- Mark Forums Read
- View Site Leaders
- What's New?
- Advanced Search
Advertiser Disclosure: Some of the products that appear on this site are from companies from which TechnologyAdvice receives compensation. This compensation may impact how and where products appear on this site including, for example, the order in which they appear. TechnologyAdvice does not include all companies or all types of products available in the marketplace.

- VB.Net Basic Tutorial
- VB.Net - Home
- VB.Net - Overview
- VB.Net - Environment Setup
- VB.Net - Program Structure
- VB.Net - Basic Syntax
- VB.Net - Data Types
- VB.Net - Variables
- VB.Net - Constants
- VB.Net - Modifiers
- VB.Net - Statements
- VB.Net - Directives
- VB.Net - Operators
- VB.Net - Decision Making
- VB.Net - Loops
- VB.Net - Strings
- VB.Net - Date & Time
- VB.Net - Arrays
- VB.Net - Collections
- VB.Net - Functions
- VB.Net - Subs
- VB.Net - Classes & Objects
- VB.Net - Exception Handling
- VB.Net - File Handling
- VB.Net - Basic Controls
- VB.Net - Dialog Boxes
- VB.Net - Advanced Forms
- VB.Net - Event Handling
- VB.Net Advanced Tutorial
- VB.Net - Regular Expressions
- VB.Net - Database Access
- VB.Net - Excel Sheet
- VB.Net - Send Email
- VB.Net - XML Processing
- VB.Net - Web Programming
- VB.Net Useful Resources
- VB.Net - Quick Guide
- VB.Net - Useful Resources
- VB.Net - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
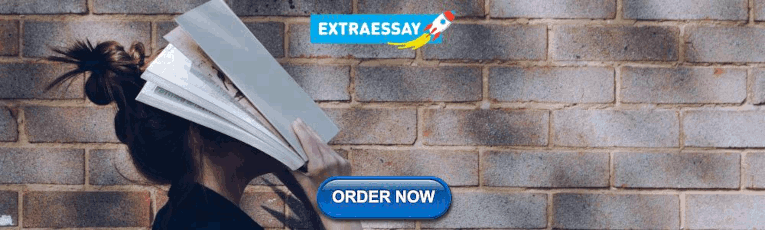
VB.Net - Assignment Operators
There are following assignment operators supported by VB.Net −
Try the following example to understand all the assignment operators available in VB.Net −
When the above code is compiled and executed, it produces the following result −
VB .NET Language in a Nutshell by Steven Roman PhD
Get full access to VB .NET Language in a Nutshell and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Assignment Operators
Along with the equal operator, there is one assignment operator that corresponds to each arithmetic and concatenation operator. Its symbol is obtained by appending an equal sign to the arithmetic or concatenation symbol.
The arithmetic and concatenation operators work as follows. They all take the form:
where <operator> is one of the arithmetic or concatenation operators. This is equivalent to:
To illustrate, consider the addition assignment operator. The expression:
is equivalent to:
which simply adds 1 to x. Similarly, the expression:
which concatenates the string "end" to the end of the string s.
All of the “shortcut” assignment operators—such as the addition assignment operator or the concatenation assignment operator—are new to VB .NET.
The assignment operators are:
The equal operator, which is both an assignment operator and a comparison operator. For example:
Note that in VB .NET, the equal operator alone is used to assign all data types; in previous versions of VB, the Set statement had to be used along with the equal operator to assign an object reference.
Addition assignment operator. For example:
adds 1 to the value of lNumber and assigns the result to lNumber.
Subtraction assignment operator. For example:
subtracts 1 from the value of lNumber and assigns the ...
Get VB .NET Language in a Nutshell now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
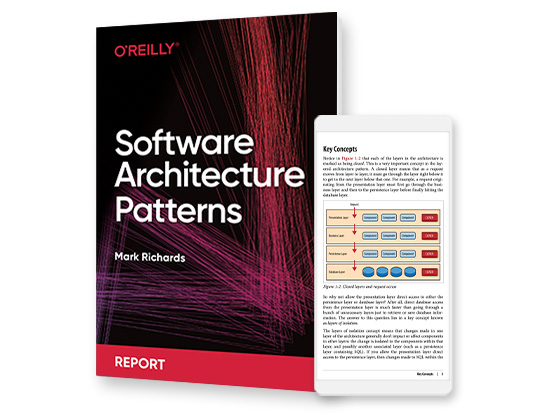
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
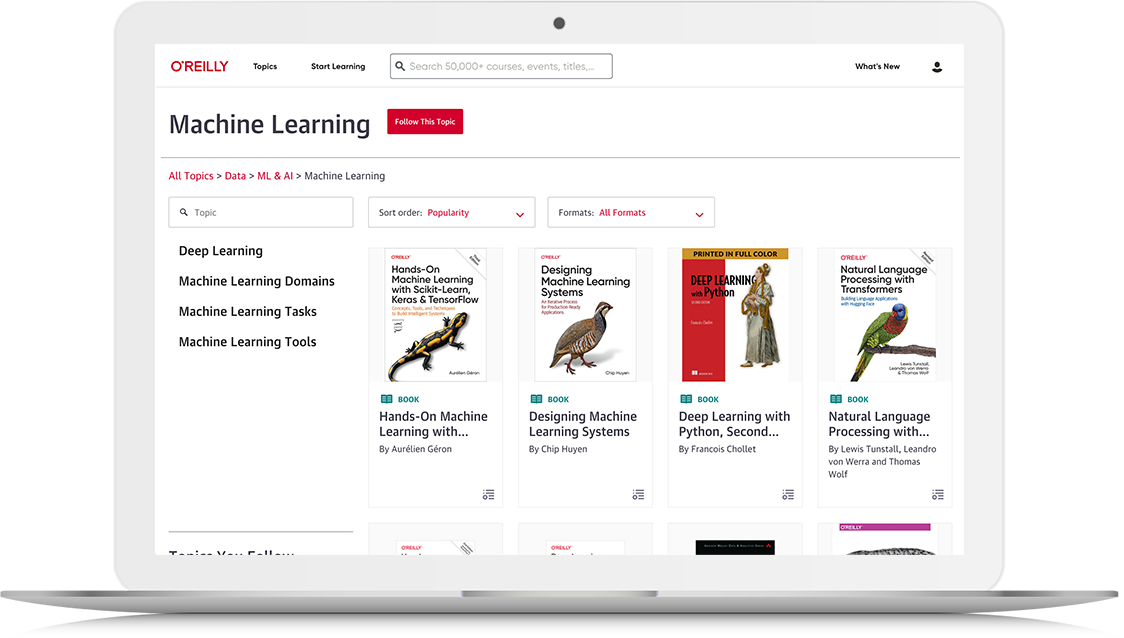
404 Not found
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
+= Operator (Visual Basic)
- 11 contributors
Adds the value of a numeric expression to the value of a numeric variable or property and assigns the result to the variable or property. Can also be used to concatenate a String expression to a String variable or property and assign the result to the variable or property.
variableorproperty Required. Any numeric or String variable or property.
expression Required. Any numeric or String expression.
The element on the left side of the += operator can be a simple scalar variable, a property, or an element of an array. The variable or property cannot be ReadOnly .
The += operator adds the value on its right to the variable or property on its left, and assigns the result to the variable or property on its left. The += operator can also be used to concatenate the String expression on its right to the String variable or property on its left, and assign the result to the variable or property on its left.
When you use the += operator, you might not be able to determine whether addition or string concatenation will occur. Use the &= operator for concatenation to eliminate ambiguity and to provide self-documenting code.
This assignment operator implicitly performs widening but not narrowing conversions if the compilation environment enforces strict semantics. For more information on these conversions, see Widening and Narrowing Conversions . For more information on strict and permissive semantics, see Option Strict Statement .
If permissive semantics are allowed, the += operator implicitly performs a variety of string and numeric conversions identical to those performed by the + operator. For details on these conversions, see + Operator .
Overloading
The + operator can be overloaded , which means that a class or structure can redefine its behavior when an operand has the type of that class or structure. Overloading the + operator affects the behavior of the += operator. If your code uses += on a class or structure that overloads + , be sure you understand its redefined behavior. For more information, see Operator Procedures .
The following example uses the += operator to combine the value of one variable with another. The first part uses += with numeric variables to add one value to another. The second part uses += with String variables to concatenate one value with another. In both cases, the result is assigned to the first variable.
The value of num1 is now 13, and the value of str1 is now "103".
- Assignment Operators
- Arithmetic Operators
- Concatenation Operators
- Operator Precedence in Visual Basic
- Operators Listed by Functionality
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Performing Calculations in VB.NET
Precedence rules determine the order in which operators within expressions are evaluated. Operators are applied in the precedence order shown in Table 3-1. Operators within a row have equal precedence. For operators of equal precedence within the same expression, the order of evaluation is from left to right, as they appear in an expression. Order of evaluation can be explicitly controlled by using parentheses.
Table 3-1. Operator Precedence in VB.NET
Output and formatting.
The Console class in the System namespace supports two simple methods for performing output:
WriteLine writes out a string followed by a new line.
Write writes out just the string without the new line.
You can write out other data types by relying on the ToString method of System.Object , which will provide a string representation of any data type. System.Object is the base class from which all classes inherit. We will discuss this class in Chapter 6, where you will also see how to override ToString for your own custom data types. You can use the string concatenation operator & to build up an output string.
The output is all on one line:
Placeholders
A more convenient way to build up an output string is to use placeholders such as {0}, {1}, and so on. An equivalent way to do the output shown above is
The program OutputDemo illustrates the output operations just discussed.
We will generally use placeholders for our output from now on. Placeholders can be combined with formatting characters to control output format.
Format Strings
VB.NET has extensive formatting capabilities, which you can control through placeholders and format strings.
Simple placeholders: {n}, where n is 0, 1, 2, , indicating which variable to insert
Control width: {n,w}, where w is width (positive for right justified and negative for left justified) of the inserted variable
Format string: {n:S}, where S is a format string indicating how to display the variable
Width and format string: {n,w:S}
A format string consists of a format character followed by an optional precision specifier . Table 3-2 shows the available format characters.
Table 3-2. Format Characters
Sample formatting code.
The sample program in Ira\Step1 provides an example. The header uses width specifiers, and the output inside the loop uses width specifiers and the currency format character.
Control Structures
The preceding code fragment illustrates a While End While loop. VB.NET supports several control structures, including the If and Select decision statements as well as For and While loops .
While End While
Do While Loop
Do Until Loop
Do Loop While
Do Loop Until
For Each Next
If Then End If
If Then Else End If
If Then ElseIf Then End If
Select Case End Select
With End With
SyncLock End SyncLock
Try Catch Finally
Most of these will be familiar to Visual Basic programmers. The Throw and Try statements are used in exception handling. We will discuss exceptions later in this chapter. The SyncLock statement can be used to enforce synchronization in multithreading situations. We will discuss multithreading in Chapter 10.
Select Case Statement
A Select Case statement is used to execute one of several groups of statements, depending on the value of a test expression. The test expression must be one of the following data types: Boolean , Byte , Char , Date , Double , Decimal , Integer , Long , Object , Short , Single , or String .
After a particular case statement is executed, control automatically continues after the following End Select . The program SelectDemo illustrates use of the Select Case statement in VB.NET.
Our Ira\Step1 example program has a method IraTotal for computing the total IRA accumulation by use of a formula. In VB.NET, every function is a method of some class or module; there are no freestanding global functions. If a method does not refer to any instance variables of the class, the method can be declared as Shared . We will discuss instance data of a class later in this chapter. If a method is accessed only from within a single class, it may be designated as Private . Note the use of the Private keyword in the Ira\Step1 example. The Shared keyword is not used however, since the example uses a module instead of a class. All methods in a module are shared by default.
Also in the Ira\Step1 example, note the use of the Pow and Round methods of the Math class, which is another class in the System namespace. These methods are shared methods. To call a shared method from outside the class in which it is defined, place the name of the class followed by a period before the method name.
Console Input in VB.NET
Our first Ira program is not too useful, because the data are hardcoded. To perform the calculation for different data, you would have to edit the source file and recompile. What we really want to do is allow the user of the program to enter the data at runtime.
An easy, uniform way to do input for various data types is to read the data as a string and then convert it to the desired data type. Use the ReadLine method of the System.Console class to read in a string. Use the ToXxxx methods of the System.Convert class to convert the data to the type you need. This can be seen in the Ira\Step2 example.
Although console input in VB.NET is fairly simple, we can make it even easier using object-oriented programming. We can encapsulate the details of input in an easy-to-use wrapper class, InputWrapper (which is not part of VB.NET or the .NET Framework class library, and was created for this book).
Using the Inputwrapper Class
In VB.NET, you instantiate a class by using the New keyword.
This code creates the object instance iw of the InputWrapper class.
The InputWrapper class wraps interactive input for several basic data types. The supported data types are int , double , decimal , and string . Methods getInt , getDouble , getDecimal , and getString are provided to read those types from the command line. A prompt string is passed as an input parameter. The directory InputWrapper contains the files InputWrapper.vb , which implements the class, and TestInputWrapper.vb , which tests the class. (For convenience, we provide the file InputWrapper.vb in each project where we use it.)
You can use the InputWrapper class without knowing its implementation. With such encapsulation, complex functionality can be hidden by an easy-to-use interface. (A listing of the InputWrapper class is in the next section.)
Here is the code for Ira\Step2 . We read in the deposit amount, the interest rate, and the number of years, and we compute the IRA accumulation year by year. The first input is done directly, and then we use the InputWrapper class. The bolded code illustrates how to use the InputWrapper class. Instantiate an InputWrapper object iw by using new . Prompt for and obtain input data by calling the appropriate getXXX method.
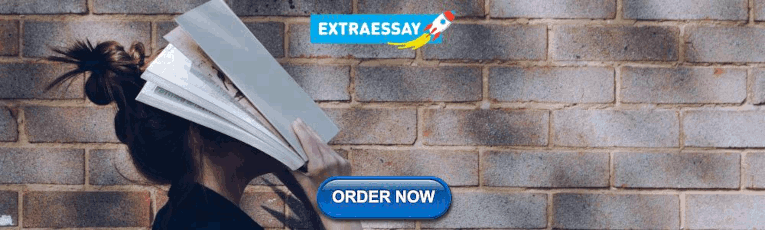
Inputwrapper Class Implementation
The InputWrapper class is implemented in the file InputWrapper.vb . You should find the code reasonably intuitive, given what you already know about classes.
Note that, unlike the method IraTotal , the methods of the InputWrapper class are used outside of the class so they are marked as public .
If bad input data is presented, an exception will be thrown. Exceptions are discussed in Chapter 5.
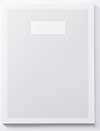
- IT Project+ Study Guide
- Scope Planning
- Comprehensive Project Plan
- Project Control
- Project Closure
- The Pipe-and-Filter Style
- Work Assignment Style
- Advanced Concepts
- What to Document
- Prerequisites
- Postfix Delivery Transports
- POP and IMAP
- Spam Detection
- External Databases
- A Closer Look at HelloWorld
- Understanding Thread Priority
- Example Five: VoteDialog
- Layout Management
- Substituting Variables into an Existing String
- Solving a System of Linear Equations
- Ignoring Case When Sorting Strings
- Hiding Setup and Cleanup in a Block Method
- Web Development Ruby on Rails
- Getting BIND
- Creating a named.conf File
- Making Your Services Easy to Find
- Configuring a Name Server Not to Forward Certain Queries
- Restarting a Name Server Automatically If It Dies
Compound-Assignment Operators
- Java Programming
- PHP Programming
- Javascript Programming
- Delphi Programming
- C & C++ Programming
- Ruby Programming
- Visual Basic
- M.A., Advanced Information Systems, University of Glasgow
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound-Assignment Operators in Java
Java supports 11 compound-assignment operators:
Example Usage
To assign the result of an addition operation to a variable using the standard syntax:
But use a compound-assignment operator to effect the same outcome with the simpler syntax:
- Conditional Operators
- Java Expressions Introduced
- Understanding the Concatenation of Strings in Java
- The 7 Best Programming Languages to Learn for Beginners
- Math Glossary: Mathematics Terms and Definitions
- The Associative and Commutative Properties
- The JavaScript Ternary Operator as a Shortcut for If/Else Statements
- Parentheses, Braces, and Brackets in Math
- Basic Guide to Creating Arrays in Ruby
- Dividing Monomials in Basic Algebra
- C++ Handling Ints and Floats
- An Abbreviated JavaScript If Statement
- Using the Switch Statement for Multiple Choices in Java
- How to Make Deep Copies in Ruby
- Definition of Variable
- The History of Algebra
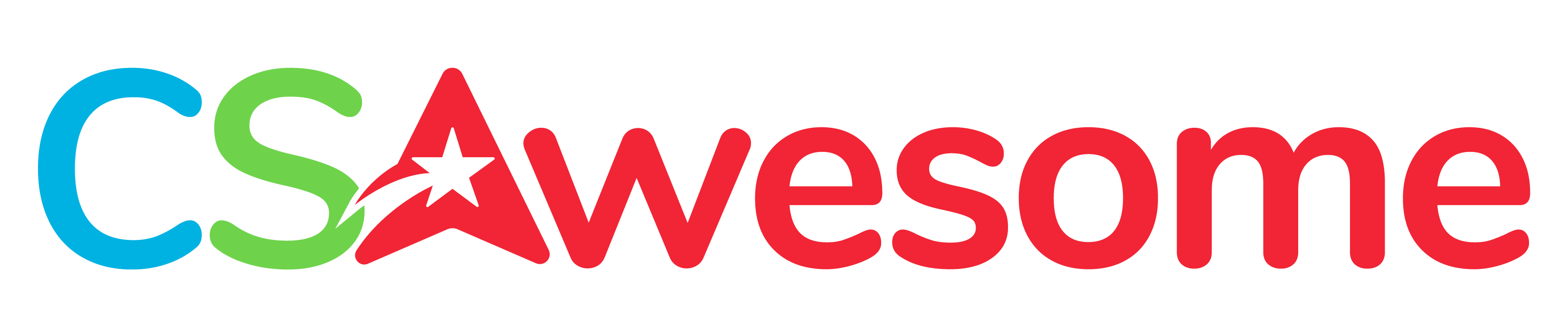
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Getting Started
- 1.1.1 Preface
- 1.1.2 About the AP CSA Exam
- 1.1.3 Transitioning from AP CSP to AP CSA
- 1.1.4 Java Development Environments
- 1.1.5 Growth Mindset and Pair Programming
- 1.1.6 Pretest for the AP CSA Exam
- 1.1.7 Survey
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Values
- 1.7 Unit 1 Summary
- 1.8 Mixed Up Code Practice
- 1.9 Toggle Mixed Up or Write Code Practice
- 1.10 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.4. Expressions and Assignment Statements" data-toggle="tooltip">
- 1.6. Casting and Ranges of Values' data-toggle="tooltip" >
Time estimate: 45 min.
1.5. Compound Assignment Operators ¶
Compound assignment operators are shortcuts that do a math operation and assignment in one step. For example, x += 1 adds 1 to the current value of x and assigns the result back to x . It is the same as x = x + 1 . This pattern is possible with any operator put in front of the = sign, as seen below. If you need a mnemonic to remember whether the compound operators are written like += or =+ , just remember that the operation ( + ) is done first to produce the new value which is then assigned ( = ) back to the variable. So it’s operator then equal sign: += .
Since changing the value of a variable by one is especially common, there are two extra concise operators ++ and -- , also called the plus-plus or increment operator and minus-minus or decrement operator that set a variable to one greater or less than its current value.
Thus x++ is even more concise way to write x = x + 1 than the compound operator x += 1 . You’ll see this shortcut used a lot in loops when we get to them in Unit 4. Similarly, y-- is a more concise way to write y = y - 1 . These shortcuts only exist for + and - as they don’t really make sense for other operators.
If you’ve heard of the programming language C++, the name is an inside joke that C, an earlier language which C++ is based on, had been incremented or improved to create C++.
Here’s a table of all the compound arithmetic operators and the extra concise incremend and decrement operators and how they relate to fully written out assignment expressions. You can run the code below the table to see these shortcut operators in action!
Run the code below to see what the ++ and shorcut operators do. Click on the Show Code Lens button to trace through the code and the variable values change in the visualizer. Try creating more compound assignment statements with shortcut operators and work with a partner to guess what they would print out before running the code.
If you look at real-world Java code, you may occassionally see the ++ and -- operators used before the name of the variable, like ++x rather than x++ . That is legal but not something that you will see on the AP exam.
Putting the operator before or after the variable only changes the value of the expression itself. If x is 10 and we write, System.out.println(x++) it will print 10 but aftewards x will be 11. On the other hand if we write, System.out.println(++x) , it will print 11 and afterwards the value will be 11.
In other words, with the operator after the variable name, (called the postfix operator) the value of the variable is changed after evaluating the variable to get its value. And with the operator before the variable (the prefix operator) the value of the variable in incremented before the variable is evaluated to get the value of the expression.
But the value of x after the expression is evaluated is the same in either case: one greater than what it was before. The -- operator works similarly.
The AP exam will never use the prefix form of these operators nor will it use the postfix operators in a context where the value of the expression matters.

1-5-2: What are the values of x, y, and z after the following code executes?
- x = -1, y = 1, z = 4
- This code subtracts one from x, adds one to y, and then sets z to to the value in z plus the current value of y.
- x = -1, y = 2, z = 3
- x = -1, y = 2, z = 2
- x = 0, y = 1, z = 2
- x = -1, y = 2, z = 4
1-5-3: What are the values of x, y, and z after the following code executes?
- x = 6, y = 2.5, z = 2
- This code sets x to z * 2 (4), y to y divided by 2 (5 / 2 = 2) and z = to z + 1 (2 + 1 = 3).
- x = 4, y = 2.5, z = 2
- x = 6, y = 2, z = 3
- x = 4, y = 2.5, z = 3
- x = 4, y = 2, z = 3
1.5.1. Code Tracing Challenge and Operators Maze ¶
Use paper and pencil or the question response area below to trace through the following program to determine the values of the variables at the end.
Code Tracing is a technique used to simulate a dry run through the code or pseudocode line by line by hand as if you are the computer executing the code. Tracing can be used for debugging or proving that your program runs correctly or for figuring out what the code actually does.
Trace tables can be used to track the values of variables as they change throughout a program. To trace through code, write down a variable in each column or row in a table and keep track of its value throughout the program. Some trace tables also keep track of the output and the line number you are currently tracing.
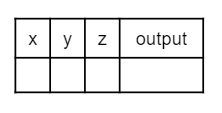
Trace through the following code:
1-5-4: Write your trace table for x, y, and z here showing their results after each line of code.
After doing this challenge, play the Operators Maze game . See if you and your partner can get the highest score!
1.5.2. Summary ¶
Compound assignment operators ( += , -= , *= , /= , %= ) can be used in place of the assignment operator.
The increment operator ( ++ ) and decrement operator ( -- ) are used to add 1 or subtract 1 from the stored value of a variable. The new value is assigned to the variable.
The use of increment and decrement operators in prefix form (e.g., ++x ) and inside other expressions (i.e., arr[x++] ) is outside the scope of this course and the AP Exam.
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
IDE0054 "Use compound assignment" false positive in object initializer #33382
dgrunwald commented Feb 14, 2019
jnm2 commented Mar 9, 2019
Sorry, something went wrong.
mavasani commented Mar 9, 2019
CyrusNajmabadi commented Mar 9, 2019
Jnm2 commented mar 9, 2019 • edited.
No branches or pull requests
JetBrains Rider 2024.1 Help
Code inspection: use compound assignment.
This inspection suggests using a compound assignment expression to make the code more concise and easier to read. Compound assignments are shorthand ways of combining arithmetic, boolean, bitwise, and other binary operators with the assignment operator = . They can help reduce repetitive code and make the intent of the code clearer.
The most commonly used compound assignment expression is probably the addition assignment ( x += y ), but there are other compound assignments that can come in handy. Here are some examples:
- Skip to main content
- Skip to primary sidebar
- Skip to secondary sidebar

Building Compound Controls
A compound control provides a visible interface that consists of multiple Windows controls. The controls that make up a compound control are known as constituent controls. As a result, this type of control doesn’t inherit the functionality of any specific control. You must implement its properties and methods with custom code. This isn’t as bad as it sounds, because a compound control inherits the UserControl object, which exposes quite a few members of its own (the Anchoring and Docking properties, for example, are exposed by the UserControl object, and you need not implement these properties — thank Microsoft). You will add your own members, and in most cases you’ll be mapping the properties and methods of the compound controls to a property or method of one of its constituent controls. If your control contains a TextBox control, for example, you can map the custom control’s WordWrap property to the equivalent property of the TextBox. The following property procedure demonstrates how to do it:
You don’t have to maintain a private variable for storing the value of the custom control’s WordWrap property. When this property is set, the Property procedure assigns the property’s value to the TextBox1.WordWrap property. Likewise, when this property’s value is requested, the procedure reads it from the constituent control and returns it. In effect, the custom control’s WordWrap property affects directly the functionality of one of the constituent controls.
The same logic applies to events. Let’s say your compound control contains a TextBox and a ComboBox control, and you want to raise the TextChanged event when the user edits the TextBox control, and the SelectionChanged event when the user selects another item in the ComboBox control. First, you must declare the two events:
Then, you must raise the two events from within the appropriate event handlers: the Text-Changed event from the TextBox1 control’s TextChanged event handler, and the SelectionChanged event from the ComboBox1 control’s SelectedIndexChanged event handler:
The ColorEdit Control Example
In this section, you’re going to build a compound control that’s similar to the Color dialog box ( Download the project files ). The ColorEdit control allows you to specify a color by adjusting its red, green, and blue components with three scroll bars, or to select a color by name. The control’s surface at runtime on a form is shown in Figure 8.4.
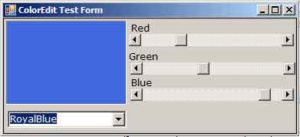
Create a new Windows Control Library project, the ColorEdit project. Save the solution and then add a new Windows Application project, the TestProject, and make it the solution’s startup project, just as you did with the first sample project of this chapter.
Now open the UserControl object and design its interface, as shown in Figure 8.4. Place the necessary controls on the UserControl object’s surface and align them just as you would do with a Windows form. The three ScrollBar controls are named RedBar, GreenBar, and BlueBar, respectively. The Minimum property for all three controls is 0; the Maximum for all three is 255. This is the valid range of values for a color component. The control at the top-left corner is a Label control with its background color set to Black. (We could have used a PictureBox control in its place.) The role of this control is to display the selected color.
The ComboBox at the bottom of the custom control is the NamedColors control, which is populated with color names when the control is loaded. The Color class exposes 140 properties, which are color names (Beige, Azure, and so on). Don’t bother entering all the color names in the ComboBox control; just open the ColorEdit project and you will find the AddNamedColors() subroutine, which does exactly that.
The user can specify a color by sliding the three ScrollBar controls or by selecting an item in the ComboBox control. In either case, the Label control’s Background color will be set to the selected color. If the color is specified with the ComboBox control, the three ScrollBars will adjust to reflect the color’s basic components (red, green, and blue). Not all possible colors that you can specify with the three ScrollBars have a name (there are approximately 16 million colors). That’s why the ComboBox control contains the Unknown item, which is selected when the user specifies a color by setting its basic components.
Finally, the ColorEdit control exposes two properties: NamedColor and SelectedColor. The NamedColor property retrieves the selected color’s name. If the color isn’t selected from the ComboBox control, the value Unknown will be returned. The SelectedColor property returns or sets the current color. Its type is Color, and it can be assigned any expression that represents a color value. The following statement will assign the form’s BackColor property to the SelectedColor property of the control:
You can also specify a color value with the FromARGB method of the Color object:
The implementation of the SelectedColor property (shown in Listing 8.3) is straightforward. The Get section of the procedure assigns the Label’s background color to the SelectedColor property. The Set section of the procedure extracts the three color components from the value of the property and assigns them to the three ScrollBar controls. Then it calls the ShowColor subroutine to update the display. (You’ll see shortly what this subroutine does.)
Listing 8.3: SelectedColor Property Procedure
The NamedColor property (see Listing 8.4) is read-only and is marked with the ReadOnly keyword in front of the procedure’s name. This property retrieves the value of the ComboBox control and returns it.
Listing 8.4: NamedColor Property Procedure
When the user selects a color name in the ComboBox control, the code retrieves the corresponding color value with the Color.FromName method. This method accepts a color name as an argument (a string) and returns a color value, which is assigned to the namedColor variable. Then the code extracts the three basic color components with the R, G, and B properties. (These properties return the red, green, and blue color components, respectively.) Listing 8.5 shows the code behind the ComboBox control’s SelectedIndexChanged event, which is fired every time a new color is selected by name.
Listing 8.5: Specifying a Color by Name
The ShowColor() subroutine simply sets the Label’s background color to the value specified by the three ScrollBar controls. Even when you select a color value by name, the control’s code sets the three ScrollBars to the appropriate values. This way, we don’t have to write additional code to update the display. The ShowColor() subroutine is quite trivial:
The single statement in this subroutine picks up the values of the three basic colors from the ScrollBar controls and creates a new color value with the FromARGB method of the Color object. The first argument is the transparency of the color (the A, or alpha channel), and we set it to 255 for a completely opaque color. You can edit the project’s code to take into consideration the transparency channel as well. If you do, you must replace the Label control with a PictureBox control and display an image in it. Then draw a rectangle with the specified color on top of it. If the color isn’t completely opaque, you’ll be able to see the underlying image and visually adjust the transparency channel.
Testing the ColorEdit Control
To test the new control, you must place it on a form. Build the ColorEdit control and switch to the test project (add a new project to the current solution if you haven’t done so already). Add an instance of the new custom control to the form. You don’t have to enter any code in the test form. Just run it and see how you specify a color, either with the scroll bars or by name. You can also read the value of the selected color through the SelectedColor property. The code behind the Color Form button on the test form does exactly that (it reads the selected color and paints the form with this color):
Related lessons:
- Building User-Drawn Controls
- Customizing List Controls
- Designing Windows Controls
- Enhancing Existing Controls
- ListBox and CheckedListBox Controls
- Designing Irregularly Shaped Controls

- Visual Basic.NET
- Systems Analysis
- ** Browse Articles **
- Getting Started with Visual Basic 2008
- Using Variables and Data Types
- Programming Fundamentals
- Windows Controls
- Working with Forms
- Custom Classes
- Working with Objects
- Building Custom Windows Controls
- Handling Strings, Characters and Dates
- Storing Data in Collections
- Accessing Folders and Files
- Serialization and XML
- Querying Collections and XML with LINQ
- Drawing and Painting
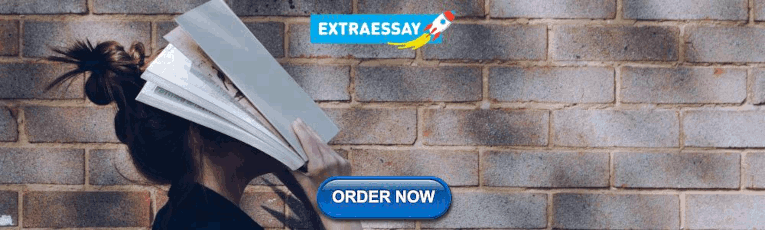
IMAGES
VIDEO
COMMENTS
These rules concern the use of compound assignment. IDE0074 is reported for coalesce compound assignments and IDE0054 is reported for other compound assignments. Options. The option value specifies whether or not compound assignments are desired. For information about configuring options, see Option format. dotnet_style_prefer_compound_assignment
The following are the assignment operators defined in Visual Basic. = Operator ^= Operator *= Operator /= Operator \= Operator += Operator-= Operator <<= Operator >>= Operator &= Operator. See also. Operator Precedence in Visual Basic; Operators Listed by Functionality; Statements
Re: (newbie question) "Use compound assignment" in a simple program. Originally Posted by David Anton. It both compiles and runs for me. The issue about compound assignment is just a suggestion by Visual Studio - you can rewrite that statement as "n /= 2", but your version is still correct. I just compiled it again, and I am getting a different ...
Try the following example to understand all the assignment operators available in VB.Net −. Module assignment. Sub Main() Dim a As Integer = 21 Dim pow As Integer = 2 Dim str1 As String = "Hello! " Dim str2 As String = "VB Programmers" Dim c As Integer. c = a. Console.WriteLine("Line 1 - = Operator Example, _.
myFactory.GetNextObject().MyProperty += 5; You _certainly wouldn't do. myFactory.GetNextObject().MyProperty = myFactory.GetNextObject().MyProperty + 5; You could again use a temp variable, but the compound assignment operator is obviously more succinct. Granted, these are edge cases, but it's not a bad habit to get into.
Apr 30, 2009 at 18:57. from the Java Language Specification, the difference lies in the number of times x+1 is evaluated: "A compound assignment expression of the form E1 op= E2 is equivalent to E1 = (T) ( (E1) op (E2)), where T is the type of E1, except that E1 is evaluated only once. Note that the implied cast to type T" Which proves your ...
Remarks. The element on the left side of the &= operator can be a simple scalar variable, a property, or an element of an array. The variable or property cannot be ReadOnly. The &= operator concatenates the String expression on its right to the String variable or property on its left, and assigns the result to the variable or property on its left.
A variety of compound assignment operations can be performed using operators of this type. For a list of these operators and more information about them, see Assignment Operators (Visual Basic). The concatenation assignment operator (&=) is useful for adding a string to the end of already existing strings, as the following example illustrates.
The assignment operators are: =. The equal operator, which is both an assignment operator and a comparison operator. For example: oVar1 = oVar2. Note that in VB .NET, the equal operator alone is used to assign all data types; in previous versions of VB, the Set statement had to be used along with the equal operator to assign an object reference.
Chapter 5 Using compound assignment and iteration statements After completion this chapter, you will be able to: Update the value of a variable on usage mix assignment operators. Write while … - Selection from Microsoft Visual C# Step by Step, 10th Edition [Book]
This style rule concerns with the use of compound assignments. The option value specifies whether or not they are desired. IDE0074 is reported for coalesce compound assignments and IDE0054 for other compound assignments. \n dotnet_style_prefer_compound_assignment \n
Visual Basic does not support compound assignment operators as shown in the C# sample. ... It seems to me that there isn't an existing combination of the bitwise-or-plus-assignment operator in VB.NET. But there is a bitwise-or operator, and an assignment operator, which you can combine manually: flags = flags Or MyEnum.SomeFlag
This assignment operator implicitly performs widening but not narrowing conversions if the compilation environment enforces strict semantics. For more information on these conversions, see Widening and Narrowing Conversions.For more information on strict and permissive semantics, see Option Strict Statement.. If permissive semantics are allowed, the += operator implicitly performs a variety of ...
One of the newest features of VB.NET is its new compound assignment operators that perform arithmetic operations as part of the assignment. Here are examples from the Ira program: i += 1 ' this is equivalent to i = i + 1 total += amount + interest ' this is equivalent to ' total = total + amount + interest . There are six new compound ...
Compound-Assignment Operators. Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
1.5. Compound Assignment Operators¶. Compound assignment operators are shortcuts that do a math operation and assignment in one step. For example, x += 1 adds 1 to the current value of x and assigns the result back to x.It is the same as x = x + 1.This pattern is possible with any operator put in front of the = sign, as seen below. If you need a mnemonic to remember whether the compound ...
1. When using C#, the compound assignment operators ( +=, *=, etc.) are automatically created from the corresponding binary operator: Compound assignment operators cannot be explicitly overloaded. However, when you overload a binary operator, the corresponding compound assignment operator, if any, is also implicitly overloaded.
There is an IDE0054 "Use compound assignment" hint for level = level - 1, but this doesn't make sense in an object initializer. The text was updated successfully, but these errors were encountered: All reactions. ...
Compound assignments are shorthand ways of combining arithmetic, boolean, bitwise, and other binary operators with the assignment operator =. They can help reduce repetitive code and make the intent of the code clearer. The most commonly used compound assignment expression is probably the addition assignment ( x += y ), but there are other ...
15. Expanding on Mark's correct answer. This type of assignment style is not possible in VB.Net. The C# version of the code works because in C# assignment is an expression which produces a value. This is why it can be chained in this manner. In VB.Net assignment is a statement and not an expression. It produces no value and cannot be changed.
Building Compound Controls. A compound control provides a visible interface that consists of multiple Windows controls. The controls that make up a compound control are known as constituent controls. As a result, this type of control doesn't inherit the functionality of any specific control. You must implement its properties and methods with ...