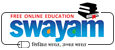
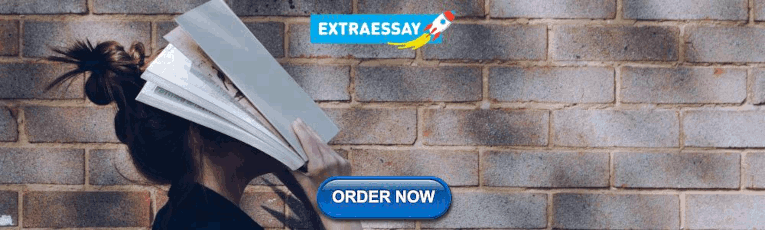
Problem Solving Through Programming In C
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
Note: This exam date is subjected to change based on seat availability. You can check final exam date on your hall ticket.
Page Visits
Course layout, books and references, instructor bio.
Prof. Anupam Basu
Course certificate.
- Assignment score = 25% of average of best 8 assignments out of the total 12 assignments given in the course.
- ( All assignments in a particular week will be counted towards final scoring - quizzes and programming assignments).
- Unproctored programming exam score = 25% of the average scores obtained as part of Unproctored programming exam - out of 100
- Proctored Exam score =50% of the proctored certification exam score out of 100

DOWNLOAD APP

SWAYAM SUPPORT
Please choose the SWAYAM National Coordinator for support. * :
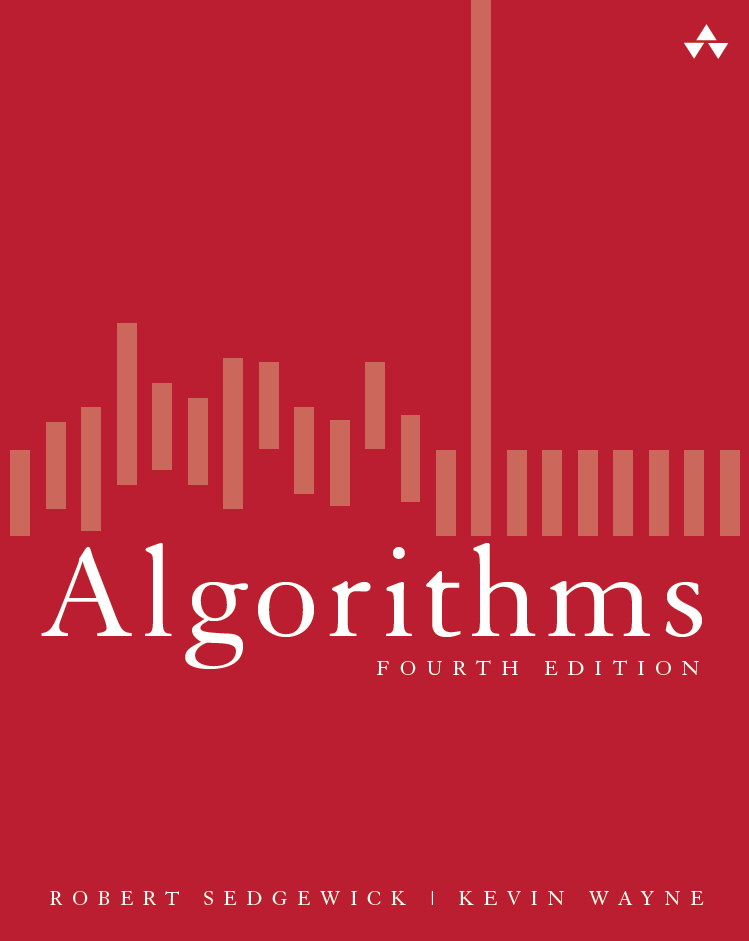
Flipped classroom.
- Each week, send an email to all students in the class that briefly describes activities for that week (lectures, reading, and programming assignments drawn from the book or from this booksite).
- Students watch the lecture videos at their own pace, do the readings, and work on the programming assignments.
Self-study.
Available lectures..

Problem Solving and Programming In C Notes and Study Material PDF Free Download
Problem Solving and Programming in C Notes: C is one of the popular programming languages that are simple and flexible. It is a general-purpose programming language that is widely used in different kinds of applications. Operating Systems like Windows and many others are written in C language. Git, Python Interpreter, and Oracle Database are also written in C. This language is often called the base knowledge of programming. If you know this language, it becomes easier for you to learn any other programming language. This is a simple language which can provide faster execution. The demand for C developers is very high in the job market. This programming language can extend itself. It contains various kinds of functions that are part of the library. In this article, you will find complete details about problem-solving and programming of C language Lecture Notes .
Problem Solving And Programming In C Notes and Study Material PDF Free Download
Problem solving and programming in c reference books, problem solving and programming in c curriculum, list of problem solving and programming in c important questions.
- FAQs on Problem Solving And Programming In C Notes
Introduction to Problem Solving And Programming In C
Ritchie first developed this language in 1972. It is a structured language that is widely used in the software development field. For every software developer, it is important to know the C language. This language can handle low-level activities and can be compiled easily. This language is primarily used in UNIX. This language is the successor of the B language. C language is used in databases, utilities, text editors, assemblers, operating systems, and language compilers. In C programming course, you will learn this programming language from scratch. You will find all the study materials of this widely used language from this article.
Anyone interested in making a career in software development should learn C programming. It is because this language is considered as the base language of every other programming language. If you plan to do a course on C programming, you can find the right study material through this article. We have made a list of some important study materials on C programming. You can check out computer programming terminologies once before starting C programming course.
It is a relatively small language, but it is very useful. You need to learn some simple things in C programming. This language was mainly discovered so that programmers can interact with the machines efficiently. To learn this language, you must read the right set of books. We have made a list of some important books on C language.
- C Programming Absolute Beginner’s Guide.
- C Programming Language.
- The C Programming Language 2nd Edition.
- C Programming: A Modern Approach.
- Expert C Programming: Deep Secrets.
- C: The Complete Reference.
- Head First C: A Brain-Friendly Guide.
- Computer Fundamentals and Programming in C.
- Low-Level Programming by Igor Zhirkov
- C in a Nutshell by Peter Prinz & Tony Crawford
Before starting the course on C programming, you must know the syllabus. It is crucial to understand the syllabus. The syllabus of C programming varies depending on the type of course and institution. However, the basic structure of the C programming’s syllabus remains the same. In this article, you will get to know about the necessary details taught in C programming.
C Programming Language Syllabus
Fundamentals of C Language
About C tutorial
Important points about C
Applications of C
C Language and English Language
Features of C
C, C++ and Java
Overview of C Language
History of C
First Program in C Hello World
Basic Structure of C Programming
Tokens in C
Keywords in C
Identifiers in C
Format Specifiers
Format Specifiers Examples
Data Types in C Language
Introduction to Data Types in C
int Data Type in C
float Data Type in C
double Data Type in C
char Data Type in C
Variable in C Language
Variable Introduction in C
Variable Declaration and Initialization
Variable types and Scope in C
Local Variable in C
static Variable in C
Global variables in C
Storage Class in C
Constant in C Language
Constants in C
Operators and Enums in C Language
Introduction to Operator
Arithmetic Operators in C
Relational Operators in C
Bit-wise Operators in C
Logical Operators in C
Assignment Operators in C
Conditional Operator in C
size of() Operator in C
Operator Precedence
Decision Making of C Language
Decision Making in C Introduction
if Statement
if-else Statement
Nested if Statement
if- else if Ladder
switch case
Loop control in C Language
Loop Introduction in C
while loop in C
do-while Loop In C
for Loop in C
Control Flow in C Programming
break Statement in C
continue Statement in C
goto statement in C
Array in C Language
Single Dimensional Array
Multi-Dimensional Array in C
String in C Language
Introduction to String
Function in C Language
Function in C
Function Calling in C
return type in Function
Call by Value in C
User Define Function
Predefined Functions
String functions in C
All String Functions
strcat() function
strncat() function
strcpy() function
strncpy() function
strlen() function
strcmp() function
strcmpi() function
strchr() function
strrchr() function
strstr() function
strrstr() function
strdup() function
strlwr() function
strupr() function
strrev() function
strset() function
strnset() function
strtok() function
Recursion in c
Introduction to Recursion
Direct and Indirect Recursion
Pointer in C Language
Pointer in C
types of pointer
NULL pointer
Dangling Pointer
Void/Generic Pointers
Wild Pointer
Near, Far and Huge Pointer
Pointer Expressions and Arithmetic
Pointer and Array
Strings as pointers
Pointer to Function
Call by Reference in C
Structure in C Language
Structure in C
Nested Structure in C
The array of Structures in C
Pointer to Structure
Structure to Function in C
typedef in C
typedef vs #define in C
Union in C Language
File Input/Output
Introduction to File
File Operation in c
Dynamic Memory Allocation
Introduction to DMA
calloc() and free() function
realloc() and free() function
C Pre-processor
Introduction about Pre-processor
- What is the difference between ++i and i++?
- Give a brief note on the volatile keyword.
- What are the basic data types related to C?
- Explain syntax errors.
- How can you create a decrement and increment statement in C?
- Explain dangling pointer in C.
- What is called the prototype function in C?
- What is a header file? Explain its usage in C programming.
- Explain pointer on a pointer in C language.
- How can you save data in a stack data structure type?
Browse Course Material
Course info, instructors.
- Daniel Weller
- Sharat Chikkerur
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Practical programming in c, problem set 1.
Problem set on writing, compiling, and debugging C programs, preprocessor macros, the C file structure, variables, functions and program statements, and returning from functions.

You are leaving MIT OpenCourseWare
- Computer Science and Engineering
- Introduction to Problem Solving and Programming (Video)
- Co-ordinated by : IIT Kanpur
- Available from : 2009-12-31
- Watch on YouTube
- Assignments
- Transcripts

THE PATH TO SUCCESS IN EXAM...
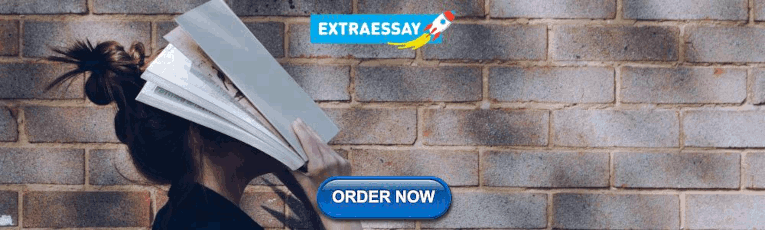
Introduction to Problem Solving – Notes
Introduction to problem solving.
- Steps for problem solving ( analysing the problem, developing an algorithm, coding, testing and debugging).
- flow chart and
- pseudo code,
Decomposition
Introduction
Computers is machine that not only use to develop the software. It is also used for solving various day-to-day problems.
Computers cannot solve a problem by themselves. It solve the problem on basic of the step-by-step instructions given by us.
Thus, the success of a computer in solving a problem depends on how correctly and precisely we –
- Identifying (define) the problem
- Designing & developing an algorithm and
- Implementing the algorithm (solution) do develop a program using any programming language.
Thus problem solving is an essential skill that a computer science student should know.
Steps for Problem Solving-
1. Analysing the problem
Analysing the problems means understand a problem clearly before we begin to find the solution for it. Analysing a problem helps to figure out what are the inputs that our program should accept and the outputs that it should produce.
2. Developing an Algorithm
It is essential to device a solution before writing a program code for a given problem. The solution is represented in natural language and is called an algorithm.
Algorithm: A set of exact steps which when followed, solve the problem or accomplish the required task.
Coding is the process of converting the algorithm into the program which can be understood by the computer to generate the desired solution.
You can use any high level programming languages for writing a program.
4. Testing and Debugging
The program created should be tested on various parameters.
- The program should meet the requirements of the user.
- It must respond within the expected time.
- It should generate correct output for all possible inputs.
- In the presence of syntactical errors, no output will be obtained.
- In case the output generated is incorrect, then the program should be checked for logical errors, if any.
Software Testing methods are
- unit or component testing,
- integration testing,
- system testing, and
- acceptance testing
Debugging – The errors or defects found in the testing phases are debugged or rectified and the program is again tested. This continues till all the errors are removed from the program.
Algorithm is a set of sequence which followed to solve a problem.
Algorithm for an activity ‘riding a bicycle’: 1) remove the bicycle from the stand, 2) sit on the seat of the bicycle, 3) start peddling, 4) use breaks whenever needed and 5) stop on reaching the destination.
Algorithm for Computing GCD of two numbers:
Step 1: Find the numbers (divisors) which can divide the given numbers.
Step 2: Then find the largest common number from these two lists.
A finite sequence of steps required to get the desired output is called an algorithm. Algorithm has a definite beginning and a definite end, and consists of a finite number of steps.
Characteristics of a good algorithm
- Precision — the steps are precisely stated or defined.
- Uniqueness — results of each step are uniquely defined and only depend on the input and the result of the preceding steps.
- Finiteness — the algorithm always stops after a finite number of steps.
- Input — the algorithm receives some input.
- Output — the algorithm produces some output.
While writing an algorithm, it is required to clearly identify the following:
- The input to be taken from the user.
- Processing or computation to be performed to get the desired result.
- The output desired by the user.
Representation of Algorithms
There are two common methods of representing an algorithm —
Flowchart — Visual Representation of Algorithms
A flowchart is a visual representation of an algorithm. A flowchart is a diagram made up of boxes, diamonds and other shapes, connected by arrows. Each shape represents a step of the solution process and the arrow represents the order or link among the steps. There are standardised symbols to draw flowcharts.
Start/End – Also called “Terminator” symbol. It indicates where the flow starts and ends.
Process – Also called “Action Symbol,” it represents a process, action, or a single step. Decision – A decision or branching point, usually a yes/no or true/ false question is asked, and based on the answer, the path gets split into two branches.
Input / Output – Also called data symbol, this parallelogram shape is used to input or output data.
Arrow – Connector to show order of flow between shapes.
Question: Write an algorithm to find the square of a number. Algorithm to find square of a number. Step 1: Input a number and store it to num Step 2: Compute num * num and store it in square Step 3: Print square
The algorithm to find square of a number can be represented pictorially using flowchart

A pseudocode (pronounced Soo-doh-kohd) is another way of representing an algorithm. It is considered as a non-formal language that helps programmers to write algorithm. It is a detailed description of instructions that a computer must follow in a particular order.
- It is intended for human reading and cannot be executed directly by the computer.
- No specific standard for writing a pseudocode exists.
- The word “pseudo” means “not real,” so “pseudocode” means “not real code”.
Keywords are used in pseudocode:
Question : Write an algorithm to calculate area and perimeter of a rectangle, using both pseudocode and flowchart.
Pseudocode for calculating area and perimeter of a rectangle.
INPUT length INPUT breadth COMPUTE Area = length * breadth PRINT Area COMPUTE Perim = 2 * (length + breadth) PRINT Perim The flowchart for this algorithm

Benefits of Pseudocode
- A pseudocode of a program helps in representing the basic functionality of the intended program.
- By writing the code first in a human readable language, the programmer safeguards against leaving out any important step.
- For non-programmers, actual programs are difficult to read and understand, but pseudocode helps them to review the steps to confirm that the proposed implementation is going to achieve the desire output.
Flow of Control :
The flow of control depicts the flow of process as represented in the flow chart. The process can flow in
In a sequence steps of algorithms (i.e. statements) are executed one after the other.
In a selection, steps of algorithm is depend upon the conditions i.e. any one of the alternatives statement is selected based on the outcome of a condition.
Conditionals are used to check possibilities. The program checks one or more conditions and perform operations (sequence of actions) depending on true or false value of the condition.
Conditionals are written in the algorithm as follows: If is true then steps to be taken when the condition is true/fulfilled otherwise steps to be taken when the condition is false/not fulfilled
Question : Write an algorithm to check whether a number is odd or even. • Input: Any number • Process: Check whether the number is even or not • Output: Message “Even” or “Odd” Pseudocode of the algorithm can be written as follows: PRINT “Enter the Number” INPUT number IF number MOD 2 == 0 THEN PRINT “Number is Even” ELSE PRINT “Number is Odd”
The flowchart representation of the algorithm
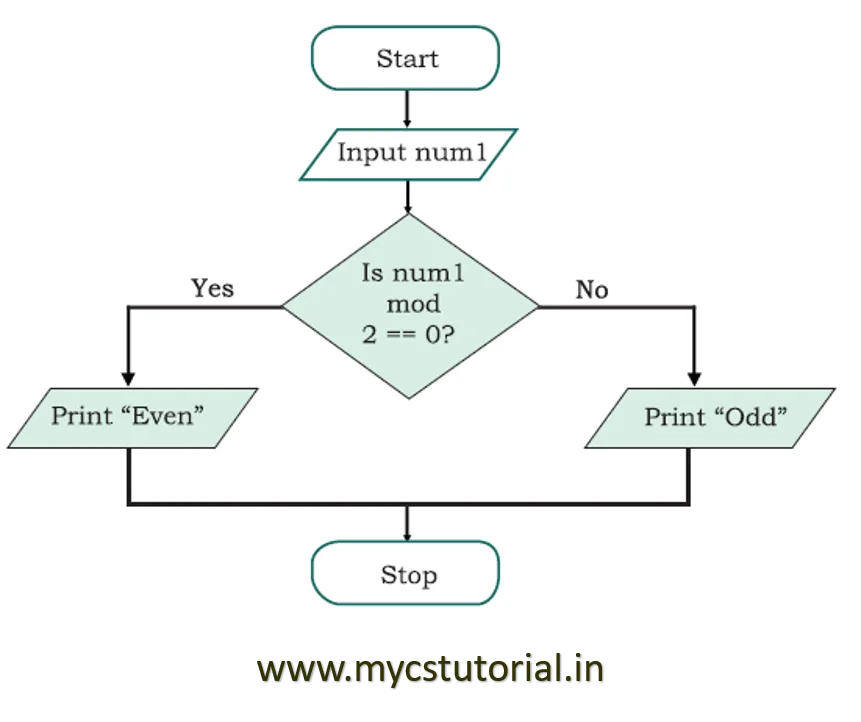
Repetitions are used, when we want to do something repeatedly, for a given number of times.
Question : Write pseudocode and draw flowchart to accept numbers till the user enters 0 and then find their average. Pseudocode is as follows:
Step 1: Set count = 0, sum = 0 Step 2: Input num Step 3: While num is not equal to 0, repeat Steps 4 to 6 Step 4: sum = sum + num Step 5: count = count + 1 Step 6: Input num Step 7: Compute average = sum/count Step 8: Print average The flowchart representation is
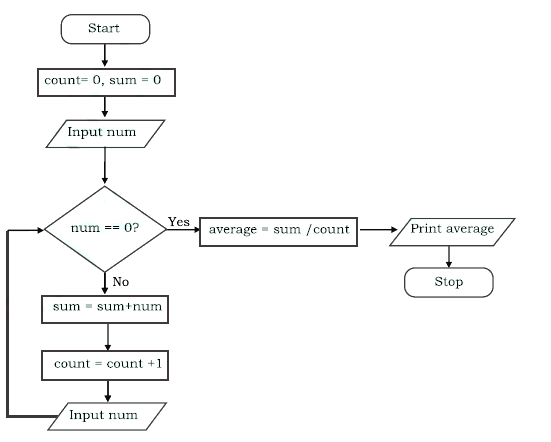
Once an algorithm is finalised, it should be coded in a high-level programming language as selected by the programmer. The ordered set of instructions are written in that programming language by following its syntax.
The syntax is the set of rules or grammar that governs the formulation of the statements in the language, such as spelling, order of words, punctuation, etc.
Source Code: A program written in a high-level language is called source code.
We need to translate the source code into machine language using a compiler or an interpreter so that it can be understood by the computer.
Decomposition is a process to ‘decompose’ or break down a complex problem into smaller subproblems. It is helpful when we have to solve any big or complex problem.
- Breaking down a complex problem into sub problems also means that each subproblem can be examined in detail.
- Each subproblem can be solved independently and by different persons (or teams).
- Having different teams working on different sub-problems can also be advantageous because specific sub-problems can be assigned to teams who are experts in solving such problems.
Once the individual sub-problems are solved, it is necessary to test them for their correctness and integrate them to get the complete solution.
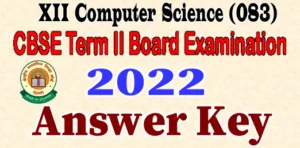
- Input Output in Python

Related Posts

Society, Law, and Ethics: Societal Impacts – Notes
Data structure: stacks – notes.

Python Revision Tour I : Basics of Python – Notes

Introduction to Python Module – Notes

Sorting Techniques in Python – Notes
Dictionary handling in python – notes, tuples manipulation in python notes, list manipulation – notes, leave a comment cancel reply.
You must be logged in to post a comment.
You cannot copy content of this page

Problem Solving and Programming | Unit 1 – 6 Notes
Course objectives:.
Prime objective is to give students a basic introduction to programming and problem solving with computer language Python. And to introduce students not merely to the coding of computer programs, but to computational thinking, the methodology of computer programming, and the principles of good program design including modularity and encapsulation.
- To understand problem solving, problem solving aspects, programming and to know about various program design tools.
- To learn problem solving with computers
- To learn basics, features and future of Python programming.
- To acquaint with data types, input output statements, decision making, looping and functions in Python
- To learn features of Object Oriented Programming using Python
- To acquaint with the use and benefits of files handling in Python
Examination Scheme:
In Semester : 30 Marks End Semester: 70 Marks PR: 25 Marks
Download Notes
Download question papers 🔗, unit i: problem solving, programming and python programming.
General Problem Solving Concepts- Problem solving in everyday life, types of problems, problem solving with computers, difficulties with problem solving, problem solving aspects, top down design. Problem Solving Strategies, Program Design Tools: Algorithms, Flowcharts and Pseudo-codes, implementation of algorithms. Basics of Python Programming: Features of Python, History and Future of Python, Writing and executing Python program, Literal constants, variables and identifiers, Data Types, Input operation, Comments, Reserved words, Indentation, Operators and expressions, Expressions in Python.
Unit 2: Decision Control Statements
Decision Control Statements: Decision control statements, Selection/conditional branching Statements: if, if-else, nested if, if-elif-else statements. Basic loop Structures/Iterative statements: while loop, for loop, selecting appropriate loop. Nested loops, The break, continue, pass, else statement used with loops. Other data types- Tuples, Lists and Dictionary.
Unit 3: Functions and Modules
Need for functions, Function: definition, call, variable scope and lifetime, the return statement. Defining functions, Lambda or anonymous function, documentation string, good programming practices. Introduction to modules, Introduction to packages in Python, Introduction to standard library modules.
Unit 4: Strings
Strings and Operations- concatenation, appending, multiplication and slicing. Strings are immutable, strings formatting operator, built in string methods and functions. Slice operation, ord() and chr() functions, in and not in operators, comparing strings, Iterating strings, the string module.
Unit 5: Object Oriented Programming
Programming Paradigms-monolithic, procedural, structured and object oriented, Features of Object oriented programming-classes, objects, methods and message passing, inheritance, polymorphism, containership, reusability, delegation, data abstraction and encapsulation. Classes and Objects: classes and objects, class method and self object, class variables and object variables, public and private members, class methods.
Unit 6: File Handling and Dictionaries
Files: Introduction, File path, Types of files, Opening and Closing files, Reading and Writing files. Dictionary method. Dictionaries- creating, assessing, adding and updating values. Case Study: Study design, features, and use of any recent, popular and efficient system developed using Python. (This topic is to be excluded for theory examination).
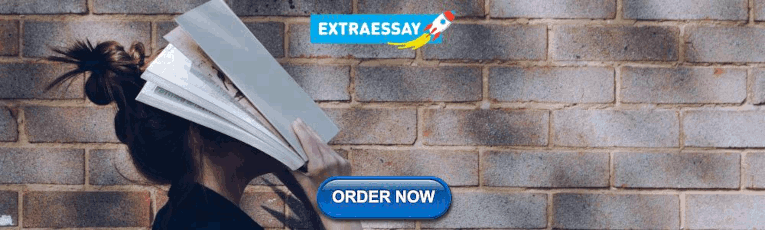
IMAGES
VIDEO
COMMENTS
CS151: Problem Solving and Programming Lecture Notes Algorithms for multiplying non-negative binary numbers Algorithm 1: Given the multiplication A Bwhere Aand Bare non-negative binary numbers. Let C start with the value zero While B is not zero Add A to C Subtract 1 from B Return the value of C. Algorithm 2: Given the multiplication A Bwhere ...
Courses. Computer Science and Engineering. NOC:Problem Solving through Programming in C (Video) Syllabus. Co-ordinated by : IIT Kharagpur. Available from : 2017-12-21. Lec : 1.
Programming for problem solving using C Notes Unit - I Computer History, Hardware, Software, Programming Languages and Algorithms: Components andfunctions of a Computer System, Concept of Hardware and Software Programming Languages: Low- level and High-level Languages, Program Design Tools: Algorithm, Flowchart, Pseudo code.
Introduction to Programming and Problem Solving Relevant Reading: Chapters1and 2 I. Introductory course materials A. Coursesyllabus B. Lecturenotes week 1 (these notes) C. Lab1writeup D. Program1writeup II.What is a program? A. Inthe most simple terms, a program is a list of instructions. B. Inthis sense, humans followprograms frequently,for ...
COMP1405/1005 - An Introduction to Computer Science and Problem Solving Fall 2011 - 4- There are also other types of programming languages such as functional programming languages and logic programming languages. According to the Tiobe index (i.e., a good site for ranking the popularity of programming languages), as of February 2011 the 10 most
PROGRAMMING FOR PROBLEM SOLVING L T P C 3 1 - 3 COURSE OBJECTIVES: The students will be able to 1. Understand the use of computer system in problem solving and to build program logic with algorithms and flowcharts. 2. To learn the syntax and semantics of C Programming Language 3. To learn the usage of structured programming approach in solving ...
Learners enrolled: 29073. ABOUT THE COURSE : This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors. Implement conditional branching, iteration and recursion.
This section provides the lecture notes for each session of the course along with supporting code files. ... Introduction to Computers and Engineering Problem Solving. Menu. More Info Syllabus Instructor Insights Readings Lecture Notes ... assignment_turned_in Programming Assignments with Examples. co_present Instructor Insights.
LECTURE NOTES 1 Introduction. Writing, compiling, and debugging C programs. Hello world. 2 Variables and datatypes, operators. 3 Control flow. Functions and modular programming. Variable scope. Static and global variables. 4 More control flow. Input and output. 5 Pointers and memory addressing. Arrays and pointer arithmetic. Strings.
Course abstract. This course is aimed at enabling the students to 1. Formulate simple algorithms for arithmetic and logical problems 2. Translate the algorithms to programs (in C language) 3. Test and execute the programs and correct syntax and logical errors 4. Implement conditional branching, iteration and recursion 5.
MIT OpenCourseWare is a web based publication of virtually all MIT course content. OCW is open and available to the world and is a permanent MIT activity
PROGRAMMING FOR PROBLEM SOLVING - MRCET
LECTURE NOTES FOR Problem Solving Through Programming (in C) I YEAR I SEMESTER B.TECH (COMPUTER SCIENCE AND ENGINEERING) 2018-19 Department of Computer Science and Engineering ACE ENGINEERING COLLEGE (NBA Accredited B.Tech Courses: EEE, ECE, CSE) (Affiliated to Jawaharlal Nehru Technological University, Hyderabad, Telangana)
Lecture 23: Linear Programming. The quintessential problem-solving model is known as linear programming, and the simplex method for solving it is one of the most widely used algorithms. In this lecture, we given an overview of this central topic in operations research and describe its relationship to algorithms that we have considered.
Introduction to Problem Solving: Problem-solving strategies, Problem identification, Problem understanding, Algorithm development, Solution planning (flowcharts ... Problem solving - Lecture notes 1. Problem Solving and Programming. Lecture notes. 100% (9) ... Problem Solving and Programming (COMS 304) 12 Documents. Students shared 12 documents ...
January 31, 2024 by veer. Problem Solving and Programming in C Notes: C is one of the popular programming languages that are simple and flexible. It is a general-purpose programming language that is widely used in different kinds of applications. Operating Systems like Windows and many others are written in C language.
Jones and Harrow present programming concepts in the context of solving problems. Each chapter introduces a problem first, and then covers the C language elements needed to solve it. Students can see how a program is built from its simplest beginning to its final polished form. This book introduces beginning programming concepts using the C language.
So, the file name prog1 might be a valid filename for a C program. A text editor is usually used to enter the C program into a file. For example, vi is a popular text editor used on Unix systems. The program that is entered into the file is known as the source program because it represents the original form of the program expressed in the C ...
Problem set on writing, compiling, and debugging C programs, preprocessor macros, the C file structure, variables, functions and program statements, and returning from functions. Browse Course Material Syllabus Calendar Lecture Notes ... notes Lecture Notes. group_work Projects. assignment_turned_in Programming Assignments with ...
NPTEL :: Computer Science and Engineering - Introduction to Problem Solving and Programming. Courses. Computer Science and Engineering. Introduction to Problem Solving and Programming (Video) Syllabus. Co-ordinated by : IIT Kanpur. Available from : 2009-12-31. Lec : 1.
Implementing the algorithm (solution) do develop a program using any programming language. Thus problem solving is an essential skill that a computer science student should know. Steps for Problem Solving-1. Analysing the problem. Analysing the problems means understand a problem clearly before we begin to find the solution for it.
Dictionaries- creating, assessing, adding and updating values. Case Study: Study design, features, and use of any recent, popular and efficient system developed using Python. (This topic is to be excluded for theory examination). Download the Notes of Problem Solving & Programming [PPS] for Pune University SPPU. For the first year engineering.
correct program. PROBLEM SOLVING TECHNIQUES Problem solving technique is a set of techniques that helps in providing logic for solving a problem. Problem solving can be expressed in the form of 1. Algorithms. 2. Flowcharts. 3. Pseudo codes. 4. Programs 1.ALGORITHM It is defined as a sequence of instructions that describe a method for solving a ...