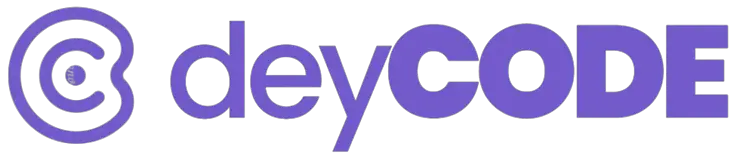
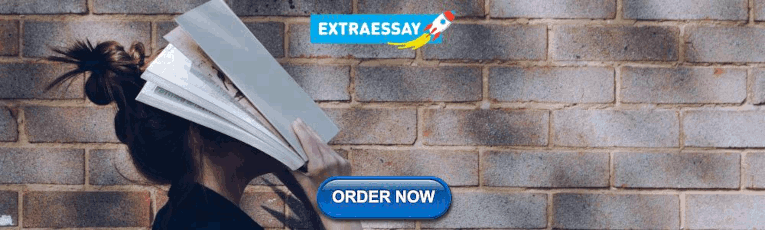
Dynamic constant assignment – Ruby
Quick Fix: To change the value of a constant in a method, you can use the #replace method for String or Array constants. This allows the object to take on a new value without actually changing the object itself.
The Solutions:
Solution 1:.
In Ruby, constants are meant to be immutable and remain unchanged throughout the program. However, when you assign a new value to a constant within a method, it’s considered a dynamic constant assignment, which is not allowed.
The problem is that each time you run the method, you create a new string object and assign it to the constant. This makes the constant non-constant because its value can change. Even though the contents of the string remain the same, the string object itself is different each time the method is called.
To resolve this issue, consider using an instance variable instead. Instance variables are associated with an instance of a class and can be changed within methods. Here’s an example:
class MyClass def initialize @my_constant = "blah" end
def my_method @my_constant = "new value" end end
In this case, `@my_constant` is an instance variable, and its value can be changed within the `my_method` method. However, the constant `MYCONSTANT` remains unchanged.
Alternatively, if you genuinely want to change the value of a constant within a method, you can use the `#replace` method for String or Array constants. The `#replace` method allows you to modify the contents of the object without changing the object itself.
class MyClass BAR = "blah"
def cheat(new_bar) BAR.replace new_bar end end
Using `#replace`, you can change the value of the constant `BAR` within the `cheat` method without creating a new string object.
Solution 2: Using const_set to Assign Constants Dynamically
Dynamically assigning constants inside methods is discouraged in Ruby, as constants are meant to be immutable. However, if you genuinely need to do this, you can use the `const_set` method.
Here’s an example:
The `const_set` method takes two arguments: the name of the constant as a symbol, and the value you want to assign to it. In this example, we’re assigning the string “foo” to the constant `MY_CONSTANT`. After calling `my_method`, you can access the constant using `MyClass::MY_CONSTANT`.
However, using `const_set` is not recommended for regular use. Consider using class variables, class attributes, or instance variables instead, depending on your specific needs.
Solution 3: Static constant assignment vs dynamic constant assignment
In Ruby, variables assigned with an uppercase are called constants. Another difference between variables and constants is that variables can be dynamically assigned, while constants can only be assigned once. This is because constants are statically defined, meaning their value remains consistent throughout the running program, while variables can change their values during runtime. Therefore, a dynamic constant assignment error occurs when attempting to reassign a constant that has already been assigned a value.
To resolve this issue, there are two common approaches:
- Declare the constant outside of the method :
In this case, "MY_CONSTANT" is declared outside the "my_method" method, making it a statically assigned constant that can be accessed within the method.
- Use a variable :
By declaring "my_local_variable" in the method, it becomes a regular variable that can be dynamically assigned and used within the method.
These approaches ensure that you avoid dynamic constant assignment errors and maintain the integrity of your program’s constants.
Solution 4: Dynamic assignment is not allowed
In Ruby, it is not allowed to define constants inside a method. Constants should be defined outside methods, at the top level or nested inside a module or class.
The error message, “dynamic constant assignment error,” means that you cannot assign a value to a constant in a dynamic way. In other words, you cannot change the value of a constant after it has been defined.
In your example, you are trying to assign the string “blah” to the constant MYCONSTANT inside the mymethod method. This is not allowed because MYCONSTANT is a constant and therefore its value cannot be changed.
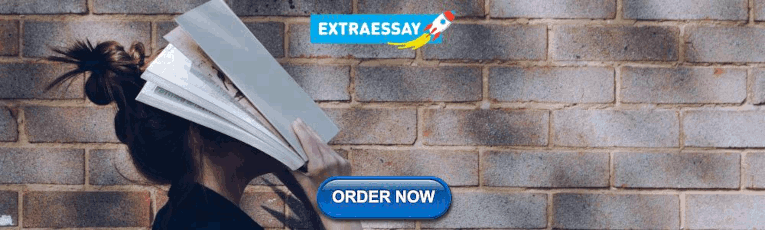
Solution 5: Dynamic Constant Assignment
In Ruby, variables are created by assigning them values using the `=` operator. If the variable name starts with a capital letter, it is considered a constant. Constants are meant to be immutable, meaning their values cannot be changed. Trying to assign a new value to a constant results in a “dynamic constant assignment error.”
The code you provided assigns a string value to the variable `MYCONSTANT` inside the `mymethod` method of the `MyClass` class. Since the variable name starts with capital letters, Ruby interprets it as a constant. Attempting to change the constant’s value later in the code would cause the error.
To fix this issue, you can use lowercase letters for the variable name, indicating that it is a regular variable. Here’s an example:
Now, `myconstant` is a regular variable, and you can assign different values to it without encountering the “dynamic constant assignment error.”
[Fixed] AWS Lambda Error (tesseract not installed) – Tesseract
Kotlin Native how to convert ByteArray to String? – Kotlin
© 2024 deycode.com

Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Dynamic constant assignment using non-ascii variable #2079
djberg96 commented Aug 26, 2020
eregon commented Aug 27, 2020
Sorry, something went wrong.
Successfully merging a pull request may close this issue.
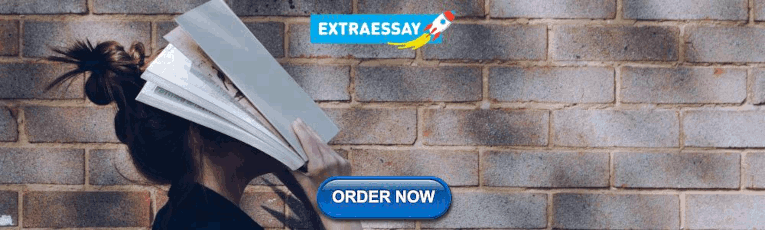
COMMENTS
Your problem is that each time you run the method you are assigning a new value to the constant. This is not allowed, as it makes the constant non-constant; even though the contents of the string are the same (for the moment, anyhow), the actual string object itself is different each time the method is called. For example:
Dynamic constant assignment Ruby [duplicate] Ask Question Asked 9 years, 4 ... dynamic constant assignment Typing_Test = Test.new The script itself is below... #Script name: Typing Challenge #Description: Demonstrating how to apply conditional logic in order to analyze user input and control #the execution of the script through a computer ...
sean_liu September 26, 2008, 7:03pm 6. I guess that the 'dynamic constant assignment' means that you can assing. to a. constant in a method. And the work around is. def self.set (value) const_set ("AB". value) end. Or, if you aren't in a class method.
These approaches ensure that you avoid dynamic constant assignment errors and maintain the integrity of your program's constants. Solution 4: Dynamic assignment is not allowed. In Ruby, it is not allowed to define constants inside a method. Constants should be defined outside methods, at the top level or nested inside a module or class. The ...
Props for the effort - that actually was a lot of code. I you are tired of the various puts, you can always do: alias e puts. Or something like that.
the first odd number index will be numbers.find_index(odd_numbers.first) for the constants you can assign a proc to the constant : Odd = Proc.new{ |n| n%2 == 1 } then call the constant like this: Odd.call(10) #=> false, similarly you can define a proc for even numbers. what happening here is every time the method get called the constant will be ...
I'm getting rather odd error messages in my AI I was hoping you could help me out #Amend n = set() k = [n] i = set() N = [set()] q = 0 h = [set()] m = [set()] t = 0 ...
Ruby on Rails is a popular web development framework that utilizes the Ruby programming language. One of the key features of Ruby on Rails is the use of structs, which are a way to define custom data structures. However, sometimes when using structs in Ruby on Rails, you may encounter a "dynamic constant assignment" syntax […]
I have a constant set up like that : ALL_LOCALES => {"it"=>"Italian", "fr"=>"Français", "de"=>"Deutsch", "en- GB"=>"English (UK)", "es"=>"Español (España)", "pt-PT ...
Fix wrong constant/identifier detection in lexer for non-ascii encodings ivoanjo/truffleruby. 2 participants. From one of our libraries we have this: def variance μ = mean reduce (0) { |m, i| m + (i - μ).square } / (length - 1) end In TruffleRuby, this results in: SyntaxError: dynamic constant assignment.
What is constant about a variable that changes everytime the locale changes? And if you have multiple users with different locales, potentially changes with every request? Given how changeable it is, why do you want to confuse the future maintainer by implying it is constant when it isn't.