Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
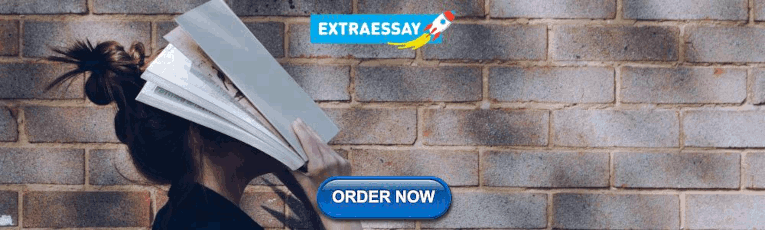
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Here are the assignment answers you need.
vklsaravanan/nptel-programmingInJava
Folders and files, repository files navigation.

Programming In Java
Exercise1_1 - Complete the code segment to find the perimeter and area of a circle given a value of radius. Exercise1_2 - Complete the code segment to find the largest among three numbers x,y, and z. You should use if-then-else construct in Java. Exercise1_3 - Consider First n even numbers starting from zero(0).Complete the code segment to calculate sum of all the numbers divisible by 3 from 0 to n. Print the sum. Exercise1_4 - Complete the code segment to check whether the number is an Armstrong number or not. Exercise1_5 - Complete the code segment to help Ragav , find the highest mark and average mark secured by him in "s" number of subjects.
Exercise2_1 - Complete the code segment to call the method print() of class Student first and then call print() method of class School. Exercise2_2 - Complete the code segment to call the method print() of class given class Printer to print the following. Exercise2_3 - Complete the code segment to call print() method of class Question by creating a method named ‘studentMethod()’. Exercise2_4 - Complete the code segment to call default constructor first and then any other constructor in the class. Exercise2_5 - Complete the code segment to debug / complete the program which is intended to print 'NPTEL JAVA'.
Excercise3_1 - This program is related to the generation of Fibonacci numbers.>For example: 0,1, 1,2, 3,5, 8, 13,… is a Fibonacci sequence where 13 is the 8th Fibonacci number. Exercise3_2 - Define a class Point with two fields x and y each of type double. Also, define a method distance(Point p1, Point p2) to calculate the distance between points p1 and p2 and return the value in double. Exercise3_3 - A class Shape is defined with two overloading constructors in it. Another class Test1 is partially defined which inherits the class Shape. The ..., Exercise3_4 - This program to exercise the call of static and non-static methods. A partial code is given defining two methods, namely sum( ) and multiply ( ). You......, Exercise3_5 - Complete the code segment to swap two numbers using call by object reference.
Exercise4_1 - Complete the code segment to execute the following program successfully. You should import the correct package(s) and/or class(s) to complete the code. Exercise4_2 - Complete the code segment to print the current year. Your code should compile successfully. Exercise4_3 - The program in this assignment is attempted to print the following output: Exercise4_4 - Complete the code segment to call the default method in the interface First and Second. Exercise4_5 - Modify the code segment to print the following output.
Exercise5_1 - An interface Number is defined in the following program. You have to declare a class A,which will implement the interface Number. Note that the method findSqr(n) will return the square of the number n. Exercise5_2 - An interface Number is defined in the following program. You have to declare a class A,which will implement the interface Number. Note that the method findSqr(n) will return the square of the number n. Exercise5_3 - Complete the code segment to catch the ArithmeticException in the following, if any. On the occurrence of such an exception, your program should print “Exception caught: Division by zero.” If there is no such exception, it will print the result of division operation on two integer values. Exercise5_4 - In the following program, an array of integer data to be initialized. During the initialization, if a user enters a value other than integer value, then it will throw InputMismatchException.., Exercise5_5 - In the following program, there may be multiple exceptions. You have to complete the code using only one try-catch block to handle all the possible exceptions.
Exercise6_1 - Complete the code segment to print the following using the concept of extending the Thread class in Java: Exercise6_2 - In the following program, a thread class Question62 is created using the Runnable interface Complete the main() to create a thread object of the class Question62 and run the thread. It should print the output as given below. Exercise6_3 - Given a snippet of code, add necessary codes to print the following: -----------------OUTPUT------------------- Name of thread 't1':Thread-0 Name of thread 't2':Thread-1 New name of thread 't1':Week 6 Assignment Q5 New name of thread 't2':Week 6 Assignment Q5 New ------------------------------------------------- Exercise6_4 - Execution of two or more threads occurs in a random order. The keyword 'synchronized' in Java is used to control the execution of thread .., Exercise6_5 - In the following program, a thread class Question62 is created using the Runnable interface Complete the main() to create a thread object of the class Question62 and run the thread. It should print the output as given below.
Exercise7_1 - Complete the following code fragment to read three integer values from the keyboard and find the sum of the values. Declare a variable "sum" of type int and store the result in it. Exercise7_2 - Complete the code segment to catch the exception in the following, if any. On the occurrence of such an exception, your program should print “Please enter valid data” .If there is no such exception, it will print the "square of the number". Exercise7_3 - A byte char array is initialized. You have to enter an index value"n". According to index your program will print the byte and its corresponding char value. Complete the code segment to catch the exception in the.., Exercise7_4 - The following program reads a string from the keyboard and is stored in the String variable "s1".., Exercise7_5 -A string "s1" is already initialized. You have to read the index "n" from..,
Exercise8_1 - Write a program which will print a pyramid of " " 's of height "n" and print the number of " " 's in the pyramid. Exercise8_2 - Write a program which will print a pascal pyramid of " " 's of height "l" . Exercise8_3 - Write a program which will print a pyramid of "numbers" 's of height "n" and print the sum of all number's in the pyramid. Exercise8_4 - Write a program to print symmetric Pascal's triangle of " " 's of height "l" of odd length . If input "l" is even then your program will print "Invalid line number". Exercise8_5 - Write a program to display any digit(n) from 0-9 represented as a "7 segment display".
Exercise9_1 - Complete the code to develop a BASIC CALCULATOR that can perform operations like Addition, Subtraction, Multiplication and Division. Exercise9_2 - Complete the code to develop an ADVANCED CALCULATOR that emulates all the functions of the GUI Calculator as shown in the image. Exercise9_3 - Complete the code to perform a 45 degree anti clock wise rotation with respect to the center of a 5 × 5 2D Array as shown below: Exercise9_4 - A program needs to be developed which can mirror reflect any 5 × 5 2D character array into its side-by-side reflection. Write suitable code to achieve this transformation as shown below: Exercise9_5 - Write suitable code to develop a 2D Flip-Flop Array with dimension 5 × 5, which replaces all input elements with values 0 by 1 and 1 by 0. An example is shown below:
Exercise10_1 - The following code needs some package to work properly. Write appropriate code to import the required package(s) in order to make the program compile and execute successfully. Exercise10_2 - Write the JDBC codes needed to create a Connection interface using the DriverManager class and the variable DB_URL. Check whether the connection is successful using 'isAlive(timeout) Exercise10_3 - Due to some mistakes in the below code, the code is not compiled/executable. Modify and debug the JDBC code to make it execute successfully. Exercise10_4 - Complete the code segment to rename an already created table named ‘PLAYERS’ into ‘SPORTS’. Exercise10_5 - Complete the code segment to rename an already created table named ‘PLAYERS’ into ‘SPORTS’.

Author @vklsaravanan
Connect with me:
- Java 100.0%
- Software Engineering Tutorial
- Software Development Life Cycle
- Waterfall Model
- Software Requirements
- Software Measurement and Metrics
- Software Design Process
- System configuration management
- Software Maintenance
- Software Development Tutorial
- Software Testing Tutorial
- Product Management Tutorial
- Project Management Tutorial
- Agile Methodology
- Selenium Basics
NPTEL (Swayam) Course Examination Experience
- GATE Examination Experience
- JEE-Mains Examination Experience
- IIT-JEE Examination Experience
- IB JIO Examination Experience 2023
- NIT Warangal Admission Experience
- TS EAMCET Exam Experience
- NPTEL Star Award Experience 2023
- NIT Silchar Admission Experience
- SRM Admission Experience
- Tata Elxsi Interview Experience (Off-Campus)
- B.Tech CSE Semester Exam Experience
- 12 Board Exam Preparation Experience
- EPAM Interview Experience (Off-Campus)
- NDA Exam Experience
- 10 Board Exam Preparation Experience
- NEET Coaching Experience
- IGDTUW Admission Experience
NPTEL is an acronym for National Programme on Technology Enhanced Learning which is an initiative by seven Indian Institutes of Technology (IIT Bombay, Delhi, Guwahati, Kanpur, Kharagpur, Madras, and Roorkee) and the Indian Institute of Science (IISc) for creating course contents in engineering and science.
Many students who dream of attending scholarly institutions, through NPTEL will have access to quality content from them.
There are courses on multiple streams both technical and non-technical. The courses are for 4 weeks, 8 weeks and 12 weeks usually.
I have taken many courses on NPTEL up until now but have taken exams for the following courses.
- An introduction to programming through: C++ 12 Weeks (by Professor Abhiram G Ranade)
- Programming in Java: 12 Weeks (by Professor Debasis Samanta)
- Data structures and algorithms using Java: 12 Weeks (by prof Debasis Samanta)
Introduction to programming through C++: The procedures for all the examinations were similar, here I will be talking about “An introduction to Programming through C++”
- The 12-week course was taught by Prof. Abhiram G. Ranade of the Computer Science and Engineering department at IIT Bombay.
- It is a core undergraduate course where each week new topics were covered very thoroughly. Each week has assignments consisting of 5 coding questions and 10 MCQs of that week’s subject.
- About the 10th-week applications for exams will be opened, and we will be asked to select our examination centers. The course is free to enroll but a fee of 1000/- is to be paid for the exam.
- Prior exams we will be notified to download hall tickets and should take a physical copy of it and an identity card along with us to the exam hall.
- The exam is for 3 hours, each of us will be assigned an individual computer. Exam will have mostly MCQs depending on the course and is for 100 marks. The questions are ranged from easy to hard and everything will be by the course itself.
Criteria to get a Certificate:
- Average assignment score = 25% of the average of best 8 assignments out of the total 12 assignments given in the course.
- Exam score = 75% of the proctored certification exam score out of 100
- Final score = Average assignment score + Exam score
You will be eligible for the certificate only if Average Assignment Score >= 10/25 & Exam score>= 30/75
An e certificate will be provided after the results are announced and the criteria’s fulfilled
Please Login to comment...
Similar reads.
- C++ Programs
- Data Structures
- Experienced
- Experiences
- Interview Experiences
- Programming Language
- Software Engineering
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- All Courses
- Privacy Policy

Swayam Solver
Learn Programming & Prepare for NPTEL Exams... Swayam Solver is your one-stop destination for NPTEL exam preparation.
NPTEL Programming in Java Jan 2024 Week 12
Week 12 : programming assignment 1.
Write a program that calculates the letter grade based on a numerical score entered by the user.
§ >=80 - A
§ 70-79 - B
§ 60-69 - C
§ 50-59 - D
§ 40-49 - P
§ <40 - F
Follow the naming convention as given in the main method of the suffix code.
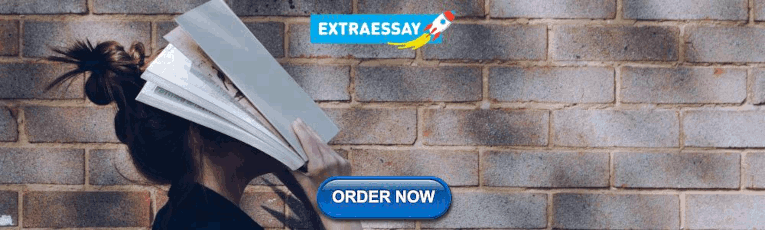
Week 12 : Programming Assignment 2
Write a program that validates a user's password based on the following criteria (in the following order):
§ 1. The password must be at least 8 characters long.
§ 2. The password must contain at least one uppercase letter (A-Z).
§ 3. The password must contain at least one lowercase letter (a-z).
§ 4. The password must contain at least one digit (0-9).
Take the following assumptions regarding the input:
§ The input will not contain any spaces
Your output should print the rule that it violates exactly as defined above.
If the password violates multiple rules then the first rule it violates should take priority .
For example:
Input:
Output:
Your password is invalid. The password must be at least 8 characters long.
Week 12 : Programming Assignment 3
Write a program that analyzes a given text and provides the following information:
§ Number of words: The total number of words in the text.
§ Longest word: The longest word found in the text.
§ Vowel count: The total number of vowels (a, e, i, o, u) in the text.
§ The input will only contain lowerspace characters[a-z] and spaces.
§ The last word will not end with a space nor with any special character.
You need to print exactly as shown in the test case.
Do not print anyting else while taking input.
Week 12 : Programming Assignment 4
Write a Java program to calculate the area of different shapes using inheritance.
Each shape should extend the abstact class Shape
Your task is to make the following classes by extending the Shape class:
§ Circle (use Math.PI for area calculation)
§ Rectangle
§ Square
Each Shape subclass should have these private variables apart from the private variables for storing sides or radius .
private String shapeType ; private double area ;
Week 12 : Programming Assignment 5
Implement two classes, Car and Bike , that implement the Vehicle interface. Each class should have appropriate attributes and constructors. Ensure that the start , accelerate , and brake methods are implemented correctly for each vehicle.
No comments:
Post a comment.
Keep your comments reader friendly. Be civil and respectful. No self-promotion or spam. Stick to the topic. Questions welcome.
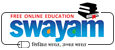
Programming in Java
Note: This exam date is subjected to change based on seat availability. You can check final exam date on your hall ticket.
Page Visits
Course layout, books and references, instructor bio.
Prof. Debasis Samanta
Course certificate.
- Assignment score = 25% of average of best 8 assignments out of the total 12 assignments given in the course.
- ( All assignments in a particular week will be counted towards final scoring - quizzes and programming assignments).
- Unproctored programming exam score = 25% of the average scores obtained as part of Unproctored programming exam - out of 100
- Proctored Exam score =50% of the proctored certification exam score out of 100

DOWNLOAD APP

SWAYAM SUPPORT
Please choose the SWAYAM National Coordinator for support. * :
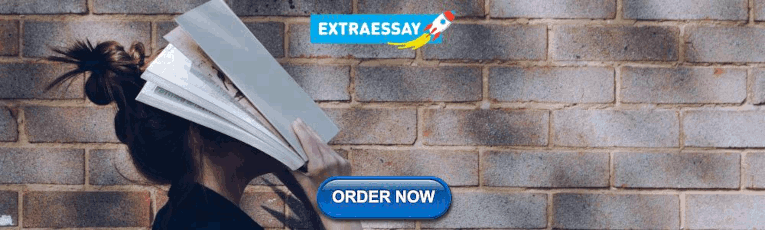
IMAGES
VIDEO
COMMENTS
Course layout. Week 1 : Overview of Object-Oriented Programming and Java. Week 2: Java Programming Elements. Week 3: Input-Output Handling in Java. Week 4: Encapsulation. Week 5: Inheritance. Week 6: Exception Handling. Week 7: Multithreaded Programming. Week 8: Java Applets and Servlets.
🌟 Welcome to my NPTEL Programming in Java repository! 🚀 This repository is a comprehensive collection of my solutions and detailed notes for the NPTEL Programming in Java course. It's designed for learners, Java enthusiasts, and anyone eager to delve into the world of Java programming. Resources
NPTEL: Exam Registration is open now for Jan 2022 courses! ... Programming in Java - Assignment-6 Solution Released Dear Participants, The Assignment-6 of Week- 6 Solution for the course Programming in Java has been released in the portal. Please go through the solution and in case of any doubt post your queries in the forum.
Java Week 6:Q2 In the following program, a thread class ThreadRun is created using the Runnable interface which prints "Thread using Runnable interface". Complete the main class to create a thread object of the class ThreadRun and run the thread, Java Week 6:Q3 A part of the Java program is given, which can be completed in many ways, for example using the concept of thread, etc. Follow the ...
Java Week 1:Q1 To find the perimeter and area of a circle given a value of radius. Java Week 1:Q2 To find the largest among three numbers x, y, and z. Java Week 1:Q3 Consider First n even numbers starting from zero (0) and calculate sum of all the numbers divisible by 3 from 0 to n. Print the sum. Java Week 1:Q4 To check whether the number is ...
The assignment 0 for the course Programming In Java has been released. This assignment is based on a prerequisite of the course. Kindly note that marks obtained in this assignment will not be considered for the final assessment. You can find the assignment under Week 0 unit on the left-hand side of your screen.
NPTEL-Programming-in-Java Assignment Solutions with Explanations and Course Guide. This Repository has the ultimate guide to crack the exam of Programming in Java Course taught by Prof.Debasis Samanta Sir from I.I.T(Indian Institute of Technology) Kharagpur. This will also help you practice Java.
This repository in NPTEL course Programming in Java Question and Quiz answer. - Programming-in-Java-NPTEL/week 12/Exercise 12.1.java at master · sumitnce1/Programming-in-Java-NPTEL
Week 1: 1D array, list and vector, 2D matrices and tables of objects. Week 2:Java implementation of 1D and 2D arrays and its operations. Week 3: Linked lists and its various operations, stack and queue. Week 4: Java implementation of linked lists, stack and queue. Week 5: Binary trees: Representation and operations.
Exercise1_2 - Complete the code segment to find the largest among three numbers x,y, and z. You should use if-then-else construct in Java. Exercise1_3 - Consider First n even numbers starting from zero (0).Complete the code segment to calculate sum of all the numbers divisible by 3 from 0 to n. Print the sum.
🔊 NPTEL Programming In Java WEEK8 Quiz Assignment Solutions | Swayam Jan 2024 | IIT Kharagpur | GATE NPTEL⛳ABOUT THE COURSE :With the growth of Information ...
NPTEL » Object Oriented System Development using UML, Java and Patterns Announcements About the Course Ask a Question Progress Mentor - Week 6. ... The due date for submitting this assignment has passed As per our records you have not submitted this assignment. Due on 2020-10-28, 23:59 IST.
Average assignment score = 25% of the average of best 8 assignments out of the total 12 assignments given in the course. Exam score = 75% of the proctored certification exam score out of 100. Final score = Average assignment score + Exam score. You will be eligible for the certificate only if Average Assignment Score >= 10/25 & Exam score>= 30/75.
NPTEL Programming in Java Jan 2024 Week 6 to 12 Posted on March 01, 2024 Please scroll down for latest Programs. 👇 . Week 6 : Programming Assignment 1. Due on 2024-03-14, 23:59 IST. ... Assignment will be evaluated only after submitting using Submit button below. If you only save as or compile and run the Program , your assignment will not ...
Programming In Java Week 8 Assignment 8 Answers Solution Quiz | 2024-JanJoin NPTEL - Programming in Java : https://telegram.me/ProgrammingInJavaNPTELFollow W...
There will be sections under the course outline "Online Programming Test (October 22)" that will have the online programming exam link. 4. You can click on this link and attempt the programming exam. Date: October 22, 2022 (Saturday) (Duration of the session will be 2 hrs) First Session: 10.00AM - 12.00PM.
Due on 2024-04-11, 23:59 IST. Due to some mistakes in the below code, the code is not compiled/executable. Modify and debug the JDBC code to make it execute successfully. Your last recorded submission was on 2024-03-30, 17:29 IST. Select the Language for this assignment. File name for this program : import java.sql.*;
The password must be at least 8 characters long. Follow the naming convention as given in the main method of the suffix code. Your last recorded submission was on 2024-04-06, 18:47 IST. Select the Language for this assignment. File name for this program : import java.util.Scanner; public class W12_P2 {.
Course layout. Week 1 : Overview of Object-Oriented Programming and Java. Week 2: Java Programming Elements. Week 3: Input-Output Handling in Java. Week 4: Encapsulation. Week 5: Inheritance. Week 6: Exception Handling. Week 7: Multithreaded Programming. Week 8: Java Applets and Servlets.