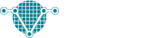
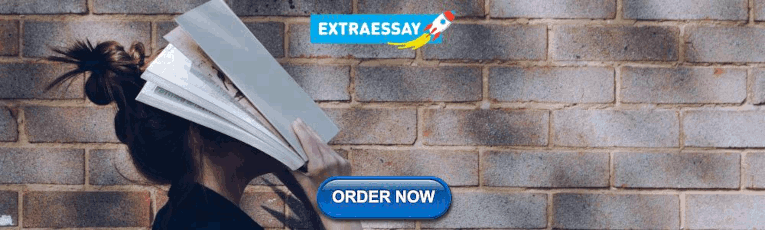
SystemVerilog Queues
A queue is a variable size and ordered collection of elements (homogeneous element).
To understand it is considered the same as a single-dimensional unpacked array that grows and reduces automatically if it is a bounded queue.
Types of queues in SystemVerilog
- Bounded queue: Queue having a specific size or a limited number of entries.
- Unbounded queue: Queue having non-specific queue size or unlimited entries.
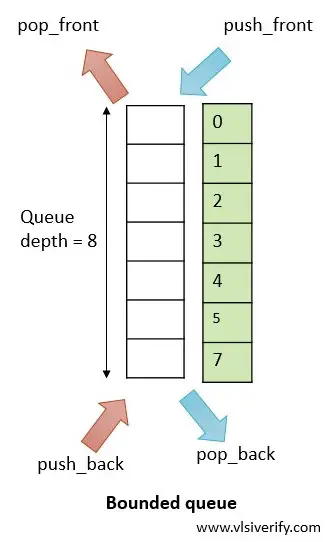
Declaration of a queue in SystemVerilog
For Example:
SystemVerilog Queue methods
Systemverilog queue example, example for shuffle method.
Let’s see how the shuffle method shuffles queue’s items.
Array of queues
An array can store queues. In the below example,
array[0] stores a queue of even numbers.
array[1] stores a queue of odd numbers.
array[2] stores a queue of multiple hundreds.
Initialization of array of queues
- Based on array index
- Without using an array index
Array of queues Example
System Verilog Tutorials

SystemVerilog Queue
Table of Contents
SystemVerilog Tutorial
SV Introduction
Verification
Packed Array
Unpacked Array
Fixed Array
Dynamic Array
Associative Array
Queue in SystemVerilog
A queue in SystemVerilog is a variable-size, ordered collection of homogeneous elements . “Variable size” means adding or removing elements as needed without specifying a fixed size in advance. “Ordered collection” indicates that the elements are stored in the order in which they were added, following a FIFO (First-In-First-Out) order. SystemVerilog provides various methods to manipulate queues.
A SystemVerilog queue stores elements of the same data type , maintaining an ordered collection in a First-In-First-Out (FIFO) manner. This ensures the elements are arranged in the order they were added, with indexing starting from 0. Each element in the queue is assigned an index value, starting from 0 for the first element, 1 for the second, and so forth.
Accessing elements via their index values facilitates easy retrieval or manipulation of specific elements within the queue. Queue elements can be accessed by index value.
SystemVerilog queue is dynamic but don’t require the ` new` operator for memory allocation or resizing. They automatically manage memory allocation and deallocation internally, enabling the user to add or remove elements without explicit memory management.
Queue capable of holding infinite elements. The queue’s capacity is practically boundless in terms of dynamically increasing its size to accommodate elements. However, it’s important to note that the available memory in the system might limit the actual capacity.
The `queue_name[$]` syntax represents a queue capable of dynamically handling an arbitrary number of elements. This dynamic nature allows for flexibility in accommodating elements without explicitly allocating memory.
data_type queue_name[$];
where: data_type – data type of the queue elements. queue_name – name of the queue.
Queue methods in systemverilog:

Method Description
size() returns the number of elements in the queue
insert() insert the given element at the specified index position of queue
delete() deletes the element at the specified index position of the queue
pop_front() remove and return the first element of the queue
pop_back() remove and return the last element of the queue
push_front() insert the element at the front of the queue
push_back() insert the element at the end of the queue
shuffle() shuffle the queue elements in a random manner
reverse() reverse the queue elements order
You can have an unbounded or bounded queue.
Bounded queue: queue with a restricted number of entries or queue size defined.
int queue1[$:7];
Unbounded queue: queue with infinite entries or queue size not specified;
int queue1[$];
Bounded Queue in systemverilog:
A bounded queue in SystemVerilog is a queue with a restricted number of entries or queue size defined that allows you to store and manage a fixed number of elements in a first-in, first-out (FIFO). Unlike an array, a bounded queue has a fixed size, and when it’s full, adding new elements will require removing older elements to make space.

Here’s an example of how you can implement a bounded queue in SystemVerilog:
Unbounded Queue in systemverilog:
In SystemVerilog, an unbounded queue is a dynamic data structure that doesn’t have a predefined fixed size. It grows dynamically as elements are added and shrinks as elements are removed.

Here’s an example of an unbounded queue in SystemVerilog:
Also read: Queue codes in SystemVerilog
Privacy Policy - Terms and Conditions
SystemVerilog Dynamic Arrays and Queues
In this post, we talk about the different types of dynamic arrays we can use in SystemVerilog, including normal dynamic arrays and their associated methods , queues and their associated methods and finally associative arrays .
As we talked about in a previous post, we can declare either static or dynamic arrays in SystemVerilog.
When we declare a static array, a fixed amount of memory is allocated to the array at compile time . We have discussed SystemVerilog static arrays in depth in a previous blog post.
In contrast, we can allocate extra memory or resize a dynamic array while a simulation is running. As a result of this, we can only use dynamic arrays in our testbench code and not in synthesizable code.
In the rest of this post we talk about the way we can use dynamic arrays in our SystemVerilog code.
There are actually three different types of dynamic array which we can use in SystemVerilog - dynamic arrays, queues and associative arrays.
We use each of these three types of array to perform a slightly different task in our testbench. We discuss all three of these arrays in more detail int he rest of this post
SystemVerilog Dynamic Arrays
SystemVerilog dynamic arrays are a special class of array which can be resized whilst a simulation is running.
This differentiates them from static arrays which are allocated memory during compile time and have a fixed sized for the duration of a simulation.
This is useful as we may have instances where we don't know exactly how many elements our array will require when we compile our code.
The code snippet below shows how we declare a dynamic array in SystemVerilog.
When we create a dynamic array, we must always declare it as an unpacked type array .
As dynamic arrays are initially empty, we have to use the new keyword to allocate memory to the array before we can use it. We also declare the number of elements in the array when we use the new keyword.
The code example below shows how we would declare a dynamic array and then allocate the memory for 4 elements.
Dynamic Array Methods
Dynamic arrays are slightly more complex to work with than static arrays as we have to manage the size of the array in our code.
As a result of this, a number of methods are included in the SystemVerilog to help us manage dynamic arrays.
We have already seen one of the most important of these methods in the previous section - the new method.
In addition to this, we also commonly use the delete and size methods to manage our dynamic arrays.
Let's take a closer a look at each of these methods in more detail.
- The new Method
As we have previously seen, we use the new method to allocate memory to our dynamic array.
The code snippet below shows the general syntax we use to call the new method.
The <size> field in this construct is used to specify how many elements there will be in the array.
We use the <values> field to assign values to the array after it has allocated memory. We can exclude this field if we are creating an empty array.
For example, we would use the code shown below to allocate memory for 8 elements in a dynamic array.
We can call the new method as many times as necessary in our code. Each time we call the new method, we effectively create a new array and allocate memory to it.
For example, we might need to replace our array of 8 elements with a new array which has 16 elements. In this case, we would simply call the new method a second time to resize our array.
The code snippet below shows how we would use the new method to do this.
When we use the new method in this way, any contents which were in the old array are deleted. This means that we create an entirely new, empty array when using this method.
However, we can also keep the existing contents of our array when we call the new method.
To do this, we need to pass the existing array to the new method using the <value> field we mentioned before. This will place the contents of the old array at the start of the resized array.
For example, suppose that we had created a dynamic array which consists of 8 elements and assigned some data to it.
If we now wanted to resize the array to 16 elements and keep the existing data, we could do this using the code shown below. This code can also be simulated on eda playground .
- The delete Method
We use the delete method to remove the entire contents of a dynamic array.
When we call this method, it not only deletes the contents of the dynamic array but also deallocate the memory. In this sense, we can consider the delete method to be roughly equivalent to the free function in C .
The code snippet below shows the general syntax of the delete method.
In this construct, the <name> field is used to identify the dynamic array which we are calling the method on.
The SystemVerilog code below shows how we would use the delete method in practise. This code can also be simulated on eda playground .
- The size Method
We use the size method to determine how large our dynamic array is at any given time.
When we call this method it returns a value which is equal to the number of elements in an array.
As we can see from this, it performs a similar function to the $size macro which we mentioned in the post on static arrays.
The code snippet below shows the general syntax for this method.
The SystemVerilog code below shows how we would use the size method in practise. This code can also be simulated on eda playground .
SystemVerilog Queues
SystemVerilog queues are a type of array which are automatically resized when we add or remove elements to the array.
The code snippet below shows how we would declare a queue type in SystemVerilog.
In this construct, we use the $ symbol inside of square brackets to indicate that we are creating a queue type.
We use the optional <max_length> field to limit the amount of elements which a queue can have.
However, we often exclude the <max_length> field from our declaration. When we do this, our queue can contain an unlimited amount of elements.
Queues which have an unlimited amount of elements are known as unbounded arrays whilst queues which are declared using the <max_length> field are known as bounded arrays.
When we declare a queue we can initialize it using a comma separated list of values between a pair of curly braces. This is similar to the use of array literals which we discussed in the previous post.
The SystemVerilog code below shows how we declare both a bounded and an unbounded queue. We also initialize both of the example queues with 2 elements.
- Dynamic Arrays vs Queues
Dynamic arrays and queues actually perform very similar functions in SystemVerilog as they are both allocated memory at run time. As a result of this, we can resize both of these data structures whilst our code is running.
However, we use them for different purposes as they are optimized for slightly different operations.
We use queues when we are only interested in adding or removing elements at the beginning or end of the array.
The reason for this is that dynamic arrays are stored in contiguous memory addresses during simulation. As a result of this, when we resize a dynamic array it is often necessary for the entire array to be moved to a new location in memory.
In contrast, SystemVerilog queues are implemented in a similar way to linked lists in other programming languages.
This means that it is much quicker to add or remove elements to a queue as there is no need to move the existing elements in the array.
We typically use SystemVerilog queues to emulate either FIFO or LIFO type memories. We often see queues used in this way to move transactions between different blocks in a SystemVerilog based testbench.
However, when we want to access elements in the middle of the data structure then dynamic arrays are more efficient
The reason for this is that our simulator must start from either the beginning or end of the queue and loop through the memory until it reaches the required element. Again, this behavior is similar to linked lists in other programming languages.
In contrast, our simulator can directly access any element in dynamic array.
As we can see from this, it is clearly much quicker for our simulator to retrieve data from the middle of a dynamic array as less memory accesses are required.
Queue Methods
SystemVerilog queues are more complex that static arrays due to the fact that they require dynamic memory allocation .
As a result of this, we have a number of in built methods which can use to manipulate the contents of our queue.
This is in contrast to arrays where we can directly access individual elements to manipulate the contents of the array.
Let's take a closer a look at the most important queue methods in SystemVerilog.
- Push to Queue
When we want to add data to a SystemVerilog queue, we can use either the push_front or the push_back method.
The push_front method inserts the specified data onto the front of the queue whilst the push_back method inserts the data at the end fo the queue.
We can think of the front of the queue as being equivalent to the lowest indexed element of a normal array.
In contrast, when we talk about the back of a queue this is equivalent to the highest indexed element of a normal array type.
The picture below illustrates the concept of push_front and push_back queue methods.
The code snippet below shows the general syntax we use to call the push_front and push_back methods.
In this construct we use the <queue_name> field to identify the queue we are adding data to.
We then use the <value> field to specify the value of the data which we are adding to the queue. We can use either a hard coded value or a variable to add data to our queue.
The SystemVerilog code below shows how we use the push_front and push_back methods in practise. This code can also be simulated on eda playground .
- Pop From Queue
When we want to get some data from a SystemVerilog queue we use either the pop_front or pop_back methods.
The pop_front method retrieves data from the front of the queue whilst the pop_back method retrieves the data at the end fo the queue.
As with push_front method, the front of the queue is equivalent to the lowest indexed element of a normal array.
In constrast, the back of a queue is equivalent to the highest indexed element of a normal array type.
When we call either of these methods, the required element is removed from the queue and the value of this element is returned by the function.
The picture below illustrates the concept of pop_front and pop_back queue methods.
The code snippet below shows the general syntax we use to call the pop_front and pop_back methods.
In this construct we use the <queue_name> field to identify the queue we are retrieving data from.
The SystemVerilog code below shows how we use the pop_front and pop_back methods in practise. We can also simulate this code on eda playground .
- Insert and Delete Methods
We can also use the insert and delete methods to add or remove an element in a SystemVerilog queue.
In contrast to the push and pop methods, we use these two methods to add or remove elements at a specific location in the queue.
As a result, we can use these methods to modify elements in the middle of our array.
However, we should be aware that these methods are less efficient than the push and pop methods. In addition, they can also be inefficient in comparison to the equivalent methods in dynamic arrays.
In fact, when we make extensive use of the insert or delete methods this may be a good indication that we should be using a dynamic array instead.
The code snippet below shows the general syntax we use to call the insert and delete methods.
In this construct we use the <queue_name> field to identify the queue which we are modifying.
We use the <value> field to specify the value of the data which we are adding to the queue. We can use either a hard coded value or a variable to add data to our queue.
In the case of the insert method, we use the <index> field to specify where the new element will be inserted in our queue.
In the case of the delete method, we use the <index> field to specify which element of the queue will be removed.
However, we can omit the <index> field when we use the delete method. When we do this, the entire content of our queue will be deleted.
The SystemVerilog code below shows how we use the insert and delete methods in practise. This code can also be simulated on eda playground.
SystemVerilog Associative Arrays
The final type of array which we can use in SystemVerilog is the associative array.
When we declare an associative array, memory for the array is not allocated at compile time and we can resize the array during simulation.
Therefore, associative arrays are very similar to the dynamic arrays which we discussed previously in this post.
However, the way that we index associative arrays is different from the way that we index dynamic arrays.
As we previously saw, we use sequential integers to index different elements of a dynamic array.
In contrast, we can use any value that we want to index the different elements of an associative array in SystemVerilog.
For example, we could use non sequential integers if we wanted to model the contents of a sparsely populated memory.
However, we could also use a string to give a physical name to the indexes in an associative array.
As we can see from this, we can think of associative arrays as being roughly equivalent to key-value pairs in other programming languages.
The SystemVerilog below shows the general syntax we use to declare an associative array.
When we use associative arrays, we must use the same data type for all of the indexes. This means that we can't mix int types and strings, for example.
We use the <key_type> field to declare what data type we will use for the index.
When we use associative arrays in our SystemVerilog code, the simulator has to search for the memory location of each element in the array when we either read or write to it.
As a result of this, associative arrays are less efficient than both static and dynamic arrays. This normally results in slowly execution times for our test benches.
Therefore, we generally prefer to use either a static or dynamic array instead of associative arrays whenever it is possible.
- Assigning Data to an Associative Array
We can assign values to an associative array using the same techniques which we discussed in the previous post.
However, when we use array literals to we have to specify not only the data which we are assigning but also the value of the index we want associated with that data.
The SystemVerilog code below shows the general syntax we use to assign data to an associative array using array literals.
In this construct, we use the <key> field to set the value of the index in the array. The <data> field is then used to set the value of the data which is written to the array.
We can also use square brackets to access elements in an associative array. The syntax for this is exactly the same as we use for both static and dynamic arrays.
The SystemVerilog code below shows how we use associative arrays in practise. This code can also be simulated on eda playground .
What is the difference between static arrays and dynamic arrays in SystemVerilog?
Static arrays have a fixed number of elements which are determined at compile time. In contrast, dynamic arrays don't have a fixed sized and we can add or remove elements during simulation.
Write the code to declare a dynamic array of int types and allocate the memory for 8 elements
Which method would we use to deallocate the memory of a dynamic array?
We use the delete method to discard the contents of a dynamic array and dellocate any memory associated with it.
Write some code which creates a queue of int types and initializes 3 elements to 0.
When would we use queues instead of dynamic arrays in SystemVerilog? Why would we use queues in this circumstance.
We use queues when we only want to add or remove data from the beginning or end of the array. The reason for this is that it is more efficient to resize queues than it is to resize dynamic arrays. However, it is more efficient to access elements in the middle of a dynamic array.
Write some code which declares an associative array of int types and uses string type indexes.
2 comments on “SystemVerilog Dynamic Arrays and Queues”
nice post !please keep up good work ! Thank you.
In the " insert and delete"section about
// Create a queue with some initial values in it int example_q [$] = { 1, 2 }; // Insert a value into the
the code example is no complete ?
Hi Kaia, Thanks for pointing that out, I have updated the post so that the example is compete now.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
- Learning Materials and Initial Setup
- Module – sc_module
- Time, Events and Processes
- Primitive Channels
- Signal Channels
- Module Hierarchy And Connectivity
SystemVerilog: Tips And Tricks When Working With Queues
In this post I put together a few tips and tricks I’ve came across over the years related to SystemVerilog queues.
Access the last element of a queue
Sorting a queue.
When I had to sort some queue the first option in my head was to implement some simple algorithm like Bubble Sort . However, SystemVerilog queue comes with a useful and flexible function called sort() . For example if you want to sort a queue of integers in ascending order then you simply call the function sort() . For reverse sorting there is a similar function called rsort() :
Which produces the output:
But the big flexibility of these rsort() and sort() functions is in their “ with ” clause and this is best emphasize when we have to sort a queue of objects based on some field from the object’s class. Let’s consider a simple class containg some basic information about a player:
Sorting a queue of players by score can be done using just one simple line of code:
The output is this:
You can try the example yourself on EDA Playground .
Searching a queue
An other extremely useful feature of the queues is their search capability. The queues have some build-in functions which makes searching very easy and all of them have this with clause. As in the previous example, we have a queue of players, but this time let’s make the list of players a little bit larger:
Searching all the players which score equal to 5 is just one line of code:
The result is this:
There are other similar functions very useful for searching, and all have this very useful with clause:
- find() – returns all the elements satisfying the given expression
- find_index() – returns the indexes of all the elements satisfying the given expression
- find_first() – returns the first element satisfying the given expression
- find_first_index() – returns the index of the first element satisfying the given expression
- find_last() – returns the last element satisfying the given expression
- find_last_index() – returns the index of the last element satisfying the given expression
You can try this example in EDA Playground .
That’s it! If you have other tips that you want to share feel free to add them in a comment below.
Cristian Slav
HI Cristian,
Nice and concise article as always I’d add the (in)famous sum() method along with the “with” clause for example
int x[$] = ‘{1,4,17,3,10,55,50,10,3}; int z;
z=x.sum(); $display(“sum()=%0d”,z); //you get 192 as result
z=numbers.sum(it) with (it>6); $display(“sum with (it>6)=%0d”, z); //you get 0 as result
Depending on what you want you need to do some changes
a- Counting of then number of elements greater than 6 (note the cast to int is required to force the result as the it>6 returns a 1-bit result)
z=x.sum() with (int'(it>6)); $display(“Count the number of elements that are > 6 =%0d”, z);
b- Sum of elements whose values are greater than 6
z=x.sum() with (it>6?it:0); $display(“Sum the elements that are > 6 = %0d”, z);
That’s a great example! Thanks for sharing it!
Hi Cristian Slav, I’m so happy for this article because I gained something from it. players.sort(p) with (p.score); In the above mentioned statement, ‘p’ indicates the handle for the queue of objects which are all the types of class player. Please let me know if i’m wrong and please give me some description on this. Thanks & Regards, Prathyusha
Hi Cristian Slav, Could you please give me some description for this “players.sort(p) with (p.score)”. I understood in this way, where ‘p’ is the handle for the queue of objects(players) which are all are type of class player. Please let me know if i’m wrong.
Thanks & Regards, Prathyusha Gadanchi
Leave a Reply Cancel Reply
Your email address will not be published.
© Copyright 2015 Cristian Slav
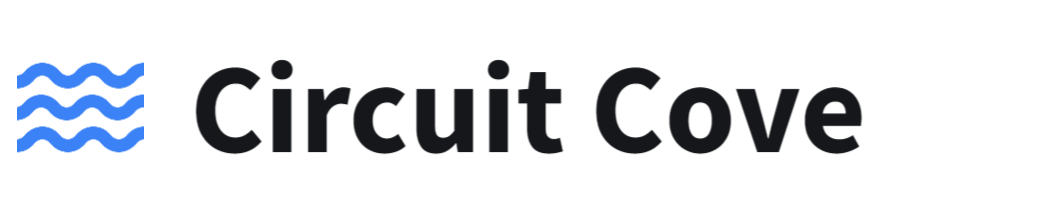
Exploring SystemVerilog Queues: A Comprehensive Guide
SystemVerilog is a robust hardware description and verification language that enables designers to develop and examine digital systems. One of the critical data structures available in SystemVerilog is the queue . In this guide, we'll cover the fundamentals of queues in SystemVerilog, learn about their operators and methods, discuss real-world applications, and examine some practical examples.
Understanding Queues in SystemVerilog
Defining queues.
A queue in SystemVerilog is a dynamic, ordered set of homogeneous elements that allow quick access, insertion, and deletion. To define a queue, we apply the same syntax as unpacked arrays, but replace the array size with $ . Here's a straightforward example of defining a queue of integers:
Initializing Queues
You can initialize queues using assignment patterns or unpacked array concatenations. For instance, this is how to initialize a queue with some integer values:
Queue Operations
SystemVerilog queues provide various operators to access and modify their elements.
Element Access
You can access elements in a queue using the indexing operator, as shown below:
Queue Slicing
Queues also support slicing, which enables you to generate a new queue from a range of elements within the original queue:
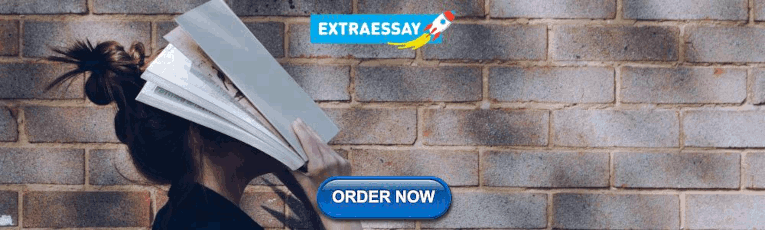
Comparing Queues
You can use equality operators to compare two queues:
Built-in Queue Methods
Alongside operators, SystemVerilog queues provide various built-in methods to manage their elements.
The size() method determines the number of elements in a queue:
The insert() method adds a specified element at a particular index within the queue:
The delete() method can either remove all elements in a queue or eliminate an element at a specific index:
Pop_front()
The pop_front() method extracts and returns the first element of the queue:
The pop_back() method extracts and returns the last element of the queue:
Push_front()
The push_front() method adds a specified element to the beginning of the queue:
Push_back()
The push_back() method adds a specified element to the end of the queue:
Managing Queue References
It is essential to understand how queue element references can be affected by various operations while working with SystemVerilog queues.
Persistent References
A reference to a queue element remains valid as long as the element is not removed or the entire queue is not replaced.
Outdated References
Some queue operations, like assignment or using methods like delete() , pop_front() , and pop_back() , may cause references to become outdated, and using them could result in unpredictable behavior. Therefore, it's crucial to ensure that you're working with valid references after performing such operations.
Real-World Examples and Applications of Queues in SystemVerilog
Queues are versatile and can be applied in numerous SystemVerilog use cases. Some common examples include:
Transaction buffers
Queues can be used to store and manage transactions between modules in a verification environment, allowing you to control the order of transaction execution and maintain a flexible buffer size.
Pipeline modeling
Queues can be employed to model pipeline stages in a design, providing an efficient method for handling data flow within the pipeline and ensuring the correct timing between stages.
Testcase configuration
In testbench environments, queues can help manage test configurations, enabling you to run various test scenarios by modifying a queue's contents dynamically.
By mastering SystemVerilog queues, you'll be well-equipped to handle various design and verification challenges that require dynamic, ordered data structures. Experiment with the examples provided and apply queues to your unique use cases to make the most of this powerful feature in SystemVerilog.
- Randomization
- Random Stability
- String Methods
- Convert hex, int, bin to string
- Convert string to hex, int, bin
- 10 Useful Utilities
SystemVerilog Queues Cheatsheet ¶
Example code on EDA Playground
Initialize queue ¶
Size of queue ¶, push into queue ¶, pop from queue ¶, insert and delete ¶, function to print queue contents in a single line ¶.
If you would like to be notified when a new article is published, please sign up. If you found this content useful then please consider supporting this site! 🫶
Sign-up for the Newsletter
Every month or so I send out a newsletter with notable technical papers, notifications about new articles and lessons from my experience.
If you found this content useful then please consider supporting this site! 🫶
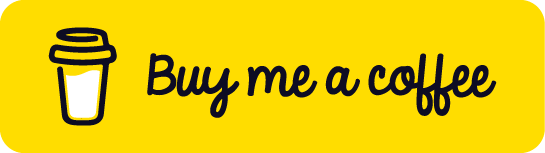
Very Large Scale Integration (VLSI)
VLSI Encyclopedia - Connecting VLSI Engineers
Featured post
Top 5 books to refer for a vhdl beginner.
VHDL (VHSIC-HDL, Very High-Speed Integrated Circuit Hardware Description Language) is a hardware description language used in electronic des...
Thursday 29 January 2015
Systemverilog queue.
- The size() method returns the number of items in the queue. If the queue is empty, it returns 0.
- The insert() method inserts the given item at the specified index position.
- The delete() method deletes the item at the specified index.
- The pop_front() method removes and returns the first element of the queue.
- The pop_back() method removes and returns the last element of the queue.
- The push_front() method inserts the given element at the front of the queue.
- The push_back() method inserts the given element at the end of the queue.
No comments:
Post a comment.
Please provide valuable comments and suggestions for our motivation. Feel free to write down any query if you have regarding this post.
- Understanding real, realtime and shortreal variables of SystemVerilog This post will help you to understand the difference between real, realtime and shortreal data types of SystemVerilog and its usage. ...

- SystemVerilog Constants In previous post of this SystemVerilog Tutorial we talked about enumerated type in detail. Now we will look at the constants in SystemVe...
Event Queue and Design Guidelines
- First Online: 01 November 2021
Cite this chapter
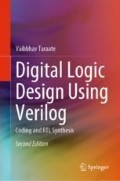
- Vaibbhav Taraate 2
2031 Accesses
It is essential to follow the coding and design guidelines while coding the RTL design. The design and coding guidelines will improve the performance of design, readability, and reusability. This chapter discusses few important design and coding guidelines for the combinational logic design.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
- Durable hardcover edition
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Author information
Authors and affiliations.
1 Rupee S T (Semiconductor Training @ Rs.1), Pune, Maharashtra, India
Vaibbhav Taraate
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to Vaibbhav Taraate .
Rights and permissions
Reprints and permissions
Copyright information
© 2022 The Author(s), under exclusive license to Springer Nature Singapore Pte Ltd.
About this chapter
Taraate, V. (2022). Event Queue and Design Guidelines. In: Digital Logic Design Using Verilog. Springer, Singapore. https://doi.org/10.1007/978-981-16-3199-3_7
Download citation
DOI : https://doi.org/10.1007/978-981-16-3199-3_7
Published : 01 November 2021
Publisher Name : Springer, Singapore
Print ISBN : 978-981-16-3198-6
Online ISBN : 978-981-16-3199-3
eBook Packages : Engineering Engineering (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
Verilog Assignments
Variable declaration assignment, net declaration assignment, assign deassign, force release.
- Procedural continuous
Legal LHS values
An assignment has two parts - right-hand side (RHS) and left-hand side (LHS) with an equal symbol (=) or a less than-equal symbol (<=) in between.
The RHS can contain any expression that evaluates to a final value while the LHS indicates a net or a variable to which the value in RHS is being assigned.
Procedural Assignment
Procedural assignments occur within procedures such as always , initial , task and functions and are used to place values onto variables. The variable will hold the value until the next assignment to the same variable.
The value will be placed onto the variable when the simulation executes this statement at some point during simulation time. This can be controlled and modified the way we want by the use of control flow statements such as if-else-if , case statement and looping mechanisms.
An initial value can be placed onto a variable at the time of its declaration as shown next. The assignment does not have a duration and holds the value until the next assignment to the same variable happens. Note that variable declaration assignments to an array are not allowed.
If the variable is initialized during declaration and at time 0 in an initial block as shown below, the order of evaluation is not guaranteed, and hence can have either 8'h05 or 8'hee.
Procedural blocks and assignments will be covered in more detail in a later section.
Continuous Assignment
This is used to assign values onto scalar and vector nets and happens whenever there is a change in the RHS. It provides a way to model combinational logic without specifying an interconnection of gates and makes it easier to drive the net with logical expressions.
Whenever b or c changes its value, then the whole expression in RHS will be evaluated and a will be updated with the new value.
This allows us to place a continuous assignment on the same statement that declares the net. Note that because a net can be declared only once, only one declaration assignment is possible for a net.
Procedural Continuous Assignment
- assign ... deassign
- force ... release
This will override all procedural assignments to a variable and is deactivated by using the same signal with deassign . The value of the variable will remain same until the variable gets a new value through a procedural or procedural continuous assignment. The LHS of an assign statement cannot be a bit-select, part-select or an array reference but can be a variable or a concatenation of variables.
These are similar to the assign - deassign statements but can also be applied to nets and variables. The LHS can be a bit-select of a net, part-select of a net, variable or a net but cannot be the reference to an array and bit/part select of a variable. The force statment will override all other assignments made to the variable until it is released using the release keyword.
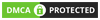
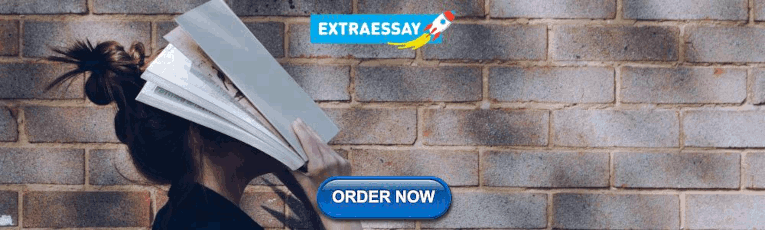
IMAGES
VIDEO
COMMENTS
Queue in SystemVerilog. A queue is a variable-size, ordered collection of homogeneous elements. Queues are declared using the same syntax as unpacked arrays, but specifying $ as the array size. In queue 0 represents the first, and $ representing the last entries. A queue can be bounded or unbounded.
SystemVerilog Queue methods are several built-in methods in addition to array operators to operate on the queue and are listed in the table below. Methods. Description. function int size (); Returns the number of items in the queue, 0 if empty. function void insert (input integer index, input element_t item);
SystemVerilog Queues. A queue is a variable size and ordered collection of elements (homogeneous element). To understand it is considered the same as a single-dimensional unpacked array that grows and reduces automatically if it is a bounded queue.
A queue in SystemVerilog is a variable-size, ordered collection of homogeneous elements. "Variable size" means adding or removing elements as needed without specifying a fixed size in advance. "Ordered collection" indicates that the elements are stored in the order in which they were added, following a FIFO (First-In-First-Out) order.
Queues. In SystemVerilog, queues provide a powerful and flexible way to manage collections of data. They are similar to dynamic arrays in the sense that they can grow and shrink during runtime. However, queues offer additional capabilities that make them even more flexible and useful in a range of scenarios.
The SystemVerilog code below shows how we would use the size method in practise. This code can also be simulated on eda playground. // Declaration of the example array. logic [7:0] example []; // Allocate memory for 8 elements. example = new[8]; // Use the size method to get the number of elements. example.size();
1. my_value = my_queue [ my_queue [ my_queue. size() - 1]] However I just recently learned that I can use this very short syntax to get the last element of a queue: 1. my_value = my_queue [ $] You can even do some arithmetic operation with that $ symbol to get for example the second to last element: 1. my_value = my_queue [ $ - 1] Try it ...
SystemVerilog is a robust hardware description and verification language that enables designers to develop and examine digital systems. One of the critical data structures available in SystemVerilog is the queue.In this guide, we'll cover the fundamentals of queues in SystemVerilog, learn about their operators and methods, discuss real-world applications, and examine some practical examples.
Reference examples for SystemVerilog Queues. SystemVerilog SystemVerilog Queues Cheatsheet¶. Example code on EDA Playground
Queue Design in SystemVerilog: Entry is stored into the Queue in a certain order. The order could be as simple as find any first vacant entry or find a next vacant entry from previous allocation or find the last entry that became available recently. Queues are used in Digital design when the Data from a Stream is needed to be stored into a ...
SystemVerilog Queue. Queue is a variable size, ordered collection of homogeneous elements which can grow and shrink. The size of a queue is variable similar to a dynamic array, but a queue may be empty with no element and it is still a valid data structure. Queues can be used as LIFO (Last In First Out) Buffer or FIFO (First In First Out) type ...
In reply to mittal: You can just make an assignment from queue to dynamic array, and the dynamic array takes on the size of the queue. mon_tran.data=data_que; If you mean to say that your are randomizing the dynamic array with a size larger than the queue, and want to insert the queue into the dynamic array leaving the additional elements with ...
@TudorTimi, SystemVerilog tried to do everything just using the concatenation syntax first. Then ambiguities arose in certain cases when trying to determine if each operand in the concatenation {operand1,operand2,operand3} was to be evaluated in a self-determined context (like in a Verilog Concatenation) or in the context of an assignment (like in an assignment pattern).
Blocking vs. Nonblocking Assignments • Verilog supports two types of assignments within always blocks, with subtly different behaviors. • Blocking assignment (=): evaluation and assignment are immediate ... Section 5.3 The stratified event queue The Verilog event queue is logically segmented into five different regions. Events
SystemVerilog offers much flexibility in building complicated data structures through the different types of arrays. Static Arrays Dynamic Arrays Associative Arrays Queues Static Arrays A static array is one whose size is known before compilation time. In the example shown below, a static array of 8-.
7.1 Verilog Stratified Event Queue. Verilog supports the two kinds of the assignments in the procedural blocks. These assignments are named as blocking (=) and non-blocking (<=) assignments. It is always recommended to use the blocking assignments while describing the combinational logic design. The reason being quite simple to understand, but ...
This is used to assign values onto scalar and vector nets and happens whenever there is a change in the RHS. It provides a way to model combinational logic without specifying an interconnection of gates and makes it easier to drive the net with logical expressions. // Example model of an AND gate. wire a, b, c;
Hope somebody can help me with what on the face of it is very simple. I've been doing SystemVerilog for a total of four days now and my first task is to create an array of queues. I've created with… int fred [4][$] //4 wide array of queues of int Now to write to one queue I tried using… fred[2].push_back(77) to push the integer 77 into queue 2. Questa does not like the "[2]". Thanks.
5. I have primarily a C++ background. I was tracking down a bug in some SystemVerilog code I am working on and was surprised to find what I thought was an object-copying assignment was actually a reference assignment. This simplified code shows what I mean: var cls_obj obj1; obj1 = obj_array[i];