array Pointer
As you can see, this declaration is similar to the pointer to a variable. It is again because this pointer points to the first element of the array.
Note: The array name is also a pointer to its first element so we can also declare a pointer to the array as: type *ptr_name = arr_name;
Examples of Pointer to an Array in C++
Example 1: program to demonstrate that array name is the pointer to its first element.
From the above code, we can see that the pointer “ptr” and “arr” point to the same memory address and to the same element.
Example 2: Program to Traverse Whole Array using Pointer
Pointers to multidimensional arrays in c++.
Pointers to multidimensional arrays are declared in a different way as compared to single-dimensional arrays. Here, we also need to mention the information about the dimensions and their size in the pointer declaration. Also, we need to take care of operator precedence of array subscript operator [] and dereference operator * as a little mistake can lead to the creation of whole different pointer.
Syntax to Declare Pointer to 2D Array
For example,
Here, int arr[3][4] declares an array with 3 rows (outer arrays) and 4 columns (inner arrays) of integers. If we declare an array as int arr[n][m] then n is the number of rows or we can say number of arrays it stores and m denotes the number of element each array(row) have in integers as int data type is declared.
Syntax to Declare Pointer to 3D Array
For example, for array int arr[1][2][3], we can delcare a pointer to it as:
Examples of Pointer to Multidimensional Arrays
Example 1: accessing array elements using pointer., example 2: program to pass 3d array to functions, applications of pointers to arrays in c++.
Following are some main applications of pointers to arrays in C++:
- Passing Arrays to Functions : Pointers allow us to pass arrays to functions without copying the entire array. This can improve performance for large arrays.
- Dynamic Memory Allocation : Pointers are used to allocate and deallocate memory dynamically at runtime using functions like new and delete. We can use pointers to arrays to create dynamic arrays.
- Accessing Array Elements : Pointers can be used to access elements of an array in an efficient manner.
Please Login to comment...
Similar reads.
- cpp-pointer
- Geeks Premier League 2023
- Geeks Premier League
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
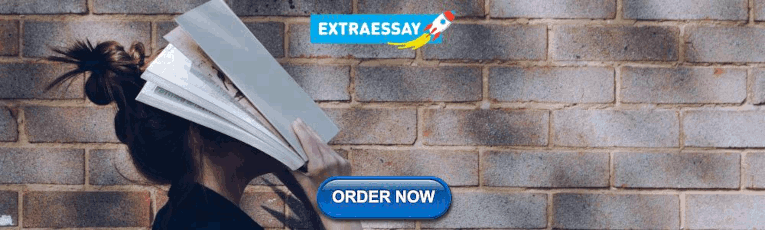
IMAGES
VIDEO
COMMENTS
13 3. You can't assign an array to a pointer. But you can assign a pointer to a pointer. Like uint8_t ptr = str; - Some programmer dude. Oct 10, 2021 at 11:23. 1. As for the problem for your current code, you declare rcm to be an array of pointers, not a pointer to an array. The type of &str is uint8_t (*)[11] (and yes, the size is part of ...
data_type (*var_name)[size_of_array];. Here: data_type is the type of data that the array holds.; var_name is the name of the pointer variable.; size_of_array is the size of the array to which the pointer will point.; Example. int (*ptr)[10]; Here ptr is pointer that can point to an array of 10 integers. Since subscript have higher precedence than indirection, it is necessary to enclose the ...
An array name is a constant pointer to the first element of the array. Therefore, in this declaration, int balance[5]; balance is a pointer to &balance[0], which is the address of the first element of the array.. Example. In this code, we have a pointer ptr that points to the address of the first element of an integer array called balance.. #include <stdio.h> int main(){ int *ptr; int balance ...
Pointers and array names can pretty much be used interchangeably; however, there are exceptions. You cannot assign a new pointer value to an array name. The array name will always point to the first element of the array. In the function returnSameIfAnyEquals, you could however assign a new value to workingArray, as it is just a pointer to the ...
In most contexts, array names decay to pointers. In simple words, array names are converted to pointers. That's the reason why you can use pointers to access elements of arrays. However, you should remember that pointers and arrays are not the same. There are a few cases where array names don't decay to pointers.
2) You can also use array name to initialize the pointer like this: p = var; because the array name alone is equivalent to the base address of the array. val==&val [0]; 3) In the loop the increment operation (p++) is performed on the pointer variable to get the next location (next element's location), this arithmetic is same for all types of ...
Pointers to Arrays — char *envp[] One tricky part of CS 107 for many students is getting comfortable with what the memory looks like for pointers to arrays, particularly when the arrays themselves are filled with pointers. This week's assignment has a good example: envp. With arrays: 1. Always draw a picture!!!1! Make up the
It is generally used in C Programming when we want to point at multiple memory locations of a similar data type in our C program. We can access the data by dereferencing the pointer pointing to it. Syntax: pointer_type *array_name [array_size]; Here, pointer_type: Type of data the pointer is pointing to. array_name: Name of the array of pointers.
int *ptr = arr; creates a pointer ptr that points to the first element of the array arr. In C, arrays are zero-indexed, so the first element can also be accessed using arr + 0. so that means the first element's address is the same as the array's address (i.e., arr or arr + 0).
Now you can use pointer p to access address and value of each element in the array. It is important to note that assignment of a 1-D array to a pointer to int is possible because my_arr and p are of the same base type i.e pointer to int.In general (p+i) denotes the address of the ith element and *(p+i) denotes the value of the ith element.. There are some differences between the name of the ...
4. In this program, the elements are stored in the integer array data[]. Then, the elements of the array are accessed using the pointer notation. By the way, data[0] is equivalent to *data and &data[0] is equivalent to data. data[1] is equivalent to *(data + 1) and &data[1] is equivalent to data + 1. data[2] is equivalent to *(data + 2) and ...
For better understanding of the declaration and initialization of the pointer - click here. and refer to the program for its implementation. NOTE: You cannot decrement a pointer once incremented. p--won't work. Pointer to Array. Use a pointer to an array, and then use that pointer to access the array elements. For example,
Explanation of the program. int* pc, c; Here, a pointer pc and a normal variable c, both of type int, is created. Since pc and c are not initialized at initially, pointer pc points to either no address or a random address. And, variable c has an address but contains random garbage value.; c = 22; This assigns 22 to the variable c.That is, 22 is stored in the memory location of variable c.
It is said to be good practice to assign NULL to the pointers currently not in use. 7. Void Pointer. The Void pointers in C are the pointers of type void. It means that they do not have any associated data type. ... C Pointers and Arrays. In C programming language, pointers and arrays are closely related. An array name acts like a pointer ...
The pointer to a pointer in C is used when we want to store the address of another pointer. The first pointer is used to store the address of the variable. And the second pointer is used to store the address of the first pointer. That is why they are also known as double-pointers. We can use a pointer to a pointer to change the values of normal ...
You need to create an int variable somewhere in memory for the int * variable to point at. Your second example does this, but it does other things that aren't relevant here. Here's the simplest thing you need to do: int main(){. int variable; int *ptr = &variable; *ptr = 20; printf("%d", *ptr); return 0;
Access Array Elements. You can access elements of an array by indices. Suppose you declared an array mark as above. The first element is mark[0], the second element is mark[1] and so on.. Declare an Array Few keynotes:
C and C++ use the = symbol for the assignment operator and == for the comparison operator. ... For example, programmers suppose that there are many bugs in C and C++ code related to null pointer dereference and array overrun. Indeed, such bugs are common.
An array is not a pointer (although a name of an array often decays to a pointer to its first element). To make the above code to work, you can declare a as a pointer: int *a;. The print function takes an int* (or a decayed array) anyway.
Pointers to an Array in C++. Pointers to an array is the pointer that points to the array. It is the pointer to the first element of the pointer instead of the whole array but we can access the whole array using pointer arithmetic. Syntax to Declare Array Pointer. // for array of type: type arr_name[size];