JavaScript Object Destructuring, Spread Syntax, and the Rest Parameter – A Practical Guide

In JavaScript, we use objects to store multiple values as a complex data structure. There are hardly any JavaScript applications that do not deal with objects.
Web developers commonly extract values from an object property to use further in programming logic. With ES6, JavaScript introduced object destructuring to make it easy to create variables from an object's properties.
In this article, we will learn about object destructuring by going through many practical examples. We will also learn how to use the spread syntax and the rest parameter . I hope you enjoy it.
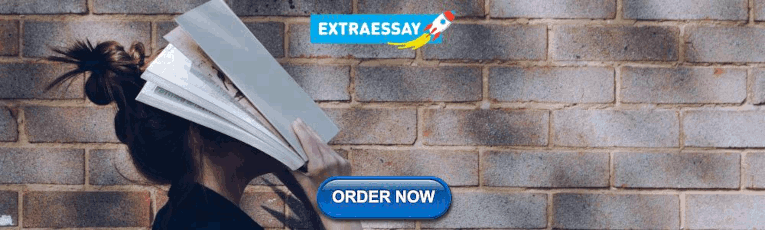
Object Destructuring in JavaScript
We create objects with curly braces {…} and a list of properties. A property is a key-value pair where the key must be a string or a symbol, and the value can be of any type, including another object.
Here we have created a user object with three properties: name, address, and age. The real need in programming is to extract these property values and assign them to a variable.
For example, if we want to get the value of the name and age properties from the user object, we can do this:
This is undoubtedly a bit more typing. We have to explicitly mention the name and age property with the user object in dot(.) notation, then declare variables accordingly and assign them.
We can simplify this process using the new object destructuring syntax introduced in ES6.
JavaScript Object Destructuring is the syntax for extracting values from an object property and assigning them to a variable. The destructuring is also possible for JavaScript Arrays.
By default, the object key name becomes the variable that holds the respective value. So no extra code is required to create another variable for value assignment. Let's see how this works with examples.
Basic Object Destructuring Example
Let's take the same user object that we referred to above.
The expression to extract the name property value using object destructuring is the following:
As you see, on the left side of the expression, we pick the object property key ( name in this case) and place it inside the {} . It also becomes the variable name to hold the property value.
The right side of the expression is the actual object that extracts the value. We also mention the keywords, const , let and so on to specify the variable's scope.

So, how do we extract values from more than one object property? Simple – we keep adding the object keys inside the {} with commas separating them. In the example below, we destructure both the name and age properties from the user object.
Variable Declaration Rule
The keywords let and const are significant in object destructuring syntax. Consider the example below where we have omitted the let or const keyword. It will end up in the error, Uncaught SyntaxError: Unexpected token '=' .
What if we declare the variable in advance and then try to destructure the same name's key from the object? Nope, not much luck here either. It is still syntactically incorrect.
In this case, the correct syntax is to put the destructuring expression inside parenthesis ( (...) ).
Please note that the parenthesis are required when you want to omit the let or const keyword in the destructuring expression itself.
Add a New Variable & Default Value
We can add a new variable while destructuring and add a default value to it. In the example below, the salary variable is non-existent in the user object. But we can add it in the destructuring expression and add a default value to it.
The alternative way to do the above is this:
There is a considerable advantage to the flexibility of adding a variable with a default value. The default value of this new variable is not necessarily going to be any constant value always. We can compute the value of it from other destructured property values.
Let's take a user object with two properties, first_name and last_name . We can now compute the value of a non-existent full_name using these two properties.
Isn't that elegant and useful!
Add Aliases
You can give an alias name to your destructured variables. It comes in very handy if you want to reduce the chances of variable name conflicts.
In the example below, we have specified an alias name for the property address as permanentAddress .
Please note, an attempt to access the variable address here will result in this error:

Nested Object Destructuring
An object can be nested. This means that the value of an object property can be another object, and so on.
Let's consider the user object below. It has a property called department with the value as another object. But let's not stop here! The department has a property with the key address whose value is another object. Quite a real-life scenario, isn't it?
How do we extract the value of the department property? Ok, it should be straight-forward by now.
And here's the output when you log department :

But, let's go one more nested level down. How do we extract the value of the address property of the department ? Now, this may sound a bit tricky. However, if you apply the same object destructuring principles, you'll see that it's similar.
Here's the output when you log address :

In this case, department is the key we focus on and we destructure the address value from it. Notice the {} around the keys you want to destructure.
Now it's time to take it to the next level. How do we extract the value of city from the department's address? Same principle again!
The output when you log city is "Bangalore".
It can go any level nested down.
The rule of thumb is to start with the top-level and go down in the hierarchy until you reach the value you want to extract.
Dynamic Name Property
Many times you may not know the property name (key) of an object while destructuring it. Consider this example. We have a user object:
Now the method getValue(key) takes a property key name and should return the value of it.
So, how do we write the definition of the getValue(key) method using the destructuring syntax?
Well, the syntax is very much the same as creating aliases. As we don't know the key name to hard-code in the destructuring syntax, we have to enclose it with square brackets ( [...] ).
Destructure to the Function Parameter
This one is my favorites, and it practically reduces lots of unnecessary code. You may want just a couple of specific property values to pass as a parameter to the function definition, not the entire object. Use object destructuring to function parameter in this case.
Let's take the user object example once again.
Suppose we need a function to return a string using the user's name and age. Say something like Alex is 43 year(s) old! is the return value when we call this:
We can simply use destructuring here to pass the name and age values, respectively, to the function definition. There is no need to pass the entire user object and then extract the values from it one by one. Please have a look:
Destructure Function Return Value
When a function returns an object and you are interested in specific property values, use destructuring straight away. Here is an example:
It is similar to the basic object destructuring we saw in the beginning.
Destructure in Loops
You can use object destructuring with the for-of loop. Let's take an array of user objects like this:
We can extract the property values with object destructuring using the for-of loop.
This is the output:
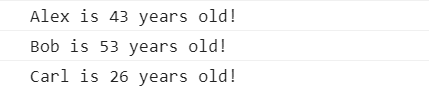
The Console object
In JavaScript, console is a built-in object supported by all browsers. If you have noticed, the console object has many properties and methods, and some are very popular, like console.log() .

Using the destructuring object syntax, we can simplify the uses of these methods and properties in our code. How about this?
Spread Syntax in JavaScript
The Spread Syntax (also known as the Spread Operator) is another excellent feature of ES6. As the name indicates, it takes an iterable (like an array) and expands (spreads) it into individual elements.
We can also expand objects using the spread syntax and copy its enumerable properties to a new object.
Spread syntax helps us clone an object with the most straightforward syntax using the curly braces and three dots {...} .
With spread syntax we can clone, update, and merge objects in an immutable way. The immutability helps reduce any accidental or unintentional changes to the original (Source) object.
The Object Destructuring and Spread syntaxes are not the same thing in JavaScript.
Create a Clone of an Object
We can create a cloned instance of an object using the spread syntax like this:
You can alternatively use object.assign() to create a clone of an object. However, the spread syntax is much more precise and much shorter.
The spread syntax performs a shallow copy of the object. This means that none of the nested object instances are cloned.
Add Properties to Objects
We can add a new property (key-value pair) to the object using the spread syntax . Note that the actual object never gets changed. The new property gets added to the cloned object.
In the example below, we are adding a new property ( salary ) using the spread syntax.
Update Properties
We can also update an existing property value using the spread syntax. Like the add operation, the update takes place on the object's cloned instance, not on the actual object.
In the example below, we are updating the value of the age property:
Update Nested Objects
As we have seen, updating an object with the spread syntax is easy, and it doesn't mutate the original object. However, it can be a bit tricky when you try to update a nested object using the spread syntax. Let's understand it with an example.
We have a user object with a property department . The value of the department property is an object which has another nested object with its address property.
Now, how can we add a new property called, number with a value of, say, 7 for the department object? Well, we might try out the following code to achieve it (but that would be a mistake):
As you execute it, you will realize that the code will replace the entire department object with the new value as, {'number': 7} . This is not what we wanted!

How do we fix that? We need to spread the properties of the nested object as well as add/update it. Here is the correct syntax that will add a new property number with the value 7 to the department object without replacing its value:
The output is the following:

Combine (or Merge) two Objects
The last practical use of the spread syntax in JavaScript objects is to combine or merge two objects. obj_1 and obj_2 can be merged together using the following syntax:
Note that this way of merging performs a shallow merge . This means that if there is a common property between both the objects, the property value of obj_2 will replace the property value of obj_1 in the merged object.
Let's take the user and department objects to combine (or merge) them together.
Merge the objects using the spread syntax, like this:
The output will be the following:

If we change the department object like this:
Now try to combine them and observe the combined object output:
The output will be:

The name property value of the user object is replaced by the name property value of the department object in the merged object output. So be careful of using it this way.
As of now, you need to implement the deep-merge of objects by yourself or make use of a library like lodash to accomplish it.
The Rest Parameter in JavaScript
The Rest parameter is kind of opposite to the spread syntax. While spread syntax helps expand or spread elements and properties, the rest parameter helps collect them together.
In the case of objects, the rest parameter is mostly used with destructuring syntax to consolidate the remaining properties in a new object you're working with.
Let's look at an example of the following user object:
We know how to destructure the age property to create a variable and assign the value of it. How about creating another object at the same time with the remaining properties of the user object? Here you go:

In the output we see that the age value is 43 . The rest parameter consolidated the rest of the user object properties, name and address , in a separate object.
To summarize,
- Object destructuring is new syntax introduced in ES6. It helps create variables by extracting the object's properties in a much simpler way.
- If you are working with (or planning to use) a framework/library like angular , react , or vue , you will be using a lot of object destructuring syntax.
- Object destructuring and Spread syntax are not the same thing.
- Spread syntax (also known as the Spread Operator) is used to copy the enumerable properties of an object to create a clone of it. We can also update an object or merge with another object using the spread syntax.
- The Rest parameter is kind of the opposite of the Spread syntax. It helps to consolidate (or collect) the remaining object properties into a new object while destructuring is done.
Before we go
I hope you've found this article insightful, and that it helps you start using these concepts more effectively. Let's connect. You will find me active on Twitter (@tapasadhikary) . Please feel free to give a follow.
You can find all the source code examples used in this article in my GitHub repository - js-tips-tricks . Are you interested in doing some hands-on coding based on what we have learned so far? Please have a look at the quiz here , and you may find it interesting.
You may also like these articles:
- How to Learn Something New Every Day as a Software Developer
- How to find blog content ideas effortlessly?
- Why do you need to do Side Projects as A Developer?
- 16 side project GitHub repositories you may find useful
Writer . YouTuber . Creator . Mentor
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Extract the assignment of from this expression with sonarQube

when I launch a sonar control I find myself with a smells code for the following function:
I get the following sonar alert:
Extract the assignment of “item.media” from this expression.
What would be the solution to avoid this sonar message?
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra that is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, items list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
JavaScript: The Definitive Guide, 6th Edition by David Flanagan
Get full access to JavaScript: The Definitive Guide, 6th Edition and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Assignment Expressions
JavaScript uses the = operator to assign a value to a variable or property. For example:
The = operator expects its left-side operand to be an lvalue: a variable or object property (or array element). It expects its right-side operand to be an arbitrary value of any type. The value of an assignment expression is the value of the right-side operand. As a side effect, the = operator assigns the value on the right to the variable or property on the left so that future references to the variable or property evaluate to the value.
Although assignment expressions are usually quite simple, you may sometimes see the value of an assignment expression used as part of a larger expression. For example, you can assign and test a value in the same expression with code like this:
If you do this, be sure you are clear on the difference between the = and == operators! Note that = has very low precedence and parentheses are usually necessary when the value of an assignment is to be used in a larger expression.
The assignment operator has right-to-left associativity, which means that when multiple assignment operators appear in an expression, they are evaluated from right to left. Thus, you can write code like this to assign a single value to multiple variables:
Assignment with Operation
Besides the normal = assignment operator, JavaScript supports a number of other assignment operators that provide shortcuts by combining assignment with some other operation. For example, the += operator performs addition and assignment. The following expression:
is equivalent to this one:
As you might expect, the += operator works for numbers or strings. For numeric operands, it performs addition and assignment; for string operands, it performs concatenation and assignment.
Similar operators include -= , *= , &= , and so on. Table 4-3 lists them all.
Table 4-3. Assignment operators
In most cases, the expression:
where op is an operator, is equivalent to the expression:
In the first line, the expression a is evaluated once. In the second it is evaluated twice. The two cases will differ only if a includes side effects such as a function call or an increment operator. The following two assignments, for example, are not the same:
Get JavaScript: The Definitive Guide, 6th Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
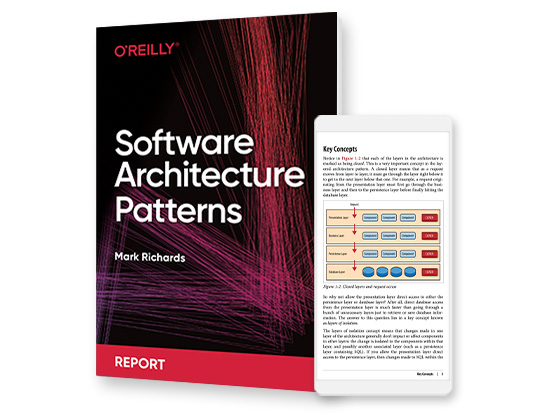
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
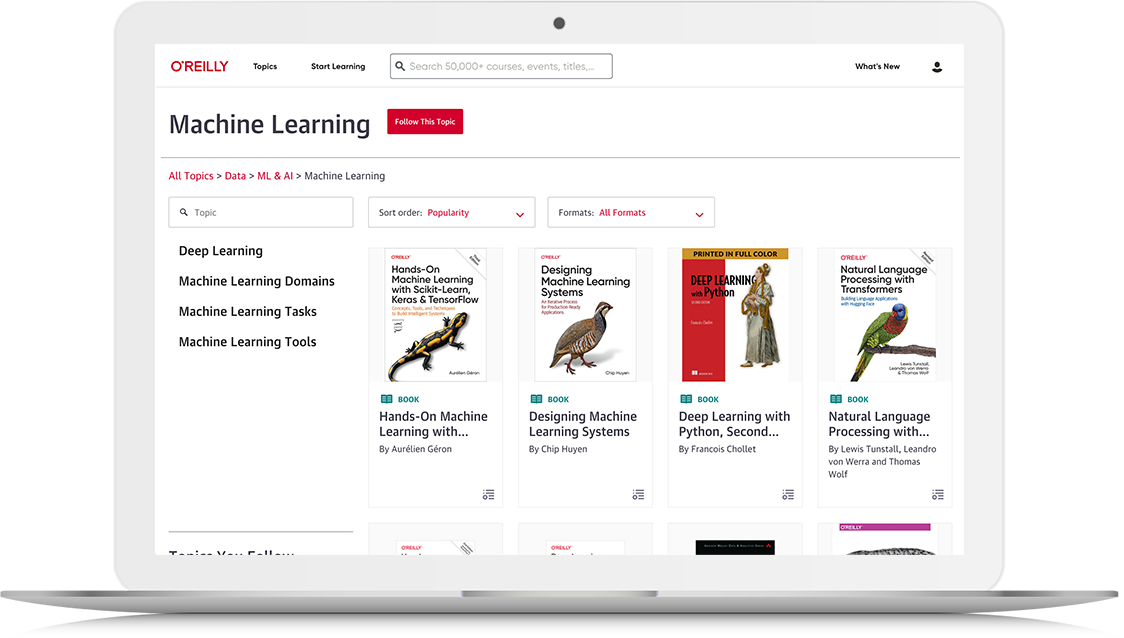
How to use destructuring assignment in JavaScript
Destructuring assignment is a powerful feature in JavaScript that allows you to extract values from arrays or properties from objects and assign them to variables in a concise and expressive way.
Destructuring assignment can be useful for:
- Reducing the amount of code and improving readability.
- Assigning default values to variables in case the source value is undefined.
- Renaming the variables that you assign from the source.
- Swapping the values of two variables without using a temporary variable.
- Extracting values from nested arrays or objects.
Array destructuring
Array destructuring in JavaScript is a syntax that allows you to extract individual elements from an array and assign them to distinct variables. Enclose the variables you want to assign values to within square brackets [] on the left-hand side of the assignment operator = , and place the array you want to destructure on the right-hand side.
Object destructuring
Object destructuring in JavaScript is a syntax that allows you to extract individual properties from an object and assign them to distinct variables in a single line of code, significantly reducing the boilerplate code needed compared to traditional methods like dot notation or bracket notation.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
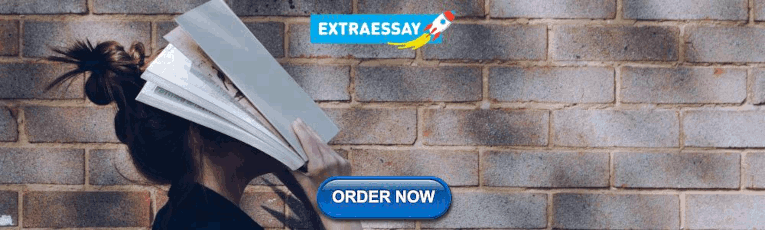
Destructuring Assignment in JavaScript
- How to swap variables using destructuring assignment in JavaScript ?
- Division Assignment(/=) Operator in JavaScript
- Exponentiation Assignment(**=) Operator in JavaScript
- What is a Destructuring assignment and explain it in brief in JavaScript ?
- Describe closure concept in JavaScript
- Copy Constructor in JavaScript
- Classes In JavaScript
- JavaScript Function() Constructor
- Multiple Class Constructors in JavaScript
- Implementation of Array class in JavaScript
- Functions in JavaScript
- JavaScript TypeError - Invalid assignment to const "X"
- Scoping & Hoisting in JavaScript
- How to set default values when destructuring an object in JavaScript ?
- What is Parameter Destructuring in TypeScript ?
- JavaScript Hoisting
- Addition Assignment (+=) Operator in Javascript
- Array of functions in JavaScript
- Enums in JavaScript
- How to calculate the number of days between two dates in JavaScript ?
- Convert a String to an Integer in JavaScript
- How to append HTML code to a div using JavaScript ?
- How to Open URL in New Tab using JavaScript ?
- Difference between var and let in JavaScript
- How do you run JavaScript script through the Terminal?
- Remove elements from a JavaScript Array
- How to read a local text file using JavaScript?
- JavaScript console.log() Method
- JavaScript Number toString() Method
Destructuring Assignment is a JavaScript expression that allows to unpack values from arrays, or properties from objects, into distinct variables data can be extracted from arrays, objects, nested objects and assigning to variables . In Destructuring Assignment on the left-hand side defined that which value should be unpacked from the sourced variable. In general way implementation of the extraction of the array is as shown below: Example:
- Array destructuring:
Object destructuring:
Array destructuring: Using the Destructuring Assignment in JavaScript array possible situations, all the examples are listed below:
- Example 1: When using destructuring assignment the same extraction can be done using below implementations.
- Example 2: The array elements can be skipped as well using a comma separator. A single comma can be used to skip a single array element. One key difference between the spread operator and array destructuring is that the spread operator unpacks all array elements into a comma-separated list which does not allow us to pick or choose which elements we want to assign to variables. To skip the whole array it can be done using the number of commas as there is a number of array elements.
- Example 3: In order to assign some array elements to variable and rest of the array elements to only a single variable can be achieved by using rest operator (…) as in below implementation. But one limitation of rest operator is that it works correctly only with the last elements implying a subarray cannot be obtained leaving the last element in the array.
- Example 4: Values can also be swapped using destructuring assignment as below:
- Example 5: Data can also be extracted from an array returned from a function. One advantage of using a destructuring assignment is that there is no need to manipulate an entire object in a function but just the fields that are required can be copied inside the function.
- Example 6: In ES5 to assign variables from objects its implementation is
- Example 7: The above implementation in ES6 using destructuring assignment is.
- Example1: The Nested objects can also be destructured using destructuring syntax.
- Example2: Nested objects can also be destructuring
Please Login to comment...
Similar reads.
- JavaScript-Questions
- Web Technologies
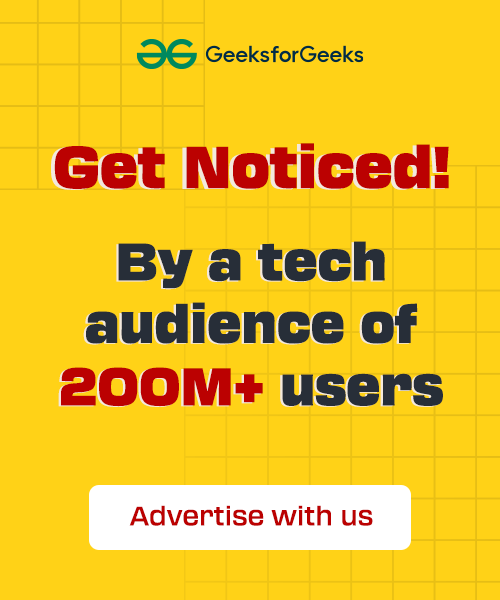
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
How to use object & array destructuring in JavaScript
Array destructuring, skipping values, destructuring a part of the array, assigning values to an object, default values, swapping variables, object destructuring, using a new variable name, computed property name, extracting arrays from the object, nested objects destructuring, destructuring a part of the object.
The destructuring assignment is a handy addition to ES6 that allows us to extract values from arrays , or properties from objects , into a bunch of distinct variables. It is a special syntax that unpacks arrays and objects into multiple variables.
Destructuring is extremely useful when you don't want to pass the object or the array to a function as a whole but as individual pieces. Let us see how it is done in the following examples.
The array destructuring syntax automatically creates variables with the values from the corresponding items in an array:
The unwanted items of the array can be skipped by using an extra comma and leaving the variable names empty:
You can even choose to destructure a part of the array and assign the rest of the collection to a new variable:
What if you want to assign the array's values to an object? You can easily do it with array destructuring:
If there are fewer values in the array than variables specified in the assignment, there won't be any error. Destructuring automatically assigns default values to extra variables:
You can also define your own default values for variables in the assignment:
The age property falls back to 30 because it is not defined in the array.
The destructuring assignment can also be used to swap the values of two variables:
The destructuring assignment also works with JavaScript objects. However, the assignment variables must have matching names with the object's keys. This is because the object keys are in a variety of order.
Here is a basic example:
If you want to assign values of an object to new keys instead of using the name of the existing object keys, you can do the following:
Just like array destructuring, the default values can also be used in object destructuring:
You can also set default values when you assign value to a new variable:
The computed property name is another ES6 feature that works for object destructuring. It allows the names of the object properties in JavaScript object literal notation to be computed dynamically.
Here is an example that computes the name of the object property by using "Computed Property Name" notation:
The object destructuring also works for the arrays present inside the object as values:
The object may contain nested objects when destructuring. You have to define the same nesting structure at the left side of the assignment to extract values from deeper objects:
All properties of the user object are assigned to variables in the assignment. Finally, we have name , city , and country distinct variables. Note that there is no variable for place as we only extracted its content.
Just like arrays, you can also destructure a part of the object and assign the rest of the object to a new variable:
✌️ Like this article? Follow me on Twitter and LinkedIn . You can also subscribe to RSS Feed .
You might also like...
- Get the length of a Map in JavaScript
- Delete an element from a Map in JavaScript
- Get the first element of a Map in JavaScript
- Get an element from a Map using JavaScript
- Update an element in a Map using JavaScript
- Add an element to a Map in JavaScript
The simplest cloud platform for developers & teams. Start with a $200 free credit.
Buy me a coffee ☕
If you enjoy reading my articles and want to help me out paying bills, please consider buying me a coffee ($5) or two ($10). I will be highly grateful to you ✌️
Enter the number of coffees below:
✨ Learn to build modern web applications using JavaScript and Spring Boot
I started this blog as a place to share everything I have learned in the last decade. I write about modern JavaScript, Node.js, Spring Boot, core Java, RESTful APIs, and all things web development.
The newsletter is sent every week and includes early access to clear, concise, and easy-to-follow tutorials, and other stuff I think you'd enjoy! No spam ever, unsubscribe at any time.
- JavaScript, Node.js & Spring Boot
- In-depth tutorials
- Super-handy protips
- Cool stuff around the web
- 1-click unsubscribe
- No spam, free-forever!
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- Skip to main content
- Select language
- Skip to search
- Add a translation
- Print this page
- Destructuring assignment
Pulling values from a regular expression match
Es6 version, computed object property names and destructuring, firefox-specific notes.
This is an experimental technology, part of the ECMAScript 6 (Harmony) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
{a, b} = {a:1, b:2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal.
However, the ({a, b} = {a:1, b:2}) form is valid, as is the var {a, b} = {a:1, b:2} form.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data. Once you've created these packages of data, you can use them any way you want to. You can even return them from functions.
One particularly useful thing you can do with destructuring assignment is to read an entire structure in a single statement, although there are a number of interesting things you can do with them, as shown in the section full of examples that follows.
This capability is similar to features present in languages such as Perl and Python.
Array destructuring
Simple example, assignment without declaration.
Destructuring assignment can be made without a declaration in the assignment statement.
Swapping variables
After executing this code, b is 1 and a is 3. Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Multiple-value returns
Thanks to destructuring assignment, functions can return multiple values. While it's always been possible to return an array from a function, this provides an added degree of flexibility.
As you can see, returning results is done using an array-like notation, with all the values to return enclosed in brackets. You can return any number of results in this way. In this example, f() returns the values [1, 2] as its output.
The statement [a, b] = f() assigns the results of the function to the variables in brackets, in order: a is set to 1 and b is set to 2.
You can also retrieve the return values as an array:
In this case, a is an array containing the values 1 and 2.
Ignoring some returned values
You can also ignore return values that you're not interested in:
After running this code, a is 1 and b is 3. The value 2 is ignored. You can ignore any (or all) returned values this way. For example:
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to pull the parts out of this array easily, ignoring the full match if it is not needed.
Object destructuring
The ( .. ) around the assignment statement is required syntax when using object literal destructuring assignment without a declaration.
Function argument defaults
Es5 version.
In Firefox, default values for destructuring assignments are not yet implemented: var { x = 3 } = {} and var [foo = "bar"] = []. See bug 932080 and also bug 1018628 for destructured default values in functions.
Module (non-ES6) loading
Destructuring can help to load specific subsets of a non-ES6 module like here in the Add-on SDK :
Nested object and array destructuring
For of iteration and destructuring, pulling fields from objects passed as function parameter.
This pulls the id , displayName and firstName from the user object and prints them.
Computed property names, like on object literals , can be used with destructuring.
Specifications
Browser compatibility.
- Firefox provided a non-standard language extension in JS1.7 for destructuring. This extension has been removed in Gecko 40 (Firefox 40 / Thunderbird 40 / SeaMonkey 2.37). See bug 1083498 .
- Assignment operators
Document Tags and Contributors
- Destructuring
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- JavaScript basics
- JavaScript technologies overview
- Introduction to Object Oriented JavaScript
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- Standard built-in objects
- ArrayBuffer
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread operator
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Statements and declarations
- Legacy generator function
- for each...in
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template strings
- Deprecated features
- New in JavaScript
- ECMAScript 5 support in Mozilla
- ECMAScript 6 support in Mozilla
- ECMAScript 7 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
S1121 possible false positive when using with expressions on records #4417
rubiktubik commented May 12, 2021
pavel-mikula-sonarsource commented May 12, 2021
- 👍 1 reaction
- 🎉 1 reaction
Sorry, something went wrong.
No branches or pull requests
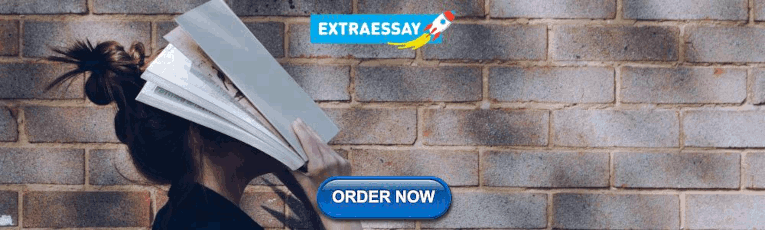
COMMENTS
Extract the assignment of from this expression with sonarQube. Ask Question Asked 1 year, 8 months ago. ... Extract the assignment of "item.media" from this expression. What would be the solution to avoid this sonar message? javascript; sonarqube; ... Convert string to expression in JavaScript. 3.
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ...
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. ... the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object ...
The expression to extract the name property value using object destructuring is the following: const { name } = user; console.log(name); // Output, Alex As you see, on the left side of the expression, we pick the object property key ( name in this case) and place it inside the {} .
Extract the assignment of from this expression with sonarQube. javascript sonarqube. Greg-A. asked 24 Aug, 2022. when I launch a sonar control I find myself with a smells code for the following function: JavaScript. 13. 1. const changeMedia = (value: any, step: any) => {.
It's called "destructuring assignment," because it "destructurizes" by copying items into variables. However, the array itself is not modified. It's just a shorter way to write: // let [firstName, surname] = arr; let firstName = arr [0]; let surname = arr [1]; Ignore elements using commas.
Assignment Expressions. JavaScript uses the = operator to assign a value to a variable or property. For example: i = 0 // Set the variable i to 0. o.x = 1 // Set the property x of object o to 1. The = operator expects its left-side operand to be an lvalue: a variable or object property (or array element).
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Array destructuring in JavaScript is a syntax that allows you to extract individual elements from an array and assign them to distinct variables. Enclose the variables you want to assign values to within square brackets [] on the left-hand side of the assignment operator =, and place the array you want to destructure on the right-hand side.
The ( ..) around the assignment statement is required syntax when using object literal destructuring assignment without a declaration. {a, b} = {a: 1, b: 2} is not valid stand-alone syntax, as the {a, b} on the left-hand side is considered a block and not an object literal. However, ({a, b} = {a: 1, b: 2}) is valid, as is var {a, b} = {a: 1, b: 2} NOTE: Your ( ..) expression needs to be ...
Last Updated : 20 Feb, 2023. Destructuring Assignment is a JavaScript expression that allows to unpack values from arrays, or properties from objects, into distinct variables data can be extracted from arrays, objects, nested objects and assigning to variables. In Destructuring Assignment on the left-hand side defined that which value should be ...
How to use object & array destructuring in JavaScript. The destructuring assignment is a handy addition to ES6 that allows us to extract values from arrays, or properties from objects, into a bunch of distinct variables. It is a special syntax that unpacks arrays and objects into multiple variables.
Apart from the dedicated statement syntaxes, you can also use almost any expression as a statement on its own. The expression statement syntax requires a semicolon at the end, but the automatic semicolon insertion process may insert one for you if the lack of a semicolon results in invalid syntax.. Because the expression is evaluated and then discarded, the result of the expression is not ...
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes. The destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
In JavaScript the = operator is an expression and evaluates the assigned value. Because it is an expression it can be used anywhere an expression is allowed even though it causes a side-effect. Thus: settings.maxId != null && (params.max_id = settings.maxId)
Expected behavior. No Warning. Actual behavior. Getting a S1121 Warning with "Extract the assignment of 'Value' from this expression.". Known workarounds. Disable the rule. Related information. C#/VB.NET Plugins version - SonarLint 4.3
This study aimed to investigate how diets supplemented with DHA-rich algae affect the expression of liver lipid synthesis genes in dairy goat bucks. The results revealed that when supplemented with DHA-rich algae, liver weight and serum HDL-C were significantly increased (p < 0.05), as well as serum LDL-C was significantly decreased (p < 0.05). Transcriptome sequencing indicated that algae ...
if you get the data from Ajax request you need to parse it like this: var newData=JSON.parse(data).pri_tag; if not, you don't need to parse that: var newData=data.pri_tag; edited Mar 25, 2014 at 8:21. answered Mar 25, 2014 at 8:04. Super Hornet. 2,867 5 29 58.