- Books Get Your Hands Dirty on Clean Architecture Stratospheric
- Contribute Become an Author Writing Guide Author Workflow Author Payment
- Services Book me Advertise
- Categories Spring Boot Java Node Kotlin AWS Software Craft Simplify! Meta Book Reviews
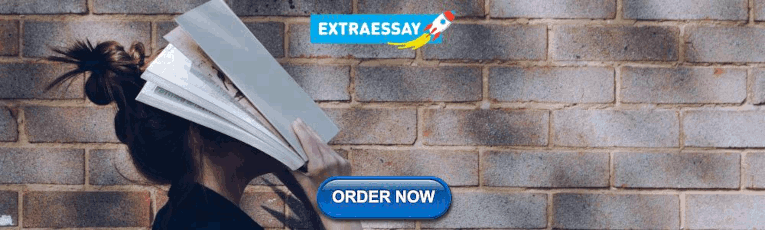
Creating and Analyzing Java Heap Dumps
- March 1, 2021
As Java developers, we are familiar with our applications throwing OutOfMemoryErrors or our server monitoring tools throwing alerts and complaining about high JVM memory utilization.
To investigate memory problems, the JVM Heap Memory is often the first place to look at.
To see this in action, we will first trigger an OutOfMemoryError and then capture a heap dump. We will next analyze this heap dump to identify the potential objects which could be the cause of the memory leak.
Example Code
What is a heap dump.
Whenever we create a Java object by creating an instance of a class, it is always placed in an area known as the heap. Classes of the Java runtime are also created in this heap.
The heap gets created when the JVM starts up. It expands or shrinks during runtime to accommodate the objects created or destroyed in our application.
When the heap becomes full, the garbage collection process is run to collect the objects that are not referenced anymore (i.e. they are not used anymore). More information on memory management can be found in the Oracle docs .
Heap dumps contain a snapshot of all the live objects that are being used by a running Java application on the Java heap. We can obtain detailed information for each object instance, such as the address, type, class name, or size, and whether the instance has references to other objects.
Heap dumps have two formats:
- the classic format, and
- the Portable Heap Dump (PHD) format.
PHD is the default format. The classic format is human-readable since it is in ASCII text, but the PHD format is binary and should be processed by appropriate tools for analysis.
Sample Program to Generate an OutOfMemoryError
To explain the analysis of a heap dump, we will use a simple Java program to generate an OutOfMemoryError :
We keep on allocating the memory by running a for loop until a point is reached, when JVM does not have enough memory to allocate, resulting in an OutOfMemoryError being thrown.
Finding the Root Cause of an OutOfMemoryError
We will now find the cause of this error by doing a heap dump analysis. This is done in two steps:
- Capture the heap dump
- Analyze the heap dump file to locate the suspected reason.
We can capture heap dump in multiple ways. Let us capture the heap dump for our example first with jmap and then by passing a VM argument in the command line.
Generating a Heap Dump on Demand with jmap
jmap is packaged with the JDK and extracts a heap dump to a specified file location.
To generate a heap dump with jmap , we first find the process ID of our running Java program with the jps tool to list down all the running Java processes on our machine:
Next, we run the jmap command to generate the heap dump file:
After running this command the heap dump file with extension hprof is created.
The option live is used to collect only the live objects that still have a reference in the running code. With the live option, a full GC is triggered to sweep away unreachable objects and then dump only the live objects.
Automatically Generating a Heap Dump on OutOfMemoryError s
This option is used to capture a heap dump at the point in time when an OutOfMemoryError occurred. This helps to diagnose the problem because we can see what objects were sitting in memory and what percentage of memory they were occupying right at the time of the OutOfMemoryError .
We will use this option for our example since it will give us more insight into the cause of the crash.
Let us run the program with the VM option HeapDumpOnOutOfMemoryError from the command line or our favorite IDE to generate the heap dump file:
After running our Java program with these VM arguments, we get this output:
As we can see from the output, the heap dump file with the name: hdump.hprof is created when the OutOfMemoryError occurs.
Other Methods of Generating Heap Dumps
Some of the other methods of generating a heap dump are:
jcmd : jcmd is used to send diagnostic command requests to the JVM. It is packaged as part of the JDK. It can be found in the \bin folder of a Java installation.
JVisualVM : Usually, analyzing heap dump takes more memory than the actual heap dump size. This could be problematic if we are trying to analyze a heap dump from a large server on a development machine. JVisualVM provides a live sampling of the Heap memory so it does not eat up the whole memory.
Analyzing the Heap Dump
What we are looking for in a Heap dump is:
- Objects with high memory usage
- Object graph to identify objects of not releasing memory
- Reachable and unreachable objects
Eclipse Memory Analyzer (MAT) is one of the best tools to analyze Java heap dumps. Let us understand the basic concepts of Java heap dump analysis with MAT by analyzing the heap dump file we generated earlier.
We will first start the Memory Analyzer Tool and open the heap dump file. In Eclipse MAT, two types of object sizes are reported:
- Shallow heap size : The shallow heap of an object is its size in the memory
- Retained heap size : Retained heap is the amount of memory that will be freed when an object is garbage collected.
Overview Section in MAT
After opening the heap dump, we will see an overview of the application’s memory usage. The piechart shows the biggest objects by retained size in the overview tab as shown here:
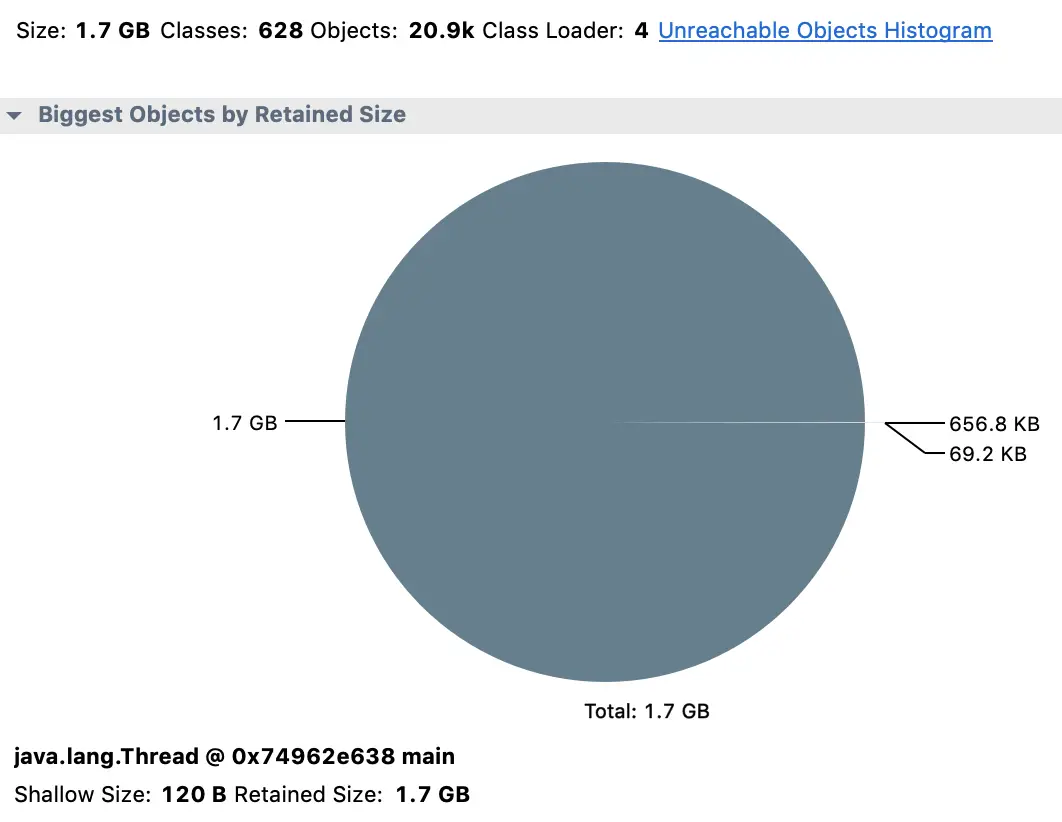
For our application, this information in the overview means if we could dispose of a particular instance of java.lang.Thread we will save 1.7 GB, and almost all of the memory used in this application.
Histogram View
While that might look promising, java.lang.Thread is unlikely to be the real problem here. To get a better insight into what objects currently exist, we will use the Histogram view:
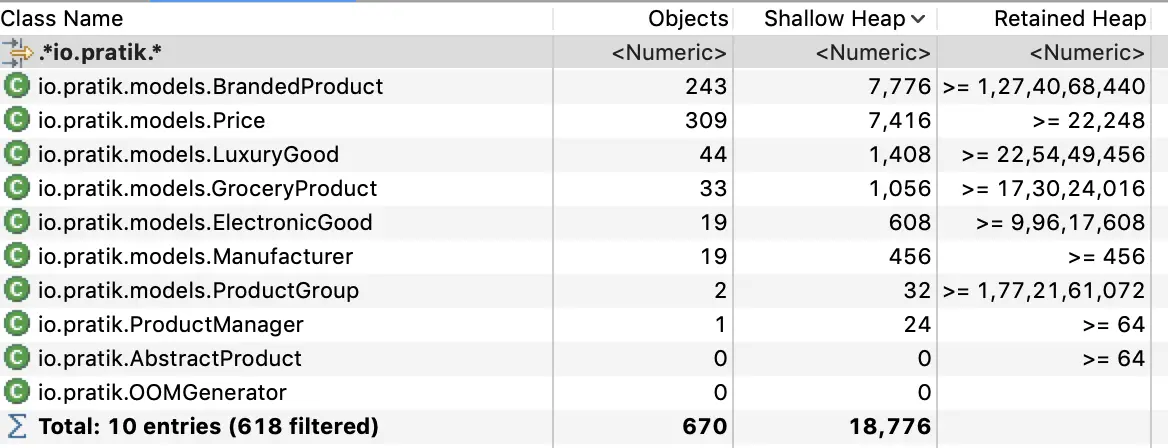
We have filtered the histogram with a regular expression “io.pratik.* " to show only the classes that match the pattern. With this view, we can see the number of live objects: for example, 243 BrandedProduct objects, and 309 Price Objects are alive in the system. We can also see the amount of memory each object is using.
There are two calculations, Shallow Heap and Retained Heap. A shallow heap is the amount of memory consumed by one object. An Object requires 32 (or 64 bits, depending on the architecture) for each reference. Primitives such as integers and longs require 4 or 8 bytes, etc… While this can be interesting, the more useful metric is the Retained Heap.
Retained Heap Size
The retained heap size is computed by adding the size of all the objects in the retained set. A retained set of X is the set of objects which would be removed by the Garbage Collector when X is collected.
The retained heap can be calculated in two different ways, using the quick approximation or the precise retained size:
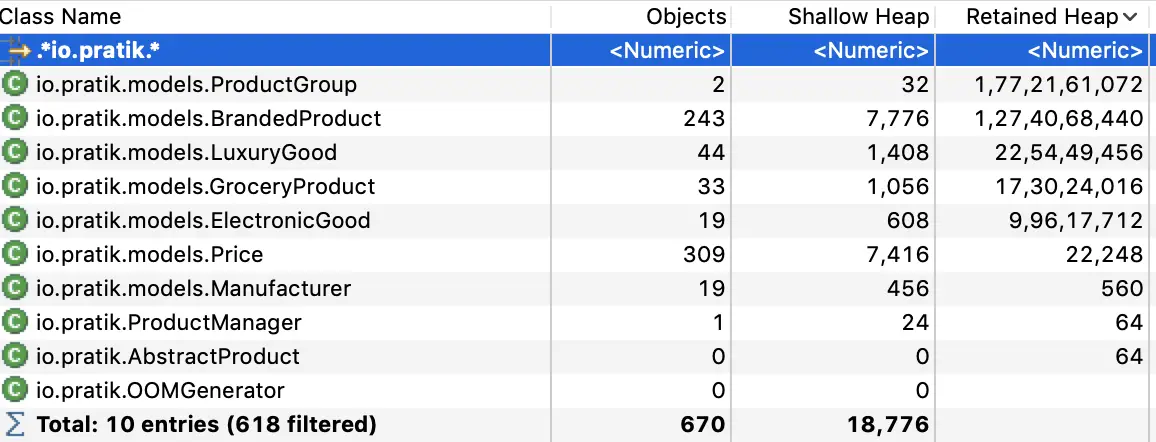
By calculating the Retained Heap we can now see that io.pratik.ProductGroup is holding the majority of the memory, even though it is only 32 bytes (shallow heap size) by itself. By finding a way to free up this object, we can certainly get our memory problem under control.
Dominator Tree
The dominator tree is used to identify the retained heap. It is produced by the complex object graph generated at runtime and helps to identify the largest memory graphs. An Object X is said to dominate an Object Y if every path from the Root to Y must pass through X.
Looking at the dominator tree for our example, we can see which objects are retained in the memory.
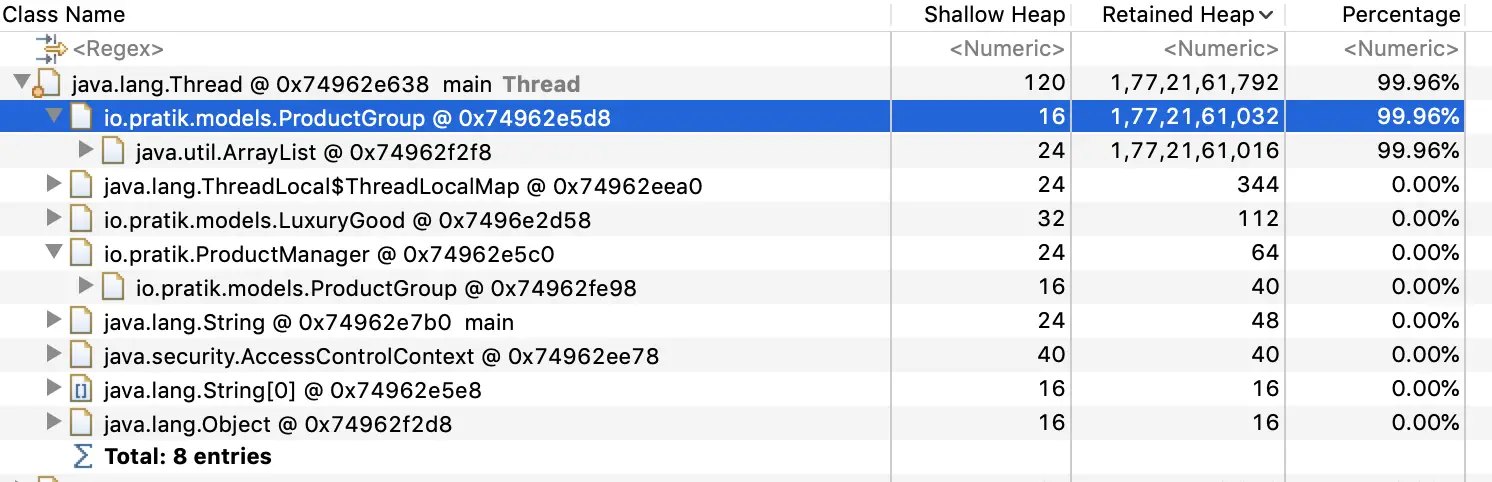
We can see that the ProductGroup object holds the memory instead of the Thread object. We can probably fix the memory problem by releasing objects contained in this object.
Leak Suspects Report
We can also generate a “Leak Suspects Report” to find a suspected big object or set of objects. This report presents the findings on an HTML page and is also saved in a zip file next to the heap dump file.
Due to its smaller size, it is preferable to share the “Leak Suspects Report” report with teams specialized in performing analysis tasks instead of the raw heap dump file.
The report has a pie chart, which gives the size of the suspected objects:
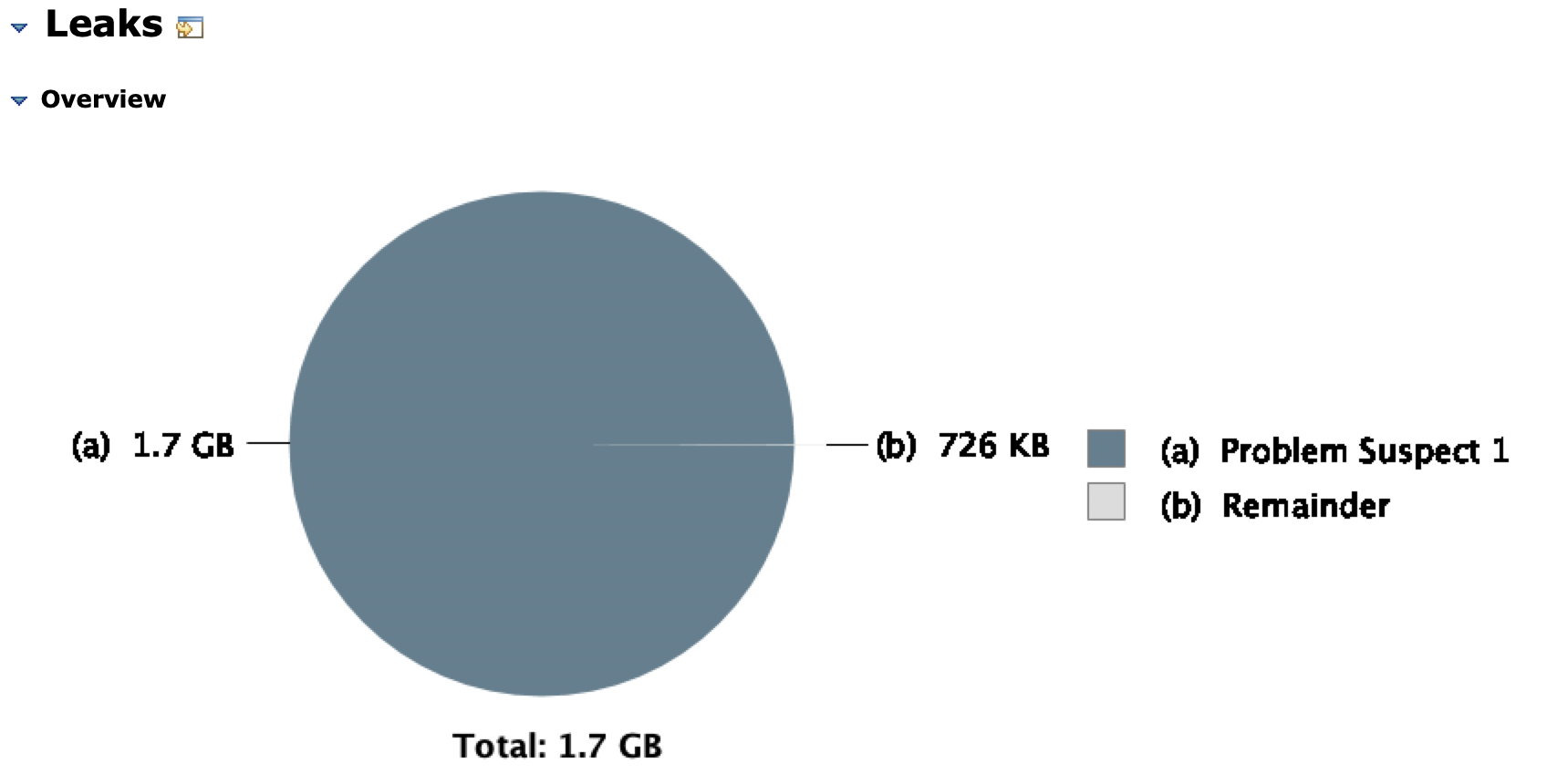
For our example, we have one suspect labeled as “Problem Suspect 1” which is further described with a short description:
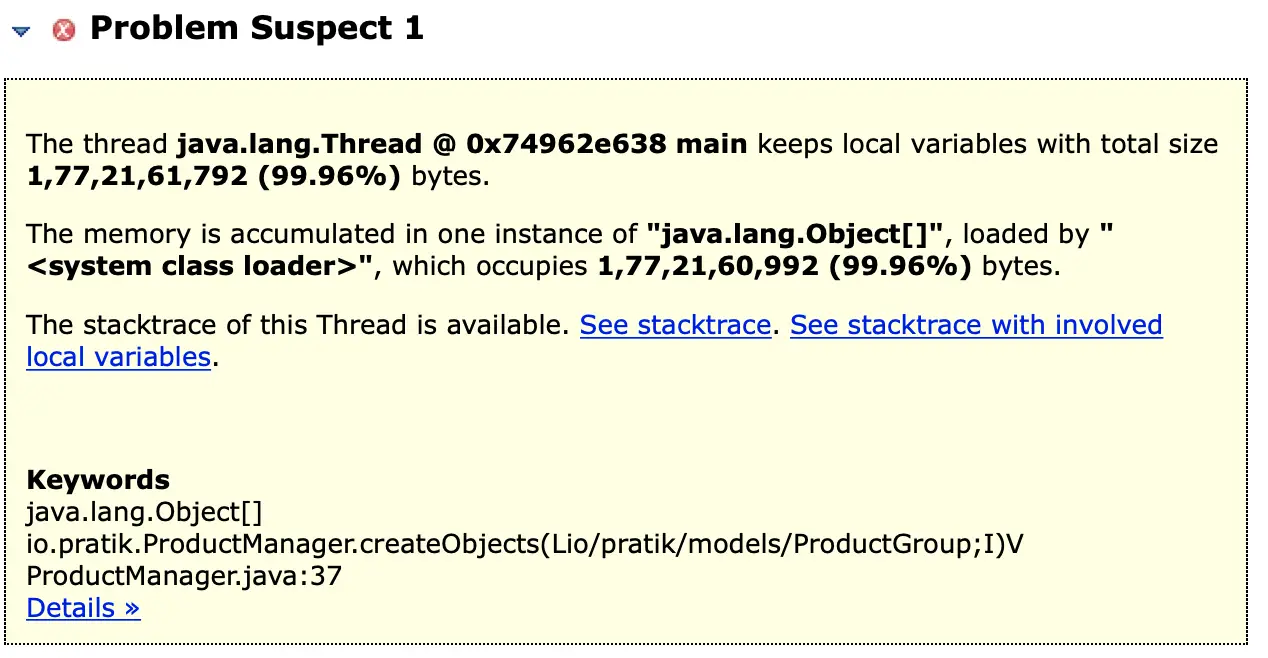
Apart from the summary, this report also contains detailed information about the suspects which is accessed by following the “details” link at the bottom of the report:
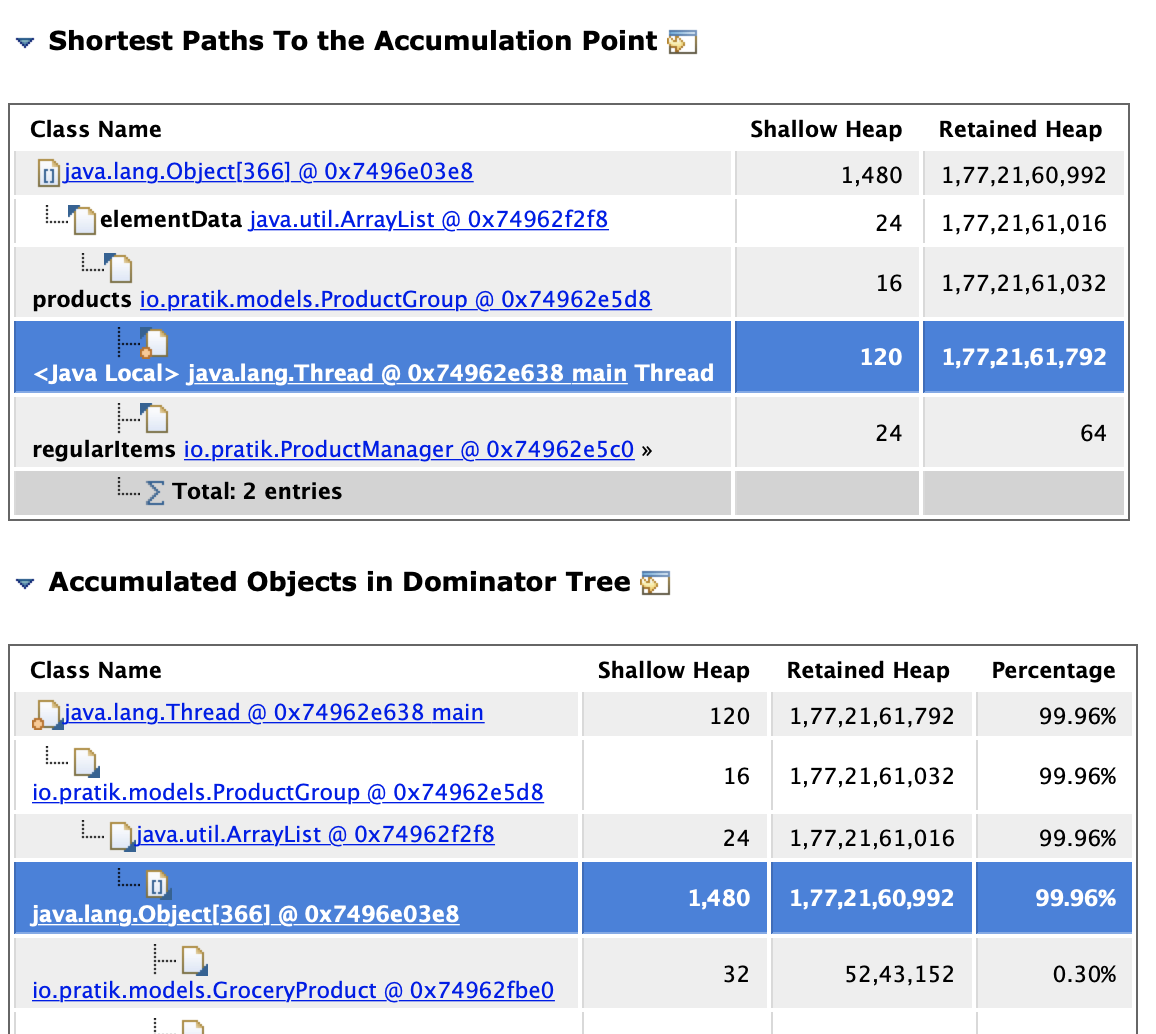
The detailed information is comprised of :
Shortest paths from GC root to the accumulation point : Here we can see all the classes and fields through which the reference chain is going, which gives a good understanding of how the objects are held. In this report, we can see the reference chain going from the Thread to the ProductGroup object.
Accumulated Objects in Dominator Tree : This gives some information about the content which is accumulated which is a collection of GroceryProduct objects here.
In this post, we introduced the heap dump, which is a snapshot of a Java application’s object memory graph at runtime. To illustrate, we captured the heap dump from a program that threw an OutOfMemoryError at runtime.
We then looked at some of the basic concepts of heap dump analysis with Eclipse Memory Analyzer: large objects, GC roots, shallow vs. retained heap, and dominator tree, all of which together will help us to identify the root cause of specific memory issues.
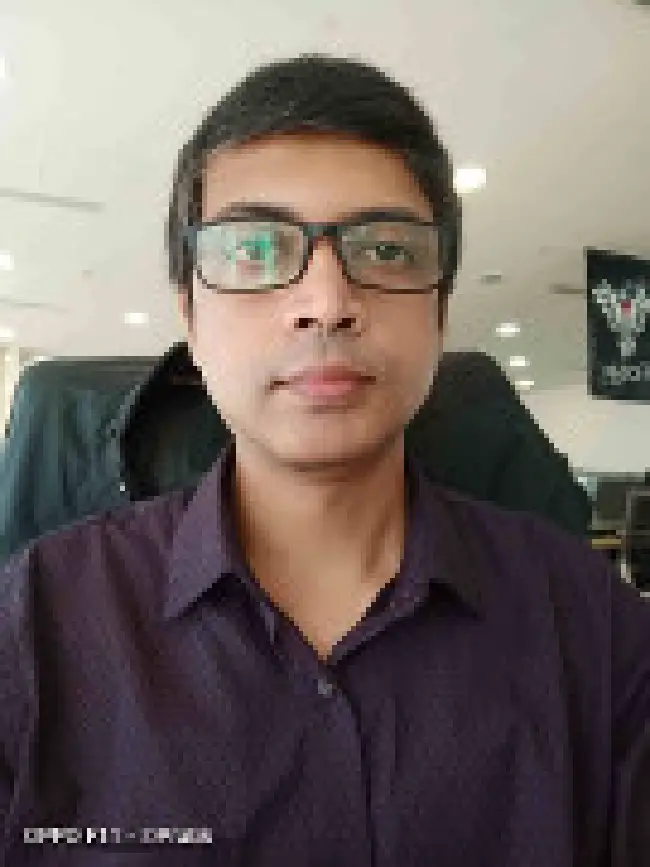
Software Engineer, Consultant and Architect with current expertise in Enterprise and Cloud Architecture, serverless technologies, Microservices, and Devops.
Recent Posts
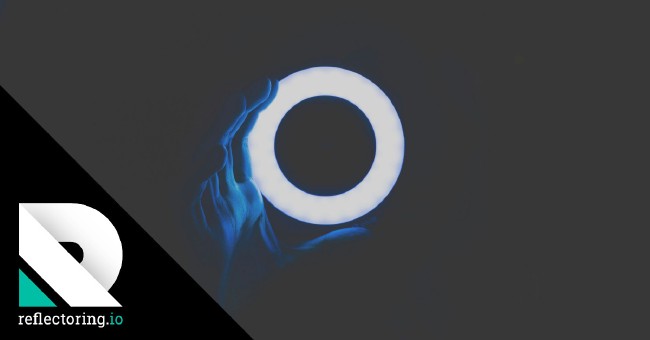
Understanding Null Safety in Kotlin
- Ezra Kanake
- March 2, 2024
One of the standout features that sets Kotlin apart is its robust approach to null safety. Null safety is a critical aspect of programming languages, aiming to eliminate the notorious null pointer exceptions that often plague developers.
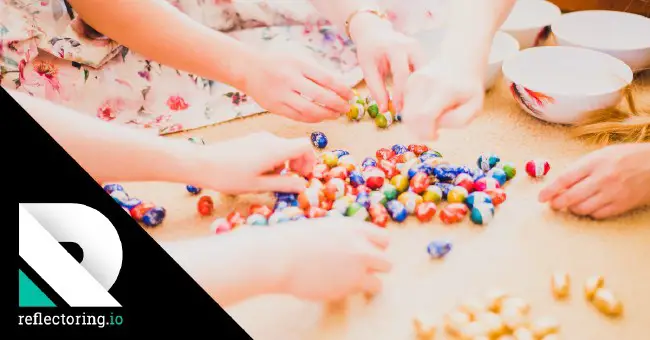
Merge Sort in Kotlin
- February 20, 2024
Sorting is a fundamental operation that plays a crucial role in various applications. Among the many sorting algorithms, merge sort stands out for its efficiency and simplicity.
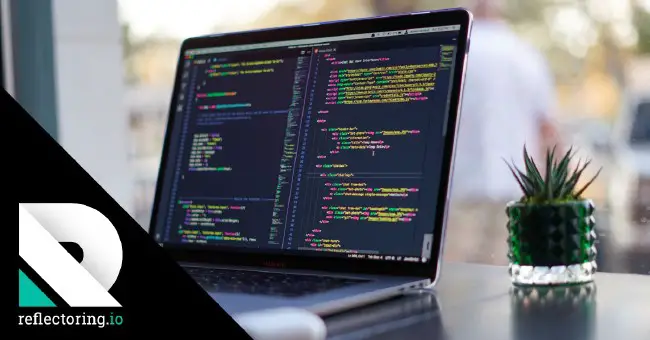
Extension Functions in Kotlin
- February 13, 2024
One of Kotlin’s standout features is extension functions, a mechanism that empowers developers to enhance existing classes without modifying their source code.
- Configuring your system
- JIT Compiler
- JITServer technology
- JITServer tuning
- AOT Compiler
- Java Attach API
- System dump
- Java 11 API
- Java 17 API
- Java 21 API
Heap dumps contain a snapshot of all the live objects that are being used by a running Java™ application on the Java heap. You can obtain detailed information for each object instance, such as the address, type, class name, or size, and whether the instance has references to other objects.
There are two formats for heap dumps; the classic format and the Portable Heap Dump (PHD) format, which is the default. Whilst the classic format is generated in ascii text and can be read, the PHD format is binary and and must be processed for analysis.
Obtaining dumps
Heap dumps are generated by default in PHD format when the Java heap runs out of space. If you want to trigger the production of a heap dump in response to other situations, or in classic format, you can use one of the following options:
- Configure the heap dump agent. For more information, see the -Xdump option.
- Use the com.ibm.jvm.Dump API programmatically in your application code. For more information, see the JVM diagnostic utilities API documentation .
Analyzing dumps
The best method to analyze a PHD heap dump is to use the Eclipse Memory Analyzer™ tool (MAT) or the IBM Memory Analyzer tool . These tools process the dump file and provide a visual representation of the objects in the Java Heap. Both tools require the Diagnostic Tool Framework for Java (DTFJ) plugin. To install the DTFJ plugin in the Eclipse IDE, select the following menu items:
The following sections contain detailed information about the content of each type of heap dump file.
Portable Heap Dump (PHD) format
A PHD format dump file contains a header section and a body section. The body section can contain information about object, array, or class records. Primitive numbers are used to describe the file format, as detailed in the following table:
General structure
The following structure comprises the header section of a PHD file:
- A UTF string indicating that the file is a portable heap dump
- An int containing the PHD version number
- 1 indicates that the word length is 64-bit.
- 2 indicates that all the objects in the dump are hashed. This flag is set for heap dumps that use 16-bit hash codes. Eclipse OpenJ9™ heap dumps use 32-bit hash codes that are created only when used. For example, these hash codes are created when the APIs Object.hashCode() or Object.toString() are called in a Java application. If this flag is not set, the presence of a hash code is indicated by the hash code flag on the individual PHD records.
- 4 indicates that the dump is from an OpenJ9 VM.
- A byte containing a tag with a value of 1 that indicates the start of the header.
- header tag 1 - not used
- header tag 2 - indicates the end of the header
- header tag 3 - not used
- header tag 4 - indicates the VM version (Variable length UTF string)
The body of a PHD file is indicated by a byte that contains a tag with a value of 2, after which there are a number of dump records. Dump records are preceded by a 1 byte tag with the following record types:
- Short object: 0x80 bit of the tag is set
- Medium object: 0x40 bit of the tag is set (top bit value is 0)
- Primitive Array: 0x20 bit if the tag is set (all other tag values have the top 3 bits with a value of 0)
- Long record: tag value is 4
- Class record: tag value is 6
- Long primitive array: tag value is 7
- Object array: tag value is 8
These records are described in more detail in the sections that follow.
The end of the PHD body is indicated by a byte that contains a tag with a value of 3.
Object records
Object records can be short, medium, or long, depending on the number of object references in the heap dump.
1. Short object record
The following information is contained within the tag byte:
The 1 byte tag, which consists of the following bits:
A byte or a short containing the gap between the address of this object and the address of the preceding object. The value is signed and represents the number of 32-bit words between the two addresses. Most gaps fit into 1 byte.
- If all objects are hashed, a short containing the hash code.
- The array of references, if references exist. The tag shows the number of elements, and the size of each element. The value in each element is the gap between the address of the references and the address of the current object. The value is a signed number of 32-bit words. Null references are not included.
2. Medium object record
These records provide the actual address of the class rather than a cache index. The following format is used:
The 1 byte tag, consisting of the following bits:
A byte or a short containing the gap between the address of this object and the address of the preceding object (See the Short object record description)
- A word containing the address of the class of this object.
- The array of references (See the Short object record description).
3. Long object record
This record format is used when there are more than 7 references, or if there are extra flags or a hash code. The following format is used:
The 1 byte tag, containing the value 4.
A byte containing flags, consisting of the following bits:
A byte , short , int , or long containing the gap between the address of this object and the address of the preceding object (See the Short object record description).
- If all objects are hashed, a short containing the hash code. Otherwise, an optional int containing the hash code if the hashed and moved bit is set in the record flag byte.
- An int containing the length of the array of references.
Array records
PHD arrays can be primitive arrays or object arrays, as described in the sections that follow.
1. Primitive array record
The following information is contained in an array record:
byte , short , int or long containing the gap between the address of this object and the address of the preceding object (See the Short object record description).
- byte , short , int or long containing the array length.
- An unsigned int containing the size of the instance of the array on the heap, including header and padding. The size is measured in 32-bit words, which you can multiply by four to obtain the size in bytes. This format allows encoding of lengths up to 16GB in an unsigned int .
2. Long primitive array record
This type of record is used when a primitive array has been hashed.
The 1 byte tag with a value of 7.
A byte containing the following flags:
a byte or word containing the gap between the address of this object and the address of the preceding object (See the Short object record description).
- a byte or word containing the array length.
3. Object array record
The following format applies:
The 1 byte tag with a value of 8.
A byte , short , int or long containing the gap between the address of this object and the address of the preceding object (See the Short object record format description).
- A word containing the address of the class of the objects in the array. Object array records do not update the class cache.
- If all objects are hashed, a short containing the hash code. If the hashed and moved bit is set in the records flag, this field contains an int .
- An final int value is shown at the end. This int contains the true array length, shown as a number of array elements. The true array length might differ from the length of the array of references because null references are excluded.
Class records
The PHD class record encodes a class object and contains the following format:
The 1 byte tag, containing the value 6.
A byte, short , int or long containing the gap between the address of this class and the address of the preceding object (See the Short object record description).
- An int containing the instance size.
- A word containing the address of the superclass.
- A UTF string containing the name of this class.
- An int containing the number of static references.
- The array of static references (See the Short object record description).
Classic Heap Dump format
Classic heap dumps are produced in ascii text on all platforms except z/OS, which are encoded in EBCDIC. The dump is divided into the following sections:
Header record
A single string containing information about the runtime environment, platform, and build levels, similar to the following example:
A record of each object instance in the heap with the following format:
The following object types ( object type ) might be shown:
- class name (including package name)
- class array type
- primitive array type
These types are abbreviated in the record. To determine the type, see the Java VM Type Signature table .
Any references found are also listed, excluding references to an object's class or NULL references.
The following example shows an object instance (16 bytes in length) of type java/lang/String , with a reference to a char array:
The object instance (length 32 bytes) of type char array, as referenced from the java/lang/String , is shown in the following example:
The following example shows an object instance (24 bytes in length) of type array of java/lang/String :
A record of each class in the following format:
The following class types ( <class type> ) might be shown:
- primitive array types
Any references found in the class block are also listed, excluding NULL references.
The following example shows a class object (80 bytes in length) for java/util/Date , with heap references:
Trailer record 1
A single record containing record counts, in decimal.
For example:
Trailer record 2
A single record containing totals, in decimal.
The values in the example reflect the following counts:
- 7147 total objects
- 22040 total references
- (12379) total NULL references as a proportion of the total references count
Java VM Type Signatures
The following table shows the abbreviations used for different Java types in the heap dump records:
- DTFJ interface
Andy Balaam's Blog
Four in the morning, still writing Free Software
How to analyse a .phd heap dump from an IBM JVM
Share on Mastodon
If you have been handed a .phd file which is a dump of the heap of an IBM Java virtual machine, you can analyse it using the Eclipse Memory Analyzer Tool (MAT), but you must install the IBM Monitoring and Diagnostic Tools first.
Download MAT from eclipse.org/mat/downloads.php . I suggest the Standalone version.
Unzip it and run the MemoryAnalyzer executable inside the zip. Add an argument to control how much memory it gets e.g. to give it 4GB:
Once it’s started, go to Help -> Install new software.
Next to “Work with” paste in the URL for the IBM Developer Toolkit update site: http://public.dhe.ibm.com/ibmdl/export/pub/software/websphere/runtimes/tools/dtfj/
Click Add…
Type in a name like “IBM Monitoring and Diagnostic Tools” and click OK.
In the list below, an item should appear called IBM Monitoring and Diagnostic Tools. Tick the box next to it, click Next, and follow the wizard to accept the license agreements and install the toolkit.
Restart Eclipse when prompted.
Choose File -> Open Heap Dump and choose your .phd file. It should open in MAT and allow you to figure out who is using all that memory.
9 thoughts on “How to analyse a .phd heap dump from an IBM JVM”
Very helpful guide.
Very nice buddy! Thank you!
If need any help on HEAPDUMP and JAVACORE for WebSphere products, please contact me!
https://www.linkedin.com/in/dougcardoso21/
Thanks Douglas!
Thanks for this… IBM product is garbage (no pun intended).
Thanks you !!
When I tried to update ini file to 4g, it did not open MAT. I needed to reset to what it was that is 1024m and then when I opened this phd file, it gave an error that Error opening heap dump is encountered. Does someone know what to do?
Very helpful , thanks.
Hi Poonam If you are on windows, type cmd in the search , go into command prompt. In the command prompt , change directory (cd) to the directory that MemoryAnalyser.exe is in. Then type MemoryAnalyzer -vmargs -Xmx4g and press enter.
- Pingback: ¿Cómo crear un volcado de almacenamiento dinámico compatible con OpenJ9 a través de API? – stack
Leave a Reply
Your email address will not be published. Required fields are marked *
Don't subscribe All new comments Replies to my comments Notify me of followup comments via e-mail. You can also subscribe without commenting.
This site uses Akismet to reduce spam. Learn how your comment data is processed .

Memory Analyzer – Standalone
Eclipse memory analyzer – standalone installation.
In this tutorial let’s see how to
- Download and start working on Eclipse Memory Analyzer – Standalone version.
- Open a java heap dump created out of sun/oracle jdk (*.hprof) and a heap dump created out of IBM jdk (*.phd) files.
Search for “eclipse memory analyzer” and download “Windows (x86_64)” version (if the windows machine has 64 bit jdk) from https://eclipse.org/mat/downloads.php

Save file and unzip it.

Launch MemoryAnalyzer.exe

If the default java version is 1.7 or greater, MemoryAnalyzer will start without any issues.

Now, we are all set to open a heap dump (*.hprof) generated out of sun/oracle jdk. But before opening lets increase the Max Java heap size argument in “MemoryAnalyzer.ini”. (If needed).
-vmargs -Xmx1024m
Navigate to File -> Open Heap Dump . Select the hprof file.

Once we select the hprof file, it may take 15-20 minutes depending on the heap dump size and CPU of the local machine, to complete analyzing and open the report as shown below.

To Open a IBM JVM Heap dump – (Portable Heap Dump (phd) format)
IBM heap dumps are generated in *.phd file format. To open *.phd heap dumps, we need to install IBM Diagnostic tool framework for java (dtfj), from the below URL.
http://public.dhe.ibm.com/ibmdl/export/pub/software/websphere/runtimes/tools/dtfj/
In Eclipse Memory Analyzer Window, Navigate to Help -> Install New Software and provide the dtfj url and press Enter.

Click Next twice, Accept the terms of the license agreements and then click Finish. IBM diagnostic tool framework will start installing. This may take 5-10 minutes. Once the installation is completed, press “Yes” to restart eclipse.

Once eclipse is restarted, we can now see *.phd files under known formats. To check this, navigate to File -> Open Heap Dump. Select the phd file.

Now the phd file will be loaded and analyzed. This step may take 15-20 minutes depending on the heap dump size.

Some general errors we may face during the initial use and solutions for them are provided below.
The above errors occur when Memory analyser was invoked with java 1.6. They disappear when Java 1.7 is used.
Heap Space.
Sometimes while parsing heap dumps, it fails in-between with the error heap space.
In such scenarios, increase the Xmx value in MemoryAnalyzer.ini and try.
Share this:
- Manage Cookies
- Working Groups
- Marketplace
- Planet Eclipse
- Report a Bug
- Mailing Lists
- Documentation
- Getting Started / Support
- How to Contribute
- IDE and Tools
- Newcomer Forum
Participate
Eclipse IDE
Breadcrumbs
- Eclipse Wiki
MemoryAnalyzer
Notice: this wiki will be going read only early in 2024 and edits will no longer be possible. please see: https://gitlab.eclipse.org/eclipsefdn/helpdesk/-/wikis/wiki-shutdown-plan for the plan..
- View source
- 2.1 Installation
- 2.2 Basic Tutorials
- 2.3 Further Reading
- 3.1 HPROF dumps from Sun Virtual Machines
- 3.2 System Dumps and Heap Dumps from IBM Virtual Machines
- 3.3 What if the Heap Dump is NOT Written on OutOfMemoryError?
- 4 Extending Memory Analyzer
The Eclipse Memory Analyzer tool (MAT) is a fast and feature-rich heap dump analyzer that helps you find memory leaks and analyze high memory consumption issues.
With Memory Analyzer one can easily
- find the biggest objects, as MAT provides reasonable accumulated size (retained size)
- explore the object graph, both inbound and outbound references
- compute paths from the garbage collector roots to interesting objects
- find memory waste, like redundant String objects, empty collection objects, etc...
Getting Started
Installation.
See the download page for installation instructions.
Basic Tutorials
Both the Basic Tutorial chapter in the MAT documentation and the Eclipse Memory Analyzer Tutorial by Lars Vogel are a good first reading, if you are just starting with MAT.
Further Reading
Check MemoryAnalyzer/Learning Material . You will find there a collection of presentations and web articles on Memory Analyzer, which are also a good resource for learning. These pages Querying Heap Objects (OQL) OQL Syntax MemoryAnalyzer/OQL also explain some of the ways to use Object Query Language (OQL)
Getting a Heap Dump
Hprof dumps from sun virtual machines.
The Memory Analyzer can work with HPROF binary formatted heap dumps . Those heap dumps are written by Sun HotSpot and any VM derived from HotSpot. Depending on your scenario, your OS platform and your JDK version, you have different options to acquire a heap dump.
Non-interactive
If you run your application with the VM flag -XX:+HeapDumpOnOutOfMemoryError a heap dump is written on the first Out Of Memory Error. There is no overhead involved unless a OOM actually occurs. This flag is a must for production systems as it is often the only way to further analyze the problem.
As per this article , the heap dump will be generated in the "current directory" of the JVM by default. It can be explicitly redirected with -XX:HeapDumpPath= for example -XX:HeapDumpPath=/disk2/dumps . Note that the dump file can be huge, up to Gigabytes, so ensure that the target file system has enough space.
Interactive
As a developer, you want to trigger a heap dump on demand. On Windows, use JDK 6 and JConsole . On Linux and Mac OS X , you can also use jmap that comes with JDK 5.
- tutorial here
Via Java VM parameters:
- -XX:+HeapDumpOnOutOfMemoryError writes heap dump on OutOfMemoryError (recommended)
- -XX:+HeapDumpOnCtrlBreak writes heap dump together with thread dump on CTRL+BREAK
- -agentlib:hprof=heap=dump,format=b combines the above two settings (old way; not recommended as the VM frequently dies after CTRL+BREAK with strange errors)
- Sun (Linux, Solaris; not on Windows) JMap Java 5 : jmap -heap:format=b <pid>
- Sun (Linux, Solaris; Windows see link) JMap Java 6 : jmap.exe -dump:format=b,file=HeapDump.hprof <pid>
- Sun (Linus, Solaris) JMap with Core Dump File: jmap -dump:format=b,file=HeapDump.hprof /path/to/bin/java core_dump_file
- Sun JConsole: Launch jconsole.exe and invoke operation dumpHeap() on HotSpotDiagnostic MBean
- SAP JVMMon: Launch jvmmon.exe and call menu for dumping the heap
Heap dump will be written to the working directory.
System Dumps and Heap Dumps from IBM Virtual Machines
Memory Analyzer may read memory-related information from IBM system dumps and from Portable Heap Dump (PHD) files with the IBM DTFJ feature installed. Once installed, then File > Open Heap Dump should give the following options for the file types:
- All known formats
- HPROF binary heap dumps
- IBM 1.4.2 SDFF
- IBM Javadumps
- IBM SDK for Java (J9) system dumps
- IBM SDK for Java Portable Heap Dumps
For a comparison of dump types, see Debugging from dumps . System dumps are simply operating system core dumps; therefore, they are a superset of portable heap dumps. System dumps are far superior than PHDs, particularly for more accurate GC roots, thread-based analysis, and unlike PHDs, system dumps contain memory contents like HPROFs. Older versions of IBM Java (e.g. < 5.0SR12, < 6.0SR9) require running jextract on the operating system core dump which produced a zip file that contained the core dump, XML or SDFF file, and shared libraries. The IBM DTFJ feature still supports reading these jextracted zips; however, newer versions of IBM Java do not require jextract for use in MAT since DTFJ is able to directly read each supported operating system's core dump format. Simply ensure that the operating system core dump file ends with the .dmp suffix for visibility in the MAT Open Heap Dump selection. It is also common to zip core dumps because they are so large and compress very well. If a core dump is compressed with .zip , the IBM DTFJ feature in MAT is able to decompress the ZIP file and read the core from inside (just like a jextracted zip). The only significant downsides to system dumps over PHDs is that they are much larger, they usually take longer to produce, they may be useless if they are manually taken in the middle of an exclusive event that manipulates the underlying Java heap such as a garbage collection, and they sometimes require operating system configuration ( Linux , AIX ) to ensure non-truncation.
In recent versions of IBM Java (> 6.0.1), by default, when an OutOfMemoryError is thrown, IBM Java produces a system dump, PHD, javacore, and Snap file on the first occurrence for that process (although often the core dump is suppressed by the default 0 core ulimit on operating systems such as Linux). For the next three occurrences, it produces only a PHD, javacore, and Snap. If you only plan to use system dumps, and you've configured your operating system correctly as per the links above (particularly core and file ulimits), then you may disable PHD generation with -Xdump:heap:none. For versions of IBM Java older than 6.0.1, you may switch from PHDs to system dumps using -Xdump:system:events=systhrow,filter=java/lang/OutOfMemoryError,request=exclusive+prepwalk -Xdump:heap:none
In addition to an OutOfMemoryError, system dumps may be produced using operating system tools (e.g. gcore in gdb for Linux, gencore for AIX, Task Manager for Windows, SVCDUMP for z/OS, etc.), using the IBM Java APIs , using the various options of -Xdump , using Java Surgery , and more.
Versions of IBM Java older than IBM JDK 1.4.2 SR12, 5.0 SR8a and 6.0 SR2 are known to produce inaccurate GC root information.
What if the Heap Dump is NOT Written on OutOfMemoryError?
Heap dumps are not written on OutOfMemoryError for the following reasons:
- Application creates and throws OutOfMemoryError on its own
- Another resource like threads per process is exhausted
- C heap is exhausted
As for the C heap, the best way to see that you won't get a heap dump is if it happens in C code (eArray.cpp in the example below):
C heap problems may arise for different reasons, e.g. out of swap space situations, process limits exhaustion or just address space limitations, e.g. heavy fragmentation or just the depletion of it on machines with limited address space like 32 bit machines. The hs_err-file will help you with more information on this type of error. Java heap dumps wouldn't be of any help, anyways.
Also please note that a heap dump is written only on the first OutOfMemoryError. If the application chooses to catch it and continues to run, the next OutOfMemoryError will never cause a heap dump to be written!
Extending Memory Analyzer
Memory Analyzer is extensible, so new queries and dump formats can be added. Please see MemoryAnalyzer/Extending_Memory_Analyzer for details.
- Tools Project
- Memory Analyzer
This page was last modified 07:12, 28 December 2022 by Erik Brangs . Based on work by Andrew Johnson , Kevin Grigorenko and Krum Tsvetkov and others .
Back to the top
.PHD File Extension
- 1. PhotoDirector Project File
- 2. Portable Heap Dump File
PhotoDirector Project File
What is a phd file.
Photo project created by PhotoDirector, a program used for editing digital photos; supports imported photos from many different camera RAW formats, including .DNG , .CR2 , .SRF , and many others; contains the library of imported images and stores any user edits; used as the native project save format and is saved with other data files and folders that contain the digital photo data.
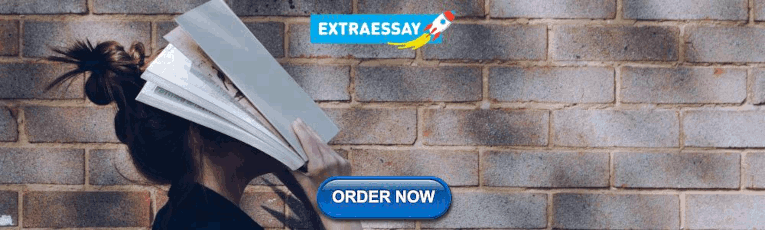
Programs that open PHD files
Portable heap dump file.
Data file created in the Portable Heap Dump format, which is used to create Java heap dump files using IBM's version of the Java Virtual Machine (JVM); may contains a record of all Java heap objects; used for debugging application errors such as memory leaks.
More Information
Portable heap dumps can be generated by setting the following environment variable parameters: IBM_HEAP_DUMP=true and IBM_HEAPDUMP=true . PHD files may be saved in a text or a binary format. However, the binary format is much smaller in file size. To specify the text format, set the IBM_JAVA_HEAPDUMP_TEXT=true environment variable.
NOTE: Portable heap dumps are typically generated by killing a running Java application. Therefore, to create a PHD file, start your Java program with the required environment variables set and then kill it.
Programs that open or reference PHD files
Verified by fileinfo.com.
The FileInfo.com team has independently researched all file formats and software programs listed on this page. Our goal is 100% accuracy and we only publish information about file types that we have verified.
If you would like to suggest any additions or updates to this page, please let us know .
PAGE CONTENTS
Memory Analyzer Configuration
Well, analyzing big heap dumps can also require more heap space. Give it some more memory (possible by running on a 64-bit machine):
Alternatively, edit the MemoryAnalyzer.ini to contain:
For more details, check out the section Running Eclipse in the Help Center. It also contains more details if you are running on Mac OS X.
If you are running the Memory Analyzer inside your Eclipse SDK, you need to edit the eclipse.ini file.
The memory intensive parts are the parsing of the dump and building of the dominator tree. Try parsing the heap dump from the command line, perhaps on a bigger machine. The dumps and index files can then be copied to a more convenient machine. Once the dump has been parsed, it usually can be opened with less memory in the GUI. As a rough estimate if the number of objects is N and the number of classes C, it might take at least T bytes to parse and build the dominator tree where: T ≈ N * 28.25 + C * 1000 + P P is the space used by the DTFJ or HPROF parsers. For a PHD file, the space could be: P ≈ C * 1000 Memory Analyzer uses additional memory for caching index files, so performance will be better if there is more memory available than the minimum required to parse a dump.
Memory Analyzer has an architectural limit of 2 31 - 3 objects, a current limit of 2 31 - 9 = 2,147,483,639 objects, but has not been tested with that many objects. The current record is a heap dump file of 159Gbytes containing 2,041,300,061 objects, which was opened with Memory Analyzer running with a 172Gbyte heap. Exceeding the limit can result in an exception such as java.lang.OutOfMemoryError: Requested length of new long[2,147,483,640] exceeds limit of 2,147,483,639 . See enable discard for options to work around this limit.
The preference page is opened via a menu option. Window > Preferences
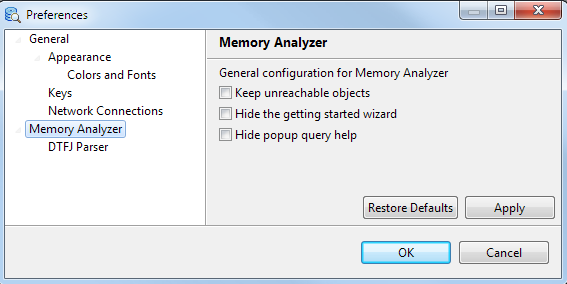
There is a option (from MAT 1.5 onwards) to display bytes in B, KB, MB, GB, or Smart formats. The default is to always display in Bytes format to match the previous MAT behavior and not cause any confusion. The option can be changed in the Eclipse preference dialog or using -Dbytes_display=(bytes|kilobytes|megabytes|gigabytes|smart) .
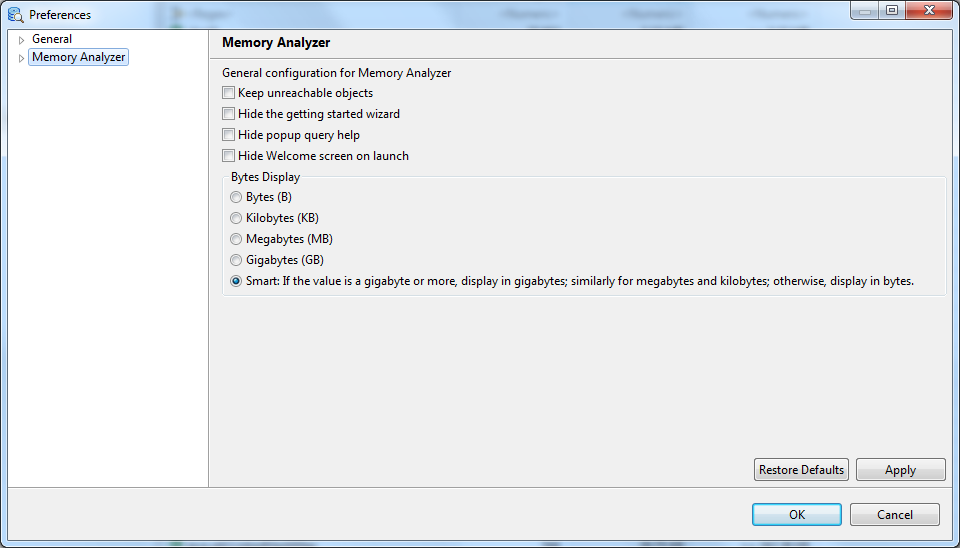
Sometimes a heap dump is generated with more objects than Memory Analyzer can handle, either from lack of heap to run Memory Analyzer itself, or because the number exceeds the Memory Analyzer limit of 2,147,483,639 objects. This option controls some experimental settings to help analyze such huge dumps, by purposely discarding objects in the original heap dump.
The discarded objects are counted in the unreachable objects histogram together with any unreachable objects discarded by Memory Analyzer after parsing but before building the dominator tree.

- If an OutOfMemoryError still occurs on a parse then more objects need to be discarded, either by increasing the discard percentage or by increasing the number of types of discarded objects by changing the pattern.
- If the leak is not apparent from the snapshot with many discarded objects then examine the unreachable objects histogram to see if any key objects have been discarded and modify the discard pattern. Ideally only primitive arrays or objects which just have primitive fields or refer to primitive arrays should be discarded. This avoids disrupting the object graph too much.
- If the types of the discarded objects look reasonable then try changing which objects have been discarded, either by varying the offset (if the discard percentage is not 100 ) or the discard seed.
- If the available heap to run Memory Analyzer is very small then try discarding all of the ordinary objects by choosing 100 for the discard percentage and .* to match all object types. The resulting snapshot will not be useful for finding leaks, but the unreachable object histogram will show the types of the objects taking most of the heap space, and might give a hint as to the problem.
This is useful when looking at paths to and from objects via local variables as the stack frames are visible in the paths to GC roots queries.
This can be useful to find out which methods are currently running and how much stack space they take up. To examine running methods then take the histogram view, filter by '\(', then sort by instances or instance size.
This can be useful to find out which methods have large JITted or byte code sizes. They can be viewed by going to the histogram view, then selecting <method type> and listing objects.
This is a change in behavior from previous releases when a warning was shown in the error log and processing continued. This default change was made to alert the user to a potential problem either with the file itself or a bug in the JVM or in MAT. You may choose to change the strictness of the parser:
- Report HTML/CSV/Text file output
- Export HTML/CSV/Text file output
- Copy > Save Value to File
- Customized Retained Set -xfile
- Compare Tables and Trees
- Find Leaks between Snapshots
No results were found for your search query.
To return expected results, you can:
- Reduce the number of search terms. Each term you use focuses the search further.
- Check your spelling. A single misspelled or incorrectly typed term can change your result.
- Try substituting synonyms for your original terms. For example, instead of searching for "java classes", try "java training"
- Did you search for an IBM acquired or sold product ? If so, follow the appropriate link below to find the content you need.
Search results are not available at this time. Please try again later or use one of the other support options on this page.
Eclipse Memory Analyzer Tool with DTFJ and IBM Extensions
The Eclipse Memory Analyzer Tool (MAT) is used for investigating Java memory issues such as OutOfMemoryErrors. This page provides downloads for the Eclipse Memory Analyzer Tool along with the IBM DTFJ extension and IBM Extensions for Memory Analyzer plugins pre-installed.
- Ensure IBM Semeru Runtimes Java 17 JDK is on your PATH
- Windows x86_64/amd64
- macOS x86_64/amd64
- macOS arm64/aarch64
- Linux x86_64/amd64
- Linux arm64/aarch64
- Linux POWER/ppc64le
- Eclipse archived update site
- Linux POWER/ppc64le 64-bit
- On macOS, open the Terminal application, change directory to where you downloaded the file and run: xattr -d com.apple.quarantine *.tar.gz
- Unzip or untar the downloaded file
- Windows: MemoryAnalyzer.exe
- macOS: Eclipse.app
- Linux: MemoryAnalyzer
Additional Information
- Update to MAT 1.15
- Upgrade to Eclipse 2023-09
- Update to IBM Java DTFJ 8.0.8.15 and Semeru DTFJ 17.0.9.0
- Add Eclipse archived update site downloads
- Update base MAT
- Upgrade DTFJ from IBM Java 8.0.8.6 and Semeru 17.0.7.0
- Enhance tWAS HTTP sessions query to show proper timezone-adjustment, oldest & newest session creation time, oldest & newest session access time, and session count by application name
- Enhance tWAS HTTP session holder name resolver for client server mode
- Add Liberty product version and installation directory to WAS Overview query
- 5 June 2023: Enhance WAS HTTP Session queries, and add Java 17 JVM arguments to handle J9 dumps.
- Update base MAT to 1.14 and Eclipse 2023-03
- MAT now requires launching with Java 17
- Update DTFJ to 8.0.8.0/11.0.18.0
- IBM Java: From com.ibm.java.diagnostics.memory.analyzer.MemoryAnalyzer-* to com.ibm.java.diagnostics.memory.analyzer.MemoryAnalyzer.ibmjava-*
- IBM Semeru Runtimes: From com.ibm.java.diagnostics.memory.analyzer.MemoryAnalyzer.openj9-* to com.ibm.java.diagnostics.memory.analyzer.MemoryAnalyzer.semeru-*
- New IEMA queries to understand the retained heap utilization of WAS XCI
- Update base MAT including leak suspects optimization
- Update DTFJ to 8.0.7.20/11.0.17.0
- Add Help } Diagnostics
- Add Thread Stack menu item
- 16 August 2022: Update DTFJ versions and the latest MAT
- 23 March 2022: Update DTFJ versions and Cognos extensions
- 10 December 2021: First version
Related Information
Eclipse Memory Analyzer Tool
WebSphere Performance Cookbook Memory Analyzer Tool Page
Document Location
Was this topic helpful.
Not useful Useful
Document Information
Modified date: 05 December 2023
ibm16537402
Page Feedback
Share your feedback
Need support.
- Submit feedback to IBM Support
1-800-IBM-7378 ( USA )
- Directory of worldwide contacts
JAVA & ANDROID HEAP
Dump analyzer.
- Auto Memory Leak Detection
- Tips to Reduce Memory 30-70%
- No Download/No Installation
Deep Learning
- Brilliant UI
- Report in seconds
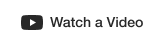
Upload Heap Dump File
Tip: For quick results compress (*.zip, *.gz) and upload heap dump file
1. POST HTTP request to API end point https://heaphero.io/analyze-hd-api?apiKey={Add Your API_KEY}
2. The body of the HTTP request should contain the Heap dump
3. HTTP response will be sent back in JSON format
Tip: For quick results compress (*.zip & *.gz) and paste http(s) or S3 presigned URL (More details)
I accept the terms of service
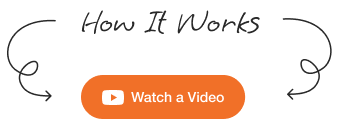
What is Remote Location?
Companies trusted and collaborated with us.
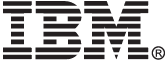
How Much Memory Your Application Wastes?
Due to inefficient programming, modern applications waste 30% to 70% of memory. HeapHero is the industry's first tool to detect the amount of wasted memory. It reports what lines of source code originating the memory wastage and solutions to fix them.
Android Memory Leak
Android mobile applications can also suffer from memory leaks, which can be attributed to poor programming practices. Memory leaks in mobile apps bare direct consumer impact and dissatisfaction. Memory leak slows down the application's responsiveness, makes it hang or crashes the application entirely. It will leave an unpleasant and negative user experience.
Java Memory Leak
A Memory leak is a type of resource drain that occurs when an application allocates memory and does not release after finish using it. This allocated memory can not be used for any other purpose and it remains wasted. As a consequence, Java applications will exhibit one or more of these non-desirable behaviors: poor response time, long JVM pauses, application hang, or even crash.
OutOfMemoryError
One common indication of a memory problem is the java.lang.OutOfMemoryError. This error is typically thrown when there is insufficient space to create a new object in the Java heap. There are 8 flavors of OutOfMemoryError. Each flavor of OutOfMemoryError has different causes and solutions.
Memory Regression
Sometimes the latest version of an application might be consuming more memory than the previous version. You need to analyze what are the large objects residing in the memory? Where is it being created? Where is it being held up? Answers to all these questions can be found in Heap Hero's Heap Analysis Report.

Memory Hogs
Wrong data structure choice, created but unused data structure, overallocated and underutilized data structure, suboptimal data type usage (i.e., using 'long' instead of 'int'), data duplication - all these can easily waste 30 - 70% of your memory. Heap Hero's intelligence report helps eliminate these memory hogs.
Universal Memory Analyzer
Hprof viewer and analyzer.
HPROF is a simple command-line tool that captures CPU/Heap profiles to identify performance bottlenecks in applications. By default, this tool writes the captured profiles to a file with '.hprof ' extension. HPROF file may contain CPU usage, heap allocation statistics, heap dump, thread stack traces and monitor states. It can be either in binary or text format. Heap Hero is a powerful tool to view and analyze HPROF files.
Universal Memory Dump Analysis
Heap Hero, a universal tool that will parse and analyze heaps dumps written in any language that runs on the JVM. It will convert Java, Scala, Jython, JRuby heap dumps to useful information to optimize your memory usage.
Reliably and quickly fix your memory problems through a precision single-page view of your heap dumps. View intuitive data display of heap histogram, largest objects, and memory leak suspects with a concisely brilliant interface.
Free Service
Our award-winning heap dump analysis tool is offered as a free service. Our tools help you to fix memory leaks, OutOfMemoryError, memory regression, memory hogs and any memory-related problems. All this power at your fingertip for free.
Android Memory Analysis
Android is the world's largest mobile platform. Heap Hero can parse and analyze the heap dumps generated from any Android devices. Heap Hero's deep learning algorithms can report memory leak suspects and objects wasting memory.
Online Heap Dump Analysis Tool
Heap Hero is the world's first and the only cloud-based heap dump analysis tool. Registration, download, or installation is not required to use the tool. Just upload your application's heap dumps & review the beautiful reports instantly.
Android Memory Optimizer
Heap Hero has built the industry's first and only REST API to analyze heap dumps. Stop manually uploading and analyzing heap dumps. Instead, analyze heap dumps from all your JVMs and Android devices in a programmatic manner through HEAP HERO's REST API .
Our award-winning deep learning algorithms have the intelligence to detect memory leaks and isolate the objects that are causing the memory leaks. Save time spent on diagnosing the memory leaks. It's all done automatically.
Collaboration
Heap Dump files consume a lot of disk space, making it very hard to share and collaborate with the team. HeapHero provides shareable URL links to heap dump analysis reports, making it a breeze to share and collaborate heap dump analysis with your fellow engineers.
Heap Dump Analysis Tool Beauty to the Beast
Analyzing heap dump doesn't have to be a tedious job. it can be fun, and it can be 'wow'.
- Online Tool
- Android Memory Analyzer
- Memory Leak Detection Tool
- Heap Analyzer
- HPROF Viewer & Analyzer
Optimize Memory
OutOfMemory Error
Detect Memory Leak
Our Services
We have optimized hundreds of open source and enterprise applications. Please take advantage of our battle-fought experience. We can either come on-site or provide remote consulting services.
Our easy to understand, fun-filled, on-site training programs are a preferred choice for several enterprises to transform thier engineers into performance experts.
Scaling in AWS
Are you looking to port your application to the AWS cloud? Are you following the AWS best practices? Are looking to lower your AWS bills? We are here to help you.
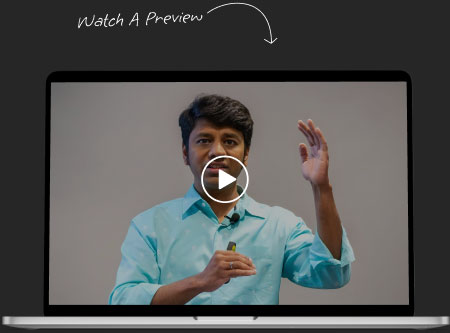
Learn JVM Performance and Troubleshooting
- Become a world class JVM performance expert
- Troubleshoot production performance problems in a fraction of time
Instructor: Ram Lakshmanan, Architect of GCeasy
What's included:
9 hours of video series with case studies and real life examples
3 months yCrash tool subscription
e-books and study material to complete this course
LinkedIn shareable certificate
1 year course subscription
Attended by engineers from all over the world from the premier brands

Check Out Our Other Products
Universal Garbage collection log analysis tool. Tune and troubleshoot memory and GC problems.
Automatically captures & analyzes GC Logs, thread dumps, heap dumps & several more artifacts to identify root cause.
Machine learning algorithms aided tool to analyze the thread dumps, core dumps, and also hs_err_pid dumps.
Top Analyzer
Parses Unix/Linux/solaris,..etc 'top' command output & generates an intuitive report to optimize the performance.
Simulates performance problems like Memory Leak, OutOfMemoryError, CPU spike, StackOverflowError etc.
Frequently Asked Questions
Java GC Tuning is made to appear as rocket science, but it's a common sense!
How to capture Java heap dump?
Heap hero isn't able to parse my heap dumps, how to generate an android heap dump, can the tool parse both java and android heap dumps, can i look at generated heap dump reports, can i install this tool locally.
If there are questions that you are looking for, please contact us at [email protected]
Want to try HeapHero?
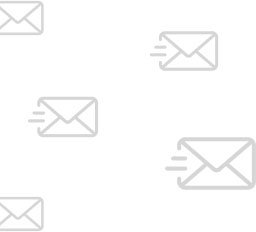
Subscribe to our newsletter
Congratulations.
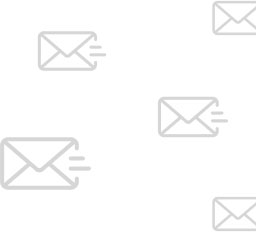

Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
PHD2 Guide Log Viewer
agalasso/phdlogview
Folders and files, repository files navigation, contributors 2.
- CMake 19.5%
- Inno Setup 1.0%

Jenna Merenstein, PhD, wins Postdoctoral Award for Professional Development!
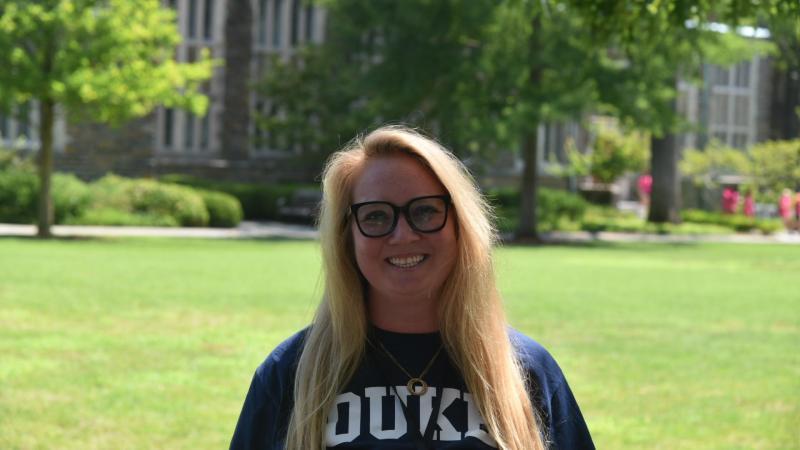
Congratulations to Jenna Merenstein, PhD, for winning a Postdoctoral Award for Professional Development from the Office of Postdoctoral Services! This award provides reimbursement for activities that support postdocs' professional growth and development. Jenna will be using her award to complete workshops and courses at the Society for Neuroscience International conference.
To read more about Jenna and this year's other award winners, visit Postdoctoral Awards for Professional Development | Duke Research & Innovation
Image from: https://scholars.duke.edu/person/jenna.merenstein  ;
- Documentation
- Getting Help
Tutorial: Analyzing PHD2 Guiding Results
A tutorial on how to interpret your Guide Log and improve your guiding performance, by Bruce Waddington. Highly recommended! Download PDF English Français Italiano 日本
- December 2023 (1)
- December 2019 (2)
- April 2018 (1)
- June 2016 (1)
- December 2015 (2)
- June 2015 (1)
- May 2015 (1)
- February 2015 (1)
- October 2014 (2)
News & Resources
December 22, 2023 - PHD2 v2.6.13 Released
December 21, 2019 - PHD2 Best Practices
December 7, 2019 - macOS Catalina
April 26, 2018 - Polar Alignment tool video tutorials
June 12, 2016 - PHD2_Broker package available
Chuck Todd: Politicians need the middle to win. It's getting harder for them to find it.
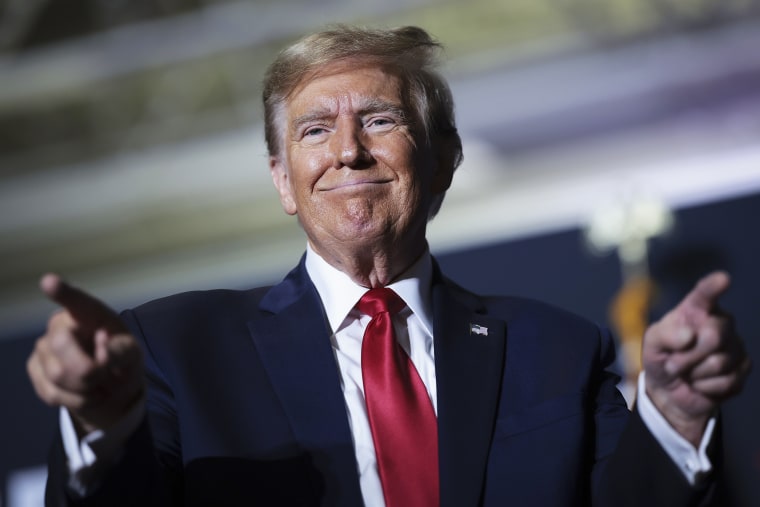
It’s not easy leading a political party these days. In a world where the expectation is that political positions are binary, with nuance not allowed, trying to come up with a one-size-fits-all position, be it on Middle East policy or reproductive rights, is quite the challenge.
No longer can someone paper over an actual point of view with the “spirit” of a political position. President Joe Biden has long been a supporter of Israel, but that hasn’t bought him much time with a Democratic base that’s growing increasingly skeptical of the Israeli government’s ability to carry out a just war.
And that brings me to former President Donald Trump’s attempts to soften opposition to him over the rise of restrictive abortion laws around the country.
Ironically, Trump’s controversial position shouldn’t, in theory, be controversial in the GOP. Trump is simply espousing what the party said it supported for decades before the Supreme Court’s 2022 Dobbs decision: Leave it to the states. But abortion conservatives want to go further with a federal limit. As is now fairly clear, simply returning the decision over reproductive rights to the states wasn’t really the goal of the anti-abortion movement pre-Dobbs. The goal was to roll back access to abortion, in whatever expedient way they could find.
Yet saying that last sentence as directly as I wrote it is unpopular. Had the GOP owned the idea of rolling back access more directly, instead of hiding behind its states-rights position, it would most likely been forced to reckon with its unpopular abortion position sooner.
But here we are, and Trump is learning the hard way that there is no middle ground on abortion inside the GOP, at least not in a post-Dobbs world. The country is quickly dividing into two camps on abortion rights: pro-access and anti-access. Pre-Dobbs, you could argue, there was a middle ground around access to abortion up until viability, which is about 24 weeks. But Dobbs changed the policy boundaries of what was possible, taking away elusive middle ground.
If states were debating access to abortion with 12- or 15- or 24-week limits as the different potential floors — deciding which of those three paths to take — then perhaps Trump’s “let the states decide” position would be seen as trying to find a middle ground. But that isn’t what has happened. A number of states, including large and diverse states like Texas and Florida, have passed unpopular and restrictive abortion laws — and it has led a lot of voters to suddenly feel like political activists. Before Dobbs, many Americans took for granted their equal right to reproductive health care no matter what states they lived in.
It’s clear what Trump is trying to do. He’s hoping that he can separate himself from the most restrictive positions on the issue. It’s striking that as nervous as Trump is that this issue could harm his chances at a second term, he didn’t go as far as supporting the ballot initiative in Florida that would essentially codify the Roe v. Wade standard in the state’s constitution. Perhaps he eventually will, if he thinks the gender gap is growing the wrong way for him.
There’s no doubt Trump was trying to replicate the rhetorical inoculation he effectively pulled off on the issue of entitlements back in 2016. He has come out against proposals to limit or slow the growth of these programs even as his party, essentially, still sees itself as the keeper of the “let’s shrink government” flame. With Trump in charge of the party, not touching entitlements is the GOP position — but it’s clear the position goes away the second Trump does, because so many elected Republicans decided to run for office under the guise of fiscal restraint.
Trump is clearly uncomfortable with any abortion restriction that is less than 15 or 16 weeks, but not enough to just say it that directly. He has hinted at it, saying he thought Florida’s six-week ban went too far. As a Florida resident, Trump will be able to choose on the ballot this fall between either an unpopular restrictive law or what the law was before his remodeled Supreme Court decided to relitigate the issue.
Trump can’t bring himself to even say something like “I personally support this restrictive law, but the country/state isn’t ready.” He could also word it like “I think Florida should change its law to 15 weeks, but I can’t vote for restoring Roe.”
I’m not sure which would be worse for Trump. But ultimately, I do think he will use the Florida ballot measure as his own barometer for all of us to see how nervous he is about the issue’s costing him another term.
In 2016, voters who didn’t trust the GOP on entitlements decided to trust Trump on the issue. There was enough cultural overlap for these older voters to give him a chance to keep the Social Security promise.
Are there enough women who care about abortion rights to trust Trump even if his party has acted another way? I’m skeptical. And the less Trump fights the six- or eight-week bans publicly, the less credible his compromise position will look. When push came to shove, Trump aligned himself with the party’s most restrictive abortion activists, and now he sees the political problem this has created. I’m not sure this is a political trap that even Houdini himself could escape. How does he distance himself from the decision to appoint the three Supreme Court justices that led to the overturning of Roe? He can do that only if he admits the justices weren’t his idea but something he outsourced. But if he admits that, then he’s admitting he somehow transacted judiciary seats for political support. It’s quite the slippery slope!
Both candidates would love to avoid talking about Gaza, and Trump would love nothing more than to stop discussing abortion, as well. The more either candidate talks about these issues, the more trouble they end up in. Ultimately, as many readers already know, I view the Dobbs decision as existential — and it’s most likely papering over lots of other divides in this country, because many affected voters view abortion rights as fundamental. And when an issue is fundamental for the way people live, they’ll vote on it over and above many other issues.
Why these abortion ballot measures will pass
As I was finishing up the column above, the Arizona Supreme Court was ruling that a 123-year-old abortion ban was now the law of the state. Meanwhile, the state is likely to have a referendum this fall to determine whether access to abortion should be guaranteed in the state’s constitution.
Knowing Arizona the way I do, I think this is as good as passed. The state might be culturally very conservative in some places, but it has a very strong libertarian streak in it — which translates to a “my bedroom, my business” attitude. As in Florida, the alternative to not passing a constitutional amendment in Arizona will be abiding by a very restrictive law. Given those two choices, it’s pretty obvious what voters will pick.
As I wrote last week , perhaps 15-week limits could become a “tolerable” restrictive floor for abortion. Perhaps. But there’s “tolerance” and there’s “preference,” and it’s pretty clear the public prefers to decide for itself whether to make this decision. It doesn’t want the government to decide for it.
If you’re wondering what these abortion propositions truly mean for the makeup of the general electorate, the biggest thing they do is motivate younger folks to show up. And if all these abortion propositions around the country do just that and juice youth turnout, they could be the difference between Biden carrying Arizona and Trump carrying it.

Chuck Todd is NBC News' chief political analyst and the former moderator of " Meet The Press ."
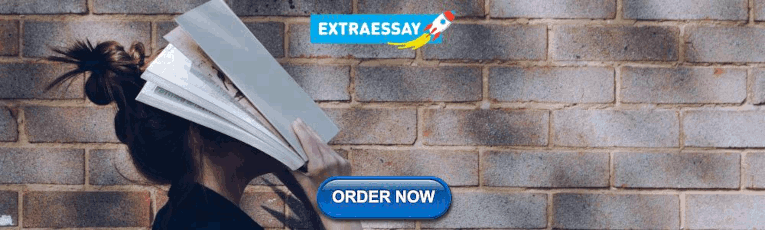
IMAGES
VIDEO
COMMENTS
I have Eclipse Memory Analyzer v1.3.1, and need to analyze some .phd heap dumps. According to this question, it is necessary to install DTJF on Eclipse Memory Analyzer.. This link in the question says: Memory Analyzer can also read memory-related information from IBM system dumps and from Portable Heap Dump (PHD) files. For this purpose one just has to install the IBM DTFJ feature into Memory ...
The best method to analyze a PHD heap dump is to use the Eclipse Memory Analyzer™ tool (MAT) or the IBM Memory Analyzer tool. These tools process the dump file and provide a visual representation of the objects in the Java Heap. ... The body of a PHD file is indicated by a byte that contains a tag with a value of 2, after which there are a ...
PHD2 is guiding software inspired by Stark Labs PHD Guiding. PHD2 is free of cost, open source, and community-developed and supported. Download v2.6.13 macOS Sonoma+ ... Download; Documentation; Getting Help; About; PHD2 Log Viewer. Andy Galasso has written this PHD2 Log File viewer for quickly visualizing your guiding performance and spotting ...
the Portable Heap Dump (PHD) format. PHD is the default format. The classic format is human-readable since it is in ASCII text, but the PHD format is binary and should be processed by appropriate tools for analysis. ... We will first start the Memory Analyzer Tool and open the heap dump file. In Eclipse MAT, two types of object sizes are ...
The best method to analyze a PHD heap dump is to use the Eclipse Memory Analyzer™ tool (MAT) or the IBM Memory Analyzer tool. These tools process the dump file and provide a visual representation of the objects in the Java Heap. ... The body of a PHD file is indicated by a byte that contains a tag with a value of 2, after which there are a ...
In the list below, an item should appear called IBM Monitoring and Diagnostic Tools. Tick the box next to it, click Next, and follow the wizard to accept the license agreements and install the toolkit. Restart Eclipse when prompted. Choose File -> Open Heap Dump and choose your .phd file. It should open in MAT and allow you to figure out who is ...
Eclipse Memory Analyzer - Standalone Installation In this tutorial let's see how to Download and start working on Eclipse Memory Analyzer - Standalone version. Open a java heap dump created out of sun/oracle jdk (*.hprof) and a heap dump created out of IBM jdk (*.phd) files. Step 1: Search for "eclipse memory analyzer" and download…
In the list below, an item should appear called IBM Monitoring and Diagnostic Tools. Tick the box next to it, click Next, and follow the wizard to accept the license agreements and install the toolkit. Restart Eclipse when prompted. Choose File -> Open Heap Dump and choose your .phd file. It should open in MAT and allow you to figure out who is ...
Portable Heap Dump (PHD) files generated with the Heap option can be compressed using the gzip compressor to reduce the file size. HPROF files can be compressed using the Gzip compressor to reduce the file size. ... but in chunks so that random access to the file is quicker. Memory Analyzer version 1.12 and later can read this format, ...
Gather all the .phd files and transfer them to your problem determination machine for analysis.; Many tools are available to analyze heap dumps that include Rational® Application Developer 6.0. WebSphere Application Server serviceability released a technology preview called Memory Dump Diagnostic For Java™.
PHD2 is telescope guiding software that simplifies the process of tracking a guide star, letting you concentrate on other aspects of deep-sky imaging or spectroscopy. Easy-to-use, "push here dummy" guiding for beginners. Sophisticated guiding and analysis tools for experienced users. Extensive support for commonly-used equipment.
Let PHD2 auto-select the guide star (Alt-s). It can be hard to visually distinguish a hot pixel from a faint guide star when you're just peering at the display. Be sure you're using either a dark library or a bad-pixel map. Apply a 2x2 or even 3x3 noise reduction filter (brain dialog/camera tab).
System Dumps and Heap Dumps from IBM Virtual Machines. Memory Analyzer may read memory-related information from IBM system dumps and from Portable Heap Dump (PHD) files with the IBM DTFJ feature installed. Once installed, then File > Open Heap Dump should give the following options for the file types: . All known formats
Portable heap dumps can be generated by setting the following environment variable parameters: IBM_HEAP_DUMP=true and IBM_HEAPDUMP=true. PHD files may be saved in a text or a binary format. However, the binary format is much smaller in file size. To specify the text format, set the IBM_JAVA_HEAPDUMP_TEXT=true environment variable.
For a PHD file, the space could be: P ≈ C * 1000 Memory Analyzer uses additional memory for caching index files, ... Memory Analyzer has an architectural limit of 2 31 - 3 objects, a current limit of 2 31 - 9 = 2,147,483,639 objects, but has not been tested with that many objects. The current record is a heap dump file of 159Gbytes containing ...
This page provides downloads for the Eclipse Memory Analyzer Tool along with the IBM DTFJ extension and IBM Extensions for Memory Analyzer plugins pre-installed. Steps. There are two builds of this tool. One build is to read dumps produced by IBM Java 8 and below. The other build is to read dumps produced by IBM Semeru Runtimes Java.
Lots of improvements to the Guiding Assistant. Updated camera support: Altair, QHY, SBIG, SSAG (Mac), ZWO ASI. New ToupTek camera support for Windows. New MallinCam SkyRaider camera support for Mac. INDI SBIG AO support. Better detection of problems like runaway guiding, excessive backlash, and calibration problems. Improved backlash compensation.
By default, this tool writes the captured profiles to a file with '.hprof ' extension. HPROF file may contain CPU usage, heap allocation statistics, heap dump, thread stack traces and monitor states. It can be either in binary or text format. Heap Hero is a powerful tool to view and analyze HPROF files.
Change Log. Open the Quick Help item on the Help menu to get brief description of how to navigate the log with the mouse. Guide log plot. Calibration plot. Settling frames after dither are automatically excluded from statistics calculation. You can also manually select ranges of frames with the mouse to exclude from the statistics.
View all files. Repository files navigation. README; GPL-3.0 license; PHD2 Log Viewer PHD2 Log Viewer is a tool for quickly visualizing your guiding performance and spotting problems in your PHD2 Guide Log. Open the Quick Help item on the Help menu to get brief description of how to navigate the log with the mouse. Andy Galasso <andy.galasso ...
PHD2 produces a log file whenever you have a guiding session. It generally has a name something like this PHD2_GuideLog_2021-09-19_214323.txt. This is the file that PHD2 log viewer will use to produce the analysis of your guiding session. So after your guiding session, run PHD2 log viewer and then go to the file menu, select the open option and ...
Joshua Kundert, PhD . Education Consultant LEAD Team . Alison Hiam. ... The more detailed analysis in Table 26 shows the decline in shortage licensure rates in cities is ... Detailed data files on one and three-year licenses with stipulations, including data by district,
Technical Supervisor: Andrea E. DeBarber, PhD Email: [email protected] Director/Clinical Consultant: P. Bart Duell, MD Phone: 503-494-3273 Sterol Analysis Laboratory Oregon Health & Science University Portland, OR 97239 Laboratory Phone: 503-494-4593 CAP # 2442607 CLIA # 38D06-56829
Jenna Merenstein, PhD, wins Postdoctoral Award for Professional Development! April 10, 2024. ... Brain Imaging and Analysis Center Box 3918 Durham, NC 27710 (919) 681-9337 [email protected]. Footer. Facebook Twitter Instagram LinkedIn. medschool.duke.edu | duke.edu | dukehealth.org. @2024 Duke University and Duke University Health System. ...
Tutorial: Analyzing PHD2 Guiding Results. A tutorial on how to interpret your Guide Log and improve your guiding performance, by Bruce Waddington. Highly recommended! Download PDF English Français Italiano 日本. December 22, 2023 -. December 21, 2019 -.
for more details on these disclaimers. Where thirdparty data are used to create a chart or table or to undertake an analysis, the third party is - identified and credited as the source. In each case, ESMA is cited by default as a source, reflecting any data management or cleaning, processing,
It's not easy leading a political party these days. Analysis: Donald Trump's effort to defuse his trouble on abortion illustrates how unpopular the GOP's longtime position on the issue has become.
Economic Analysis for the Final Per- and Polyfluoroalkyl Substances National Primary Drinking Water Regulation ... agencies' databases and files, such as inventory and violations for all regulations are correctly represented in SDWIS/Fed. Between 2006 and 2016, the EPA recorded the findings from these ...