
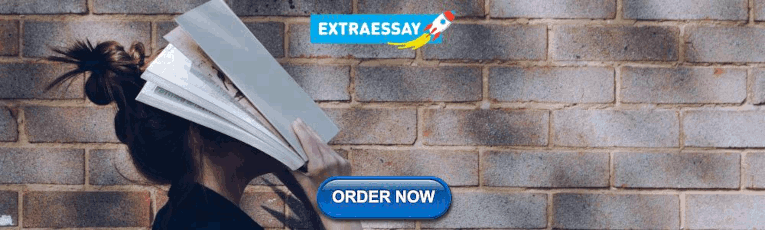
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
Using Compound Assignment Operator in a Java Program
CompoundAssignmentOperator.java

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
- Java-Operators
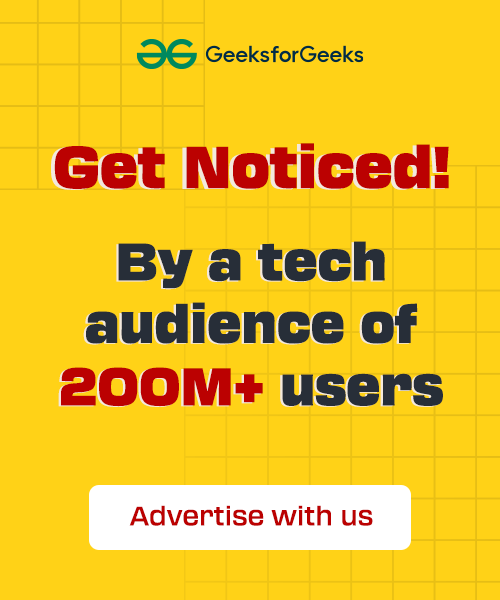
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Assignment, Arithmetic, and Unary Operators
The simple assignment operator.
One of the most common operators that you'll encounter is the simple assignment operator " = ". You saw this operator in the Bicycle class; it assigns the value on its right to the operand on its left:
This operator can also be used on objects to assign object references , as discussed in Creating Objects .
The Arithmetic Operators
The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics. The only symbol that might look new to you is " % ", which divides one operand by another and returns the remainder as its result.
The following program, ArithmeticDemo , tests the arithmetic operators.
This program prints the following:
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments . For example, x+=1; and x=x+1; both increment the value of x by 1.
The + operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo program:
By the end of this program, the variable thirdString contains "This is a concatenated string.", which gets printed to standard output.
The Unary Operators
The unary operators require only one operand; they perform various operations such as incrementing/decrementing a value by one, negating an expression, or inverting the value of a boolean.
The following program, UnaryDemo , tests the unary operators:
The increment/decrement operators can be applied before (prefix) or after (postfix) the operand. The code result++; and ++result; will both end in result being incremented by one. The only difference is that the prefix version ( ++result ) evaluates to the incremented value, whereas the postfix version ( result++ ) evaluates to the original value. If you are just performing a simple increment/decrement, it doesn't really matter which version you choose. But if you use this operator in part of a larger expression, the one that you choose may make a significant difference.
The following program, PrePostDemo , illustrates the prefix/postfix unary increment operator:
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Java Compound Assignment Operators
Java programming tutorial index.
Java provides some special Compound Assignment Operators , also known as Shorthand Assignment Operators . It's called shorthand because it provides a short way to assign an expression to a variable.
This operator can be used to connect Arithmetic operator with an Assignment operator.
For example, you write a statement:
In Java, you can also write the above statement like this:
There are various compound assignment operators used in Java:
While writing a program, Shorthand Operators saves some time by changing the large forms into shorts; Also, these operators are implemented efficiently by Java runtime system compared to their equivalent large forms.
Programs to Show How Assignment Operators Works
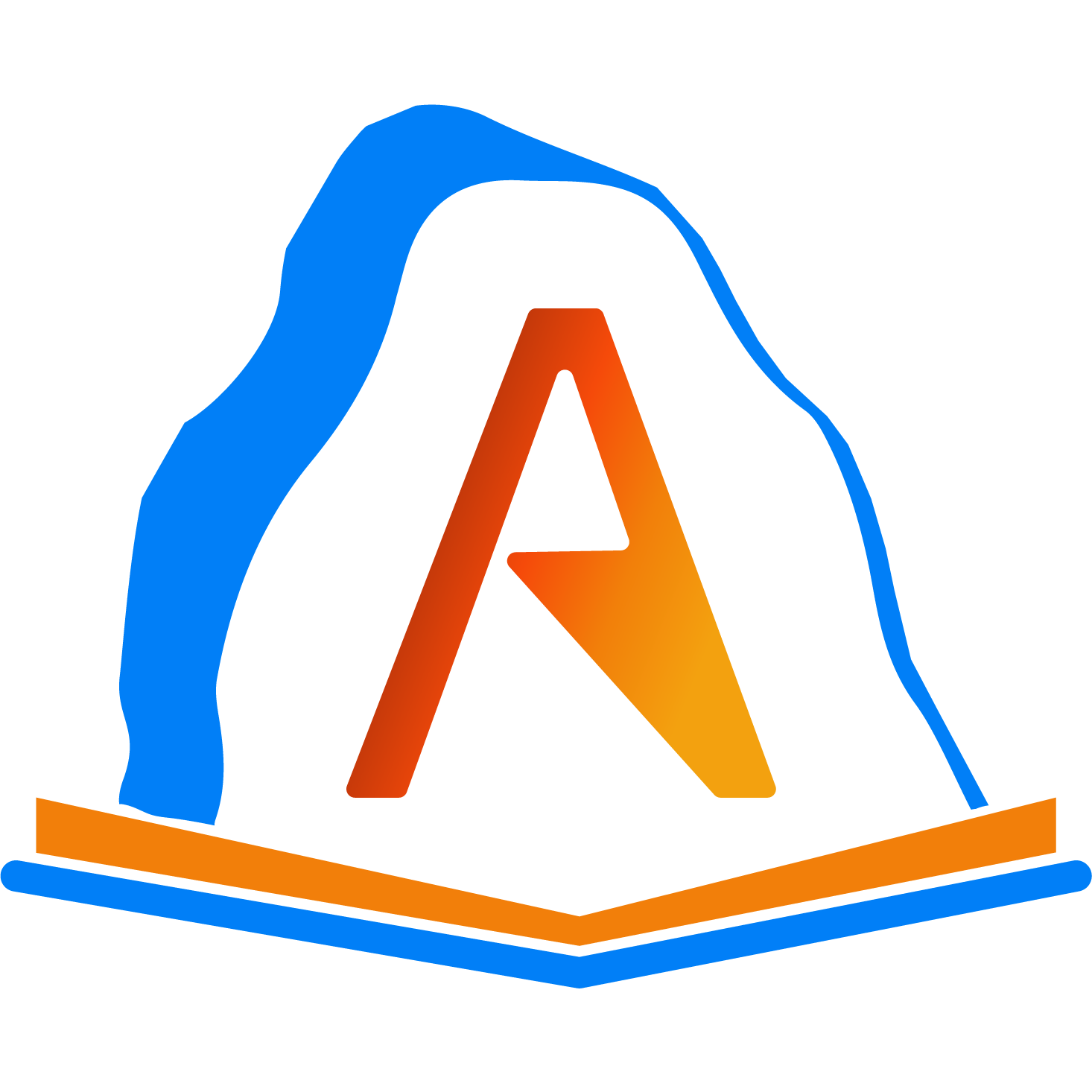
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Preface
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Variables
- 1.7 Java Development Environments (optional)
- 1.8 Unit 1 Summary
- 1.9 Unit 1 Mixed Up Code Practice
- 1.10 Unit 1 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.12 Lesson Workspace
- 1.4. Expressions and Assignment Statements" data-toggle="tooltip">
- 1.6. Casting and Ranges of Variables' data-toggle="tooltip" >
Before you keep reading...
Runestone Academy can only continue if we get support from individuals like you. As a student you are well aware of the high cost of textbooks. Our mission is to provide great books to you for free, but we ask that you consider a $10 donation, more if you can or less if $10 is a burden.
Making great stuff takes time and $$. If you appreciate the book you are reading now and want to keep quality materials free for other students please consider a donation to Runestone Academy. We ask that you consider a $10 donation, but if you can give more thats great, if $10 is too much for your budget we would be happy with whatever you can afford as a show of support.
1.5. Compound Assignment Operators ¶
Compound assignment operators are shortcuts that do a math operation and assignment in one step. For example, x += 1 adds 1 to x and assigns the sum to x. It is the same as x = x + 1 . This pattern is possible with any operator put in front of the = sign, as seen below.
The most common shortcut operator ++ , the plus-plus or increment operator, is used to add 1 to the current value; x++ is the same as x += 1 and the same as x = x + 1 . It is a shortcut that is used a lot in loops. If you’ve heard of the programming language C++, the ++ in C++ is an inside joke that C has been incremented or improved to create C++. The -- decrement operator is used to subtract 1 from the current value: y-- is the same as y = y - 1 . These are the only two double operators; this shortcut pattern does not exist with other operators. Run the following code to see these shortcut operators in action!

Run the code below to see what the ++ and shorcut operators do. Use the Codelens to trace through the code and observe how the variable values change. Try creating more compound assignment statements with shortcut operators and guess what they would print out before running the code.

1-5-2: What are the values of x, y, and z after the following code executes?
- x = -1, y = 1, z = 4
- This code subtracts one from x, adds one to y, and then sets z to to the value in z plus the current value of y.
- x = -1, y = 2, z = 3
- x = -1, y = 2, z = 2
- x = -1, y = 2, z = 4
1-5-3: What are the values of x, y, and z after the following code executes?
- x = 6, y = 2.5, z = 2
- This code sets x to z * 2 (4), y to y divided by 2 (5 / 2 = 2) and z = to z + 1 (2 + 1 = 3).
- x = 4, y = 2.5, z = 2
- x = 6, y = 2, z = 3
- x = 4, y = 2.5, z = 3
- x = 4, y = 2, z = 3
1.5.1. Code Tracing Challenge and Operators Maze ¶
Code Tracing is a technique used to simulate by hand a dry run through the code or pseudocode as if you are the computer executing the code. Tracing can be used for debugging or proving that your program runs correctly or for figuring out what the code actually does.
Trace tables can be used to track the values of variables as they change throughout a program. To trace through code, write down a variable in each column or row in a table and keep track of its value throughout the program. Some trace tables also keep track of the output and the line number you are currently tracing.
For example, given the following code:
The corresponding trace table looks like this:
Alternatively, we can show a compressed trace by listing the sequence of values assigned to each variable as the program executes. You might want to cross off the previous value when you assign a new value to a variable. The last value listed is the variable’s final value.
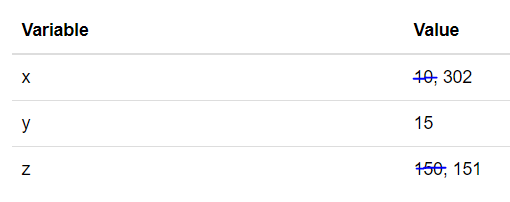
Use paper and pencil to trace through the following program to determine the values of the variables at the end. Be careful, % is the remainder operator, not division.
The final value for x is
The final value for y is
The final value for z is
1.5.2. Prefix versus Postfix Operator ¶
What do you think is printed when the following code is executed? Try to guess the output before running the code. You might be surprised at the result. Click on CodeLens to step through the execution. Notice that the second println prints the original value 7 even though the memory location for variable count is updated to the value 8.
The code System.out.println(count++) adds one to the variable after the value is printed. Try changing the code to ++count and run it again. This will result in one being added to the variable before its value is printed. When the ++ operator is placed before the variable, it is called prefix increment. When it is placed after, it is called postfix increment.
- System.out.println(score++);
- Print the value 5, then assign score the value 6.
- System.out.println(score--);
- Print the value 5, then assign score the value 4.
- System.out.println(++score);
- Assign score the value 6, then print the value 6.
- System.out.println(--score);
- Assign score the value 4, then print the value 4.
When you are new to programming, it is advisable to avoid mixing unary operators ++ and -- with assignment or print statements. Try to perform the increment or decrement operation on a separate line of code from assignment or printing.
For example, instead of writing x=y++; or System.out.println(z--); the code below makes it clear that the increment of y happens after the assignment to x , and that the value of z is printed before it is decremented.
- System.out.println(score); score++;
- System.out.println(score); score--;
- score++; System.out.println(score);
- score--; System.out.println(score);
1.5.3. Summary ¶
Compound assignment operators (+=, -=, *=, /=, %=) can be used in place of the assignment operator.
The increment operator (++) and decrement operator (–) are used to add 1 or subtract 1 from the stored value of a variable. The new value is assigned to the variable.

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
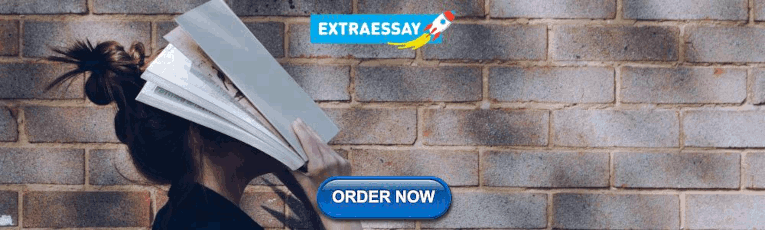
Compound assignment operators in Java
The Assignment Operators
Following are the assignment operators supported by Java language −
This will produce the following result −

I love programming (: That's all I know
Related Articles
- Compound Assignment Operators in C++
- Compound assignment operators in C#
- Assignment Operators in C++
- Perl Assignment Operators
- Assignment operators in Dart Programming
- What are Assignment Operators in JavaScript?
- What are assignment operators in C#?
- Compound operators in Arduino
- What is the difference between = and: = assignment operators?
- Initialization, declaration and assignment terms in Java
- Interesting facts about Array assignment in Java
- Division Operators in Java
- Java Boolean operators
- Java Operators Precedence
- Differences between & and && operators in Java.
Kickstart Your Career
Get certified by completing the course

- Java history and features
- Download and Install JDK
- Environment setup & first program
- Primitve Data types
- Class Access Modifiers
- Class Member Access Modifiers
- Abstract Class
- Final keyword in Java
- Static keyword in Java
- this keyword in Java
- Encapsulation
- Inheritance
- Aggregation and composition
- Polymorphism
- Overloading
- Constructors in Java
- Coupling and cohesion
- Stack and Heap in Java
- Array in Java
- 2-Dimensional Array in Java
- Enums in Java
- Return Types in Java
- Var-args in Java
- Garbage Collector
- Method finalize()
- Static Initialization Block
- Instance Initialization Block
- Static vs Instance Block
- decision-making Statements
- If Statement
- If-Else Statement
- Else-If Statement
- Nested If Statement
- Nested If-Else Statement
- Switch Statement
- Do-While Loop
- Break Statement
- Continue Statement
- Labelled Break Statement
- Labelled Continue Statement
- Arithmetic Operator
- Increment Operator
- Decrement Operator
- Compound Assignment Operators
- Relational Operators
- Logical Operators
- Conditional Operator
- instanceof operator
- Character Wrapper Class
- Boolean Wrapper Class
- Byte Wrapper Class
- Short Wrapper Class
- Integer Wrapper Class
- Long Wrapper Class
- Float Wrapper Class
- Double Wrapper Class
- Autoboxing and Unboxing
- Widening and Narrowing
- What is Exception?
- Checked Exception
- Unchecked Exception
- Exception Propagation
- Try-Catch block
- Multiple Catch Blocks
- Finally block
- Throw Keyword
- Throws Keyword
- User Defined Exception
Advertisement
+= operator
- Add operation.
- Assignment of the result of add operation.
- Understanding += operator with a code -
- Statement i+=2 is equal to i=i+2 , hence 2 will be added to the value of i, which gives us 4.
- Finally, the result of addition, 4 is assigned back to i, updating its original value from 2 to 4.
- A special case scenario for all the compound assigned operators
- All compound assignment operators perform implicit casting.
- Casting the char value ( smaller data type ) to an int value( larger data type ), so it could be added to an int value, 2.
- Finally, the result of performing the addition resulted in an int value, which was casted to a char value before it could be assigned to a char variable, ch .
Example with += operator
-= operator.
- Subtraction operation.
- Assignment of the result of subtract operation.
- Statement i-=2 is equal to i=i-2 , hence 2 will be subtracted from the value of i, which gives us 0.
- Finally, the result of subtraction i.e. 0 is assigned back to i, updating its value to 0.
Example with -= operator
*= operator.
- Multiplication operation.
- Assignment of the result of multiplication operation.
- Statement i*=2 is equal to i=i*2 , hence 2 will be multiplied with the value of i, which gives us 4.
- Finally, the result of multiplication, 4 is assigned back to i, updating its value to 4.
Example with *= operator
/= operator.
- Division operation.
- Assignment of the result of division operation.
- Statement i/=2 is equal to i=i/2 , hence 4 will be divided by the value of i, which gives us 2.
- Finally, the result of division i.e. 2 is assigned back to i, updating its value from 4 to 2.
Example with /= operator
%= operator.
- Modulus operation, which finds the remainder of a division operation.
- Assignment of the result of modulus operation.
- Statement i%=2 is equal to i=i%2 , hence 4 will be divided by the value of i and its remainder gives us 0.
- Finally, the result of this modulus operation i.e. 0 is assigned back to i, updating its value from 4 to 0.
Example with %= operator
Please share this article -.

Please Subscribe

Notifications
Please check our latest addition C#, PYTHON and DJANGO
© Copyright 2020 Decodejava.com. All Rights Reserved.
- Enterprise Java
- Web-based Java
- Data & Java
- Project Management
- Visual Basic
- Ruby / Rails
- Java Mobile
- Architecture & Design
- Open Source
- Web Services

Developer.com content and product recommendations are editorially independent. We may make money when you click on links to our partners. Learn More .

Java provides many types of operators to perform a variety of calculations and functions, such as logical , arithmetic , relational , and others. With so many operators to choose from, it helps to group them based on the type of functionality they provide. This programming tutorial will focus on Java’s numerous a ssignment operators.
Before we begin, however, you may want to bookmark our other tutorials on Java operators, which include:
- Arithmetic Operators
- Comparison Operators
- Conditional Operators
- Logical Operators
- Bitwise and Shift Operators
Assignment Operators in Java
As the name conveys, assignment operators are used to assign values to a variable using the following syntax:
The left side operand of the assignment operator must be a variable, whereas the right side operand of the assignment operator may be a literal value or another variable. Moreover, the value or variable on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. Assignment operators have a right to left associativity in that the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side variable must be declared before assignment.
You can learn more about variables in our programming tutorial: Working with Java Variables .
Types of Assignment Operators in Java
Java assignment operators are classified into two types: simple and compound .
The Simple assignment operator is the equals ( = ) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left.
Compound operators are comprised of both an arithmetic, bitwise, or shift operator in addition to the equals ( = ) sign.
Equals Operator (=) Java Example
First, let’s learn to use the one-and-only simple assignment operator – the Equals ( = ) operator – with the help of a Java program. It includes two assignments: a literal value to num1 and the num1 variable to num2 , after which both are printed to the console to show that the values have been assigned to the numbers:
The += Operator Java Example
A compound of the + and = operators, the += adds the current value of the variable on the left to the value on the right before assigning the result to the operand on the left. Here is some sample code to demonstrate how to use the += operator in Java:
The -= Operator Java Example
Made up of the – and = operators, the -= first subtracts the variable’s value on the right from the current value of the variable on the left before assigning the result to the operand on the left. We can see it at work below in the following code example showing how to decrement in Java using the -= operator:
The *= Operator Java Example
This Java operator is comprised of the * and = operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left. Here’s a program that shows the *= operator in action:
The /= Operator Java Example
A combination of the / and = operators, the /= Operator divides the current value of the variable on the left by the value on the right and then assigns the quotient to the operand on the left. Here is some example code showing how to use the /= operator in Java:
%= Operator Java Example
The %= operator includes both the % and = operators. As seen in the program below, it divides the current value of the variable on the left by the value on the right and then assigns the remainder to the operand on the left:
Compound Bitwise and Shift Operators in Java
The Bitwise and Shift Operators that we just recently covered can also be utilized in compound form as seen in the list below:
- &= – Compound bitwise Assignment operator.
- ^= – Compound bitwise ^ assignment operator.
- >>= – Compound right shift assignment operator.
- >>>= – Compound right shift filled 0 assignment operator.
- <<= – Compound left shift assignment operator.
The following program demonstrates the working of all the Compound Bitwise and Shift Operators :
Final Thoughts on Java Assignment Operators
This programming tutorial presented an overview of Java’s simple and compound assignment Operators. An essential building block to any programming language, developers would be unable to store any data in their programs without them. Though not quite as indispensable as the equals operator, compound operators are great time savers, allowing you to perform arithmetic and bitwise operations and assignment in a single line of code.
Read more Java programming tutorials and guides to software development .
Get the Free Newsletter!
Subscribe to Developer Insider for top news, trends & analysis
Latest Posts
What is the role of a project manager in software development, how to use optional in java, overview of the jad methodology, microsoft project tips and tricks, how to become a project manager in 2023, related stories, understanding types of thread synchronization errors in java, understanding memory consistency in java threads.
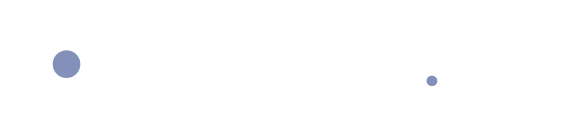
This tutorial introduces the *= operator and how to use it in Java.
The *= operator is a combined operator that consists of the * (multiply) and = (assignment) operators. This first multiplies and then assigns the result to the left operand.
This operator is also known as shorthand operator and makes code more concise. In this article, we will learn to use this operator with examples.
In this example, we used the multiply operator to get the product of a value and then assign it to use the assignment operator. This is a simple way to multiply in Java.
Now, let’s use the shorthand operator to get the remainder. See, the code has concise and produces the same result as the above code did.
Java supports several other compound assignment operators such as += , -= , *= , etc. In this example, we used other shorthand operators so that you can understand the use of these operators well.
See the example below.
Related Article - Java Operator
- Diamond Operator in Java
- Unsigned and Signed Right Bit Shift Operator in Java
- Java Bitwise Operators
- Remainder Operator in Java
- Logical Operators and Short Circuit in Java
- Operator Overloading in Java
Java Code Gists
A blog about Java. Examples for Java design patterns, Java core examples, web frameworks.
Compound Assignment Operators in Java
Compound assignment operators are the operators which are syntactically compact. It provides a syntax that is shorter than the normal ones and we use it for allocating the result to any variable for any bitwise or arithmetic calculation. Basically, before allocating the first operand’s result, the operation on the two operands is performed.
Types of compound assignment operators
These are all the possible types of compound assignment operators in language java:
This is the addition compound assignment operators assigning the value of addition.
For example, a=a+1 can be written as a+=1 .
This is the subtraction compound assignment operators assigning the value of subtraction.
For example, a=a-1 can be written as a-=1 .
This is the multiplication compound assignment operators assigning the value of multiplication.
For example, a=a*2 can be written as a*=2 .
This is the division compound assignment operators assigning the value of division.
For example, a=a/2 can be written as a/=2 .
This is the modulo compound assignment operators assigning the value of the remainder of the division.
For example, a=a%2 can be written as a%=2 .
This is the Bitwise & assignment compound operators assigning the value of logical AND operation.
For example, a=a & b can be written as a&=2 .
This is the Bitwise | assignment compound operators assigning the value of logical OR operation.
For example, a=a | b can be written as a|=2 .
This is the Bitwise ^ compound assignment operators assigning the value of logical XOR operation.
For example, a=a ^ b can be written as a^=2 .
This is the right-shift compound assignment operators assigning the value of the signed right bit shift operation.
For example, a=a >> b can be written as a>>=2 .
This is the right shift 0 filled compound assignment operators assigning the value of unsigned right bit shift operation.
For example, a=a >>> b can be written as a>>>=2 .
This is the left shift compound assignment operators assigning the value of the signed left bit shift operation.
For example, a=a << b can be written as a<<=2 .
There is an advantage of using the compound operators. This is that the complexity of the program decreases. They are faster in performing the operation of calculation and assigning. If there is a statement a=a*3, it will perform slower than a*=3.
Steps to remember- How these statements are written?
Step 1: We have to write the statement in the same format as it is given. The initial format is writing the operator between the operands.
Step 2: Now shift the arithmetic operator before the assignment operator.
Step 3: The operand equal to the “Left value” is removed. Left value is the value on the left-hand side of the assignment operator.
Step 4: By following the above steps, we would achieve the Arithmetic Compound Assignment Statement Expression.
Some rules for working out with assignment Compound operators
The expression containing the compound assignment operators are evaluated at runtime in any one of the two ways. These depend upon programming situations. The two ways of evaluation are:
- firstly, evaluation is done on the left value operand so that it produces a variable. In the case where the abrupt completion of the left-hand operand takes place, assignment expressions also terminate in an abrupt way. Then, no evaluation occurs for the operand on the right-side and therefore, there is no assignment operation.
- In an alternate case, firstly the left operand value is saved in a variable and then the evaluation of the operands on the right-side is done. If there is an abrupt termination of the evaluation, the assignment operation also terminates and therefore, there is no assignment operation.
- The binary operation indicated by the compound assignment operator is performed on the stored value of the left-sided expression and the values of the right-sided operand. If there is an abrupt termination of the operation, then assignment expressions also terminate abruptly and therefore, none of the assignment takes place.
- In other cases, the result obtained from the operation (binary) is changed to the data type of value of the left-sided variable. This conversion is put through the value sets which are defined by an appropriate standard. Lastly, the result obtained after conversion it is then transferred to the variable.
- Firstly, there is an evaluation of the references of an array that takes place on the sub-expressions to the left-sided value or the operand on the left-hand side. If there is an abrupt termination of the operation, then assignment expressions are also terminated abruptly. There is now no evaluation operation performed on the index of sub-expressions of left-sided value operand of array expressions & the operand on the right-side. This leads to a condition of no assignment.
- If the first step is not followed, in this case, evaluation is performed on the sub-expression of the index to the left value operand of array expressions. In the case where the abrupt completion of the left-hand operand takes place, the assignment expressions also terminate in an abrupt way. Then, no evaluation occurs for the operand on the right-side and therefore, there is no assignment operation.
- In another case, if the value of the sub-expression of array references or expression tends to null, then the assignment operation does not occur and therefore, then throws an exception known as NullPointerException .
- In another case where all above points are not valid, here the value assigned to the sub-expression of array references definitely points to the array. We look at this value and if this value fulfills the conditions such as the value that is less than zero, or which is greater or equals to the array’s length etcetera, then in this case, no assignment operation occurs. Also, an exception is thrown by the program known as ArrayIndexOutOfBoundsException .
- In the last case where all the above cases hold invalid, here one of the array’s component is selected which is referred by the sub-expression of array references. Selecting this component is based on the value of the index sub-expression. This value is saved in a variable. After this, the operands on the right-handed side are evaluated. If there is an abrupt termination of the operation, then expressions of assignments also terminate gets abruptly and therefore, none of the assignment does occur.
Example of resolving a statement containing assignment Comp ound operators
This is a fact that whenever a value that is bigger is assigned to a data type which is smaller in its construct and range, then to obtain a result with no errors, that is, error in compile time, we have to do an operation known as explicit typecasting. But, when we use compound arithmetic assignment operators in Java, the operation of internal type casting is performed automatically. We do not have to write an extra piece of code. Even when we assign some bigger data to a variable with small data types, we do not have to do type casting. Indeed, there are chances of loss in the information of data.
Let’s take an example for the correct code,
The code is same as:
Here the result is 7.6 but it automatically type-casted into short data type and the result obtained is 8.
This was a detailed description on the compound assignment operators in Java. Still, if you have any query, you can ask us. We would to answer all your questions.
Share this:
Further reading, leave a reply cancel reply.
This site uses Akismet to reduce spam. Learn how your comment data is processed .

- PyQt5 ebook
- Tkinter ebook
- SQLite Python
- wxPython ebook
- Windows API ebook
- Java Swing ebook
- Java games ebook
- MySQL Java ebook
Java operator
last modified January 27, 2024
In this article we show how to work with operators in Java.
An operator is a special symbol which indicates a certain process is carried out. Operators in programming languages are taken from mathematics. Programmers work with data. The operators are used to process data. An operand is one of the inputs (arguments) of an operator.
Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of the operators.
An operator usually has one or two operands. Those operators that work with only one operand are called unary operators . Those who work with two operands are called binary operators . There is also one ternary operator ?: which works with three operands.
Certain operators may be used in different contexts. For example the + operator. It can be used in different cases. It adds numbers, concatenates strings, or indicates the sign of a number. We say that the operator is overloaded .
Java sign operators
There are two sign operators: + and - . They are used to indicate or change the sign of a value.
The + and - signs indicate the sign of a value. The plus sign can be used to signal that we have a positive number. It can be omitted and it is mostly done so.
The minus sign changes the sign of a value.
Java assignment operator
The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one.
Here we assign a number to the x variable.
This expression does not make sense in mathematics, but it is legal in programming. The expression adds 1 to the x variable. The right side is equal to 2 and 2 is assigned to x.
This code line results in syntax error. We cannot assign a value to a literal.
Java concatenating strings
In Java the + operator is also used to concatenate strings.
We join three strings together.
Strings are joined with the + operator.
An alternative method for concatenating strings is the concat method.
Java increment and decrement operators
Incrementing or decrementing a value by one is a common task in programming. Java has two convenient operators for this: ++ and -- .
The above two pairs of expressions do the same.
In the above example, we demonstrate the usage of both operators.
We initiate the x variable to 6. Then we increment x two times. Now the variable equals to 8.
We use the decrement operator. Now the variable equals to 7.
And here is the output of the example.
Java arithmetic operators
The following is a table of arithmetic operators in Java.
The following example shows arithmetic operations.
In the preceding example, we use addition, subtraction, multiplication, division, and remainder operations. This is all familiar from the mathematics.
The % operator is called the remainder or the modulo operator. It finds the remainder of division of one number by another. For example, 9 % 4 , 9 modulo 4 is 1, because 4 goes into 9 twice with a remainder of 1.
Next we show the distinction between integer and floating point division.
In the preceding example, we divide two numbers.
In this code, we have done integer division. The returned value of the division operation is an integer. When we divide two integers the result is an integer.
If one of the values is a double or a float, we perform a floating point division. In our case, the second operand is a double so the result is a double.
We see the result of the program.
Java Boolean operators
In Java we have three logical operators. The boolean keyword is used to declare a Boolean value.
Boolean operators are also called logical.
Many expressions result in a boolean value. For instance, boolean values are used in conditional statements.
Relational operators always result in a boolean value. These two lines print false and true.
The body of the if statement is executed only if the condition inside the parentheses is met. The y > x returns true, so the message "y is greater than x" is printed to the terminal.
The true and false keywords represent boolean literals in Java.
The code example shows the logical and (&&) operator. It evaluates to true only if both operands are true.
Only one expression results in true .
The logical or ( || ) operator evaluates to true if either of the operands is true.
If one of the sides of the operator is true, the outcome of the operation is true.
Three of four expressions result in true .
The negation operator ! makes true false and false true.
The example shows the negation operator in action.
The || , and && operators are short circuit evaluated. Short circuit evaluation means that the second argument is only evaluated if the first argument does not suffice to determine the value of the expression: when the first argument of the logical and evaluates to false, the overall value must be false; and when the first argument of logical or evaluates to true, the overall value must be true. Short circuit evaluation is used mainly to improve performance.
An example may clarify this a bit more.
We have two methods in the example. They are used as operands in boolean expressions. We will see if they are called.
The One method returns false. The short circuit && does not evaluate the second method. It is not necessary. Once an operand is false, the result of the logical conclusion is always false. Only "Inside one" is only printed to the console.
In the second case, we use the || operator and use the Two method as the first operand. In this case, "Inside two" and "Pass" strings are printed to the terminal. It is again not necessary to evaluate the second operand, since once the first operand evaluates to true, the logical or is always true.
Java relational operators
Relational operators are used to compare values. These operators always result in a boolean value.
Relational operators are also called comparison operators.
In the code example, we have four expressions. These expressions compare integer values. The result of each of the expressions is either true or false. In Java we use the == to compare numbers. (Some languages like Ada, Visual Basic, or Pascal use = for comparing numbers.)
Java bitwise operators
Decimal numbers are natural to humans. Binary numbers are native to computers. Binary, octal, decimal, or hexadecimal symbols are only notations of a number. Bitwise operators work with bits of a binary number. Bitwise operators are seldom used in higher level languages like Java.
The bitwise negation operator changes each 1 to 0 and 0 to 1.
The operator reverts all bits of a number 7. One of the bits also determines whether the number is negative or not. If we negate all the bits one more time, we get number 7 again.
The bitwise and operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 only if both corresponding bits in the operands are 1.
The first number is a binary notation of 6, the second is 3 and the result is 2.
The bitwise or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if either of the corresponding bits in the operands is 1.
The result is 00110 or decimal 7.
The bitwise exclusive or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if one or the other (but not both) of the corresponding bits in the operands is 1.
The result is 00101 or decimal 5.
Java compound assignment operators
Compound assignment operators are shorthand operators which consist of two operators.
The += compound operator is one of these shorthand operators. The above two expressions are equal. Value 3 is added to the a variable.
Other compound operators are:
The following example uses two compound operators.
We use the += and *= compound operators.
The a variable is initiated to one. Value 1 is added to the variable using the non-shorthand notation.
Using a += compound operator, we add 5 to the a variable. The statement is equal to a = a + 5; .
Using the *= operator, the a is multiplied by 3. The statement is equal to a = a * 3; .
Java instanceof operator
The instanceof operator compares an object to a specified type.
In the example, we have two classes: one base and one derived from the base.
This line checks if the variable d points to the class that is an instance of the Base class. Since the Derived class inherits from the Base class, it is also an instance of the Base class too. The line prints true.
The b object is not an instance of the Derived class. This line prints false.
Every class has Object as a superclass. Therefore, the d object is also an instance of the Object class.
Java lambda operator
Java 8 introduced the lambda operator ( -> ).
This is the basic syntax for a lambda expression in Java. Lambda expression allow to create more concise code in Java.
The declaration of the type of the parameter is optional; the compiler can infer the type from the value of the parameter. For a single parameter the parentheses are optional; for multiple parameters, they are required.
The curly braces are optional if there is only one statement in an expression body. Finally, the return keyword is optional if the body has a single expression to return a value; curly braces are required to indicate that the expression returns a value.
In the example, we define an array of strings. The array is sorted using the Arrays.sort method and a lambda expression.
Lambda expressions are used primarily to define an inline implementation of a functional interface, i.e., an interface with a single method only. Interfaces are abstract types that are used to enforce a contract.
In the example, we create a greeting service with the help of a lambda expression.
Interface GreetingService is created. All objects implementing this interface must implement the greet method.
We create an object that implements GreetingService with a lambda expression. The object has a method that prints a message to the console.
We call the object's greet method, which prints a give message to the console.
There are some common functional interfaces, such as Function , Consumer , or Supplier .
The example uses a lambda expression to compute squares of integers.
Function is a function that accepts one argument and produces a result. The operation of the lamda expression produces a square of the given integer.
Java double colon operator
The double colon operator (::) is used to create a reference to a method.
In the code example, we create a reference to a static method with the double colon operator.
We have a static method that prints a greeting to the console.
Consumer is a functional interface that represents an operation that accepts a single input argument and returns no result. With the double colon operator, we create a reference to the greet method.
We perform the functional operation with the accept method.
Java operator precedence
The operator precedence tells us which operators are evaluated first. The precedence level is necessary to avoid ambiguity in expressions.
What is the outcome of the following expression, 28 or 40?
Like in mathematics, the multiplication operator has a higher precedence than addition operator. So the outcome is 28.
To change the order of evaluation, we can use parentheses. Expressions inside parentheses are always evaluated first. The result of the above expression is 40.
Java operators precedence list
The following table shows common Java operators ordered by precedence (highest precedence first):
Operators on the same row of the table have the same precedence. If we use operators with the same precedence, then the associativity rule is applied.
In this code example, we show a few expressions. The outcome of each expression is dependent on the precedence level.
This line prints 28. The multiplication operator has a higher precedence than addition. First, the product of 5*5 is calculated, then 3 is added.
In this case, the negation operator has a higher precedence than the bitwise OR. First, the initial true value is negated to false, then the | operator combines false and true, which gives true in the end.
Java associativity rule
Sometimes the precedence is not satisfactory to determine the outcome of an expression. There is another rule called associativity . The associativity of operators determines the order of evaluation of operators with the same precedence level.
What is the outcome of this expression, 9 or 1? The multiplication, deletion, and the modulo operator are left to right associated. So the expression is evaluated this way: (9 / 3) * 3 and the result is 9.
Arithmetic, boolean, relational, and bitwise operators are all left to right associated. The assignment operators, ternary operator, increment, decrement, unary plus and minus, negation, bitwise NOT, type cast, object creation operators are right to left associated.
In the example, we have two cases where the associativity rule determines the expression.
The assignment operator is right to left associated. If the associativity was left to right, the previous expression would not be possible.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and then the compound assignment operator is applied.
Java ternary operator
The ternary operator ?: is a conditional operator. It is a convenient operator for cases where we want to pick up one of two values, depending on the conditional expression.
If cond-exp is true, exp1 is evaluated and the result is returned. If the cond-exp is false, exp2 is evaluated and its result is returned.
In most countries the adulthood is based on the age. You are adult if you are older than a certain age. This is a situation for a ternary operator.
First the expression on the right side of the assignment operator is evaluated. The first phase of the ternary operator is the condition expression evaluation. So if the age is greater or equal to 18, the value following the ? character is returned. If not, the value following the : character is returned. The returned value is then assigned to the adult variable.
A 31 years old person is adult.
Calculating prime numbers
In the following example, we are going to calculate prime numbers.
In the above example, we deal with several operators. A prime number (or a prime) is a natural number that has exactly two distinct natural number divisors: 1 and itself. We pick up a number and divide it by numbers from 1 to the selected number. Actually, we do not have to try all smaller numbers; we can divide by numbers up to the square root of the chosen number. The formula will work. We use the remainder division operator.
We will calculate primes from these numbers.
Values 0 and 1 are not considered to be primes.
We skip the calculations for 2 and 3. They are primes. Note the usage of the equality and conditional or operators. The == has a higher precedence than the || operator. So we do not need to use parentheses.
We are OK if we only try numbers smaller than the square root of a number in question.
This is a while loop. The i is the calculated square root of the number. We use the decrement operator to decrease i by one each loop cycle. When i is smaller than 1, we terminate the loop. For example, we have number 9. The square root of 9 is 3. We will divide the 9 number by 3 and 2. This is sufficient for our calculation.
If the remainder division operator returns 0 for any of the i values, then the number in question is not a prime.
In this article we covered Java expressions. We mentioned various types of operators and described precedence and associativity rules in expressions.
Java operators - tutorial
My name is Jan Bodnar and I am a passionate programmer with many years of programming experience. I have been writing programming articles since 2007. So far, I have written over 1400 articles and 8 e-books. I have over eight years of experience in teaching programming.
List all Java tutorials .
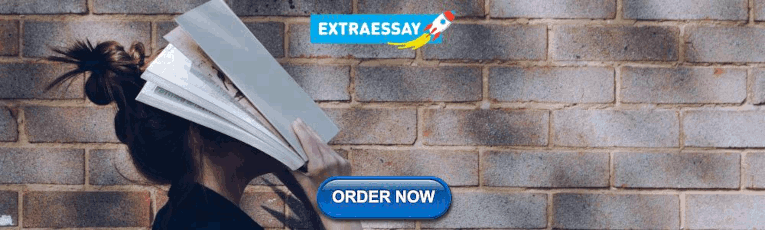
IMAGES
VIDEO
COMMENTS
The following are all possible assignment operator in java: 1. += (compound addition assignment operator) 2. ... Explanation: In the above example, we are using compound assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) apart from that we get the result as 20 because In compound assignment operator type ...
Compound Assignment Operators. An assignment operator is a binary operator that assigns the result of the right-hand side to the variable on the left-hand side. The simplest is the "=" assignment operator: int x = 5; This statement declares a new variable x, assigns x the value of 5 and returns 5. Compound Assignment Operators are a shorter ...
The compound assignment operator is the combination of more than one operator. It includes an assignment operator and arithmetic operator or bitwise operator. The specified operation is performed between the right operand and the left operand and the resultant assigned to the left operand. Generally, these operators are used to assign results ...
Compound assignment operators in Java are shorthand notations that combine an arithmetic or bitwise operation with an assignment. They allow you to perform an operation on a variable's value and then assign the result back to the same variable in a single step.
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments. For example, x+=1; and x=x+1; both increment the value of x by 1. The + operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo program:
This operator can be used to connect Arithmetic operator with an Assignment operator. For example, you write a statement: a = a+6; In Java, you can also write the above statement like this: a += 6; There are various compound assignment operators used in Java: Operator Meaning += Increments then assigns -= Decrements then assigns *= Multiplies ...
1.5. Compound Assignment Operators ¶. Compound assignment operators are shortcuts that do a math operation and assignment in one step. For example, x += 1 adds 1 to x and assigns the sum to x. It is the same as x = x + 1. This pattern is possible with any operator put in front of the = sign, as seen below. + shortcuts. - shortcuts.
Compound assignment operators in Java - The Assignment OperatorsFollowing are the assignment operators supported by Java language −OperatorDescriptionExample=Simple assignment operator. Assigns values from right side operands to left side operand.C = A + B will assign value of A + B into C+=Add AND assignment operator. It adds right ope
In all the compound assignment operators, the expression on the right side of = is always calculated first and then the compound assignment operator will start its functioning. Hence in the last code, statement i+=2*2; is equal to i=i+ (2*2), which results in i=i+4, and finally it returns 6 to i. All compound assignment operators perform ...
Java assignment operators are classified into two types: simple and compound. The Simple assignment operator is the equals ( =) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left. Compound operators are comprised of both an arithmetic, bitwise, or shift operator ...
Compound Assignment Operators Compound assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound operators, also called combined assignment operators, are a shorthand way to update the value of a variableThey are+= (addition)-= (subtraction)*= (...
Following is an example of using the concatenation operator: String nickname = "tough guy"; String message = "Hey there, " + nickname + "!"; The nickname string is effectively placed in the middle of a sentence. This code acts somewhat like a form letter in that you provide a sentence and then plug a name into it. Assignment operators.
What are Compound Assignment Operators? Compound assignment operators in Java combine two operations: an arithmetic operation (like addition, subtraction, multiplication, or division) and an assignment. These operators provide a shorthand way of updating the value of a variable.
The *= operator is a combined operator that consists of the * (multiply) and = (assignment) operators. This first multiplies and then assigns the result to the left operand. This operator is also known as shorthand operator and makes code more concise. In this article, we will learn to use this operator with examples.
As always with these questions, the JLS holds the answer. In this case §15.26.2 Compound Assignment Operators. An extract: A compound assignment expression of the form E1 op= E2 is equivalent to E1 = (T)((E1) op (E2)), where T is the type of E1, except that E1 is evaluated only once. An example cited from §15.26.2.
Step 1: We have to write the statement in the same format as it is given. The initial format is writing the operator between the operands. Step 2: Now shift the arithmetic operator before the assignment operator. Step 3: The operand equal to the "Left value" is removed.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and then the compound assignment operator is applied. $ java Associativity.java 0 0 0 0 0 Java ternary operator
The |= is a compound assignment operator ( JLS 15.26.2) for the boolean logical operator | ( JLS 15.22.2 ); not to be confused with the conditional-or || ( JLS 15.24 ). There are also &= and ^= corresponding to the compound assignment version of the boolean logical & and ^ respectively. In other words, for boolean b1, b2, these two are equivalent:
6. Take a look at the behaviour in JLS - Compound Assignment Operator. I'll quote the relevant two paragraphs here, just for the sake of completeness of the answer: Otherwise, the value of the left-hand operand is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes ...