Learn Python practically and Get Certified .
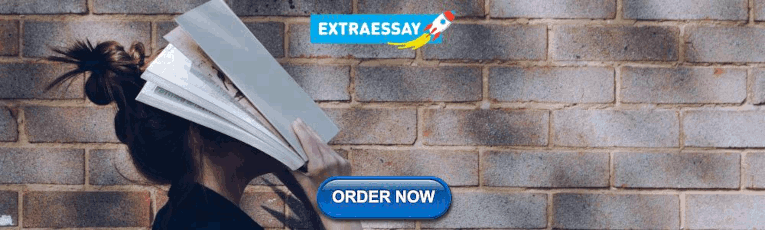
Popular Tutorials
Popular examples, reference materials, learn python interactively, built-in functions.
- Python abs()
- Python any()
- Python all()
- Python ascii()
- Python bin()
- Python bool()
- Python bytearray()
- Python callable()
- Python bytes()
- Python chr()
- Python compile()
- Python classmethod()
- Python complex()
- Python delattr()
- Python dict()
- Python dir()
- Python divmod()
- Python enumerate()
- Python staticmethod()
- Python filter()
Python eval()
- Python float()
- Python format()
- Python frozenset()
- Python getattr()
Python globals()
Python exec()
- Python hasattr()
- Python help()
- Python hex()
- Python hash()
- Python input()
- Python id()
- Python isinstance()
- Python int()
- Python issubclass()
- Python iter()
- Python list() Function
Python locals()
- Python len()
- Python max()
- Python min()
- Python map()
- Python next()
- Python memoryview()
- Python object()
- Python oct()
- Python ord()
- Python open()
- Python pow()
- Python print()
- Python property()
- Python range()
- Python repr()
- Python reversed()
- Python round()
- Python set()
- Python setattr()
- Python slice()
- Python sorted()
- Python str()
- Python sum()
- Python tuple() Function
- Python type()
- Python vars()
- Python zip()
Python __import__()
- Python super()
Python Tutorials
- Python Programming Built-in Functions
- Python Mathematical Functions
The eval() method parses the expression passed to this method and runs python expression (code) within the program.
eval() Syntax
The syntax of eval() is:
eval() Parameters
The eval() function takes three parameters:
- expression - the string parsed and evaluated as a Python expression
- globals (optional) - a dictionary
- locals (optional)- a mapping object. Dictionary is the standard and commonly used mapping type in Python.
The use of globals and locals will be discussed later in this article.
eval() Return Value
The eval() method returns the result evaluated from the expression .
Example 1: How eval() works in Python
Here, the eval() function evaluates the expression x + 1 and print() is used to display this value.
Example 2: Practical Example to Demonstrate Use of eval()
Warnings when using eval().
Consider a situation where you are using a Unix system (macOS, Linux etc) and you have imported the os module. The os module provides a portable way to use operating system functionalities like reading or writing to a file.
If you allow users to input a value using eval(input()) , the user may issue commands to change file or even delete all the files using the command: os.system('rm -rf *') .
If you are using eval(input()) in your code, it is a good idea to check which variables and methods the user can use. You can see which variables and methods are available using dir() method .
Restricting the Use of Available Methods and Variables in eval()
More often than not, all the available methods and variables used in the expression (first parameter to eval() ) may not be needed, or even may have a security hole. You may need to restrict the use of these methods and variables for eval() . You can do so by passing optional globals and locals parameters (dictionaries) to the eval() function.
1. When both globals and locals parameters omitted
If both parameters are omitted (as in our earlier examples), the expression is executed in the current scope. You can check the available variables and methods using following code:
2. Passing globals parameter; locals parameter is omitted
The globals and locals parameters (dictionaries) are used for global and local variables respectively. If the locals dictionary is omitted, it defaults to globals dictionary. Meaning, globals will be used for both global and local variables.
Note: You can check the current global and local dictionary in Python using globals() and locals() built-in methods respectively.
Example 3: Passing empty dictionary as globals parameter
If you pass an empty dictionary as globals , only the __builtins__ are available to expression (first parameter to the eval() ).
Even though we have imported the math module in the above program, expression can't access any functions provided by the math module .
Example 4: Making Certain Methods available
Here, the expression can only use the sqrt() and the pow() methods along with __builtins__ .
It is also possible to change the name of the method available for the expression as to your wish:
In the above program, square_root() calculates the square root using sqrt() . However, trying to use sqrt() directly will raise an error.
Example 5: Restricting the Use of built-ins
You can restrict the use of __builtins__ in the expression as follows:
3. Passing both globals and locals dictionary
You can make needed functions and variables available for use by passing the locals dictionary. For example:
In this program, expression can have sqrt() method and variable a only. All other methods and variables are unavailable.
Restricting the use of eval() by passing globals and locals dictionaries will make your code secure particularly when you are using input provided by the user to the eval() method.
Note: Sometimes, eval() is not secure even with limited names. When an object and its methods are made accessible, almost anything can be done. The only secure way is by validating the user input.
Sorry about that.
Python References
Python Library
How to Assign the Result of eval() to a Python Variable?
💬 Question : Say you have an expression you want to execute using the eval() function. How to store the result of the expression in a Python variable my_result ?
Before I show you the solution, let’s quickly recap the eval() function:
Recap Python eval()
Python eval(s) parses the string argument s into a Python expression, runs it, and returns the result of the expression.

Related Tutorial : Python’s eval() built-in function
Without further ado, let’s learn how you can store the result of the eval() function in a Python variable:
Method 1: Simple Assignment
The most straightforward way to store the result of an eval() expression in a Python variable is to as s ign the whole return value to the variable. For example, the expression my_result = eval('2+2') stores the result 4 in the variable my_result .
Here’s a minimal example:
This simple approach may not always work, for example, if you have a print() statement in the expression.
Read on to learn how to fix this issue next and learn something new!
Method 2: Redirect Standard Output
This method assumes you have a print() statement within the expression passed into the eval() function such as shown in the following three examples:
- eval('print(2+2)')
- eval('print([1, 2, 3, 4] + [5, 6])')
- eval('print(2+2*0)')
To get the output and store it in a variable my_result , you need to temporarily redirect the standard output to the variable.
The following code shows you how to accomplish exactly this:
If you need some assistance understanding this whole idea of redirecting the standard output, have a look at our in-depth guide on the Finxter blog.
👀 Related Article: 7 Easy Steps to Redirect Your Standard Output to a Variable (Python)
Note that this approach even works if you don’t have a print() statement in the original eval() expression because you can always artificially add the print() statement around the original expression like so:
- eval('2+2') becomes eval('print(2+2)')
- eval('2+2*0') becomes eval('print(2+2*0)')
- eval('[1, 2, 3] + [4, 5]') becomes eval('print([1, 2, 3] + [4, 5])')
Even if it’s a bit clunky, after applying this short trick, you can redirect the standard output and store the result of any eval() expression in a variable.
Method 3: Use exec()
Using only Python’s eval() function, you cannot define variables inside the expression to be evaluated. However, you can define a variable inside the exec() function that will then be added to the global namespace. Thus, you can access the defined variable in your code after termination of the exec() expression!
Here’s how that works in a minimal example:
Variable my_result is only defined in the string expression passed into exec() , but you can use it in the code like it was part of the original source code.
Recap exec() vs eval()
Python’s exec() function takes a Python program, as a string or executable object, and runs it. The eval() function evaluates an expression and returns the result of this expression. There are two main differences:
- exec() can execute all Python source code, whereas eval() can only evaluate expressions.
- exec() always returns None , whereas eval() returns the result of the evaluated expression.
- exec() can import modules, whereas eval() cannot.
You can learn more about the exec() function here:
- Related Article: Python exec() — A Hacker’s Guide to A Dangerous Function

Where to Go From Here?
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
🚀 If your answer is YES! , consider becoming a Python freelance developer ! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
Join the free webinar now!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
Python eval() function

Hello, readers. In this article we will be focusing on Python eval() function .
Understanding Python eval() function
Python eval() function converts and computes the expressions passed to it.
NOTE: Use this method only for testing purposes. The eval() function does not sanitize the expressions passed to it. It can easily become a loophole into your server if malicious users execute Python code here.
The eval() function parses the python expressions and runs the code passed to it as a parameter within the python program.
- expression : It can be any python expression(string parameter) that the user wants to compute within the python code itself.
- globals : It is a dictionary that specifies the expressions available for the eval() function to execute.
- locals : It describes the local methods and data variables that the eval() function can avail.
Example 1: Passing expression to add two local variables
In the above snippet of code, we have passed an expression ‘a+b’ to the eval() function in order to add two local variables: a, b.
Example 2: Python eval() function with user input
In the above example, we have accepted the input from the user and assigned the same to the variables. Further, we have passed the expression for the multiplication of those two input values.
Python eval() function with Pandas module
Python eval function can also operate with Pandas Module . The pandas.eval() function accepts the expression and executes the same within the python program.
- expression : The python expression enclosed within the string quotes to be executed within the python program.
- inplace : Default value=TRUE. If the python expression turns out to be an assignment expression, inplace decides to perform the operation and mutate the data frame object. If FALSE, a new dataframe gets created and is returned as a result.
Example 1: Passing an expression with inplace = TRUE
In the above example, we have created a dataframe and have passed an expression to be executed within the python script.
As the inplace is set to TRUE, the data values obtained from the expression will be stored in the same dataframe object ‘data’.
Example 2: Executing expression within python script with inplace = FALSE
In the above snippet of code, we have passed inplace = FALSE to the eval() function. Thus, the results of the python expression will be stored in a new dataframe object ‘data1’.
Security issues with eval() function
- Python eval() function is more prone to security threats.
- The sensitive user input data can easily be made available through Python eval() function.
- Thus, the parameters globals and locals is provided to restrict the access to the data directly.
- Python eval() function is used to execute python expressions within a python script directly.
- The eval() function can also be used in a similar fashion with the Pandas module .
- Python eval() function is more prone to security threats . Thus, it is necessary to check the information being passed to the eval() function before it’s execution.
Therefore, in this article, we have understood the working and the vulnerabilities of Python eval() function.
- Python eval() function — JournalDev
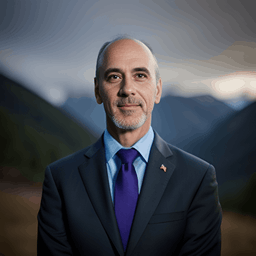
MachineLearningTutorials.org
Brief Machine Learning tutorials with examples.
Understanding Python’s eval() Function (With Examples)
Introduction.
In Python, the eval() function is a powerful and versatile tool that allows you to dynamically evaluate and execute Python expressions or statements from strings. This can be particularly useful when you want to provide users with a way to input and execute code at runtime, or when you need to evaluate mathematical or logical expressions stored as strings. However, while eval() can be a powerful tool, it also comes with certain risks that need to be carefully considered. In this tutorial, we will explore the eval() function in depth, providing explanations, use cases, precautions, and real-world examples.
Table of Contents
- What is eval() ?
- Basic Syntax
- Using eval() for Mathematical Expressions
- Using eval() for Dynamic Execution
- Security Considerations
- Real-world Examples
Example 1: Simple Arithmetic Calculator
Example 2: customizable data processing, 1. what is eval() .
The eval() function in Python is used to evaluate a dynamically provided Python expression or statement stored as a string. It takes a single argument, which is the string to be evaluated, and returns the result of the evaluation. The evaluated expression can be a mathematical operation, a conditional statement, or even a series of function calls.
While eval() can be incredibly powerful for executing dynamic code, it should be used with caution due to potential security vulnerabilities. Malicious input can lead to unintended code execution or expose sensitive information, so it’s crucial to sanitize and validate input before using eval() .
2. Basic Syntax
The basic syntax of the eval() function is as follows:
Here, expression is the Python code you want to evaluate, provided as a string. The result of the evaluation will be stored in the result variable.
3. Using eval() for Mathematical Expressions
One of the most common use cases for eval() is evaluating mathematical expressions. Let’s look at an example:
In this example, the eval() function evaluates the expression “2 + 3 * 5”, which is equivalent to 2 + (3 * 5), resulting in 17. The output of the program will be:
You can also use variables in your expressions:
In this case, the expression “x * y + y 2″ evaluates to 10 * 5 + 5 2, which is 75. The output will be:
4. Using eval() for Dynamic Execution
Another powerful use of eval() is dynamic execution of code. This can be particularly useful in scenarios where you want to allow users to input code snippets for execution. However, keep in mind that using eval() for dynamic execution can be risky, especially if you’re not in full control of the input.
Here’s an example that demonstrates dynamic execution:
In this example, the code variable contains a string with a for loop that prints the numbers from 0 to 4. When eval(code) is executed, the loop is evaluated and the output will be:
5. Security Considerations
While eval() is a powerful tool, it’s important to consider security implications. Since eval() executes arbitrary code, it can lead to code injection vulnerabilities if not used carefully. Here are a few precautions to take:
- Sanitize Input: Validate and sanitize any input that will be passed to eval() . Avoid using user-provided input directly in eval() calls without proper validation.
- Limit Scope: If possible, use the globals and locals arguments of eval() to limit the scope in which the expression is evaluated. This can help prevent unintended access to variables.
- Avoid Executing Untrusted Code: Never use eval() to execute code from untrusted sources, as this could potentially lead to malicious code execution.
6. Real-world Examples
In this example, we’ve created a simple arithmetic calculator using the eval() function. The program repeatedly prompts the user to enter an arithmetic expression, evaluates it using eval() , and prints the result. The program continues until the user enters ‘exit’.
In this example, the user is prompted to enter a processing code that will be applied to each item in the data list using eval() . The processed data is then displayed. This example showcases how eval() can be used to allow users to customize data processing operations.
The eval() function in Python is a versatile tool that enables dynamic evaluation and execution of code stored as strings. It can be used for tasks ranging from mathematical expression evaluation to dynamic code execution. However, it’s important to use eval() with caution, as improper usage can lead to security vulnerabilities. Always validate and sanitize input, and avoid using eval() for untrusted code.
By understanding the capabilities and risks associated with eval() , you can harness its power effectively and safely in your Python programs.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python Built in Functions
- abs() in Python
- Python - all() function
- Python any() function
- ascii() in Python
- bin() in Python
- bool() in Python
- Python bytes() method
- chr() Function in Python
- Python dict() Function
- divmod() in Python and its application
- Enumerate() in Python
eval in Python
- filter() in python
- float() in Python
- Python String format() Method
- Python - globals() function
- Python hash() method
- hex() function in Python
- id() function in Python
- Python 3 - input() function
- Python int() Function
- Python len() Function
- Python map() function
- Python - max() function
Python eval() function parse the expression argument and evaluate it as a Python expression and runs Python expression (code) within the program.
Python eval() Function Syntax
Syntax: eval(expression, globals=None, locals=None) Parameters: expression: String is parsed and evaluated as a Python expression globals [optional]: Dictionary to specify the available global methods and variables. locals [optional]: Another dictionary to specify the available local methods and variables. Return: Returns output of the expression.
Uses of Python eval() Function in Python
Python eval() is not much used due to security reasons, as we explored above. Still, it comes in handy in some situations like:
- You may want to use it to allow users to enter their own “scriptlets”: small expressions (or even small functions), that can be used to customize the behavior of a complex system.
- eval() is also sometimes used in applications needing to evaluate math expressions. This is much easier than writing an expression parser.
eval() Function in Python Example
Simple demonstration of eval() works.
Let us explore it with the help of a simple Python program. function_creator is a function that evaluates the mathematical functions created by the user. Let us analyze the code a bit:
- The above function takes any expression in variable x as input.
- Then the user has to enter a value of x .
- Finally, we evaluate the Python expression using the eval() built-in function by passing the expr as an argument.
Evaluating Expressions using Python’s eval()
Evaluate mathematical expressions in python.
Evaluating a mathematical expression using the value of the variable x.
Evaluate Boolean Expressions in Python
Here the eval statement x == 4 will evaluate to False because the value of x is 5, which is not equal to 4. In the second eval statement, x is None will evaluate to True because the value of x is None, and is None checks for object identity, not value equality.
Evaluate Conditional Expressions in Python
We can also evaluate condition checking on the Python eval() function.
Vulnerability issues with Python eval() Function
Our current version of solve_expression has a few vulnerabilities. The user can easily expose hidden values in the program or call a dangerous function, as eval will execute anything passed to it.
For example, if you input like this:
You will get the output:
Also, consider the situation when you have imported the os module into your Python program. The os module provides a portable way to use operating system functionalities like reading or writing a file. A single command can delete all files in your system. Of course, in most cases (like desktop programs) the user can’t do any more than they could do by writing their own Python script, but in some applications (like web apps, kiosk computers), this could be a risk!
The solution is to restrict eval to only the functions and variables we want to make available.
Making eval() safe
Python eval function comes with the facility of explicitly passing a list of functions or variables that it can access. We need to pass it as an argument in the form of a dictionary.
Now if we try to run the above programs like:
We get the output:
Let us analyze the above code step by step:
- First, we create a list of methods we want to allow as safe_list .
- Next, we create a dictionary of safe methods. In this dictionary, keys are the method names and values are their local namespaces.
- locals() is a built-in method that returns a dictionary that maps all the methods and variables in the local scope with their namespaces.
Here, we add the local variable x to the safe_dict too. No local variable other than x will get identified by the eval function.
- eval accepts dictionaries of local as well as global variables as arguments. So, in order to ensure that none of the built-in methods is available to eval expression, we pass another dictionary along with safe_dict as well, as shown below:
So, in this way, we have made our eval function safe from any possible hacks!
Please Login to comment...
Similar reads.
- Python-Built-in-functions
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
We Love Servers.
- WHY IOFLOOD?
- BARE METAL CLOUD
- DEDICATED SERVERS
Python Eval Function: Complete Guide
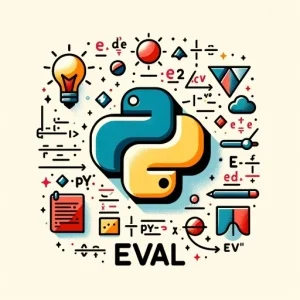
Ever found yourself puzzled by Python’s eval function? You’re not alone. Many developers find this function a bit tricky to comprehend. Think of Python’s eval function as a powerful calculator – it can evaluate expressions dynamically, making it a versatile tool in your Python toolkit.
Whether you’re looking to evaluate simple mathematical expressions or complex code snippets, understanding how to use Python’s eval function can significantly enhance your coding efficiency.
In this guide, we’ll explore the intricacies of Python’s eval function, from its basic usage to more advanced applications. We’ll also delve into potential pitfalls and discuss alternative approaches.
Let’s get started!
TL;DR: What is Python’s Eval Function?
Python’s eval() function is a powerful tool that evaluates expressions written as strings. It’s like a dynamic calculator within Python, capable of evaluating both simple and complex expressions.
Here’s a simple example:
In this example, we have a variable x set to 1. We then use the eval function to evaluate the expression ‘x + 1’ which is written as a string. The eval function evaluates the string as a Python expression, adding 1 to the value of x , and thus, the output is 2.
This is just a basic use of Python’s eval function. There’s much more to learn about this versatile function, including its advanced uses, potential pitfalls, and alternatives. So, stick around for a deeper dive!
Table of Contents
Getting Started with Python’s Eval Function
Leveraging eval for complex expressions, exploring alternatives to python’s eval function, navigating common issues with python’s eval function, python’s dynamic typing and string manipulation, python’s eval function in real-world applications, wrapping up: mastering python’s eval function.
Python’s eval function is a built-in function that parses the expression passed to it and executes Python expression(s) within it. Let’s start with a basic example:
The expression ‘4 * (6 + 2) / 2’ is a string that gets evaluated by the eval function. It follows the usual mathematical rules of precedence, so the addition is performed first, followed by multiplication and then division, yielding the result 16.0.
The Power and Pitfalls of Eval
The eval function is incredibly powerful because it can dynamically evaluate Python expressions from strings. This can be handy in many situations, like creating a simple calculator app or parsing a user’s input.
However, with great power comes great responsibility. The eval function can pose security risks if not used carefully. Since it evaluates any Python expression, a malicious user could potentially pass code that can harm your system. Therefore, it’s crucial to use eval judiciously and only with trusted input.
In the next section, we’ll dive into more advanced uses of Python’s eval function, exploring its flexibility and potential in more complex scenarios.
Python’s eval function isn’t limited to simple mathematical expressions. It can evaluate more complex expressions, and even function calls. Let’s take a look at an example:
In this example, we’re using the eval function to evaluate the string ‘pow(2, 3)’, which is a call to Python’s built-in pow function with arguments 2 and 3. The eval function evaluates this string as a Python expression, effectively executing the pow function and printing the result 8.
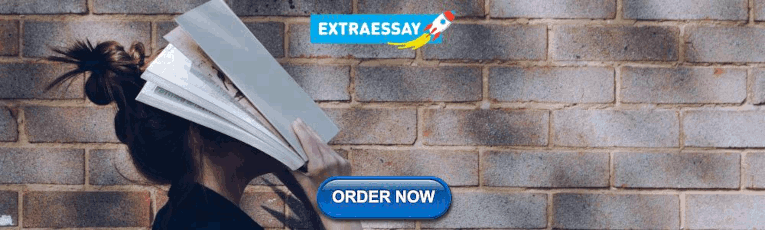
Eval with Different Scopes
The eval function can also be used with different scopes. It takes two optional dictionaries as arguments, the global and local symbol table. If provided, the expression is evaluated with those scopes. If not provided, the expression is evaluated in the current scope.
In this example, the expression ‘x * y + z’ is evaluated with x and y from the global symbol table and z from the local symbol table. Thus, the result is 18.
Handling Exceptions with Eval
Like any other Python function, the eval function can raise exceptions. For instance, if you try to evaluate a malformed expression, a SyntaxError is raised. It’s good practice to handle such exceptions when using eval.
In this example, we’re trying to evaluate the expression ‘1 / 0’ which will cause a ZeroDivisionError . By using a try-except block, we can catch this error and print a user-friendly error message instead of letting the program crash.
Remember, while Python’s eval function is a powerful tool, it’s not always the best solution. In the next section, we’ll explore some alternative approaches to eval.
While Python’s eval function is powerful and flexible, it’s not always the best choice, especially considering security and performance concerns. Let’s take a look at some alternatives and when you might want to use them.
Using literal_eval for Safe Evaluation
Python’s ast module provides a function called literal_eval that can evaluate a string containing a Python literal or container display. The key difference between eval and literal_eval is that literal_eval is safe and can only evaluate Python literals.
In this example, literal_eval is used to evaluate a string containing a list display. Unlike eval , literal_eval would raise an exception if the string contained anything other than a Python literal.
Parsing Expressions with ast.parse
For more complex needs, you can use ast.parse to parse a string into an abstract syntax tree (AST). You can then manipulate this AST and compile it into a code object, which can be executed using the exec function.
In this example, ast.parse is used to parse the expression ‘x = 2’ into an AST. This AST is then compiled into a code object, which is executed using exec , setting the value of x to 2.
Executing Code with exec
The exec function is another built-in function that can execute Python code dynamically. Unlike eval , exec can execute statements as well as expressions, but it doesn’t return a value.
In this example, exec is used to execute the statement ‘x = 2’, setting the value of x to 2.
Remember, while these alternatives can be safer or more suitable than eval in certain situations, they each have their own trade-offs. Always consider your specific needs and constraints when choosing how to evaluate or execute Python code dynamically.
While Python’s eval function is a powerful tool, it comes with its own set of challenges. Here, we’ll discuss some common issues you might encounter when using eval, along with their solutions.
Security Risks with Eval
One of the most significant risks of using eval is that it can evaluate any Python expression, potentially leading to code injection attacks. For example:
In this example, if the eval function were to evaluate the expression, it would execute a command to delete all files in your root directory! Therefore, never use eval with untrusted input. If you need to evaluate expressions from an untrusted source, consider using ast.literal_eval or parsing the expressions manually.
Performance Issues with Eval
The eval function can be slower than other methods of execution because it involves parsing and interpreting the expression. If performance is a concern, consider using compiled code or built-in functions instead.
Handling SyntaxErrors
If eval encounters a malformed expression, it raises a SyntaxError . You can handle this with a try-except block.
In this example, the eval function tries to evaluate the expression ‘1 /’, which is malformed. A SyntaxError is raised, which we catch and print a user-friendly error message.
Remember, while Python’s eval function is incredibly powerful, it’s important to use it responsibly. Always consider potential issues and alternatives, and strive to write secure and efficient code.
Understanding Python’s dynamic typing and string manipulation fundamentals can provide valuable insight into how the eval function operates.
Dynamic Typing in Python
Python is a dynamically typed language, which means that the type of a variable is checked during runtime and not in advance. This flexibility allows Python to evaluate expressions from strings dynamically, which is the core functionality of the eval function.
In this example, the variable x is initially an integer. Later, it’s reassigned to a string. Python’s dynamic typing allows this reassignment without any type errors.
String Manipulation in Python
Python provides robust features for string manipulation, which is crucial for the eval function. You can create, modify, and evaluate strings with Python’s built-in functions and methods.
In this example, we initially have the string ‘2 * 3’. We then replace ‘2’ with ‘4’ using Python’s replace method. The modified string ‘4 * 3’ is then evaluated using the eval function, yielding the result 12.
By understanding these fundamentals, you can better appreciate the power and flexibility of Python’s eval function. In the following sections, we’ll explore how eval can be used in different Python applications and direct you to more resources for deepening your understanding.
Python’s eval function has a wide range of applications, from scripting to testing and debugging. Its ability to evaluate expressions dynamically makes it a powerful tool in various Python applications.
Scripting with Eval
In scripting, eval can be used to evaluate expressions dynamically, which can be especially useful when the expressions are not known until runtime.
In this example, the script asks the user for an expression, evaluates it using eval, and prints the result.
Testing and Debugging with Eval
Eval can also be used in testing and debugging to evaluate test expressions or debug code dynamically.
In this example, the test function uses eval to evaluate the test expression and checks if the result matches the expected result.
Exploring Related Concepts
If you’re interested in Python’s eval function, you might also want to explore related concepts like Python’s exec function and the AST (Abstract Syntax Tree) module. The exec function is similar to eval but can execute Python code dynamically. The AST module allows you to parse Python code into an abstract syntax tree, which can be analyzed or modified.
Further Resources for Mastering Python’s Eval Function
To deepen your understanding of Python’s eval function and related concepts, consider exploring the following resources:
- IOFlood’s Python Built-In Functions Article – Learn how built-in functions can enhance your code readability and efficiency.
Simplifying Iteration with Python’s zip() Function – Dive into zipping lists, tuples, and more with Python’s versatile “zip.”
Simplifying Range Generation with xrange() in Python – Learn about the benefits of “xrange” over “range” in Python 2.
Python’s Official Documentation on Eval – Official Python documentation for insights on the built-in eval function.
Python’s Official Documentation on the AST Module – Get to know about Python’s abstract syntax trees (AST) module.
Real Python’s Comprehensive Eval Function Tutorial can help you gain extensive knowledge on Python’s eval function.
In this comprehensive guide, we’ve explored the ins and outs of Python’s eval function. We’ve delved into its usage, from basic mathematical expressions to complex code evaluations, and discussed its potential pitfalls, such as security risks and performance issues.
We’ve seen how to use the eval function in different scenarios, from simple calculations to advanced expressions involving function calls and different scopes. We’ve also tackled common challenges you might encounter when using eval, such as handling exceptions and dealing with SyntaxErrors.
We ventured into alternative approaches to eval, such as using ast.literal_eval , ast.parse , and exec . These alternatives can provide safer or more suitable solutions in certain situations, but like eval, they each come with their own trade-offs.
Here’s a quick comparison of these methods:
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python’s eval function and its alternatives.
With its ability to evaluate expressions dynamically, Python’s eval function is a powerful tool in your Python toolkit. Happy coding!
About Author

Gabriel Ramuglia
Gabriel is the owner and founder of IOFLOOD.com , an unmanaged dedicated server hosting company operating since 2010.Gabriel loves all things servers, bandwidth, and computer programming and enjoys sharing his experience on these topics with readers of the IOFLOOD blog.
Related Posts
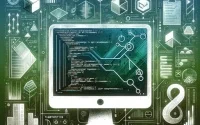
Python Built-in Functions
- Python eval() function
(Sponsors) Get started learning Python with DataCamp's free Intro to Python tutorial . Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
Updated on Jan 07, 2020
The eval() allows us to execute arbitrary strings as Python code. It accepts a source string and returns an object.
Its syntax is as follows:
If both globals and locals are omitted, the current global and local namespaces are used.
Here is an example demonstrating how eval() works:
Try it out:
The eval() is not just limited to simple expression. We can execute functions, call methods, reference variables and so on.
Note that the eval() works only with an expression. Trying to pass a statement causes a SyntaxError .
Evil eval() #
You should never pass untrusted source to the eval() directly. As it is quite easy for the malicious user to wreak havoc on your system. For example, the following code can be used to delete all the files from the system.
The above code would fail if the os module is not available in your current global scope. But we can easily circumvent this by using the __import__() built-in function.
So is there any way to make eval() safe?
Specifying Namespaces #
The eval() optionally accepts two mappings that serve as a global and local namespaces for the expression to be executed. If mapping(s) are not provided then the current values of global and local namespaces will be used.
Here are some examples:
Even though we have passed empty dictionaries as global and local namespaces, eval() still has access to built-ins (i.e __builtins__ ).
To remove built-ins from the global namespace pass a dictionary containing a key __builtins__ with the value None .
Even after removing the access to built-ins functions, eval() is still not safe. Consider the following listing.
This deceptively simple looking expression is enough to tank your CPU.
The key takeaway is that only use eval() with the trusted source.
Other Tutorials (Sponsors)
This site generously supported by DataCamp . DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
View Comments
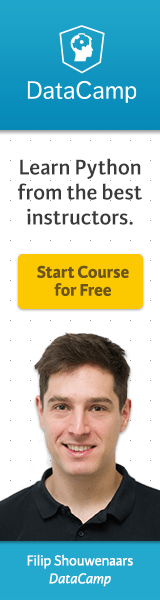
Recent Posts
- Benefits of Using Python for Cyber Security
- Vue VS React: What Are The Best JS Technologies In 2023
- Why You Should Choose Python in 2023?
- Embracing Game-Based Platforms for Enhancing Python Learning in Children
- # Comparing Python and Node.Js: Which Is Best for Your Project?
- Python abs() function
- Python bin() function
- Python id() function
- Python map() function
- Python zip() function
- Python filter() function
- Python reduce() function
- Python sorted() function
- Python enumerate() function
- Python reversed() function
- Python range() function
- Python sum() function
- Python max() function
- Python min() function
- Python len() function
- Python ord() function
- Python chr() function
- Python any() function
- Python all() function
- Python globals() function
- Python locals() function
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Python eval function.
Last Updated on October 30, 2023 by Ankit Kochar
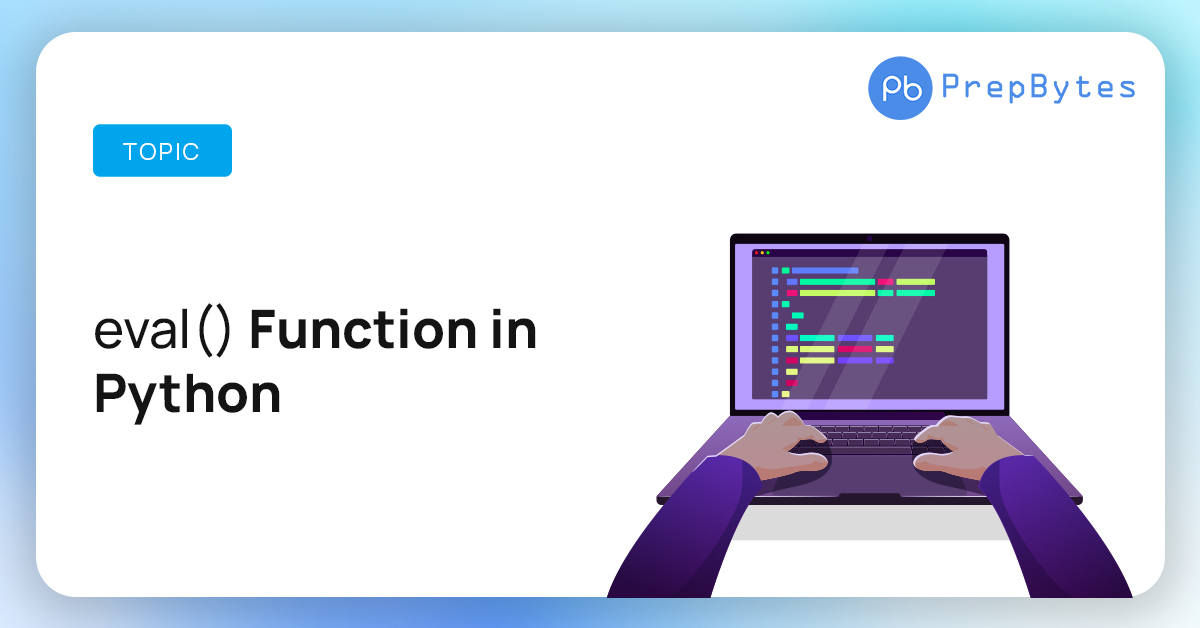
The eval function in Python is a powerful yet potentially risky feature that allows you to dynamically execute Python code from a string. It can take a string containing a Python expression or statement, evaluate it, and return the result. This capability makes eval() a versatile tool for various use cases, such as dynamic configuration, mathematical calculations, and creating mini-interpreters within your Python programs.
In this article, we’ll explore the concept of the eval function in Python in depth. We’ll discuss how to use eval(), its advantages, and common scenarios where it can be beneficial. Additionally, we’ll address the potential security risks associated with eval() and best practices for using it safely in your Python applications.
What is Eval Function in Python?
The eval in Python is a built-in function that evaluates a string as a Python expression and returns the result. It takes a single parameter which is a string containing a Python expression or statement to be evaluated. The expression can include any valid Python code, such as arithmetic operations, function calls, and control structures.
For example, if you have a string "3 + 5" and you pass it to the eval() function, it will evaluate the expression and return the result 8. Similarly, if you have a string "print(‘Hello, World!’)" and you pass it to eval(), it will execute the print() statement and print "Hello, World!" to the console.
It’s important to note that the eval() function can execute arbitrary code and therefore can be a security risk if used improperly. It should only be used with trusted input and should never be used to evaluate untrusted or user-generated input.
Here’s a simple example of using the Python eval function:
Explanation: In this example, we define a string expression that contains a Python expression. We then pass this string to the eval() function, which evaluates the expression and returns the result (14). Finally, we print the result using the print() function.
Point to Note: eval() can also be used to execute more complex expressions, such as function calls or control structures. However, as mentioned before, it should only be used with trusted input, not untrusted user-generated input, to avoid potential security risks.
Syntax of Eval Function in Python
The syntax of the eval() function in Python is
The eval() function takes one required argument, which is an expression, a string containing a valid Python expression or statement to be evaluated. In addition to the required argument, eval() can also take two optional arguments.
Parameters of Eval Function in Python
The Python eval function takes up to three parameters:
expression: This is the required parameter and represents the expression to be evaluated. It can be any valid Python expression or statement, including arithmetic expressions, function calls, or even more complex expressions involving control structures and loops.
globals (optional): This is an optional parameter that represents a dictionary of global variables. The values of these variables are used during the evaluation of the expression. If this parameter is not provided, the eval() function uses the global namespace of the calling module.
locals (optional): This is an optional parameter that represents a dictionary of local variables. The values of these variables are used during the evaluation of the expression. If this parameter is not provided, the eval() function uses the local namespace of the calling function or module.
Here’s an example that demonstrates the use of all three parameters:
Explanation: In this example, we define a dictionary global_vars containing global variables x and y. We then define a function my_function() that has a local variable z, and we use the eval() function to evaluate an expression "x + y + z" using both the global and local variables. Finally, we print the result of the expression.
Return Value of Eval Function in Python
The Python eval function returns the result of evaluating the expression that is passed to it as a string. The type of return value depends on the expression that is evaluated.
If the expression is a single Python expression that evaluates to a value, the return value of eval() will be that value. For example, if we evaluate the expression "2 + 3", the return value will be 5, which is the result of the expression.
If the expression contains multiple statements, eval() will return None. For example, if we evaluate the expression "x = 5; y = 10;", eval() will set the values of x and y but return None.
Practical Examples To Demonstrate Use Of Eval in Python
Here are some practical examples that demonstrate the use of the eval() function in Python:
Example 1: Evaluating Simple Arithmetic Expressions Below is code implementation and explanation of the example
Explanation: In this example, we define a string expression containing a simple arithmetic expression. We then use the eval() function to evaluate the expression and assign the result to the variable result. Finally, we print the value of result, which is 13.
Example 2: Evaluating Functions with Arguments The eval() function in Python can be used to evaluate functions with arguments, just like calling a regular function. Here’s an example that demonstrates how this can be done:
Explanation: In this example, we define a string expression that contains a call to a custom my_function() function with two string arguments. We define the function before evaluating the expression using eval(). The eval() function can access and use the function in the expression, as long as it’s defined in the current scope. The result of the evaluation is stored in the variable result and printed to the console, which should output the concatenated string "Hello, prepBytes!".
Example 3: Evaluating Boolean Expressions The eval() function in Python can be used to evaluate boolean expressions, just like any other Python expression. Here’s an example that demonstrates how this can be done:
Explanation: In this example, we define a string expression that contains a boolean expression to evaluate. The expression checks if the variable x is greater than 0 and the variable y is less than 10. We then define two variables x and y with values 5 and 15, respectively.
We use the eval() function to evaluate the expression by passing it as a string argument. Since x and y are defined in the current scope, eval() can access and use them in the expression. The result of the evaluation is stored in the variable result and printed to the console, which should output False.
Example 4: Evaluating Expressions using Global and Local Variables When using the eval() function in Python, it’s possible to evaluate expressions that use both global and local variables. Here’s an example that demonstrates how this can be done:
Explanation: In this example, we define a global variable x and a function evaluate_expression() that accepts an expression as an argument. Within the function, we define a local variable y and use eval() to evaluate the expression passed in as an argument.
To ensure that eval() can access the global variable x, we pass a dictionary with a single entry mapping the name ‘x’ to the value of x as the second argument to eval(). Similarly, to ensure that eval() can access the local variable y, we pass a dictionary with a single entry mapping the name ‘y’ to the value of y as the third argument to eval().
By using these dictionaries to specify the global and local variables available to eval(), we can evaluate expressions that reference both global and local variables.
Issues with Python Eval Function
The eval() function in Python can be a powerful tool for dynamically executing Python code, but it also poses a number of potential issues and risks. Here are some of the main issues to be aware of when using eval():
- Security vulnerabilities: As I mentioned earlier, the eval() function can execute any arbitrary code that is passed to it as a string, which can lead to security vulnerabilities such as injection attacks if the input is not properly sanitized or validated. This can potentially allow an attacker to execute malicious code on the system.
- Performance overhead: The eval() function can be slower than executing equivalent code directly because it has to parse and compile the input string at runtime. This can be a concern in performance-critical applications or when evaluating large or complex expressions.
- Debugging difficulties: Using eval() can make debugging more difficult because errors can occur in the evaluated code and not in the calling code. This can make it harder to diagnose and fix issues.
- Maintainability challenges: Dynamically generated code can be more difficult to maintain than code that is written directly. This can be a concern if the dynamically generated code is complex or if it changes frequently.
To address these issues, it’s important to use eval() judiciously and to follow best practices for secure coding and input validation. It’s also a good idea to consider alternative approaches such as using pre-compiled code or more limited parsing frameworks like ast.literal_eval() where possible. By taking these steps, you can minimize the risks associated with using eval() and ensure that your code is as secure, performant, and maintainable as possible.
Uses Of Python Eval Function
The eval() function in Python can be used in a variety of situations where it’s necessary to dynamically evaluate a Python expression or execute code at runtime. Here are some common use cases:
- Mathematical expressions: eval() can be used to evaluate mathematical expressions entered by the user. For example, you could use eval() to calculate the result of a user-entered equation.
- Dynamic configuration: eval() can be used to dynamically configure Python objects or modules at runtime. For example, you could use eval() to read a configuration file and dynamically set the properties of a Python object based on the contents of the file.
- Template rendering: eval() can be used to dynamically generate HTML or other text-based formats based on a template or other input. For example, you could use eval() to render a dynamically generated web page or email message.
- Custom scripting: eval() can be used to provide a custom scripting environment within a Python application. For example, you could use eval() to allow users to write and execute Python scripts within your application.
- Code generation: eval() can be used to dynamically generate Python code at runtime. For example, you could use eval() to generate code based on a set of user-defined rules or parameters.
The eval function in Python is a powerful tool for dynamically executing Python code from strings, offering versatility and convenience for certain use cases. It allows you to evaluate expressions and statements, making it useful for tasks like dynamic configuration and mathematical calculations.
In this article, we’ve delved into the world of eval in Python, discussing its usage, advantages, and potential security concerns. We’ve emphasized the importance of using eval() judiciously and provided best practices to mitigate risks when employing it in your code. As you continue to develop Python applications, remember that while eval() can be a valuable tool, it should be used sparingly and cautiously, especially when dealing with untrusted input. By mastering the art of eval() and following security best practices, you can harness its power effectively in your Python projects.
FAQs Related to Eval in Python
Here are some frequently asked questions on Python eval Function
1. Can I use eval() for complex operations, such as file I/O or network requests? It’s generally not recommended to use eval() for complex operations like file I/O or network requests. These operations should be handled using dedicated Python libraries and modules, as they can introduce security risks and are not its intended use.
2. What are some common use cases for the eval() function? Common use cases for eval() include dynamic configuration, mathematical calculations, parsing expressions from user input, and creating mini-interpreters or domain-specific languages.
3. What are the potential security risks associated with using eval()? Using eval() with untrusted or unsanitized input can lead to security vulnerabilities, as it allows execution of arbitrary code. This can lead to code injection and other malicious activities.
4. How can I use eval() safely in my Python code? To use eval() safely, avoid executing untrusted code, sanitize input, and validate expressions. Consider using alternatives like ast.literal_eval() when dealing with literals, and restrict eval() to known and safe inputs.
5. Are there alternatives to eval() in Python? Yes, Python provides alternatives like ast.literal_eval() for safely evaluating literals and exec() for executing dynamic code in a controlled environment. These alternatives may be more secure depending on your use case.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Python list functions & python list methods, python interview questions, namespaces and scope in python, what is the difference between append and extend in python, python program to check for the perfect square, python program to find the sum of first n natural numbers.
- Python »
- 3.14.0a0 Documentation »
- The Python Tutorial »
- Theme Auto Light Dark |
9. Classes ¶
Classes provide a means of bundling data and functionality together. Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state. Class instances can also have methods (defined by its class) for modifying its state.
Compared with other programming languages, Python’s class mechanism adds classes with a minimum of new syntax and semantics. It is a mixture of the class mechanisms found in C++ and Modula-3. Python classes provide all the standard features of Object Oriented Programming: the class inheritance mechanism allows multiple base classes, a derived class can override any methods of its base class or classes, and a method can call the method of a base class with the same name. Objects can contain arbitrary amounts and kinds of data. As is true for modules, classes partake of the dynamic nature of Python: they are created at runtime, and can be modified further after creation.
In C++ terminology, normally class members (including the data members) are public (except see below Private Variables ), and all member functions are virtual . As in Modula-3, there are no shorthands for referencing the object’s members from its methods: the method function is declared with an explicit first argument representing the object, which is provided implicitly by the call. As in Smalltalk, classes themselves are objects. This provides semantics for importing and renaming. Unlike C++ and Modula-3, built-in types can be used as base classes for extension by the user. Also, like in C++, most built-in operators with special syntax (arithmetic operators, subscripting etc.) can be redefined for class instances.
(Lacking universally accepted terminology to talk about classes, I will make occasional use of Smalltalk and C++ terms. I would use Modula-3 terms, since its object-oriented semantics are closer to those of Python than C++, but I expect that few readers have heard of it.)
9.1. A Word About Names and Objects ¶
Objects have individuality, and multiple names (in multiple scopes) can be bound to the same object. This is known as aliasing in other languages. This is usually not appreciated on a first glance at Python, and can be safely ignored when dealing with immutable basic types (numbers, strings, tuples). However, aliasing has a possibly surprising effect on the semantics of Python code involving mutable objects such as lists, dictionaries, and most other types. This is usually used to the benefit of the program, since aliases behave like pointers in some respects. For example, passing an object is cheap since only a pointer is passed by the implementation; and if a function modifies an object passed as an argument, the caller will see the change — this eliminates the need for two different argument passing mechanisms as in Pascal.
9.2. Python Scopes and Namespaces ¶
Before introducing classes, I first have to tell you something about Python’s scope rules. Class definitions play some neat tricks with namespaces, and you need to know how scopes and namespaces work to fully understand what’s going on. Incidentally, knowledge about this subject is useful for any advanced Python programmer.
Let’s begin with some definitions.
A namespace is a mapping from names to objects. Most namespaces are currently implemented as Python dictionaries, but that’s normally not noticeable in any way (except for performance), and it may change in the future. Examples of namespaces are: the set of built-in names (containing functions such as abs() , and built-in exception names); the global names in a module; and the local names in a function invocation. In a sense the set of attributes of an object also form a namespace. The important thing to know about namespaces is that there is absolutely no relation between names in different namespaces; for instance, two different modules may both define a function maximize without confusion — users of the modules must prefix it with the module name.
By the way, I use the word attribute for any name following a dot — for example, in the expression z.real , real is an attribute of the object z . Strictly speaking, references to names in modules are attribute references: in the expression modname.funcname , modname is a module object and funcname is an attribute of it. In this case there happens to be a straightforward mapping between the module’s attributes and the global names defined in the module: they share the same namespace! [ 1 ]
Attributes may be read-only or writable. In the latter case, assignment to attributes is possible. Module attributes are writable: you can write modname.the_answer = 42 . Writable attributes may also be deleted with the del statement. For example, del modname.the_answer will remove the attribute the_answer from the object named by modname .
Namespaces are created at different moments and have different lifetimes. The namespace containing the built-in names is created when the Python interpreter starts up, and is never deleted. The global namespace for a module is created when the module definition is read in; normally, module namespaces also last until the interpreter quits. The statements executed by the top-level invocation of the interpreter, either read from a script file or interactively, are considered part of a module called __main__ , so they have their own global namespace. (The built-in names actually also live in a module; this is called builtins .)
The local namespace for a function is created when the function is called, and deleted when the function returns or raises an exception that is not handled within the function. (Actually, forgetting would be a better way to describe what actually happens.) Of course, recursive invocations each have their own local namespace.
A scope is a textual region of a Python program where a namespace is directly accessible. “Directly accessible” here means that an unqualified reference to a name attempts to find the name in the namespace.
Although scopes are determined statically, they are used dynamically. At any time during execution, there are 3 or 4 nested scopes whose namespaces are directly accessible:
the innermost scope, which is searched first, contains the local names
the scopes of any enclosing functions, which are searched starting with the nearest enclosing scope, contain non-local, but also non-global names
the next-to-last scope contains the current module’s global names
the outermost scope (searched last) is the namespace containing built-in names
If a name is declared global, then all references and assignments go directly to the next-to-last scope containing the module’s global names. To rebind variables found outside of the innermost scope, the nonlocal statement can be used; if not declared nonlocal, those variables are read-only (an attempt to write to such a variable will simply create a new local variable in the innermost scope, leaving the identically named outer variable unchanged).
Usually, the local scope references the local names of the (textually) current function. Outside functions, the local scope references the same namespace as the global scope: the module’s namespace. Class definitions place yet another namespace in the local scope.
It is important to realize that scopes are determined textually: the global scope of a function defined in a module is that module’s namespace, no matter from where or by what alias the function is called. On the other hand, the actual search for names is done dynamically, at run time — however, the language definition is evolving towards static name resolution, at “compile” time, so don’t rely on dynamic name resolution! (In fact, local variables are already determined statically.)
A special quirk of Python is that – if no global or nonlocal statement is in effect – assignments to names always go into the innermost scope. Assignments do not copy data — they just bind names to objects. The same is true for deletions: the statement del x removes the binding of x from the namespace referenced by the local scope. In fact, all operations that introduce new names use the local scope: in particular, import statements and function definitions bind the module or function name in the local scope.
The global statement can be used to indicate that particular variables live in the global scope and should be rebound there; the nonlocal statement indicates that particular variables live in an enclosing scope and should be rebound there.
9.2.1. Scopes and Namespaces Example ¶
This is an example demonstrating how to reference the different scopes and namespaces, and how global and nonlocal affect variable binding:
The output of the example code is:
Note how the local assignment (which is default) didn’t change scope_test 's binding of spam . The nonlocal assignment changed scope_test 's binding of spam , and the global assignment changed the module-level binding.
You can also see that there was no previous binding for spam before the global assignment.
9.3. A First Look at Classes ¶
Classes introduce a little bit of new syntax, three new object types, and some new semantics.
9.3.1. Class Definition Syntax ¶
The simplest form of class definition looks like this:
Class definitions, like function definitions ( def statements) must be executed before they have any effect. (You could conceivably place a class definition in a branch of an if statement, or inside a function.)
In practice, the statements inside a class definition will usually be function definitions, but other statements are allowed, and sometimes useful — we’ll come back to this later. The function definitions inside a class normally have a peculiar form of argument list, dictated by the calling conventions for methods — again, this is explained later.
When a class definition is entered, a new namespace is created, and used as the local scope — thus, all assignments to local variables go into this new namespace. In particular, function definitions bind the name of the new function here.
When a class definition is left normally (via the end), a class object is created. This is basically a wrapper around the contents of the namespace created by the class definition; we’ll learn more about class objects in the next section. The original local scope (the one in effect just before the class definition was entered) is reinstated, and the class object is bound here to the class name given in the class definition header ( ClassName in the example).
9.3.2. Class Objects ¶
Class objects support two kinds of operations: attribute references and instantiation.
Attribute references use the standard syntax used for all attribute references in Python: obj.name . Valid attribute names are all the names that were in the class’s namespace when the class object was created. So, if the class definition looked like this:
then MyClass.i and MyClass.f are valid attribute references, returning an integer and a function object, respectively. Class attributes can also be assigned to, so you can change the value of MyClass.i by assignment. __doc__ is also a valid attribute, returning the docstring belonging to the class: "A simple example class" .
Class instantiation uses function notation. Just pretend that the class object is a parameterless function that returns a new instance of the class. For example (assuming the above class):
creates a new instance of the class and assigns this object to the local variable x .
The instantiation operation (“calling” a class object) creates an empty object. Many classes like to create objects with instances customized to a specific initial state. Therefore a class may define a special method named __init__() , like this:
When a class defines an __init__() method, class instantiation automatically invokes __init__() for the newly created class instance. So in this example, a new, initialized instance can be obtained by:
Of course, the __init__() method may have arguments for greater flexibility. In that case, arguments given to the class instantiation operator are passed on to __init__() . For example,
9.3.3. Instance Objects ¶
Now what can we do with instance objects? The only operations understood by instance objects are attribute references. There are two kinds of valid attribute names: data attributes and methods.
data attributes correspond to “instance variables” in Smalltalk, and to “data members” in C++. Data attributes need not be declared; like local variables, they spring into existence when they are first assigned to. For example, if x is the instance of MyClass created above, the following piece of code will print the value 16 , without leaving a trace:
The other kind of instance attribute reference is a method . A method is a function that “belongs to” an object. (In Python, the term method is not unique to class instances: other object types can have methods as well. For example, list objects have methods called append, insert, remove, sort, and so on. However, in the following discussion, we’ll use the term method exclusively to mean methods of class instance objects, unless explicitly stated otherwise.)
Valid method names of an instance object depend on its class. By definition, all attributes of a class that are function objects define corresponding methods of its instances. So in our example, x.f is a valid method reference, since MyClass.f is a function, but x.i is not, since MyClass.i is not. But x.f is not the same thing as MyClass.f — it is a method object , not a function object.
9.3.4. Method Objects ¶
Usually, a method is called right after it is bound:
In the MyClass example, this will return the string 'hello world' . However, it is not necessary to call a method right away: x.f is a method object, and can be stored away and called at a later time. For example:
will continue to print hello world until the end of time.
What exactly happens when a method is called? You may have noticed that x.f() was called without an argument above, even though the function definition for f() specified an argument. What happened to the argument? Surely Python raises an exception when a function that requires an argument is called without any — even if the argument isn’t actually used…
Actually, you may have guessed the answer: the special thing about methods is that the instance object is passed as the first argument of the function. In our example, the call x.f() is exactly equivalent to MyClass.f(x) . In general, calling a method with a list of n arguments is equivalent to calling the corresponding function with an argument list that is created by inserting the method’s instance object before the first argument.
In general, methods work as follows. When a non-data attribute of an instance is referenced, the instance’s class is searched. If the name denotes a valid class attribute that is a function object, references to both the instance object and the function object are packed into a method object. When the method object is called with an argument list, a new argument list is constructed from the instance object and the argument list, and the function object is called with this new argument list.
9.3.5. Class and Instance Variables ¶
Generally speaking, instance variables are for data unique to each instance and class variables are for attributes and methods shared by all instances of the class:
As discussed in A Word About Names and Objects , shared data can have possibly surprising effects with involving mutable objects such as lists and dictionaries. For example, the tricks list in the following code should not be used as a class variable because just a single list would be shared by all Dog instances:
Correct design of the class should use an instance variable instead:
9.4. Random Remarks ¶
If the same attribute name occurs in both an instance and in a class, then attribute lookup prioritizes the instance:
Data attributes may be referenced by methods as well as by ordinary users (“clients”) of an object. In other words, classes are not usable to implement pure abstract data types. In fact, nothing in Python makes it possible to enforce data hiding — it is all based upon convention. (On the other hand, the Python implementation, written in C, can completely hide implementation details and control access to an object if necessary; this can be used by extensions to Python written in C.)
Clients should use data attributes with care — clients may mess up invariants maintained by the methods by stamping on their data attributes. Note that clients may add data attributes of their own to an instance object without affecting the validity of the methods, as long as name conflicts are avoided — again, a naming convention can save a lot of headaches here.
There is no shorthand for referencing data attributes (or other methods!) from within methods. I find that this actually increases the readability of methods: there is no chance of confusing local variables and instance variables when glancing through a method.
Often, the first argument of a method is called self . This is nothing more than a convention: the name self has absolutely no special meaning to Python. Note, however, that by not following the convention your code may be less readable to other Python programmers, and it is also conceivable that a class browser program might be written that relies upon such a convention.
Any function object that is a class attribute defines a method for instances of that class. It is not necessary that the function definition is textually enclosed in the class definition: assigning a function object to a local variable in the class is also ok. For example:
Now f , g and h are all attributes of class C that refer to function objects, and consequently they are all methods of instances of C — h being exactly equivalent to g . Note that this practice usually only serves to confuse the reader of a program.
Methods may call other methods by using method attributes of the self argument:
Methods may reference global names in the same way as ordinary functions. The global scope associated with a method is the module containing its definition. (A class is never used as a global scope.) While one rarely encounters a good reason for using global data in a method, there are many legitimate uses of the global scope: for one thing, functions and modules imported into the global scope can be used by methods, as well as functions and classes defined in it. Usually, the class containing the method is itself defined in this global scope, and in the next section we’ll find some good reasons why a method would want to reference its own class.
Each value is an object, and therefore has a class (also called its type ). It is stored as object.__class__ .
9.5. Inheritance ¶
Of course, a language feature would not be worthy of the name “class” without supporting inheritance. The syntax for a derived class definition looks like this:
The name BaseClassName must be defined in a namespace accessible from the scope containing the derived class definition. In place of a base class name, other arbitrary expressions are also allowed. This can be useful, for example, when the base class is defined in another module:
Execution of a derived class definition proceeds the same as for a base class. When the class object is constructed, the base class is remembered. This is used for resolving attribute references: if a requested attribute is not found in the class, the search proceeds to look in the base class. This rule is applied recursively if the base class itself is derived from some other class.
There’s nothing special about instantiation of derived classes: DerivedClassName() creates a new instance of the class. Method references are resolved as follows: the corresponding class attribute is searched, descending down the chain of base classes if necessary, and the method reference is valid if this yields a function object.
Derived classes may override methods of their base classes. Because methods have no special privileges when calling other methods of the same object, a method of a base class that calls another method defined in the same base class may end up calling a method of a derived class that overrides it. (For C++ programmers: all methods in Python are effectively virtual .)
An overriding method in a derived class may in fact want to extend rather than simply replace the base class method of the same name. There is a simple way to call the base class method directly: just call BaseClassName.methodname(self, arguments) . This is occasionally useful to clients as well. (Note that this only works if the base class is accessible as BaseClassName in the global scope.)
Python has two built-in functions that work with inheritance:
Use isinstance() to check an instance’s type: isinstance(obj, int) will be True only if obj.__class__ is int or some class derived from int .
Use issubclass() to check class inheritance: issubclass(bool, int) is True since bool is a subclass of int . However, issubclass(float, int) is False since float is not a subclass of int .
9.5.1. Multiple Inheritance ¶
Python supports a form of multiple inheritance as well. A class definition with multiple base classes looks like this:
For most purposes, in the simplest cases, you can think of the search for attributes inherited from a parent class as depth-first, left-to-right, not searching twice in the same class where there is an overlap in the hierarchy. Thus, if an attribute is not found in DerivedClassName , it is searched for in Base1 , then (recursively) in the base classes of Base1 , and if it was not found there, it was searched for in Base2 , and so on.
In fact, it is slightly more complex than that; the method resolution order changes dynamically to support cooperative calls to super() . This approach is known in some other multiple-inheritance languages as call-next-method and is more powerful than the super call found in single-inheritance languages.
Dynamic ordering is necessary because all cases of multiple inheritance exhibit one or more diamond relationships (where at least one of the parent classes can be accessed through multiple paths from the bottommost class). For example, all classes inherit from object , so any case of multiple inheritance provides more than one path to reach object . To keep the base classes from being accessed more than once, the dynamic algorithm linearizes the search order in a way that preserves the left-to-right ordering specified in each class, that calls each parent only once, and that is monotonic (meaning that a class can be subclassed without affecting the precedence order of its parents). Taken together, these properties make it possible to design reliable and extensible classes with multiple inheritance. For more detail, see The Python 2.3 Method Resolution Order .
9.6. Private Variables ¶
“Private” instance variables that cannot be accessed except from inside an object don’t exist in Python. However, there is a convention that is followed by most Python code: a name prefixed with an underscore (e.g. _spam ) should be treated as a non-public part of the API (whether it is a function, a method or a data member). It should be considered an implementation detail and subject to change without notice.
Since there is a valid use-case for class-private members (namely to avoid name clashes of names with names defined by subclasses), there is limited support for such a mechanism, called name mangling . Any identifier of the form __spam (at least two leading underscores, at most one trailing underscore) is textually replaced with _classname__spam , where classname is the current class name with leading underscore(s) stripped. This mangling is done without regard to the syntactic position of the identifier, as long as it occurs within the definition of a class.
Name mangling is helpful for letting subclasses override methods without breaking intraclass method calls. For example:
The above example would work even if MappingSubclass were to introduce a __update identifier since it is replaced with _Mapping__update in the Mapping class and _MappingSubclass__update in the MappingSubclass class respectively.
Note that the mangling rules are designed mostly to avoid accidents; it still is possible to access or modify a variable that is considered private. This can even be useful in special circumstances, such as in the debugger.
Notice that code passed to exec() or eval() does not consider the classname of the invoking class to be the current class; this is similar to the effect of the global statement, the effect of which is likewise restricted to code that is byte-compiled together. The same restriction applies to getattr() , setattr() and delattr() , as well as when referencing __dict__ directly.
9.7. Odds and Ends ¶
Sometimes it is useful to have a data type similar to the Pascal “record” or C “struct”, bundling together a few named data items. The idiomatic approach is to use dataclasses for this purpose:
A piece of Python code that expects a particular abstract data type can often be passed a class that emulates the methods of that data type instead. For instance, if you have a function that formats some data from a file object, you can define a class with methods read() and readline() that get the data from a string buffer instead, and pass it as an argument.
Instance method objects have attributes, too: m.__self__ is the instance object with the method m() , and m.__func__ is the function object corresponding to the method.
9.8. Iterators ¶
By now you have probably noticed that most container objects can be looped over using a for statement:
This style of access is clear, concise, and convenient. The use of iterators pervades and unifies Python. Behind the scenes, the for statement calls iter() on the container object. The function returns an iterator object that defines the method __next__() which accesses elements in the container one at a time. When there are no more elements, __next__() raises a StopIteration exception which tells the for loop to terminate. You can call the __next__() method using the next() built-in function; this example shows how it all works:
Having seen the mechanics behind the iterator protocol, it is easy to add iterator behavior to your classes. Define an __iter__() method which returns an object with a __next__() method. If the class defines __next__() , then __iter__() can just return self :
9.9. Generators ¶
Generators are a simple and powerful tool for creating iterators. They are written like regular functions but use the yield statement whenever they want to return data. Each time next() is called on it, the generator resumes where it left off (it remembers all the data values and which statement was last executed). An example shows that generators can be trivially easy to create:
Anything that can be done with generators can also be done with class-based iterators as described in the previous section. What makes generators so compact is that the __iter__() and __next__() methods are created automatically.
Another key feature is that the local variables and execution state are automatically saved between calls. This made the function easier to write and much more clear than an approach using instance variables like self.index and self.data .
In addition to automatic method creation and saving program state, when generators terminate, they automatically raise StopIteration . In combination, these features make it easy to create iterators with no more effort than writing a regular function.
9.10. Generator Expressions ¶
Some simple generators can be coded succinctly as expressions using a syntax similar to list comprehensions but with parentheses instead of square brackets. These expressions are designed for situations where the generator is used right away by an enclosing function. Generator expressions are more compact but less versatile than full generator definitions and tend to be more memory friendly than equivalent list comprehensions.
Table of Contents
- 9.1. A Word About Names and Objects
- 9.2.1. Scopes and Namespaces Example
- 9.3.1. Class Definition Syntax
- 9.3.2. Class Objects
- 9.3.3. Instance Objects
- 9.3.4. Method Objects
- 9.3.5. Class and Instance Variables
- 9.4. Random Remarks
- 9.5.1. Multiple Inheritance
- 9.6. Private Variables
- 9.7. Odds and Ends
- 9.8. Iterators
- 9.9. Generators
- 9.10. Generator Expressions
Previous topic
8. Errors and Exceptions
10. Brief Tour of the Standard Library
- Report a Bug
- Show Source
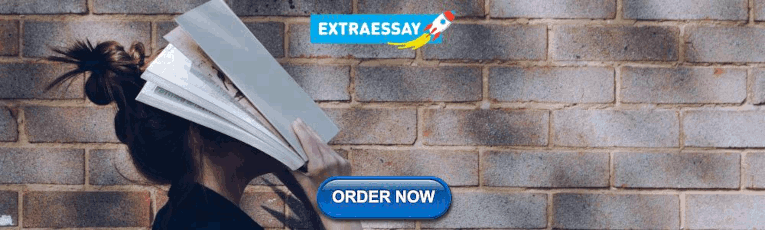
IMAGES
VIDEO
COMMENTS
You can't, since variable assignment is a statement, not an expression, and eval can only eval expressions. Use exec instead. Better yet, don't use either and tell us what you're really trying to do so that we can come up with a safe and sane solution.
Python's eval() allows you to evaluate arbitrary Python expressions from a string-based or compiled-code-based input. This function can be handy when you're trying to dynamically evaluate Python expressions from any input that comes as a string or a compiled code object.. Although Python's eval() is an incredibly useful tool, the function has some important security implications that you ...
Python's eval function evaluates expressions. It doesn't execute statements. Assignment is a statement, not an expression. That explains the problem you're having. In general. making a global change using eval is A Really Bad Idea. -
eval() Parameters. The eval() function takes three parameters:. expression - the string parsed and evaluated as a Python expression; globals (optional) - a dictionary; locals (optional)- a mapping object. Dictionary is the standard and commonly used mapping type in Python. The use of globals and locals will be discussed later in this article.
This function returns the result on evaluating the input expression. Let us see some examples of using the eval () function. Example of eval () function: eval('3*8') Output: 24. In this example, the expression is 3*8 which is 24. So, the output is 24. We can also give sequence and variables as the inputs to this function.
Related Tutorial: Python's eval() built-in function. Without further ado, let's learn how you can store the result of the eval() function in a Python variable:. Method 1: Simple Assignment. The most straightforward way to store the result of an eval() expression in a Python variable is to as s ign the whole return value to the variable. For example, the expression my_result = eval('2+2 ...
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
The built-in Python function eval() is used to evaluate Python expressions. You can pass a string containing Python, or a pre-compiled object into eval() and it will run the code and return the result.. Although Python's eval() is an incredibly useful tool, the function has some important security implications that you should consider before using it. . In this course, you'll learn how ...
Python eval() function converts and computes the expressions passed to it. NOTE: Use this method only for testing purposes. The eval() function does not sanitize the expressions passed to it. ... If the python expression turns out to be an assignment expression, inplace decides to perform the operation and mutate the data frame object. If FALSE ...
The eval() function returns the value of a Python expression passed as a string.. Security Concerns. While eval() can be useful, care must be taken to understand the security implications of this function. If eval() is used with user-generated strings, a malicious user could execute arbitrary code through the function. Good programming practice generally advises against using eval().
The eval() function in Python is used to evaluate a dynamically provided Python expression or statement stored as a string. It takes a single argument, which is the string to be evaluated, and returns the result of the evaluation. The evaluated expression can be a mathematical operation, a conditional statement, or even a series of function ...
eval() evaluates the passed string as a Python expression and returns the result. For example, eval("1 + 1") interprets and executes the expression "1 + 1" and returns the result (2). One reason you might be confused is because the code you cited involves a level of indirection.
Output: x*(x+1)*(x+2) 60. Evaluate Boolean Expressions in Python. Here the eval statement x == 4 will evaluate to False because the value of x is 5, which is not equal to 4. In the second eval statement, x is None will evaluate to True because the value of x is None, and is None checks for object identity, not value equality.
Think of Python's eval function as a powerful calculator - it can evaluate expressions dynamically, making it a versatile tool in your Python toolkit. Whether you're looking to evaluate simple mathematical expressions or complex code snippets, understanding how to use Python's eval function can significantly enhance your coding efficiency.
Python eval() function. Updated on Jan 07, 2020 The eval() allows us to execute arbitrary strings as Python code. It accepts a source string and returns an object. ... >>> >>> eval ('a=1') # assignment statement Traceback (most recent call last): File "<stdin>", line 1, in < module > File "<string>", line 1 a = 1 ^ SyntaxError: ...
The eval in Python is a built-in function that evaluates a string as a Python expression and returns the result. It takes a single parameter which is a string containing a Python expression or statement to be evaluated. The expression can include any valid Python code, such as arithmetic operations, function calls, and control structures.
1. What is eval in python and what is its syntax? Answer: eval is a built-in- function used in python, eval function parses the expression argument and evaluates it as a python expression. In simple words, the eval function evaluates the "String" like a python expression and returns the result as an integer. Syntax. The syntax of the eval ...
00:00 In the previous lesson, I showed you how to build a quick little command line calculator. In this lesson, I'll summarize the course. 00:08 This course has been about the built-in function eval() and how to use it inside of Python to evaluate expressions. Expressions are a subset of the Python language and do not include statements. 00:22 Generally, expressions are things that evaluate ...
Evaluate a Python expression as a string using various backends. ... This is the target object for assignment. It is used when there is variable assignment in the expression. If so, then target must support item assignment with string keys, and if a copy is being returned, ...
The expression string to evaluate. inplace bool, default False. If the expression contains an assignment, whether to perform the operation inplace and mutate the existing DataFrame. Otherwise, a new DataFrame is returned. **kwargs. See the documentation for eval() for complete details on the keyword arguments accepted by query(). Returns:
The reason eval and exec are so dangerous is that the default compile function will generate bytecode for any valid python expression, and the default eval or exec will execute any valid python bytecode. All the answers to date have focused on restricting the bytecode that can be generated (by sanitizing input) or building your own domain-specific-language using the AST.
Note how the local assignment (which is default) didn't change scope_test's binding of spam.The nonlocal assignment changed scope_test's binding of spam, and the global assignment changed the module-level binding.. You can also see that there was no previous binding for spam before the global assignment.. 9.3. A First Look at Classes¶. Classes introduce a little bit of new syntax, three new ...
26. eval() only allows for expressions. Assignment is not an expression but a statement; you'd have to use exec instead. Even then you could use the globals() dictionary to add names to the global namespace and you'd not need to use any arbitrary expression execution. You really don't want to do this, you need to keep data out of your variable ...
ast.walk won't help you, because when it yields an AST node, you have no idea where in the AST it is, but you definitely want to do different things with a node depending on, e.g. whether it's on the left or right of the =.. You'll probably need to write a (recursive) function to 'evaluate' an AST node, where the action to perform depends on the type of the node.