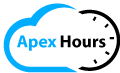
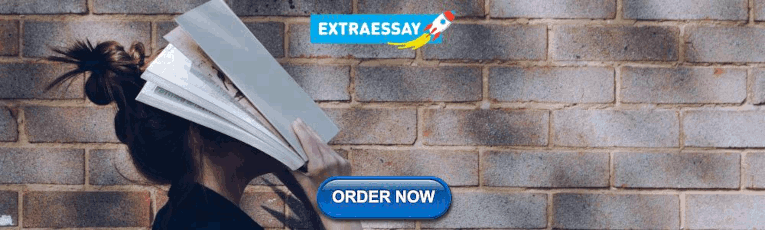
Insert/edit link
Enter the destination URL
Or link to existing content
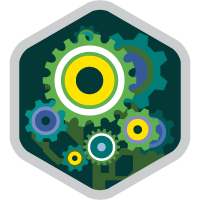
Apex Specialist
Use integration and business logic to push your Apex coding skills to the limit.
Prerequisites
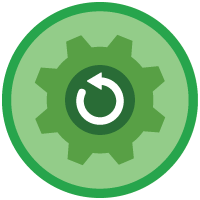
What You'll Be Doing to Earn This Superbadge
- Automate record creation using Apex triggers
- Synchronize Salesforce data with an external system using asynchronous REST callouts
- Schedule synchronization using Apex code
- Test automation logic to confirm Apex trigger side effects
- Test integration logic using callout mocks
- Test scheduling logic to confirm action gets queued
Concepts Tested in This Superbadge
- Apex Triggers
- Asynchronous Apex
- Apex Integration
- Apex Testing
Grab a pen and paper. You may want to jot down notes as you read the requirements.
Refer to the Apex Specialist Superbadge: Trailhead Challenge Help document for detailed resources and documentation.
Use the naming conventions specified in the requirements document to ensure a successful deployment.
Review the data schema in your modified Salesforce org as you read the detailed requirements below.
Set Up Development Org
Create a new Trailhead Playground or Developer Edition Org for this superbadge. Using this org for any other reason might create problems when validating the challenge.
Install this unlocked package (package ID: 04t6g000008av9iAAA). This package contains metadata you'll use to complete this challenge. If you have trouble installing this package, follow the steps in the Install a Package or App to Complete a Trailhead Challenge help article.
Add picklist values Repair and Routine Maintenance to the Type field on the Case object.
Update the Case page layout assignment to use the Case (HowWeRoll) Layout for your profile.
Rename the tab/label for the Case tab to Maintenance Request .
Update the Product page layout assignment to use the Product (HowWeRoll) Layout for your profile.
Rename the tab/label for the Product object to Equipment .
Use App Launcher to navigate to the Create Default Data tab of the How We Roll Maintenance app. Click Create Data to generate sample data for the application.
Review the newly created records to get acquainted with the data model.
Before you begin the challenges, review the help article for this superbadge . This document will help you find useful resources to complete this superbadge and assist with frequently asked questions.
Review the help article relating to superbadge challenges for more information about credential security.
There are two kinds of people in this world: those who would travel in a recreational vehicle (RV) and those who wouldn’t. The RV community is increasing exponentially across the globe. Over the past few years, HowWeRoll Rentals, the world’s largest RV rental company, has increased its global footprint and camper fleet tenfold. HowWeRoll offers travelers superior RV rental and roadside assistance services. Their tagline is, “We have great service, because that’s How We Roll!” Their rental fleet includes every style of camper vehicle, from extra large, luxurious homes on wheels to bare bones, retro Winnebagos.
You have been hired as the lead Salesforce developer to automate and scale HowWeRoll’s reach. For travelers, not every journey goes according to plan. Unfortunately, there’s bound to be a bump in the road at some point along the way. Thankfully, HowWeRoll has an amazing RV repair squad who can attend to any maintenance request, no matter where you are. These repairs address a variety of technical difficulties, from a broken axle to a clogged septic tank.
As the company grows, so does HowWeRoll’s rental fleet. While it’s great for business, the current service and maintenance process is challenging to scale. In addition to service requests for broken or malfunctioning equipment, routine maintenance requests for vehicles have grown exponentially. Without routine maintenance checks, the rental fleet is susceptible to avoidable breakdowns.
That’s where you come in! HowWeRoll needs you to automate their Salesforce-based routine maintenance system. You’ll ensure that anything that might cause unnecessary damage to the vehicle, or worse, endanger the customer is flagged. You’ll also integrate Salesforce with HowWeRoll’s back-office system that keeps track of warehouse inventory. This completely separate system needs to sync on a regular basis with Salesforce. Synchronization ensures that HowWeRoll’s headquarters (HQ) knows exactly how much equipment is available when making a maintenance request, and alerts them when they need to order more equipment.
Standard Objects
You’ll be working with the following standard objects:
- Maintenance Request (renamed Case) — Service requests for broken vehicles, malfunctions, and routine maintenance.
- Equipment (renamed Product) — Parts and items in the warehouse used to fix or maintain RVs.
Custom Objects
- Vehicle — Vehicles in HowWeRoll’s rental fleet.
- Equipment Maintenance Item — Joins an Equipment record with a Maintenance Request record, indicating the equipment needed for the maintenance request.
Entity Diagram

This section represents the culmination of your meetings with key HowWeRoll stakeholders. It’s your blueprint to programmatically automate the support and maintenance side of their business.
Automate Maintenance Requests
With the exponential increase in RV popularity worldwide, HowWeRoll is supplying hundreds more luxury and economy vehicles around the globe. Along with this increase in their rental stock comes an inevitable increase in equipment failure. HowWeRoll has an existing process to handle these failures, but they want you to build an automation for their routine maintenance. You’ll build a programmatic process that automatically schedules regular checkups on the equipment based on the date that the equipment was installed.
Use the help article for this superbadge to reference the API names of the objects and fields. This accelerates your development process by not going back and forth between Setup and your development tool.
When an existing maintenance request of type Repair or Routine Maintenance is closed, create a new maintenance request for a future routine checkup. This new maintenance request is tied to the same Vehicle and Equipment Records as the original closed request. For record keeping purposes the existing equipment maintenance items must remain tied to the original close request so new records must be created. This new request's Type should be set as Routine Maintenance. The Subject should not be null and the Report Date field reflects the day the request was created. Remember, all equipment has maintenance cycles.
Calculate the maintenance request due dates by using the maintenance cycle defined on the related equipment records. If multiple pieces of equipment are used in the maintenance request, define the due date by applying the shortest maintenance cycle to today’s date.
Design the code to work for both single and bulk maintenance requests. Bulkify the system to successfully process approximately 300 records of offline maintenance requests that are scheduled to import together. For now, don’t worry about changes that occur on the equipment record itself.
Also expose the logic for other uses in the org. Separate the trigger (named MaintenanceRequest ) from the application logic in the handler (named MaintenanceRequestHelper ). This setup makes it simpler to delegate actions and extend the app in the future.
Synchronize Inventory Management
In addition to equipment maintenance, design HowWeRoll’s inventory data synchronization with the external system in the equipment warehouse. Although the entire HQ office uses Salesforce, the warehouse team still works on a separate legacy system. With this integration, the inventory in Salesforce updates after the equipment is taken from the warehouse to service a vehicle.
Write a class that makes a REST callout to an external warehouse system to get a list of equipment that needs to be updated. The callout’s JSON response returns the equipment records that you upsert in Salesforce. Beyond inventory, ensure that other potential warehouse changes carry over to Salesforce. Your class maps the following fields:
Replacement part (always true )
Current inventory
Maintenance cycle
Warehouse SKU
Use the warehouse SKU as the external ID to identify which equipment records to update within Salesforce.
Although HowWeRoll is an international company, the remote offices follow the lead of the HQ’s work schedule. Therefore, all maintenance requests are processed during HQ’s normal business hours. You need to update Salesforce data during off hours (at 1:00 AM). This logic runs daily so that the inventory is up to date every morning at HQ.
Create Unit Tests
Test your code to ensure that it executes correctly before deploying it to production.
First, test the trigger to ensure that it works as expected. Follow best practices by testing for both positive use cases (when the trigger needs to fire) and negative use cases (when the trigger must not fire). Of course, passing a test doesn’t necessarily mean you got everything correct. So add assertions into your code to ensure you don’t get false positives. For your positive test, assert that everything was created correctly, including the relationships to the vehicle and equipment, as well as the due date. For your negative test, assert that no work orders were created.
As mentioned previously, the huge wave of maintenance requests could potentially be loaded at once. The number will probably be around 200, but to account for potential spikes, pad your class to successfully handle at least 300 records. To test this, include a positive use case for 300 maintenance requests and assert that your test ran as expected.
When you have 100% code coverage on your trigger and handler, write test cases for your callout and scheduled Apex classes. You need to have 100% code coverage for all Apex in your org.
Ensure that your code operates as expected in the scheduled context by validating that it executes after Test.stopTest() without exception. Also assert that a scheduled asynchronous job is in the queue. The test classes for the callout service and scheduled test must also have 100% test coverage.
Superbadge Complete!
+ 13000 points, ready to tackle this superbadge.
Please first complete the prerequisites and the challenge for Apex Specialist will be unlocked.
Product Area
Feature impact.
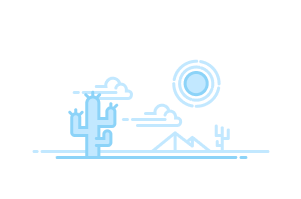
- Put Employees First with Work.com
- Standard Objects in Work.com Data Model
- Employee-User Sync for Orgs Without Existing Employee Data
- Employee-User Sync for Orgs with Existing Employee Data
- Cascade Employee Updates to Users
- Provision Users for Large Amounts of Employee Data
- Employee User Sync Considerations
- Enable Digital Experiences for Employee Workspace
- Brand Your Org
- Install the Employee Workspace Package
- Verify Employee Workspace Installation and Auto-Configuration
- Configure Field Access Settings on the Employee Object
- Assign Employee Workspace User Permission Sets
- Add Employee Workspace Admins
- Create and Link Employees and Users
- Considerations for Employee Workspace Customizations
- Activate Your Employee Workspace Site
- Add Connected Apps
- Publish Your Employee Workspace Site
- Customize the News Banner
- Customize the Hero Component
- Add Search to Your Employee Workspace Page
- Customize the Navigation Bar
- Add Default Apps to the Pinned App Widget
- Create Your Branded App for Employee Workspace
- Log in to Employee Workspace
- Collaborate With Your Peers
- Take Your Wellness Survey
- Manage Your Workplace Shifts
- Get to Know Your Colleagues
- Share Your Work Experiences
- Access Apps You Need to be Productive
- Search for Knowledge in Your Employee Workspace
- View and Manage Your Tickets
- Navigate Agent Desk
- Manage and Resolve Tickets with Agent Desk
- Create Tickets in Agent Desk
- Create and Manage Knowledge Articles for Employee Concierge
- Agent Desk Considerations
- Configure Employee Workspace and Enable Person Accounts
- Install the Employee Concierge Package
- Verify the Employee Concierge Package Installation Customizations
- Enable Omni-Channel Routing for Employee Concierge
- Meet the Employee Concierge Personas
- Review the Employee Concierge Permissions
- Assign Employee Concierge Permissions
- Assign Record Types to the System Admin Profile
- Assign Record Types to the IT Agent Profile
- Enable Knowledge Access for Knowledge Authors
- Add Custom Fields to Knowledge Page Layout
- Make Custom Fields for Knowledge Articles Visible
- Assign Users to the IT Service Agents Public Group
- Create Queues for Ticket Assignment
- Specify Default Ticket Assignments
- Create Agent Desk Assignment Rules
- Set Assignment Rules as the Default for New Tickets
- Create a Sharing Rule for Tickets
- Edit Agent Desk Utility Items
Assign the IT Case Page Layout
- Activate and Assign the IT Case Record Layout
- Enable the IT Case Path
- Share the Tier 1 Cases List View with Tier 1 Public Groups
- Create Person Accounts for Employee Records
- Employee to Person Account Field Mapping
- Add Ticket Category Picklist Values
- Add a Quick Action to the Contact Object
- Add the Custom Metadata Type for the New Ticket Category
- Associate Knowledge Article Record Types and Ticket Categories
- Set Your IT Case Page Layout for Email-to-Case Enablement
- Customize Your Email-to-Case Address Routing Settings
- Set Your Email-to-Case Support Settings
- Email-to-Case Considerations and Limitations for Employee Concierge
- What Is the Employee Service Site?
- Understanding the Employee Service Site Experience
- Employee Service Template and Custom Components
- Employee Workspace Template and Custom Components
- Customer Service Template and Custom Components
- Set Your Administration Settings
- Verify Your Site Pages and Components
- Add Service Catalog Pages and Components
- Add a My Support Tickets page
- Add the Concierge New Ticket Form
- Add a Search Page
- Add a Knowledge Page
- Add a Home Page
- Add Components to Your Home Page
- Configure Your Navigation Bar
- Add an Attachments Component to the My Support Tickets Page
- Update the IT Case Page Layout to Use Attachments
- Publish Your Site
- Customize the Ticket Details Field
- Share Resources with Employee Concierge Users
- Create a Custom Process for Manage Support Cases
- Get Started with Employee Concierge Bot
- Install the Employee Concierge Bot Unmanaged Package
- Deploy Chat Functions
- Set Up Omni-Channel Routing
- Add the Concierge Bot Chat Skill
- Add a Concierge Bot Chat Button
- Create an Embedded Service Deployment
- Customize the Pre-Chat Form
- Assign and Verify Employee Concierge Bot Permission Sets
- Add a Custom Employee Concierge Bot User Profile
- Add Branding to Employee Concierge Bot
- Deploy Employee Concierge Bot to the Chat Channel
- Select a Security Level for Your Community
- Add Concierge Bot to Your Community Template
- IT Service Center
- Install the Packages
- Verify Your Installation
- Meet the HR Service Center Personas
- Assign HR Service Center Permissions
- Add HR Service Center Admins
- Protect Access to Sensitive HR Data
- Protect Employee Tasks with a Custom Restriction Rule and Permission
- Assign Record Types to the HR Service Agent Profile
- Remove or Disable Existing HR Knowledge Articles
- Set Share Settings
- Add HR Service Agents to Groups
- Create Assignment Rules
- Set Assignment Rules as the Default for New HR Tickets
- Enable or Disable Email Notifications
- Confirm the Role Hierarchy
- Create Sharing Rules for Tickets (Optional)
- Configure the [Sample] Health Verification Flow with the...
- Share an HR Case List View with the Relevant Public Group
- Manage Person Accounts and Employee Records
- Create Ticket Categories (Optional)
- Prepare Your Team and Customize HR Service Center
- Publish Knowledge Articles for Employees
- Connect HR Service Center to Workday
- Sample HR Service Center Flows
- Link to Relevant Flows Directly from Knowledge Articles
- Add the Flow Launch Button to the Knowledge Article Layout
- Activate the Case Lightning Page
- Customize HR Service Center Service Catalog Item Samples
- Service Catalog Case Elements
- Configure HR Service Center Data Collection
- Setup Overview
- Considerations and Recommendations
- Assign HR Agents the Transition Task Record Type for the Task Object
- Configure the Employee Page Layout to Support Employee Transitions
- Configure the Transition Plan Page Layout to Support Employee...
- Configure the Transition Plan Template Layout to Support Employee...
- Configure the Transition Plan Template Tasks Layout to Support...
- Configure the HR Service Center Custom Settings
- Add Onboarding Tasks to the Employee Record Page
- Create Your Transition Plan Templates
- Create Your Transition Plan Tasks
- Join Your Transition Plan Tasks and Template
- Assign a Transition Plan
- Add the Employee and Manager Transitions Banner to the Home Page
- Add the Manager Transition Plans Page to the Employee Service...
- Add the Employee Transition Plans Page to Employee Service Community
- Configure an Experience Theme Layout for Your Employee Transitions...
- Install Employee Service Catalog
- Service Catalog Permissions
- Create Your Service Catalog
- Create a Catalog Category
- Edit a Catalog Category
- Delete a Catalog Category
- Create a Catalog Item
- Edit a Catalog Item
- Clone a Catalog Item
- Delete a Catalog Item
- Mark a Catalog Item as Featured
- Fulfillment Flows for Catalog Items
- Create a Custom Fulfillment Flow
- Edit a Custom Fulfillment Flow
- Clone a Custom Fulfillment Flow
- Delete a Custom Fulfillment Flow
- Test a Fulfillment Flow
- Retrieve Catalog Item Metadata
- Published and Deprecated Catalog Items
- Catalog Requests
- Org Prerequisites to Install the Sample Service Catalog Items Package
- Install the Sample Service Catalog Items Package
- Grant Users Access to the Sample Service Catalog Items Package
- Configure the Sample Service Catalog Items Package for the User UI
- Set Employee Field Visibility Rules
- Use Default Employee Field Visibility Settings
- Edit the Default Employee Field Visibility Settings
- Create Custom Employee Field Visibility Rules
- Configure Employee Field Visibility with the API
- Employee Field Visibility Considerations and Limitations
- Restrict the Case Feed Access for the Standard Platform User in the...
- Configure Feed Tracking for a Standard Platform User
- Remove Salesforce Chatter Accessibility for Service Agent Users
- Create Conditional Visibility Rules for the Case Feed Component
- Create a Separate Service Agent User for Each Employee User
- Modify Your Existing Users to Make Them Employees Only and Create...
- Brand the Org
- Enable Translation Workbench
- Enable Digital Experiences for Workplace Command Center
- Install the Workplace Command Center and Wellness Check Packages
- Verify Command Center and Wellness Check Installation and...
- Meet the Workplace Command Center Personas
- Add Workplace Operations and Executive Users
- Add Workplace Command Center Admins
- Set Sharing Rules for Workplace Personas
- Workplace Command Center Permission Sets
- Workplace Command Center Permission Set Groups
- Customize Workplace Command Center Permissions
- Clone the Command Center
- Enable Location Search Filtering at All Levels (Optional)
- Make Tasks Tabs Available in User Profiles
- Configure the COVID-19 Tracker Component
- Create Flows for Tasks in the Operations Feed
- Task Record Sharing in Workplace Command Center
- Add Run Flows Permissions
- Activate Wellness Check Experience Cloud Site
- Configure Wellness Check Survey Mappings
- Configure a Wellness Check Survey Reply-To Name and Email Address
- Configure Internal Users for Wellness Check Survey Authentication
- Configure External Users for Wellness Check Survey Authentication
- Manage Wellness Check Survey Translations
- Activate Wellness Check Surveys
- Add the My Wellness Component to an Experience Cloud Site
- Populate the Location Hierarchy and Recalculate Wellness Fact Records
- Create and Import Location and Address Records
- Create and Import Employee Records
- Create, Import, or Link User Records
- Understand and Disable Workplace Command Center Triggers
- Convert Legacy Employee Data to Use Full Name
- Configure Logging
- View Log Messages
- Upgrade to Workplace Command Center Package Version 3
- Update Permissions from Command Center Settings
- Add Custom Fields to the Tasks Layout
- Add the Survey Schedule History Related List to Survey Schedules
- Workplace Command Center Limitations and Allocations
- Wellness Check
- Wellness Check Survey Design Considerations
- How Do Wellness Check Survey Responses Map to the Data Model?
- Modify Wellness Check Surveys
- Modify or Create Email Templates in Salesforce Classic
- Modify or Create Email Templates in Lightning Experience
- Send Wellness Check Surveys to Employees
- Send Wellness Check Surveys to Locations
- Edit Wellness Check Survey Schedules
- Review Wellness Check Survey History
- Deactivate or Activate Wellness Check Survey Schedules
- Respond to Surveys
- View Wellness Data in the Command Center
- View Wellness Data in Employee Records
- Modify Survey Process Settings
- How Workplace Command Center Helps You
- Reduce Batch Size to Support Large-Scale Direct Child Locations
- View the Status of Locations and Sublocations
- What Is a Location Status?
- View Workplaces on a Map or in a List
- Check the Health of Your Workforce
- See Employee Availability by Location
- See Shift Metrics and Planned Occupancy at a Glance
- Create Tasks Manually in the Operations Feed
- Manage Tasks in the Operations Feed
- Filter Tasks in the Operations Feed
- See COVID-19 Information from Around the World
- Invite Employees Back to Work
- Get to Know Shift Management Personas
- Shift Management Considerations
- How Does Shift Scheduling Work?
- Enable Field Service
- Install the Field Service Managed Package
- Customize Field Service Settings
- Install the Shift Management Managed Package
- Configure Shift Management for Work.com
- Complete the Recommended Setup
- Create Employee Logins for the Shift Management App
- Update an Existing Shift Management Org
- Plan for Reduced Occupancy
- Create Facility Plans
- Create Open Shifts and Notify Your Team
- Schedule Shifts for Your Team
- Log in to the Shift Management Mobile App
- Schedule Shifts in the Mobile App
- Overview of Workplace Strategy Planner
- Components in Workplace Strategy Planner
- Workplace Strategy Planner Considerations
- Enable CRM Analytics
- Install the Strategy Planner Package
- Assign Licenses and Permissions for Workplace Strategy Planner
- Verify Workplace Strategy Planner Installation
- Create a New COVID-19 Risk App from a Template
- Schedule Dataflow Updates
- Add the COVID-19 Dashboard to Strategy Planner Home
- Add the COVID-19 Risk Component to Location Records
- Share the COVID-19 Risk App
- Confirm or Add Required Data
- Confirm Wellness Check Survey Data
- Prepare Your Team
- Prepare for Package and Template Updates
- Troubleshoot Strategy Planner Dashboard Data
- Understand Workplace Strategy Planner Criteria, Metrics, and...
- Customize Threshold Values
- Add Location Assessment Data
- How Risk Levels Are Calculated
- Read, Customize, and Share Dashboards
- Enablement Site (myTrailhead) for Learning and Wellness
- Contact Tracing for Employees
- Activate Digital Trust Cards
- Customize the Location Type Field on the Location Object
- Create a Location Trust Measure
- Preview Your Digital Trust Card
- Add Custom Colors and Fonts to Your Digital Trust Card
- Customize Your Apex Class during Digital Trust Cards Setup
- Customize Your Digital Trust Cards' Metadata
- Hide the iFrame While You Onboard Trust Card Managers
- Customize Your Trust Cards’ Query
- Create a Trust Cards Manager User Profile (from the Identity User...
- Add a New User with the Trust Cards Manager User Profile
- Assign the Trust Card Manager Permission Set to a User
- Assign a Digital Trust Card Location to a Trust Card Manager
- Define Your Trust Cards Strategy as a Retail Store Manager
- Define Your Digital Trust Cards Strategy as a Hotel Manager
- Define Your Digital Trust Cards Strategy as an Entertainment Venue...
- Get to Know the Queue Management Roles
- Create a Queue Management Permission Set
- Set Sharing Rules for Queue Management Personas
- Add Facility Admins
- Set Up Broadcast Messaging
- Add Queues to Locations
- Create an Experience Cloud Site Page
- Enable Security Code Messages for Queued Parties
- Build a Customized Experience Cloud Site
- Relate an Experience Cloud Site Page to Your Location
- Get the Site Key and Secret Key from ReCaptcha
- Add Markup and ReCaptcha Keys to Your Sign-Up Form
- Relax Security Settings and Add ReCaptcha
- Best Practices for Queue Managers
- Broadcast Messaging in Work.com
The IT Case page layout and compact page layout are added to your org when you install Employee Concierge. The compact page layout is also assigned to IT records during installation. Assign the page layout to all IT record types and related profiles to have it appear in your org.
- From service Setup, click Object Manager .
- Click Case , then Case Page Layouts .
- Click Page Layout Assignment .
- Click Edit Assignment .
- Locate the IT column and select the entire column.
- For Page Layout to Use, select IT .
- Save your changes.
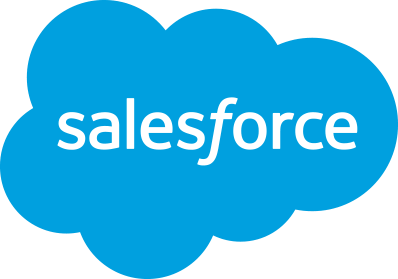
Cookie Consent Manager
General information, required cookies, functional cookies, advertising cookies.
We use three kinds of cookies on our websites: required, functional, and advertising. You can choose whether functional and advertising cookies apply. Click on the different cookie categories to find out more about each category and to change the default settings. Privacy Statement
Required cookies are necessary for basic website functionality. Some examples include: session cookies needed to transmit the website, authentication cookies, and security cookies.
Functional cookies enhance functions, performance, and services on the website. Some examples include: cookies used to analyze site traffic, cookies used for market research, and cookies used to display advertising that is not directed to a particular individual.
Advertising cookies track activity across websites in order to understand a viewer’s interests, and direct them specific marketing. Some examples include: cookies used for remarketing, or interest-based advertising.
Cookie List
- Things To Know Before Getting Started
- Creating Orgs, Connecting to Flosum and Adding Users
- Pulling Changes from Your Development Sandbox
- Version Control & Merging Changes
- Deploying Changes To Your Target Org
- Scheduling Deployment
- Rollbacks: Getting Started
- Comparing Orgs
Case Studies
Implementation guide.
- Request Flosum Upgrade
- Set the From Address for Flosum Emails
- Installing Flosum
- Creating a Connected App
- Setting up Flosum Integration User
- Connecting sandboxes to Flosum
- For Government Cloud Customers
- Setting up users in Flosum
- Security Model in Flosum
- Initializing a Repository
- Setting up workflow permissions
- Pulling changes from dev sandboxes
- Branching Strategy
- Conflict and Merge strategy
- Peer Reviews
- Static Code Analysis
- Regression Testing
- Reference Architecture
- Post-install Steps
- Purging snapshots in Flosum
- Compliance & Governance
- Salesforce DX: Scratch Org Creation
- CI/CD & Pipelines
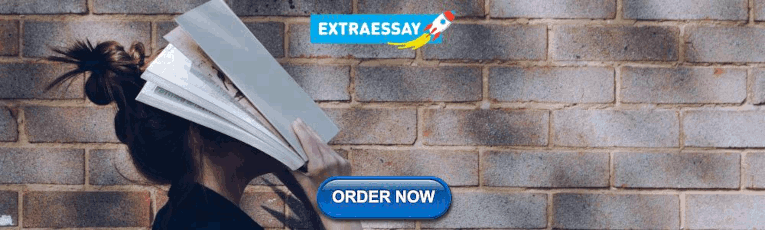
Architecture
- Flosum DevOps Architecture, Agents, and More
- Branch Updates in Flosum DevOps
- Deployment Dashboard
- Pipeline Operations
- Deployment Manager
- Domain Management
- Flosum-Slack Webhook
- Connecting Orgs to Flosum DevOps Using JWT Authentication
- Demerge Branch
- Multi-Domain Support for Multi-Git Integrations
- Multi Branch Merge
- Source Member Setup and Configuration
- Deploying Installed Packages
- Retrieve Omnistudio Components with Snapshots
- Profile Retrieval on the Branch
- How to Abort a Running Apex Job
- Snapshot From Unlocked Package
- Snapshot Detail
- Flosum Dependency Identifier
- Process Approval Validations
- Branch Permissions Functionality
- Branches Tab
- Multi-file components edit
- Lightning Test Service
- Define Destructive changes in Branches in Flosum
- New Editor in Snapshot Comparison Screen
- Profile Comparison
- Create and Commit a Branch
- Destructive Changes
- Deployment History
- Overwrite Protection Feature
- Pre-Deployment Fix
- Backsynch Process
- Advantages of Deployments
- Custom Validation Logic Before Deployments
- Test Execution During Deployments
- Scheduling Deployments
- Delete a Repository
- Archive a Branch
- Mass Delete Branches
- Download Components on Repository
- Committing Changes to the Repository
- Export Audit Trail Changes Recorded in Org
- Version Control Overview
- Getting Started with Version Control
- Audit Trail
- Maintain Settings After Org Refresh
- Keeping Orgs in Sync with Repository Without a Refresh
- Settings Tab
- Org Details
- Org Permissions
- Salesforce Flosum Permission Sets
- Data Masking
- Orgs Tab Snapshot Comparison
- Understanding Flosum Metrics
- Setting Up Flosum Metrics
- Kanban Board (Swim Lanes)
- Reports and Dashboards
- Branch State Diagram
- Getting Started with Vlocity in Flosum
- Supported Vlocity Components
- Vlocity Components
- Snapshot – Vlocity
Integrations
- Flosum-Provar Integration
- Heroku - Git Agent Integration
- Bitbucket on Cloud Environment Variables
- Flosum for the Git Developer
- Flosum & Visual Studio
- B2C Commerce Cloud Integration (Beta)
- Marketing Cloud Integration (Beta)
- Flosum Scan Integration
- Veeva Vault Integration
- GitLab on Cloud Environment Variables
- Hyperforce and Flosum
- Flosum Agent Architecture
- Agile Accelerator Integration
- Support for AccelQ Regression Testing Tool
- ServiceNow Integration
- Flosum & IntelliJ IDEA
- Unlocked Packages
- Flosum to VersionOne Integration
- How To Integrate Flosum With CodeScan
- Source Code Analysis - Apex PMD (Code Quality)
- Flosum & Jira Cloud
- Installing a TFS/Azure DevOps Connector
- Azure on Cloud Environment Variables
- Azure DevOps Pipeline Webhook
- Flosum’s Azure DevOps Story Integration
- Jira Webhooks to Flosum
- JIRA & PMD Upgrade
- Jira Webhooks Use Case
- Flosum’s On-Premise Jira Integration
- Email Notifications for Git Integration
- Requirements for Naming Branches and Repositories
- GitHub on Cloud Environment Variables
- Flosum – Git Version Control
- Source Member Tracking
- Deploy Community Components Using the Experience Bundle Metadata Type
- Partial Permission Set Deployment
- Demystifying Snapshot Retrieval Process In Flosum
- Demystifying Community Deployments Salesforce
- Understanding Salesforce Flow Deployment
- Demystifying Standard Component Deployments
- Demystifying Picklist Deployments
- Demystifying Profile Deployments
- Demystifying Lightning Pages And Components In Flosum
- Track Pending or Old Deployments
- Demystifying Record Type Deployments
- Demystifying Page/Search/Compact Layouts Deployments in Flosum
- Demystifying Translations Deployment In Flosum
- How to Retrieve Custom Metadata and Its Records in Flosum
- Deploying Custom Label Translations
- Deploying email-to-case via Flosum
- Demystifying Process Builder Deployments
- GlobalValueSet changes not being picked up
- ListView deployment failing
- Deploying Custom Metadata Records Using Flosum
- Including Reports and Email Templates in Snapshots
- Demystifying Einstein Components in Flosum
- Demystifying Deploying Public Groups
- Utilizing Flosum Agent for Metadata Retrieval
- Field History Tracking deployment
- Environment Variables
- Metadata API 52 Upgrade
- Metadata API 48 Upgrade
- Demystifying CustomObject Deployment In Flosum
- Demystifying Button, Links and Actions in Flosum
- Deploying FlexiPage Assignments
- Metadata Types retrievable only via zero day snapshot
- Metadata Component Types Supported by Flosum
Best Practice
- Sandbox Environments in ALM
- Code Coverage Check
- Purge Routine
- Optimizing your deployment
- Reducing Deployment Time With Flosum
- Improving your Governance Model
- Flosum-Git Folder Structure Rules
- Enabling MyDomain on your Flosum Org
- Updating your Callback URL
- Flosum's Retrieval & Deployment Webinar
- How to Grant Access to Flosum Support
Release Notes
- Flosum Updated Terminology - Winter 2021 Release
- Flosum DevOps 3.227 Release Notes
- Flosum DevOps 3.228 Release Notes
- Flosum DBR 3.2.0 Release Notes
- Flosum DevOps 3.226 Release Notes
- Flosum Spring '21 Release Notes
- Flosum Summer '21 Release Notes
- Flosum DevOps 3.222 Release Notes
- Flosum DevOps 3.225 Release Notes
- Flosum DevOps 3.223 Release Notes
- Flosum Spring '24 Release Notes
- Flosum Spring '24 Data Migrator Release Notes
- Flosum Winter '22 Data Migrator Release Notes
- Flosum Winter '23 Release Notes
- Flosum Winter '24 Release Notes
- Flosum Winter '21 Release Notes
- Flosum Summer '23 Release Notes
- Metadata API Version 45 Upgrade
- Flosum Summer '20 Release Notes
- Flosum Winter '20 Release Notes
- Flosum Fall '19 Release Notes
- Flosum Spring '19 Release Notes
- Flosum Summer '21 Release Webinar
- Product Adoption Webinars
- Flosum Summer '23 Release Webinar
- Flosum Spring '24 Release Webinar
- Customer Training - Pull Requests
- Flosum Winter '23 Release Webinar
- Flosum Winter '24 Release Webinar
- Using Data Migrator Overlay Steps
- Customer Training – Merging Conflicts
- Winter 2021 Release Webinar
- Flosum's Spring Release Webinar 2021
- Business Continuity Plan
- Spring '20 Related Errors
- Flosum Pipelines: Winter 2020
- Webinar: 01/30/2020 - New Feature Overview, Pipeline Enhancements, Partial Rollbacks, Vlocity Components Support & More
- Flosum Refresher Training Webinar
- Webinar: Would You Like to Learn from Top Salesforce Professionals?
- Flosum's Summer 2020 Release Webinar
Data Migrator
- Data Migrator Frequently Asked Questions
- Migration of Product and Price Rules with Custom Conditions
- DM – Connect Your Organizations
- Support for Veeva
- nCino Integration
- Data Migrator Implementation User Guide
- Sequence Creation
- How to Give a User Access to Data Migrator
- Migrating the User Object
- Knowledge Article Migration Guide
- Data Migrator Proof of Concept
- Recursive relationships
- Field Level Security for Data Migrator
- Limitations of Data Migrator
- Best Practices for using Data Migrator
- Data Migrator Winter 2020 Release Notes
- Data Backup, Restore and Archive
- Security & Compliance
- Create a Salesforce API Limit Increase Request
- Implementation Best Practices
- Getting Started with DBR
- DBR Notifications
- Architecture, Prerequisites and Considerations
- DBR Archive
- Testing & Common Errors
- User Pool App
- Setting up Multi-Factor Authentication in User Pool
- Flosum User Pool SSO Setup with Google IDP
- Flosum User Pool SSO Setup with AWS Cognito
- Flosum User Pool SSO Setup with Azure Active Directory
Trust Center
- What is the Trust Center Solution?
- Trust Center Post-installation Steps
- Schedule Trust Center Ops
- Install Flosum Agent Application
- Install Trust Center
- Trust Center License Management
- Get Started with Trust Center
- Org Security Discover
- Org Security Dashboard
- Trust Center Org Security
- Trust Center Audit Trails
- Audit Trails Discover
- Audit Trails Dashboard
- Remediate Security Violations
- Trust Center Remediation
- Remediation Discover
- Remediation Dashboard
- Profile Security Template
- Organization Security Template
- Permission Security Template
- Trust Center Templates
- CRUD Report
- Metadata Reports
- Profile/Permission Set Comparison
- Data Dictionary
- Org Comparison
- Trust Center Data Masking
- Trust Center Data Masking Template
- Trust Center Data Masking Jobs
- Flosum Trust Center Settings
- Trust Center - Frequently Asked Questions
Org Scanner
- Org Security Scanner
- Flosum Release Management Frequently Asked Questions
- Data Backup and Recovery Solution Frequently Asked Questions
Profile deployments have always been super painful in Salesforce. Flosum eases that issue to a great extent but there is still a lot of confusion on the nitty-gritties of profile deployments. This article deals with all the questions we have encountered over our years of experience.
How do profiles work in Flosum?
RETRIEVAL – When Flosum retrieves a profile from the source org, the entire XML of the profile as published by Salesforce is retrieved. Metadata API, however, does not return False values in some cases, only values marked as True. Hence if you mark a value as False, it might not be retrieved and deployed. The details are below for each situation. Note: Some Profile names are slightly different from the standard Salesforce Profile names. For example:
StandardAul = Standard Platform User
Standard = standard user, marketingprofile = marketing user, admin = system administrator.
DEPLOY – On the Flosum Deployment screen, there is a setting – "Deploy profile settings only for components included in the deployment". This setting is checked by default. If your Deployment consists of Profiles and other components, then with this setting checked, the deployment will extract only the settings relevant to the other non-profile components in Deployment from all the profiles and deploy just those settings for each Profile. Let's explain this with an example.
Say you have 3 profiles in your Deployment and among other things, each of them has the FLS settings for 2 custom fields in them. Suppose one of those custom fields is only part of the Deployment and not the other one. Then if the checkbox is checked when deploying, the FLS settings for only the custom field that is in the Deployment will be migrated to the target org, NOT of the custom field that is not in the Deployment.
In the above scenario, if the checkbox on the Deployment screen was unchecked, then the FLS settings for both the custom fields would be deployed to the target org for all the 3 profiles.
Salesforce does not change the Last Modified date of profiles even when it has been changed by an FLS change in a custom field for example. You might need to know its last modified date to pull it or use a 0-day snapshot.
Component types that impact profiles
CUSTOM FIELDS – Field Level Security (FLS) for all fields is stored in profiles. So if you are deploying a custom field and want all its FLS settings to be deployed also, you need to deploy each of the profiles that have been given FLS settings for that custom field. Disabling profile access to a field can be deployed using Flosum .
CUSTOM OBJECTS – CRUD settings for all objects are stored in profiles. So if you are deploying a custom object and want all its CRUD settings to be deployed also, you need to deploy each of the profiles that have been given CRUD settings for that custom object. Disabled CRUD settings can also be deployed (if all settings for an object are disabled, the Metadata API does not pick up the object at all and hence this scenario cannot be deployed).
PAGE LAYOUTS – In order to deploy page layout assignments, you need to deploy the page layout, the record type it is associated with and the profiles together, if the checkbox "Deploy profile settings only for components included in the deployment" is checked. Just including the page layout and profiles only will not deploy the assignment if there is a record type dependency as well.
If there is no record type dependency, then just deploying the page layout and the associated profiles will deploy the page layout assignments.
RECORD TYPES – If you just deploy a record type without any of its associated profiles from the source org, this record type will not be available to any of the profiles in the target. Deploy the record type along with its associated profiles with the checkbox "Deploy profile settings only for components included in the deployment" checked and the record type will be available to the profiles as well.
CUSTOM APP / CONNECTED APP – Deploy the Custom App along with its associated profiles with the checkbox "Deploy profile settings only for components included in the deployment" checked, and the Custom App will be available to the profiles as well. You cannot disable access to a custom app using Flosum due to Metadata API limitations.
CUSTOM TAB – In the case of Custom Tab, the Off setting can also be migrated. So if the setting is set to Default On or Default Off or Tab Hidden, they can all be migrated if the Custom Tab and the relevant profiles are in the same Deployment and the checkbox "Deploy profile settings only for components included in the deployment" is checked.
APEX CLASS / VISUALFORCE PAGE – For both classes and Visualforce pages, profile access can be granted and revoked. Keep the class or Visualforce page in the Deployment along with the relevant profiles. Doing the deployment with the "Deploy profile settings only for components included in the deployment" checkbox checked should allow to grant and revoke access both (Managed package class and Visualforce permission settings cannot be deployed).
NAMED CREDENTIALS / EXTERNAL DATA SOURCE – For all these component types, profile access can be granted and revoked. Keep the component in the Deployment along with the relevant profiles. Doing the deployment with the checkbox "Deploy profile settings only for components included in the deployment" checked, should allow to grant and revoke access both.
CUSTOM PERMISSIONS – In order to retrieve and deploy metadata components, we use the Salesforce metadata API. Salesforce Metadata API allows you to add existing custom permissions to profiles, but you cannot remove them. The behavior can be verified by using Workbench or another tool that uses the API. Several other users who have encountered the problem (unable to remove the custom permission set from the profile present in a Flosum DevOps branch) have manually executed these changes. FLEXIPAGE – Please refer to this link .
Deploying Administrative Permissions/General User Permissions
In order to deploy the settings in the Administrative Permissions/General User Permissions section of any profile, we have to deselect the "Deploy profile settings only for components included in the deployment" checkbox in the Deploy screen and have the profile as part of the Deployment. Doing this will deploy the profile as a whole. False values cannot be deployed.
Deploying IP Logins
In order to deploy the IP Login records of any profile, we have to deselect the "Deploy profile settings only for components included in the deployment" checkbox in the Deploy screen and have the profile as part of the Deployment. Doing this will deploy the profile as a whole. Any IP login records in the profile in the target org that do not exist in the source profile will be deleted and only the ones that exist in the source org profile will exist in the target org profile after deployment.
Deploying Login Hours
To deploy the Login Hours associated with any profile, we have to deselect the "Deploy profile settings only for components included in the deployment" checkbox in the Deploy screen and have the profile as part of the Deployment. Doing this will deploy the profile as a whole including the login hours.
Deploying Session Settings and Password Policies
To deploy session settings and password policies, use the "ProfilePasswordPolicy" and "ProfileSessionSetting" objects. The profile needs to exist in the target org but does not need to be part of the Deployment to deploy these settings.
Deploying a brand new profile
If you need to deploy a brand new profile in its entirety, then please make sure to deselect the "Deploy profile settings only for components included in the deployment" checkbox if you do not want to include all the other components it references as part of the Deployment. This would be the most efficient way to deploy a new profile.
Other Points
If you check the "Deploy profile settings only for components included in the deployment" checkbox, only settings related to other components in the Deployment will be deployed. No other profile settings, even the ones not related to any component, will be deployed.
Profile Deployment Example
1. Partial profile deployment: Profile exists in Target Org Use Case: Developer has added changes to recordtype, custom fields, and Layouts and added them to the existing profile. In this case, we will use the Get Profile Feature: You will retrieve changes (recordtype, custom fields, Layouts) in a snapshot and commit to a branch. On the branch, you will see a lightning button known as "Get Profile" Use this get profile button to retrieve the profile where you have made these changes. In this case, it will retrieve only partial profile changes with respect to the components in your branch and then click on the deploy button. On the deployment page you will see a checkbox "Deploy profile settings only for components included in the branch" check this checkbox (by default it will be checked, so you don't have to do anything) and deploy to the target org. Document: https://success.flosum.com/s/article/Profile-Retrieval-on-the-Branch
2. Full Profile Deployment: Profile does not exist in Target Org Upon creating a new profile manually, Salesforce creates "App Permissions, Object Permissions, Apex classes, Visualforce pages, and some system permissions" by default and its a standard salesforce behaviour. As a result, you see discrepancies in the permissions of the source and target object profiles. Furthermore, we cannot disable these permissions using deployment since this is also standard Salesforce behaviour. The following steps should help you resolve this issue. This will be a one-time activity for new profile deployment. Steps: 1. Goto the Target Org. 2. Make a clone of any "Minimum Access - Salesforce" profile with the same name as the profile which you want to deploy from the source org. 3. Remove Read permission on 'Standard Object Permissions. 4. Make all tabs 'Default Off' on Tab Settings.
Note: When including profiles in a change set, it's important to review those profiles in the target environment after deployment as not all the permissions may carry over as you might expect. If object components are not included along with profiles, newly created profiles inherit the current object level access established in the license type's corresponding standard profile in the target organization. It's critical to review CRUD access to ensure newly created or deployed profile object level access in the target organization isn't greater than access set in the source organization. Some additional information: https://help.salesforce.com/Deploy-Profiles-via-Change-Sets .
Salesforce Code Crack
Monday, july 16, 2018, how to check page layout assignment using soql query.

Hey mate, whats the name of query builder you are using?

Hi Rajesh I am Using "ORGanizer for Salesforce" chrome Extension Link:https://chrome.google.com/webstore/detail/organizer-for-salesforce/lojdmgdchjcfnmkmodggbaafecagllnh?hl=en
hello, is there a way to call the default page or determine which layout is the default using query?
- Marketing Cloud
Experiences
Access Trailhead, your Trailblazer profile, community, learning, original series, events, support, and more.
Search Tips:
- Please consider misspellings
- Try different search keywords
LayoutSection Class
Layoutsection properties, layoutsection methods.
The following are properties for LayoutSection .
- customLabel Indicates if this section's label is custom or standard (built-in).
- detailHeading Controls if this section appears in the detail page.
- editHeading Controls if this section appears in the edit page.
- label The label; either standard or custom, based on the customLabel property.
- layoutColumns Lists the layout columns. You can have one, two, or three columns, ordered left to right, are possible.
- style The style of the layout for this section.
customLabel
public Boolean customLabel {get; set;}
Property Value
Type: Boolean
detailHeading
public Boolean detailHeading {get; set;}
editHeading
public Boolean editHeading {get; set;}
public String label {get; set;}
Type: String
layoutColumns
public List<Metadata.LayoutColumn> layoutColumns {get; set;}
Type: List < Metadata.LayoutColumn >
public Metadata.LayoutSectionStyle style {get; set;}
Type: Metadata.LayoutSectionStyle
The following are methods for LayoutSection .
- clone() Makes a duplicate copy of the Metadata.LayoutSection .
public Object clone()
Return Value
Type: Object
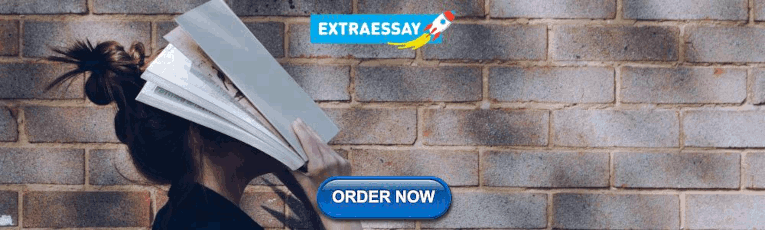
IMAGES
VIDEO
COMMENTS
Select Filters. Check the spelling of your keywords. Use more general search terms. Select fewer filters to broaden your search. After defining page layouts, assign which page layouts users see. A user's profile determines which page layout he or she sees. In addition, if your organ...
It is possible to make Tooling API ProfileLayout Object Query. [ select Layout.Name from ProfileLayout where ProfileId = :UserInfo.getProfileId() AND RecordTypeId = :record.RecordTypeId ] To make it possible to use in Apex Code you need preparation step. 1) Allow Self-Callout.
This type extends the Metadata metadata type and inherits its fullName field. To edit the Ideas layout, specify it by name in the package.xml file. In package.xml, use this code to retrieve the Ideas layout. <types> <members>Idea-Idea Layout</members> <name>Layout</name> </types>. Note.
From a page layout, click Mini Page Layout. Assign page layouts to users based on profiles and record types. This determines which layouts users will see when viewing different record types. From a page layout, click Page Layout Assignment. Go to the fields area to verify that all field access settings are correct.
From , choose Setup. Click Object Manager and select Knowledge. Click Record Types. Click New. Select an existing record type to use as a template. The new record type includes the picklist values of the selected record type. Maria chooses Master. Enter a label for your record type. Maria enters Procedure.
There are two ways:-. Using Metadata API:- You can get page layouts by Metadata API by defining the Object and Page layout name in package.xml. Using Tooling API:- Tooling API has object named "Layout" which can be queried. There is no way to query the Layouts by SOQL in Apex. Share. Improve this answer. edited Jun 15, 2020 at 8:11. Community Bot.
Record Type Record types in Salesforce are used to classify records based on certain criteria, which allows organizations to define different business processes, picklist values, page layouts, and even sharing rules for different types of records. Here are some use cases for record types in Salesforce: Customization: Record types allow you to customize different pages […]
0. First, use the Metadata API to Retrieve () the desired Profile. Look in the Profile XML file for the layoutAssignments element. It will look something like this: <layoutAssignments>. <layout>object-layout</layout>. <recordType>object.recordtype</recordType>. </layoutAssignments>. This defines the junction between the object, record type, and ...
In short term, the layout assignment information is listed in RecordType instead of Profile. When you retrieve whole SObject Describe from this endpoint:
Update the Product page layout assignment to use the Product (HowWeRoll) ... You need to have 100% code coverage for all Apex in your org. Ensure that your code operates as expected in the scheduled context by validating that it executes after Test.stopTest() without exception. Also assert that a scheduled asynchronous job is in the queue.
Page layout assignment: The future is App Builder/Dynamic Forms, so Salesforce will not invest in bringing page layout assignment to permission sets. This means that the very base permissions will still be managed via the profile. They aren't dead, but they aren't going to be as important as they were previously. ... Apex classes ...
The compact page layout is also assigned to IT records during installation. Assign the page layout to all IT record types and related profiles to have it appear in your org. From service Setup, click Object Manager. Click Case, then Case Page Layouts. Click Page Layout Assignment. Click Edit Assignment. Locate the IT column and select the ...
How do I define page layout assignments for different record types via metadata XML files? Stack Exchange Network Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Layout assignments; Object permissions; Permission dependencies; ... Indicates which top-level Apex classes have methods that users assigned to this profile can execute. ... Home page assignments related to user profile must also have a corresponding app assignment because more granular Home page assignments are supported. ...
PAGE LAYOUTS - In order to deploy page layout assignments, you need to deploy the page layout, the record type it is associated with and the profiles together, if the checkbox "Deploy profile settings only for components included in the deployment" is checked. Just including the page layout and profiles only will not deploy the assignment if ...
SELECT Layout.Name, Layout.TableEnumOrId, ProfileId, Profile.Name, RecordTypeId FROM ProfileLayout where recordtypeid='012f4000001DjwsAAC' Check Tooling API Guide to get more information on this. Share
This post explains how to check page layout assignment using SOQL. 1. We can see the Page Layout is assigned to any profile or not. 2. We can see the RecordTypeId also, means we can See the Record Type assignment also. Note: Use Tooling API to Query, Please Select Tooling API while Querying. If you are using Developer Console to query.
Controls if this section appears in the edit page. label. The label; either standard or custom, based on the customLabel property. layoutColumns. Lists the layout columns. You can have one, two, or three columns, ordered left to right, are possible. style. The style of the layout for this section.