JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript operators.
Javascript operators are used to perform different types of mathematical and logical computations.
The Assignment Operator = assigns values
The Addition Operator + adds values
The Multiplication Operator * multiplies values
The Comparison Operator > compares values
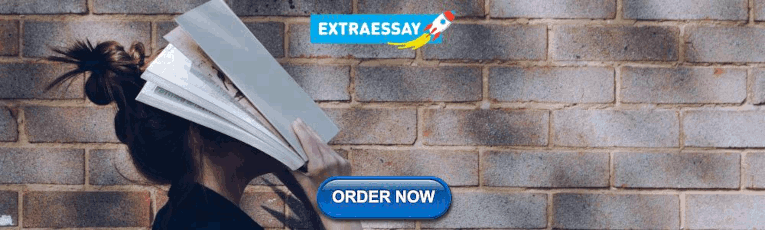
JavaScript Assignment
The Assignment Operator ( = ) assigns a value to a variable:
Assignment Examples
Javascript addition.
The Addition Operator ( + ) adds numbers:
JavaScript Multiplication
The Multiplication Operator ( * ) multiplies numbers:
Multiplying
Types of javascript operators.
There are different types of JavaScript operators:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- String Operators
- Logical Operators
- Bitwise Operators
- Ternary Operators
- Type Operators
JavaScript Arithmetic Operators
Arithmetic Operators are used to perform arithmetic on numbers:
Arithmetic Operators Example
Arithmetic operators are fully described in the JS Arithmetic chapter.
Advertisement
JavaScript Assignment Operators
Assignment operators assign values to JavaScript variables.
The Addition Assignment Operator ( += ) adds a value to a variable.
Assignment operators are fully described in the JS Assignment chapter.
JavaScript Comparison Operators
Comparison operators are fully described in the JS Comparisons chapter.
JavaScript String Comparison
All the comparison operators above can also be used on strings:
Note that strings are compared alphabetically:
JavaScript String Addition
The + can also be used to add (concatenate) strings:
The += assignment operator can also be used to add (concatenate) strings:
The result of text1 will be:
When used on strings, the + operator is called the concatenation operator.
Adding Strings and Numbers
Adding two numbers, will return the sum, but adding a number and a string will return a string:
The result of x , y , and z will be:
If you add a number and a string, the result will be a string!
JavaScript Logical Operators
Logical operators are fully described in the JS Comparisons chapter.
JavaScript Type Operators
Type operators are fully described in the JS Type Conversion chapter.
JavaScript Bitwise Operators
Bit operators work on 32 bits numbers.
The examples above uses 4 bits unsigned examples. But JavaScript uses 32-bit signed numbers. Because of this, in JavaScript, ~ 5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
Bitwise operators are fully described in the JS Bitwise chapter.
Test Yourself With Exercises
Multiply 10 with 5 , and alert the result.
Start the Exercise
Test Yourself with Exercises!
Exercise 1 » Exercise 2 » Exercise 3 » Exercise 4 » Exercise 5 »

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
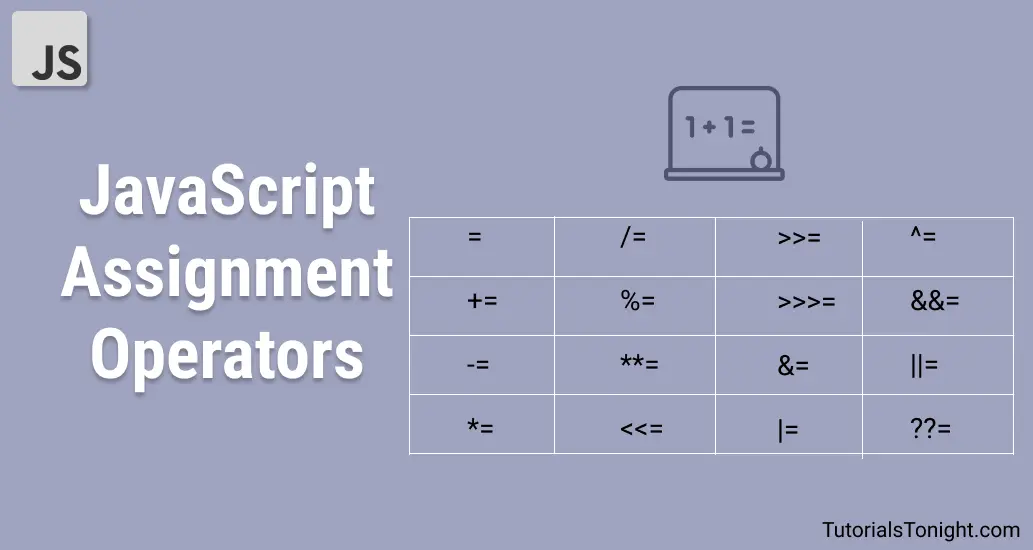
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Comparison and Logical Operators
JavaScript Booleans
JavaScript Bitwise Operators
JavaScript Ternary Operator
- JavaScript Object.is()
- JavaScript console.log()
JavaScript operators are special symbols that perform unique operations on one or more operands (values). For example,
The + operator performs addition, we have used it here to add the operands 2 and 3 .
JavaScript Operator Types
Here is a list of different JavaScript operators you will learn in this tutorial:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- String Operators
- Miscellaneous Operators
1. JavaScript Arithmetic Operators
We use arithmetic operators to perform arithmetic calculations like addition, subtraction, etc. For example,
Here, we used the - operator to subtract 3 from 5 .
Commonly Used Arithmetic Operators
Example 1: arithmetic operators in javascript, example 2: javascript increment and decrement operators.
To learn more about the increment and decrement operators, visit Increment ++ and Decrement -- Operators .
2. JavaScript Assignment Operators
We use assignment operators to assign values to variables. For example,
Here, we used the = operator to assign the value 5 to variable x .
Commonly Used Assignment Operators
Example 3: assignment operators in javascript, 3. javascript comparison operators.
We use comparison operators to compare two values and return a boolean value ( true or false ). For example,
Here, we have used the > comparison operator to check whether a (whose value is 3 ) is greater than b (whose value is 2 ).
Since 3 is greater than 2 , we get true as output.
Commonly Used Comparison Operators
Example 4: comparison operators in javascript.
The equality operators ( == and != ) convert both operands to the same type before comparing their values. For example,
Here, we used the == operator to compare the number 3 and the string 3 .
By default, JavaScript converts string 3 to number 3 and compares the values.
However, the strict equality operators ( === and !== ) do not convert operand types before comparing their values. For example,
Here, JavaScript didn't convert string 4 to number 4 before comparing their values.
Thus, the result is false , as number 4 isn't equal to string 4 .
4. JavaScript Logical Operators
We use logical operators to perform logical operations on boolean values. For example,
Here, && is the logical operator AND . Since both x < 6 and y < 5 are true , the combined result is true .
Commonly Used Logical Operators
Example 5: logical operators in javascript.
Note: We use comparison and logical operators in decision-making and loops. You will learn about them in detail in later tutorials.
We use bitwise operators to perform binary operations on integers.
Note: We rarely use bitwise operators in everyday programming. If you are interested, visit JavaScript Bitwise Operators to learn more.
6. JavaScript String Operators
In JavaScript, you can use the + operator to concatenate (join) two or more strings . For example,
Here, we used + on strings to perform concatenation. However, when we use + with numbers, it performs addition.
7. JavaScript Miscellaneous Operators
JavaScript has many more operators besides the ones we listed above.
Thus, we have categorized them as miscellaneous operators. You will learn them in detail in later tutorials.
Commonly Used Miscellaneous Operators
- JavaScript typeof Operator
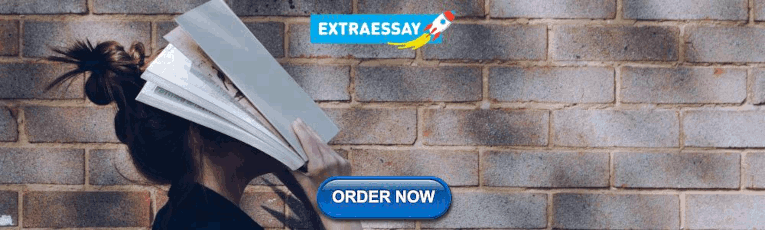
Table of Contents
- Introduction
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
- JavaScript String Operators
- JavaScript Miscellaneous Operators
Video: JavaScript Operators
Sorry about that.
Related Tutorials
JavaScript Tutorial
Home » JavaScript Tutorial » JavaScript Logical Assignment Operators
JavaScript Logical Assignment Operators
Summary : in this tutorial, you’ll learn about JavaScript logical assignment operators, including the logical OR assignment operator ( ||= ), the logical AND assignment operator ( &&= ), and the nullish assignment operator ( ??= ).
ES2021 introduces three logical assignment operators including:
- Logical OR assignment operator ( ||= )
- Logical AND assignment operator ( &&= )
- Nullish coalescing assignment operator ( ??= )
The following table shows the equivalent of the logical assignments operator:
The Logical OR assignment operator
The logical OR assignment operator ( ||= ) accepts two operands and assigns the right operand to the left operand if the left operand is falsy:
In this syntax, the ||= operator only assigns y to x if x is falsy. For example:
In this example, the title variable is undefined , therefore, it’s falsy. Since the title is falsy, the operator ||= assigns the 'untitled' to the title . The output shows the untitled as expected.
See another example:
In this example, the title is 'JavaScript Awesome' so it is truthy. Therefore, the logical OR assignment operator ( ||= ) doesn’t assign the string 'untitled' to the title variable.
The logical OR assignment operator:
is equivalent to the following statement that uses the logical OR operator :
Like the logical OR operator, the logical OR assignment also short-circuits. It means that the logical OR assignment operator only performs an assignment when the x is falsy.
The following example uses the logical assignment operator to display a default message if the search result element is empty:
The Logical AND assignment operator
The logical AND assignment operator only assigns y to x if x is truthy:
The logical AND assignment operator also short-circuits. It means that
is equivalent to:
The following example uses the logical AND assignment operator to change the last name of a person object if the last name is truthy:
The nullish coalescing assignment operator
The nullish coalescing assignment operator only assigns y to x if x is null or undefined :
It’s equivalent to the following statement that uses the nullish coalescing operator :
The following example uses the nullish coalescing assignment operator to add a missing property to an object:
In this example, the user.nickname is undefined , therefore, it’s nullish. The nullish coalescing assignment operator assigns the string 'anonymous' to the user.nickname property.
The following table illustrates how the logical assignment operators work:
- The logical OR assignment ( x ||= y ) operator only assigns y to x if x is falsy.
- The logical AND assignment ( x &&= y ) operator only assigns y to x if x is truthy.
- The nullish coalescing assignment ( x ??= y ) operator only assigns y to x if x is nullish.
Learn JavaScript Operators – Logical, Comparison, Ternary, and More JS Operators With Examples

JavaScript has many operators that you can use to perform operations on values and variables (also called operands)
Based on the types of operations these JS operators perform, we can divide them up into seven groups:
Arithmetic Operators
Assignment operators, comparison operators, logical operators.
- Ternary Operators
The typeof Operator
Bitwise operators.
In this handbook, you're going to learn how these operators work with examples. Let's start with arithmetic operators.
The arithmetic operators are used to perform mathematical operations like addition and subtraction.
These operators are frequently used with number data types, so they are similar to a calculator. The following example shows how you can use the + operator to add two variables together:
Here, the two variables x and y are added together using the plus + operator. We also used the console.log() method to print the result of the operation to the screen.
You can use operators directly on values without assigning them to any variable too:
In JavaScript, we have 8 arithmetic operators in total. They are:
- Subtraction -
- Multiplication *
- Remainder %
- Exponentiation **
- Increment ++
- Decrement --
Let's see how these operators work one by one.
1. Addition operator
The addition operator + is used to add two or more numbers together. You've seen how this operator works previously, but here's another example:
You can use the addition operator on both integer and floating numbers.
2. Subtraction operator
The subtraction operator is marked by the minus sign − and you can use it to subtract the right operand from the left operand.
For example, here's how to subtract 3 from 5:
3. Multiplication operator
The multiplication operator is marked by the asterisk * symbol, and you use it to multiply the value on the left by the value on the right of the operator.
4. Division operator
The division operator / is used to divide the left operand by the right operand. Here are some examples of using the operator:
5. Remainder operator
The remainder operator % is also known as the modulo or modulus operator. This operator is used to calculate the remainder after a division has been performed.
A practical example should make this operator easier to understand, so let's see one:
The number 10 can't be divided by 3 perfectly. The result of the division is 3 with a remainder of 1. The remainder operator simply returns that remainder number.
If the left operand can be divided with no remainder, then the operator returns 0.
This operator is commonly used when you want to check if a number is even or odd. If a number is even, dividing it by 2 will result in a remainder of 0, and if it's odd, the remainder will be 1.
6. Exponentiation operator
The exponentiation operator is marked by two asterisks ** . It's one of the newer JavaScript operators and you can use it to calculate the power of a number (based on its exponent).
For example, here's how to calculate 10 to the power of 3:
Here, the number 10 is multiplied by itself 3 times (10 _ 10 _ 10)
The exponentiation operator gives you an easy way to find the power of a specific number.
7. Increment operator
The increment ++ operator is used to increase the value of a number by one. For example:
This operator gives you a faster way to increase a variable value by one. Without the operator, here's how you increment a variable:
Using the increment operator allows you to shorten the second line. You can place this operator before or next to the variable you want to increment:
Both placements shown above are valid. The difference between prefix (before) and postfix (after) placements is that the prefix position will execute the operator after that line of code has been executed.
Consider the following example:
Here, you can see that placing the increment operator next to the variable will print the variable as if it has not been incremented.
When you place the operator before the variable, then the number will be incremented before calling the console.log() method.
8. Decrement operator
The decrement -- operator is used to decrease the value of a number by one. It's the opposite of the increment operator:
Please note that you can only use increment and decrement operators on a variable. An error occurs when you try to use these operators directly on a number value:
You can't use increment or decrement operator on a number directly.
Arithmetic operators summary
Now you've learned the 8 types of arithmetic operators. Excellent! Keep in mind that you can mix these operators to perform complex mathematical equations.
For example, you can perform an addition and multiplication on a set of numbers:
The order of operations in JavaScript is the same as in mathematics. Multiplication, division, and exponentiation take a higher priority than addition or subtraction (remember that acronym PEMDAS? Parentheses, exponents, multiplication and division, addition and subtraction – there's your order of operations).
You can use parentheses () to change the order of the operations. Wrap the operation you want to execute first as follows:
When using increment or decrement operators together with other operators, you need to place the operators in a prefix position as follows:
This is because a postfix increment or decrement operator will not be executed together with other operations in the same line, as I have explained previously.
Let's try some exercises. Can you guess the result of these operations?
And that's all for arithmetic operators. You've done a wonderful job learning about these operators.
Let's take a short five-minute break before proceeding to the next type of operators.
The second group of operators we're going to explore is the assignment operators.
Assignment operators are used to assign a specific value to a variable. The basic assignment operator is marked by the equal = symbol, and you've already seen this operator in action before:
After the basic assignment operator, there are 5 more assignment operators that combine mathematical operations with the assignment. These operators are useful to make your code clean and short.
For example, suppose you want to increment the x variable by 2. Here's how you do it with the basic assignment operator:
There's nothing wrong with the code above, but you can use the addition assignment += to rewrite the second line as follows:
There are 7 kinds of assignment operators that you can use in JavaScript:
The arithmetic operators you've learned in the previous section can be combined with the assignment operator except the increment and decrement operators.
Let's have a quick exercise. Can you guess the results of these assignments?
Now you've learned about assignment operators. Let's continue and learn about comparison operators.
As the name implies, comparison operators are used to compare one value or variable with something else. The operators in this category always return a boolean value: either true or false .
For example, suppose you want to compare if a variable's value is greater than 1. Here's how you do it:
The greater than > operator checks if the value on the left operand is greater than the value on the right operand.
There are 8 kinds of comparison operators available in JavaScript:
Here are some examples of using comparison operators:
The comparison operators are further divided in two types: relational and equality operators.
The relational operators compare the value of one operand relative to the second operand (greater than, less than)
The equality operators check if the value on the left is equal to the value on the right. They can also be used to compare strings like this:
String comparisons are case-sensitive, as shown in the example above.
JavaScript also has two versions of the equality operators: loose and strict.
In strict mode, JavaScript will compare the values without performing a type coercion. To enable strict mode, you need to add one more equal = symbol to the operation as follows:
Since type coercion might result in unwanted behavior, you should use the strict equality operators anytime you do an equality comparison.
Logical operators are used to check whether one or more expressions result in either true or false .
There are three logical operators available in JavaScript:
These operators can only return Boolean values. For example, you can determine whether '7 is greater than 2' and '5 is greater than 4':
These logical operators follow the laws of mathematical logic:
- && AND operator – if any expression returns false , the result is false
- || OR operator – if any expression returns true , the result is true
- ! NOT operator – negates the expression, returning the opposite.
Let's do a little exercise. Try to run these statements on your computer. Can you guess the results?
These logical operators will come in handy when you need to assert that a specific requirement is fulfilled in your code.
Let's say a happyLife requires a job with highIncome and supportiveTeam :
Based on the requirements, you can use the logical AND operator to check whether you have both requirements. When one of the requirements is false , then happyLife equals false as well.
Ternary Operator
The ternary operator (also called the conditional operator) is the only JavaScipt operator that requires 3 operands to run.
Let's imagine you need to implement some specific logic in your code. Suppose you're opening a shop to sell fruit. You give a $3 discount when the total purchase is $20 or more. Otherwise, you give a $1 discount.
You can implement the logic using an if..else statement as follows:
The code above works fine, but you can use the ternary operator to make the code shorter and more concise as follows:
The syntax for the ternary operator is condition ? expression1 : expression2 .
You need to write the condition to evaluate followed by a question ? mark.
Next to the question mark, you write the expression to execute when the condition evaluates to true , followed by a colon : symbol. You can call this expression1 .
Next to the colon symbol, you write the expression to execute when the condition evaluates to false . This is expression2 .
As the example above shows, the ternary operator can be used as an alternative to the if..else statement.
The typeof operator is the only operator that's not represented by symbols. This operator is used to check the data type of the value you placed on the right side of the operator.
Here are some examples of using the operator:
The typeof operator returns the type of the data as a string. The 'number' type represents both integer and float types, the string and boolean represent their respective types.
Arrays, objects, and the null value are of object type, while undefined has its own type.
Bitwise operators are operators that treat their operands as a set of binary digits, but return the result of the operation as a decimal value.
These operators are rarely used in web development, so you can skip this part if you only want to learn practical stuff. But if you're interested to know how they work, then let me show you an example.
A computer uses a binary number system to store decimal numbers in memory. The binary system only uses two numbers, 0 and 1, to represent the whole range of decimal numbers we humans know.
For example, the decimal number 1 is represented as binary number 00000001, and the decimal number 2 is represented as 00000010.
I won't go into detail on how to convert a decimal number into a binary number as that's too much to include in this guide. The main point is that the bitwise operators operate on these binary numbers.
If you want to find the binary number from a specific decimal number, you can Google for the "decimal to binary calculator".
There are 7 types of bitwise operators in JavaScript:
- Left Shift <<
- Right Shift >>
- Zero-fill Right Shift >>>
Let's see how they work.
1. Bitwise AND operator
The bitwise operator AND & returns a 1 when the number 1 overlaps in both operands. The decimal numbers 1 and 2 have no overlapping 1, so using this operator on the numbers return 0:
2. Bitwise OR operator
On the other hand, the bitwise operator OR | returns all 1s in both decimal numbers.
The binary number 00000011 represents the decimal number 3, so the OR operator above returns 3.
Bitwise XOR operator
The Bitwise XOR ^ looks for the differences between two binary numbers. When the corresponding bits are the same, it returns 0:
5 = 00000101
Bitwise NOT operator
Bitwise NOT ~ operator inverts the bits of a decimal number so 0 becomes 1 and 1 becomes 0:
Bitwise Left Shift operator
Bitwise Left Shift << shifts the position of the bit by adding zeroes from the right.
The excess bits are then discarded, changing the decimal number represented by the bits. See the following example:
The right operand is the number of zeroes you will add to the left operand.
Bitwise Right Shift operator
Bitwise Right Shift >> shifts the position of the bits by adding zeroes from the left. It's the opposite of the Left Shift operator:
Bitwise Zero-fill Right Shift operator
Also known as Unsigned Right Shift operator, the Zero-fill Right Shift >>> operator is used to shift the position of the bits to the right, while also changing the sign bit to 0 .
This operator transforms any negative number into a positive number, so you can see how it works when passing a negative number as the left operand:
In the above example, you can see that the >> and >>> operators return different results. The Zero-fill Right Shift operator has no effect when you use it on a positive number.
Now you've learned how the bitwise operators work. If you think they are confusing, then you're not alone! Fortunately, these operators are scarcely used when developing web applications.
You don't need to learn them in depth. It's enough to know what they are.
In this tutorial, you've learned the 7 types of JavaScript operators: Arithmetic, assignment, comparison, logical, ternary, typeof, and bitwise operators.
These operators can be used to manipulate values and variables to achieve a desired outcome.
Congratulations on finishing this guide!
If you enjoyed this article and want to take your JavaScript skills to the next level, I recommend you check out my new book Beginning Modern JavaScript here .
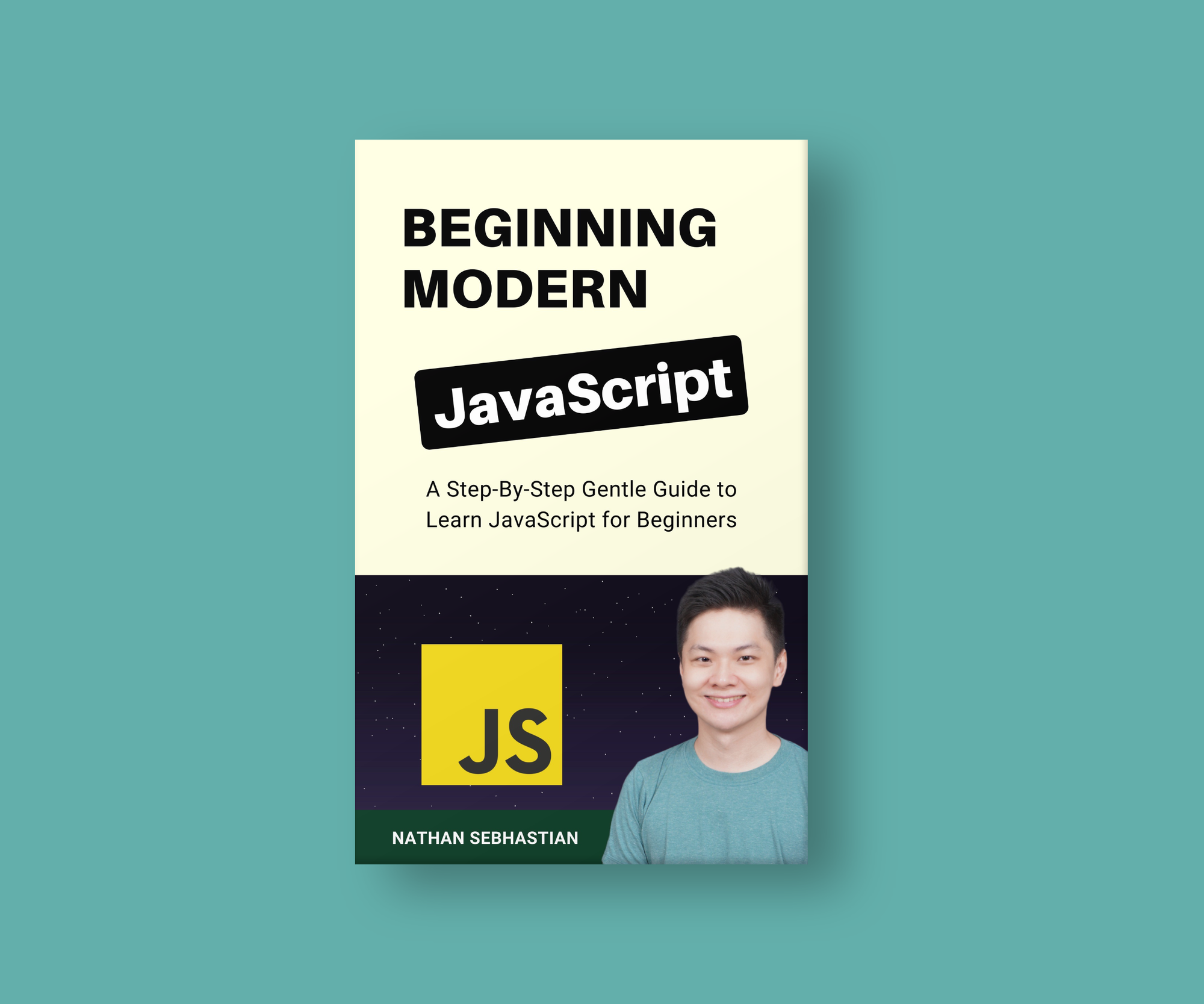
The book is designed to be easy to understand and accessible to anyone looking to learn JavaScript. It provides a step-by-step gentle guide that will help you understand how to use JavaScript to create a dynamic application.
Here's my promise: You will actually feel like you understand what you're doing with JavaScript.
Until next time!
JavaScript Full Stack Developer currently working with fullstack JS using React and Express. Nathan loves to write about his experience in programming to help other people.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
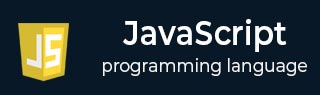
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Garbage Collection
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- ECMAScript 2023
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Generators
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Random Number
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - Export and Import
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Execution Context
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Design Patterns
- JavaScript - Reference Type
- JavaScript - LocalStorage
- JavaScript - SessionStorage
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Common Mistakes
- JavaScript - Performance
- JavaScript - Best Practices
- JavaScript - Style Guide
- JavaScript - Ninja Code
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Resources
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
JavaScript Assignment Operators
The assignment operators in JavaScript are used to assign values to the variables. These are binary operators. An assignment operator takes two operands , assigns a value to the left operand based on the value of right operand. The left operand is always a variable and the right operand may be literal, variable or expression.
An assignment operator first evaluates the expression and then assign the value to the variable (left operand).
A simple assignment operator is equal (=) operator. In the JavaScript statement "let x = 10;", the = operator assigns 10 to the variable x.
We can combine a simple assignment operator with other type of operators such as arithmetic, logical, etc. to get compound assignment operators. Some arithmetic assignment operators are +=, -=, *=, /=, etc. The += operator performs addition operation on the operands and assign the result to the left hand operand.
Arithmetic Assignment Operators
In this section, we will cover simple assignment and arithmetic assignment operators. An arithmetic assignment operator performs arithmetic operation and assign the result to a variable. Following is the list of operators with example −
Simple Assignment (=) Operator
Below is an example of assignment chaining −
Addition Assignment (+=) Operator
The JavaScript addition assignment operator performs addition on the two operands and assigns the result to the left operand. Here addition may be numeric addition or string concatenation.
In the above statement, it adds values of b and x and assigns the result to x.
Example: Numeric Addition Assignment
Example: string concatenation assignment, subtraction assignment (-=) operator.
The subtraction assignment operator in JavaScript subtracts the value of right operand from the left operand and assigns the result to left operand (variable).
In the above statement, it subtracts b from x and assigns the result to x.
Multiplication Assignment (*=) Operator
The multiplication assignment operator in JavaScript multiplies the both operands and assign the result to the left operand.
In the above statement, it multiplies x and b and assigns the result to x.
Division Assignment (/=) Operator
This operator divides left operand by the right operand and assigns the result to left operand.
In the above statement, it divides x by b and assigns the result (quotient) to x.
Remainder Assignment (%=) Operator
The JavaScript remainder assignment operator performs the remainder operation on the operands and assigns the result to left operand.
In the above statement, it divides x by b and assigns the result (remainder) to x.
Exponentiation Assignment (**=) Operator
This operator performs exponentiation operation on the operands and assigns the result to left operand.
In the above statement, it computes x**b and assigns the result to x.
JavaScript Bitwise Assignment operators
A bitwise assignment operator performs bitwise operation on the operands and assign the result to a variable. These operations perform two operations, first a bitwise operation and second the simple assignment operation. Bitwise operation is done on bit-level. A bitwise operator treats both operands as 32-bit signed integers and perform the operation on corresponding bits of the operands. The simple assignment operator assigns result is to the variable (left operand).
Following is the list of operators with example −
Bitwise AND Assignment Operator
The JavaScript bitwise AND assignment (&=) operator performs bitwise AND operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs bitwise AND on x and b and assigns the result to the variable x.
Bitwise OR Assignment Operator
The JavaScript bitwise OR assignment (|=) operator performs bitwise OR operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs bitwise OR on x and b and assigns the result to the variable x.
Bitwise XOR Assignment Operator
The JavaScript bitwise XOR assignment (^=) operator performs bitwise XOR operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs bitwise XOR on x and b and assigns the result to the variable x.
JavaScript Shift Assignment Operators
A shift assignment operator performs bitwise shift operation on the operands and assign the result to a variable (left operand). These are a combinations two operators, the first bitwise shift operator and second the simple assignment operator.
Following is the list of the shift assignment operators with example −
Left Shift Assignment Operator
The JavaScript left shift assignment (<<=) operator performs left shift operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs left shift on x and b and assigns the result to the variable x.
Right Shift Assignment Operator
The JavaScript right shift assignment (>>=) operator performs right shift operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs right shift on x and b and assigns the result to the variable x.
Unsigned Right Shift Assignment Operator
The JavaScript unsigned right shift assignment (>>>=) operator performs unsigned right shift operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs unsigned right shift on x and b and assigns the result to the variable x.
JavaScript Logical Assignment operators
In JavaScript, a logical assignment operator performs a logical operation on the operands and assign the result to a variable (left operand). Each logical assignment operator is a combinations two operators, the first logical operator and second the simple assignment operator.
Following is the list of the logical assignment operators with example −
Firefox is no longer supported on Windows 8.1 and below.
Please download Firefox ESR (Extended Support Release) to use Firefox.
Download Firefox ESR 64-bit
Download Firefox ESR 32-bit
Firefox is no longer supported on macOS 10.14 and below.
Mozilla Foundation Security Advisory 2024-18
Security vulnerabilities fixed in firefox 125.
- Firefox 125
# CVE-2024-3852: GetBoundName in the JIT returned the wrong object
Description.
GetBoundName could return the wrong version of an object when JIT optimizations were applied.
- Bug 1883542
# CVE-2024-3853: Use-after-free if garbage collection runs during realm initialization
A use-after-free could result if a JavaScript realm was in the process of being initialized when a garbage collection started.
- Bug 1884427
# CVE-2024-3854: Out-of-bounds-read after mis-optimized switch statement
In some code patterns the JIT incorrectly optimized switch statements and generated code with out-of-bounds-reads.
- Bug 1884552
# CVE-2024-3855: Incorrect JIT optimization of MSubstr leads to out-of-bounds reads
In certain cases the JIT incorrectly optimized MSubstr operations, which led to out-of-bounds reads.
- Bug 1885828
# CVE-2024-3856: Use-after-free in WASM garbage collection
A use-after-free could occur during WASM execution if garbage collection ran during the creation of an array.
- Bug 1885829
# CVE-2024-3857: Incorrect JITting of arguments led to use-after-free during garbage collection
The JIT created incorrect code for arguments in certain cases. This led to potential use-after-free crashes during garbage collection.
- Bug 1886683
# CVE-2024-3858: Corrupt pointer dereference in js::CheckTracedThing<js::Shape>
It was possible to mutate a JavaScript object so that the JIT could crash while tracing it.
- Bug 1888892
# CVE-2024-3859: Integer-overflow led to out-of-bounds-read in the OpenType sanitizer
On 32-bit versions there were integer-overflows that led to an out-of-bounds-read that potentially could be triggered by a malformed OpenType font.
- Bug 1874489
# CVE-2024-3860: Crash when tracing empty shape lists
An out-of-memory condition during object initialization could result in an empty shape list. If the JIT subsequently traced the object it would crash.
- Bug 1881417
# CVE-2024-3861: Potential use-after-free due to AlignedBuffer self-move
If an AlignedBuffer were assigned to itself, the subsequent self-move could result in an incorrect reference count and later use-after-free.
- Bug 1883158
# CVE-2024-3862: Potential use of uninitialized memory in MarkStack assignment operator on self-assignment
The MarkStack assignment operator, part of the JavaScript engine, could access uninitialized memory if it were used in a self-assignment.
- Bug 1884457
# CVE-2024-3863: Download Protections were bypassed by .xrm-ms files on Windows
The executable file warning was not presented when downloading .xrm-ms files. Note: This issue only affected Windows operating systems. Other operating systems are unaffected.
- Bug 1885855
# CVE-2024-3302: Denial of Service using HTTP/2 CONTINUATION frames
There was no limit to the number of HTTP/2 CONTINUATION frames that would be processed. A server could abuse this to create an Out of Memory condition in the browser.
- Bug 1881183
- VU#421644 - HTTP/2 CONTINUATION frames can be utilized for DoS attacks
# CVE-2024-3864: Memory safety bug fixed in Firefox 125, Firefox ESR 115.10, and Thunderbird 115.10
Memory safety bug present in Firefox 124, Firefox ESR 115.9, and Thunderbird 115.9. This bug showed evidence of memory corruption and we presume that with enough effort this could have been exploited to run arbitrary code.
- Memory safety bug fixed in Firefox 125, Firefox ESR 115.10, and Thunderbird 115.10
# CVE-2024-3865: Memory safety bugs fixed in Firefox 125
Memory safety bugs present in Firefox 124. Some of these bugs showed evidence of memory corruption and we presume that with enough effort some of these could have been exploited to run arbitrary code.
- Memory safety bugs fixed in Firefox 125
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JS Arithmetic Operators
- Addition(+) Arithmetic Operator in JavaScript
- Subtraction(-) Arithmetic Operator in JavaScript
- Multiplication(*) Arithmetic Operator in JavaScript
- Division(/) Arithmetic Operator in JavaScript
- Modulus(%) Arithmetic Operator in JavaScript
- Exponentiation(**) Arithmetic Operator in JavaScript
- Increment(+ +) Arithmetic Operator in JavaScript
- Decrement(--) Arithmetic Operator in JavaScript
- JavaScript Arithmetic Unary Plus(+) Operator
- JavaScript Arithmetic Unary Negation(-) Operator
JS Assignment Operators
- Addition Assignment (+=) Operator in Javascript
- Subtraction Assignment( -=) Operator in Javascript
- Multiplication Assignment(*=) Operator in JavaScript
- Division Assignment(/=) Operator in JavaScript
- JavaScript Remainder Assignment(%=) Operator
- Exponentiation Assignment(**=) Operator in JavaScript
- Left Shift Assignment (<<=) Operator in JavaScript
Right Shift Assignment(>>=) Operator in JavaScript
- Bitwise AND Assignment (&=) Operator in JavaScript
- Bitwise OR Assignment (|=) Operator in JavaScript
- Bitwise XOR Assignment (^=) Operator in JavaScript
- JavaScript Logical AND assignment (&&=) Operator
- JavaScript Logical OR assignment (||=) Operator
- Nullish Coalescing Assignment (??=) Operator in JavaScript
JS Comparison Operators
- Equality(==) Comparison Operator in JavaScript
- Inequality(!=) Comparison Operator in JavaScript
- Strict Equality(===) Comparison Operator in JavaScript
- Strict Inequality(!==) Comparison Operator in JavaScript
- Greater than(>) Comparison Operator in JavaScript
- Greater Than or Equal(>=) Comparison Operator in JavaScript
- Less Than or Equal(
JS Logical Operators
- NOT(!) Logical Operator inJavaScript
- AND(&&) Logical Operator in JavaScript
- OR(||) Logical Operator in JavaScript
JS Bitwise Operators
- AND(&) Bitwise Operator in JavaScript
- OR(|) Bitwise Operator in JavaScript
- XOR(^) Bitwise Operator in JavaScript
- NOT(~) Bitwise Operator in JavaScript
- Left Shift (
- Right Shift (>>) Bitwise Operator in JavaScript
- Zero Fill Right Shift (>>>) Bitwise Operator in JavaScript
JS Unary Operators
- JavaScript typeof Operator
- JavaScript delete Operator
JS Relational Operators
- JavaScript in Operator
- JavaScript Instanceof Operator
JS Other Operators
- JavaScript String Operators
- JavaScript yield Operator
- JavaScript Pipeline Operator
- JavaScript Grouping Operator
The Right Shift Assignment Operator is represented by “>>=”. This operator shifts the first operand to the right and assigns the result to the variable. It can also be explained as shifting the first operand to the right in a specified amount of bits which is the second operand integer and then assigning the result to the first operand.
Where –
- a is the first operand, and
- b is the second operand.
Example 1: In this example, we will see the implementation of the right shift assignment.
Example 2: In this example, we will see assigning the right shift operator to the variable.
We have a complete list of Javascript Assignment Operators, Please check this article Javascript Assignment Operator .
Supported Browser:
Please Login to comment...
Similar reads.
- Web Technologies
- Otter.ai vs. Fireflies.ai: Which AI Transcribes Meetings More Accurately?
- Google Chrome Will Soon Let You Talk to Gemini In The Address Bar
- AI Interior Designer vs. Virtual Home Decorator: Which AI Can Transform Your Home Into a Pinterest Dream Faster?
- Top 10 Free Webclipper on Chrome Browser in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
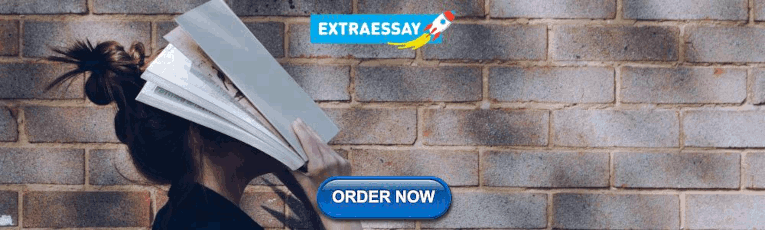
IMAGES
VIDEO
COMMENTS
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
Division Assignment Operator (/=) The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable. Example: Javascript. let yoo = 10; const moo = 2; // Expected output 5 console.log(yoo = yoo / moo); // Expected output Infinity console.log(yoo /= 0); Output:
Javascript operators are used to perform different types of mathematical and logical computations. Examples: The Assignment Operator = assigns values. The Addition Operator + adds values. ... The Addition Assignment Operator (+=) adds a value to a variable. Assignment. let x = 10; x += 5;
An assignment operator ( =) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Here is the list of all assignment operators in JavaScript: In the following table if variable a is not defined then assume it to be 10. Operator Description Example Equivalent to = Assignment operator: a = 10: a = 10 += Addition assignment operator: a += 10: a = a + 10-= Subtraction assignment operator: a -= 10: a = a - 10 *=
JavaScript Assignment Operators. We use assignment operators to assign values to variables. For example, let x = 5; Here, we used the = operator to assign the value 5 to variable x. Commonly Used Assignment Operators. Operator Name Example = Assignment Operator: a = 7; += Addition Assignment:
This is because the assignment operator returns the value that is assigned. First, b is set to 5. Then the a is also set to 5 — the return value of b = 5, a.k.a. right operand of the assignment. As another example, the unique exponentiation operator has right-associativity, whereas other arithmetic operators have left-associativity.
The boolean operators in JavaScript can return an operand, and not always a boolean result as in other languages. The Logical OR operator ( ||) returns the value of its second operand, if the first one is falsy, otherwise the value of the first operand is returned. For example: "foo" || "bar"; // returns "foo".
The logical OR assignment operator ( ||=) accepts two operands and assigns the right operand to the left operand if the left operand is falsy: In this syntax, the ||= operator only assigns y to x if x is falsy. For example: console .log(title); Code language: JavaScript (javascript) Output: In this example, the title variable is undefined ...
An operator is a special character or series of characters that perform a task in JavaScript. Assignment Operator. This operator uses the equals sign (=) to assign a value to a variable. let x = 42; In the snippet above, a variable x is declared and the numeric value 42 is assigned to it.
The second group of operators we're going to explore is the assignment operators. Assignment operators are used to assign a specific value to a variable. The basic assignment operator is marked by the equal = symbol, and you've already seen this operator in action before: let x = 5; After the basic assignment operator, there are 5 more ...
The assignment operators in JavaScript are used to assign values to the variables. These are binary operators. An assignment operator takes two operands, assigns a value to the left operand based on the value of right operand. The left operand is always a variable and the right operand may be literal, variable or expression.
The JavaScript Assignment operators are used to assign values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: var i = 10; The below table displays all the JavaScript assignment operators. JavaScript Assignment Operators. Example. Explanation. =.
Basic keywords and general expressions in JavaScript. These expressions have the highest precedence (higher than operators ). The this keyword refers to a special property of an execution context. Basic null, boolean, number, and string literals. Array initializer/literal syntax. Object initializer/literal syntax.
The sign = denotes the simple assignment operator. JavaScript also has several compound assignment operators, which is actually shorthand for other operators. A list of all such operators are listed below. Table of Contents. List of Assignment operators; Simple Assignement Operator; Compound Assignment Operators;
This operator is used to perform a bitwise OR operation on both operands and assign the result to the left operands. Syntax: a |= b. Where -. a = First operand. b = Second operand. Example 1: In this example, we will use basic numeric values to perform Bitwise OR Assignment Operation. Javascript.
Description. Logical AND assignment short-circuits, meaning that x &&= y is equivalent to x && (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not truthy, due to short-circuiting of the logical AND operator. For example, the following does not throw an error, despite x being ...
The MarkStack assignment operator, part of the JavaScript engine, could access uninitialized memory if it were used in a self-assignment. References. Bug 1884457 # CVE-2024-3863: Download Protections were bypassed by .xrm-ms files on Windows Reporter Eduardo Braun Prado working with Trend Micro Zero Day Initiative
The Right Shift Assignment Operator is represented by ">>=". This operator shifts the first operand to the right and assigns the result to the variable. It can also be explained as shifting the first operand to the right in a specified amount of bits which is the second operand integer and then assigning the result to the first operand. Syntax: