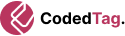
- Conditional Operators
Conditional Assignment Operator in PHP is a shorthand operator that allow developers to assign values to variables based on certain conditions.
In this article, we will explore how the various Conditional Assignment Operators in PHP simplify code and make it more readable.
Let’s begin with the ternary operator.
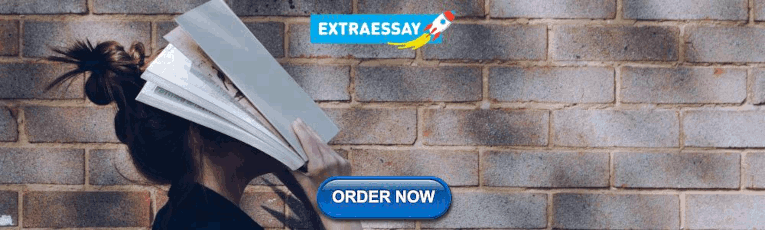
Ternary Operator Syntax
The Conditional Operator in PHP, also known as the Ternary Operator, assigns values to variables based on a certain condition. It takes three operands: the condition, the value to be assigned if the condition is true, and the value to be assigned if the condition is false.
Here’s an example:
In this example, the condition is $score >= 60 . If this condition is true, the value of $result is “Pass”. Otherwise, the value of $result is “Fail”.
To learn more, visit the PHP ternary operator tutorial . Let’s now explore the section below to delve into the Null Coalescing Operator in PHP.
The Null Coalescing Operator (??)
The Null Coalescing Operator, also known as the Null Coalescing Assignment Operator, assigns a default value to a variable if it is null. The operator has two operands: the variable and the default value it assigns if the variable is null. Here’s an example:
So, In this example, if the $_GET['name'] variable is null, the value of $name is “Guest”. Otherwise, the value of $name is the value of $_GET['name'] .
Here’s another pattern utilizing it with the assignment operator. Let’s proceed.
The Null Coalescing Assignment Operator (??=)
The Null Coalescing Operator with Assignment, also known as the Null Coalescing Assignment Operator, assigns a default value to a variable if it is null. The operator has two operands: the variable and the default value it assigns if the variable is null. Here’s an example:
So, the value of $name is null. The Null Coalescing Assignment Operator assigns the value “Guest” to $name. Therefore, the output of the echo statement is “Welcome,”Guest!”.
Moving into the following section, you’ll learn how to use the Elvis operator in PHP.
The Elvis Operator (?:)
In another hand, The Elvis Operator is a shorthand version of the Ternary Operator. Which assigns a default value to a variable if it is null. It takes two operands: the variable and the default value to be assigned if the variable is null. Here’s an example:
In this example, if the $_GET['name'] variable is null, the value“Guest” $name s “Guest”. Otherwise, the value of $name is the value of $_GET['name'] .
Let’s summarize it.
Wrapping Up
The Conditional Assignment Operators in PHP provide developers with powerful tools to simplify code and make it more readable. You can use these operators to assign values to variables based on certain conditions, assign default values to variables if they are null, and perform shorthand versions of conditional statements. By using these operators, developers can write more efficient and elegant code.
Did you find this article helpful?
Sorry about that. How can we improve it ?
- Facebook -->
- Twitter -->
- Linked In -->
- Install PHP
- Hello World
- PHP Constant
- PHP Comments
PHP Functions
- Parameters and Arguments
- Anonymous Functions
- Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
Control Structures
- If-else Block
- Break Statement
PHP Operators
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- PHP String Operators
- Array Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Null Coalescing Operator
Data Format and Types
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
String and Patterns
- Remove the Last Char
What are the conditional assignment operators in PHP?
Get Started With Data Science
Learn the fundamentals of Data Science with this free course. Future-proof your career by adding Data Science skills to your toolkit — or prepare to land a job in AI, Machine Learning, or Data Analysis.
Introduction
Operators in PHP are signs and symbols which are used to indicate that a particular operation should be performed on an operand in an expression. Operands are the operation objects, or targets. An expression contains operands and operators arranged in a logical manner.
In PHP, there are numerous operators, which are grouped into the following categories:
Logical operators
String operators
Array operators
Arithmetic operators
Assignment operators
Comparison operators
Increment/Decrement
Conditional assignment operators
Some examples of these operators are:
- - Subtraction
- * Multiplication
- -- and so much more.
What are conditional assignment operators?
Conditional assignment operators , as the name implies, assign values to operands based on the outcome of a certain condition. If the condition is true , the value is assigned. If the condition is false , the value is not assigned.
There are two types of conditional assignment operators: tenary operators and null coalescing operators .
1. Tenary operators ?:
Basic syntax.
Where expr1 , expr2 , and expr3 are either expressions or variables.
From the syntax, the value of $y will be evaluated. This value of $y is evaluated to expr2 if expr1 = TRUE . The value of $y is expr3 if expr1 = FALSE .
2. Null coalescing operators ??
The ?? operator was introduced in PHP 7 and is basically used to check for a NULL condition before assignment of values.
Where varexp1 and varexp2 are either expressions or variables.
The value of $z is varexp1 if and only if varexp1 exists, and is not NULL. If varexp1 does not exist or is NULL, the value of $z is varexp2 .
Let’s use some code snippets to paint a more vivid description of these operators.
Explanation
In the code above, the new value of $y and $z is set based on the evaluation result of expr1 and varexp1 , respectively.
RELATED TAGS
CONTRIBUTOR
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
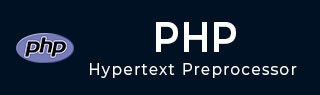
- PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP – Conditional Operators Examples
You would use conditional operators in PHP when there is a need to set a value depending on conditions. It is also known as ternary operator . It first evaluates an expression for a true or false value and then executes one of the two given statements depending upon the result of the evaluation.
Ternary operators offer a concise way to write conditional expressions. They consist of three parts: the condition, the value to be returned if the condition evaluates to true, and the value to be returned if the condition evaluates to false.
The syntax is as follows −
Ternary operators are especially useful for shortening if-else statements into a single line. You can use a ternary operator to assign different values to a variable based on a condition without needing multiple lines of code. It can improve the readability of the code.
However, you should use ternary operators judiciously, else you will end up making the code too complex for others to understand.
Try the following example to understand how the conditional operator works in PHP. Copy and paste the following PHP program in test.php file and keep it in your PHP Server's document root and browse it using any browser.
It will produce the following output −
- Language Reference
Table of Contents
- Operator Precedence
- Increment and Decrement
- Error Control
An operator is something that takes one or more values (or expressions, in programming jargon) and yields another value (so that the construction itself becomes an expression).
Operators can be grouped according to the number of values they take. Unary operators take only one value, for example ! (the logical not operator ) or ++ (the increment operator ). Binary operators take two values, such as the familiar arithmetical operators + (plus) and - (minus), and the majority of PHP operators fall into this category. Finally, there is a single ternary operator , ? : , which takes three values; this is usually referred to simply as "the ternary operator" (although it could perhaps more properly be called the conditional operator).
A full list of PHP operators follows in the section Operator Precedence . The section also explains operator precedence and associativity, which govern exactly how expressions containing several different operators are evaluated.
Improve This Page
User contributed notes 4 notes.

Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
- Course List
- Introduction to PHP
- Environment setup
- First PHP application
- Keywords & Identifiers
- Control structures
- Conditional: if, else, switch, match, ternary
- Iteration (loops): do, while, foreach, for
- Statements: break, continue
- Intermediate
- Multi-dimensional arrays
- Include & Require
- Form Handling
- Form Validation
- State management
- Sessions & Cookies
- File handling
- Object-Oriented Programming
- OOP: Classes & Objects
- OOP: $this calling object
- OOP: Constructors & Destructors
- OOP: Inheritance
- OOP: Polymorphism
- OOP: Composition
- OOP: Encapsulation - Access Modifiers
- Error Handling
- Error & Exception Handling
- MySQL/MariaDB
- Connect & Disconnect
- Create a database
- Create a database table
- Insert data
- Select data
- Update data
- Delete data
PHP Operators Tutorial
In this tutorial we learn more about symbols that act as operators in PHP and allow us to perform various operations throughout our application.
These operations include arithmetic, assignment, compound assignment, comparison, logical and more.
What are operators?
- Array operators
- Arithmetic operators
- Assignment operators
- Conditional assignment operators
- Comparison operators
- Incremental operators
- Logical operators
- String operators
Operators are symbols that have special meaning in PHP, and they indicate the types of operations that can be done.
As an example, we can consider the + symbol as an operator. It adds whatever number is on the right to the number on the left. This is an operation that is taking place on two operands.
PHP categorizes operators into different types:
Array operators are used to compare arrays. The array operators below may not make any sense at this point, if you are a beginner. We will cover these operators again in the tutorial lesson on arrays.
Arithmetic operators are used with numerical values to perform common mathematical arithmetic.
Assignment operators are used with numerical values to assign a value to a variable.
Conditional assignment operators will set values depending on the outcome of certain conditions. The conditional operators below may not make any sense at this point, if you are a beginner. We will cover them again in the tutorial lesson on conditional control flow.
The null coalescing operator is not available in versions below PHP v7 .
Comparison operators will compare two values against each other and return a true or false boolean value.
The following table lists comparison operators in PHP.
It is important for programmers coming from Javascript to remember that three equal signs === represents evaluating both equal value and equal type, and not just equal value .
Incremental operators are used to increase a value incrementally, and decremental operators are used to decrease a value. These operators are what is known as unary operators and as such only require one operand.
The following table lists incremental and decremental operators in PHP.
In the example above we can see that in both pre and post increments the value is incremented. The choice of which to use is determined by which value we want to work with:
- If we want to work with the already incremented value, we choose the pre-increment.
- If we want to work with the value before it is incremented and increment it afterwards, we choose the post-increment.
In most situations it won’t matter because we only want the value to actually increment, and not specifically use the value. We will look into increments and decrements more in the tutorial lesson on looping control flow.
Logical operators are used to combine conditional evaluations. We will work with them again in the tutorial lesson on conditional control flow.
The following table lists logical operators in PHP.
String operators are operators that combine (concatenate) strings together.
The following table lists string operators in PHP.
- Summary: Points to remember
- Operators are like keywords that have special meaning in PHP, and indicate operations that can be done.
- The null coalescing operator ? ? is not available in versions below PHP 7.
- Javascript programmers should note that === means evaluating both equal value and equal type, and not only equal value.
- These operators will be used more throughout the tutorial series, and will become much clearer for you.
- What are data types
PHP Advance
Operators - php basics.
Operators are symbols that instruct the PHP processor to carry out specific actions. For example, the addition ( + ) symbol instructs PHP to add two variables or values, whereas the greater-than ( > ) symbol instructs PHP to compare two values.
PHP operators are grouped as follows:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
Arithmetic Operators
The PHP arithmetic operators are used in conjunction with numeric values to perform common arithmetic operations such as addition, subtraction, multiplication, and so on.
Sample usage of Arithmetic Operators:
Assignment Operators
Assignment operators are used to assign values to variables.
Sample usage of Assignment Operators:
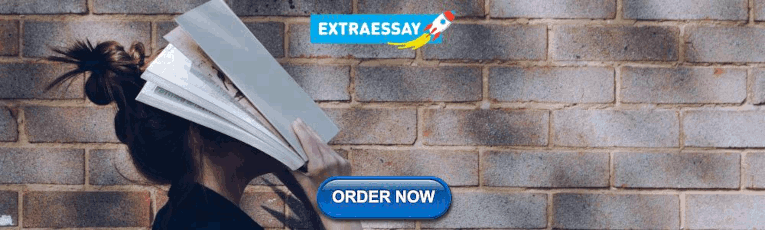
Comparison Operators
Comparison operators are used to compare two values in a Boolean fashion.
Sample usage of Comparison Operators:
Increment / Decrement Operators
Increment operators are used to increment a variable's value while decrement operators are used to decrement.
Sample usage of Increment / Decrement Operators:
Logical Operators
Logical operators are typically used to combine conditional statements.
Sample usage of Logical Operators:
String Operators
String operators are specifically designed for strings.
Sample usage of String Operators:
Array Operators
Array operators are used to compare arrays.
Conditional Assignment Operators
Conditional assignment operators are used to set a value depending on conditions.
Sample usage of Conditional Assignment Operators:
Create the following variables: $num1 , $num2 , $num3 . Assign the integer 3 to $num1 and 9 to $num2 . Multiply $num1 by $num2 and assign the product to $num3 . Print the result on $num3
- Introduction
- What is PHP?
- What can you do with PHP?
- Why use PHP?
- Requirements for this tutorial
- Echo and Print
- Conditionals
- Switch...Case
- Foreach Loop
- Do...While Loop
- Break/Continue
- Sorting Arrays
- Date and Time
- Include Files
- File Handling
- File Upload
- Form Validation
- Error Handling
- Classes and Objects
- Constructor and Destructor
- Access Modifiers
- Inheritance
- Abstract Classes
PHP Functions and Methods
- PHP Functions and Methods Index
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php if statements.
Conditional statements are used to perform different actions based on different conditions.
PHP Conditional Statements
Very often when you write code, you want to perform different actions for different conditions. You can use conditional statements in your code to do this.
In PHP we have the following conditional statements:
- if statement - executes some code if one condition is true
- if...else statement - executes some code if a condition is true and another code if that condition is false
- if...elseif...else statement - executes different codes for more than two conditions
- switch statement - selects one of many blocks of code to be executed
PHP - The if Statement
The if statement executes some code if one condition is true.
Output "Have a good day!" if 5 is larger than 3:
We can also use variables in the if statement:
Output "Have a good day!" if $t is less than 20:
PHP Exercises
Test yourself with exercises.
Output "Hello World" if $a is greater than $b .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Conditions in PHP
Hi! Here comes another PHP lesson. Today's topic is one of the most favorite among those who are starting to program. Still, because the conditions in PHP are what allows us to compose various algorithms. Depending on the conditions, the program will behave one way or another. And it is thanks to them that we can get different results with different input data. PHP has several constructs that you can use to implement conditions. All of them are used, and have their advantages in different situations, or, if you like, conditions. There are only conditions around, right? So. After all, no one will argue that in real life, depending on the circumstances, we act differently. In programming, this is no less important, and now we will learn this.
- PHP tutorial for beginners
- MySQL tutorial for beginners
- PHP tutorial for advanced
- PHP tutorial for professionals
As you should remember from the last lesson, in PHP, depending on the operator, the operands are cast to a certain type. Conditional operators in PHP follow the same rules, and here the operand is always cast to a boolean value. If this value is true , then we consider that the condition is met, and if it is false , then the condition is not met. Depending on whether the condition is met, we can do or not do any actions. And here I propose to consider the first conditional statement - if .
"if" statement
This is the simplest and most commonly used operator. In general, the construction looks like this:
And in real life, the use of the if statement looks like this:
Here we have explicitly passed the value true to the condition. Of course, this is completely pointless. Let's use a condition to define numbers greater than 10. It's quite simple:
And after running we will see the result:
Number greater than 10 Quizzes PHP Quiz for beginners PHP Quiz for advanced MySQL Quiz for beginners All quizzes
"if-else" construct
Is it possible to make it so that when the condition is not met, another code is executed? Yes, you certainly may! To do this, use the else statement along with the if statement. It is written after the curly braces that enclose the code that is executed when the condition is met. And the structure looks like this:
Here again, a message will be displayed on the screen:
However, if we change the input data, and at the very beginning we assign the value 8 to the variable $x , then a message will be displayed:
Number less than or equal to 10
Try it right now.
"if-elseif-else" construct: multiple conditions
In case you need to check several conditions, an elseif statement is added after the if statement. It will check the condition only if the first condition is not met. For example:
In this case, the screen will display:
Number equal to 10
And yes, you can add else after this statement. The code inside it will be executed if none of the conditions are met:
The result of this code, I believe, does not need to be explained. Yes, by the way, a whole list of elseifs is possible. For example, like this:
Cast to boolean
Remember, in the lesson about data types in PHP , we learned how to explicitly cast values to any type. For example:
The result will be true . Working in the same way, only the implicit conversion always happens in the condition. For example, the following condition:
It will succeed because the number 3 will be converted to true . The following values will be cast to false :
- '' (empty string)
- 0 (number 0)
- [] (empty array)
Thus, any non-zero number and non-zero string will be converted to true and the condition will be met. The exception is a string consisting of one zero:
It will also be converted to false .
I covered this topic with casting to boolean in the homework assignment for this tutorial. Be sure to complete it. Now let's move on to the next conditional statement.
"switch" statement
In addition to the if-else construct, there is one more conditional operator. This is switch . This is a very interesting operator that requires memorization of several rules. Let's first see what it looks like in the following example:
At first glance, this operator may seem rather complicated. However, if you understand, then everything becomes clear. An expression is specified in the switch operand. In our case, this is the $x variable, or rather its value is 1 .
In curly braces, we enumerate case statements, after which we indicate the value with which the value of the switch operand is compared. The comparison is not strict, that is, as if we were using the == operator. And if the condition is met, then the code specified after the colon is executed. If none of the conditions is met, then the code from the default section is executed, which, in general, may not exist, and then nothing will be executed. Please note that inside each case section, at the end, we have written a break statement. This is done so that after the code is executed, if the condition is met, the condition check does not continue. That is, if there was no break at the end of the case 1 section, then after the text
The number is 1
would be displayed, the comparison condition with 2 would continue to be fulfilled, and then the code in the default section would also be executed. Don't forget to write break !
switch vs if
In general, this code could also be written using the if-elseif-else construct:
But in the form of a switch-case construct, the code in this particular case looks simpler. And that's why:
- we immediately see what exactly we are comparing (the $x variable) and understand that we are comparing this value in each condition, and not any other;
- it is more convenient for the eye to perceive what we are comparing with - the case 1, case 2 sections are visually perceived easier, the compared value is more noticeable.
And again about switch
And I haven’t said everything about switch yet - you can write several case-s in a row, then the code will be executed provided that at least one of them is executed. For example:
Agree, it can be convenient.
Okay, let's go over the features of the switch statement that you should always keep in mind.
- break breaks a set of conditions, do not forget to specify it;
- default section will be executed if none of the conditions are met. It may be completely absent;
- several _case_s can be written in a row, then the code in the section will be executed if at least one of the conditions is met.
A little practice
Well, remember the conditional operators? Let's put it into practice with more real examples.
Even or Odd
Here is one example - you need to determine whether a number is even or not. To do this, we need to check that the remainder after dividing by 2 will be 0 . Read more about operators here . Let's do that:
Try changing the value of the $x variable yourself. Cool, yeah? It is working!
The absolute value of a number
Let's now learn how to calculate the modulus of a number. If the number is greater than or equal to zero, then you need to print this number itself, if it is less, change the sign from minus to plus.
Absolute value: 2
As we can see, everything worked out successfully.
Ternary operator
In addition, PHP has another operator, which is a shortened form of the if-else construct. This is a ternary operator. However, it returns different results depending on whether the condition is met or not. In general, its use is as follows:
Or using the same example of finding the absolute value of a number:
Cool, yeah? The ternary operator fits in very elegantly when solving simple problems like this.
And some more practice
Conditions can be placed inside each other and in general, what can you not do with them. For example:
What is the result
Friends, I hope you enjoyed the lesson. If so, I will be glad if you share it on social networks or tell your friends. This is the best support for the project. Thanks to those who do it. If you have any questions or comments - write about it in the comments. And now - we are all quickly doing our homework, there are even more interesting examples with conditions. Bye everyone!
Try the following conditions:
- if ('string') {echo 'Condition met';}
- if (0) {echo 'Condition met';}
- if (null) {echo 'Condition met';}
- if (5) {echo 'Condition met';}
Explain the result.
Use the ternary operator to determine if a number is even or odd and print the result.
- Hello world
- Reverse Words in a String
- Even numbers
- PHP Quiz for beginners
- PHP Quiz for advanced
- MySQL Quiz for beginners
PHP Assignment Operators
Php tutorial index.
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
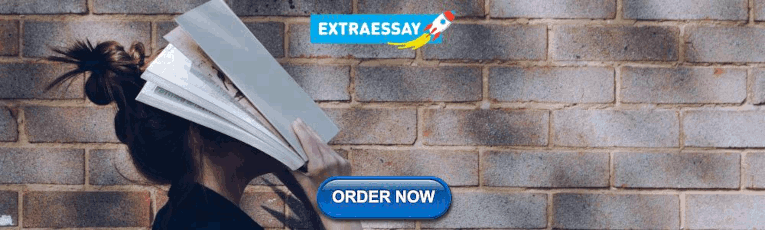
IMAGES
VIDEO
COMMENTS
Conditional Assignment Operator in PHP is a shorthand operator that allow developers to assign values to variables based on certain conditions. In this article, we will explore how the various Conditional Assignment Operators in PHP simplify code and make it more readable. Let's begin with the ternary operator. Ternary Operator Syntax
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword. Assignment by Reference.
PHP Assignment Operators. The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
In this, for x, firstly the condition (a>b) is evaluated. If this condition becomes true, then x will become the value 5 (ie, x=5). But if the condition (a>b) becomes false, then x will attain the value 9 (ie, x=9). Ternary Operator. Sometimes conditional operator ? : is also called a ternary operator.
To continue practicing conditional statements: Try using different operators: <, >, ==, ===. Combine operators with and or or. Recreate an if statement using a ternary, null coalescing, or spaceship operator. For more information on how to code in PHP, check out other tutorials in the How To Code in PHP series.
Conditional assignment operators, as the name implies, assign values to operands based on the outcome of a certain condition. If the condition is true, the value is assigned. If the condition is false, the value is not assigned. There are two types of conditional assignment operators: tenary operators and null coalescing operators.
PHP - Conditional Operators Examples - You would use conditional operators in PHP when there is a need to set a value depending on conditions. It is also known as ternary operator. It first evaluates an expression for a true or false value and then executes one of the two given statements depending upon the result of the evaluation.
if. ¶. The if construct is one of the most important features of many languages, PHP included. It allows for conditional execution of code fragments. PHP features an if structure that is similar to that of C: statement. As described in the section about expressions, expression is evaluated to its Boolean value.
Finally, there is a single ternary operator, ? :, which takes three values; this is usually referred to simply as "the ternary operator" (although it could perhaps more properly be called the conditional operator). A full list of PHP operators follows in the section Operator Precedence. The section also explains operator precedence and ...
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
php; variable-assignment; conditional-operator; Share. Improve this question. Follow edited Aug 19, 2009 at 18:15. chaos. 123k 33 33 ... PHP conditional assignment. 12. Operator and assignment at the same time in PHP? 7. php comparison operator in assignment. Hot Network Questions
Conditional assignment operators Conditional assignment operators will set values depending on the outcome of certain conditions. The conditional operators below may not make any sense at this point, if you are a beginner. We will cover them again in the tutorial lesson on conditional control flow. The following table lists conditional ...
Operators are symbols that instruct the PHP processor to carry out specific actions. For example, the addition (+) symbol instructs PHP to add two variables or values, whereas the greater-than (>) symbol instructs PHP to compare two values.PHP operators are grouped as follows: Arithmetic operators; Assignment operators; Comparison operators; Increment/Decrement operators
PHP Conditional Statements. Very often when you write code, you want to perform different actions for different conditions. You can use conditional statements in your code to do this. In PHP we have the following conditional statements: if statement - executes some code if one condition is true
As you should remember from the last lesson, in PHP, depending on the operator, the operands are cast to a certain type. Conditional operators in PHP follow the same rules, and here the operand is always cast to a boolean value. If this value is true, then we consider that the condition is met, and if it is false, then the condition is not met ...
But mixing logical and assignment operators in one statement is bad. This is called obfuscation and perl write-only style, makes reading this code harder. ... PHP Collective Join the discussion. ... php variable assignment inside if conditional. 9. Assign variable within condition if true. 0. Assigning a variable a value depending on its value. 0.
PHP Assignment Operators. PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right. Operator.
JS vs PHP: assignment operator precedence when used with logical-or. Related. 3. PHP Conditional Operator and Self Assignment. 4. When echoing boolean false, no text is printed. 6. Understanding operator precedence in php. 0. Php comparison precedence confusion. 1. Nested PHP ternary operator precedence. 2.
Description. Assignment operators allow writing a value to a variable. The first operand must be a variable and the basic assignment operator is "=". The value of an assignment expression is the final value assigned to the variable. In addition to the regular assignment operator "=", several other assignment operators are composites of an ...
Second, note that these operators (and or && ||) are short-circuit operators. That means if the answer can be determined with certainty from the first expression, the second one is never evaluated. Again this doesn't matter for your debugged line above, but it is extremely important when you are combining these operators with assignments, because