- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
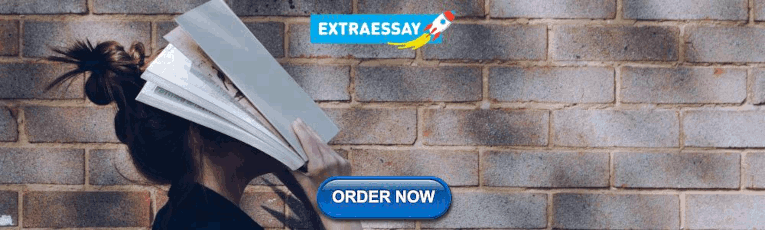
Assignment Operators in Programming
- Operator Associativity in Programming
- C++ Assignment Operator Overloading
- What are Operators in Programming?
- Assignment Operators In C++
- Types of Operators in Programming
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- JavaScript Assignment Operators
- How to Create Custom Assignment Operator in C++?
- Assignment Operators in Python
- Assignment Operators in C
- Compound assignment operators in Java
- Java Assignment Operators with Examples
- When should we write our own assignment operator in C++?
- Parallel Assignment in Ruby
- Self assignment check in assignment operator
- Different Forms of Assignment Statements in Python
- What is the difference between = (Assignment) and == (Equal to) operators
- Rules for operator overloading
- Program to print ASCII Value of a character
- Introduction of Programming Paradigms
- Program for Hexadecimal to Decimal
- Program for Decimal to Octal Conversion
- A Freshers Guide To Programming
- ASCII Vs UNICODE
- How to learn Pattern printing easily?
- Loop Unrolling
- How to Learn Programming?
- Program to Print the Trapezium Pattern
Assignment operators in programming are symbols used to assign values to variables. They offer shorthand notations for performing arithmetic operations and updating variable values in a single step. These operators are fundamental in most programming languages and help streamline code while improving readability.
Table of Content
What are Assignment Operators?
- Types of Assignment Operators
- Assignment Operators in C++
- Assignment Operators in Java
- Assignment Operators in C#
- Assignment Operators in Javascript
- Application of Assignment Operators
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign ( = ), which assigns the value on the right side of the operator to the variable on the left side.
Types of Assignment Operators:
- Simple Assignment Operator ( = )
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
Below is a table summarizing common assignment operators along with their symbols, description, and examples:
Assignment Operators in C:
Here are the implementation of Assignment Operator in C language:
Assignment Operators in C++:
Here are the implementation of Assignment Operator in C++ language:
Assignment Operators in Java:
Here are the implementation of Assignment Operator in java language:
Assignment Operators in Python:
Here are the implementation of Assignment Operator in python language:
Assignment Operators in C#:
Here are the implementation of Assignment Operator in C# language:
Assignment Operators in Javascript:
Here are the implementation of Assignment Operator in javascript language:
Application of Assignment Operators:
- Variable Initialization : Setting initial values to variables during declaration.
- Mathematical Operations : Combining arithmetic operations with assignment to update variable values.
- Loop Control : Updating loop variables to control loop iterations.
- Conditional Statements : Assigning different values based on conditions in conditional statements.
- Function Return Values : Storing the return values of functions in variables.
- Data Manipulation : Assigning values received from user input or retrieved from databases to variables.
Conclusion:
In conclusion, assignment operators in programming are essential tools for assigning values to variables and performing operations in a concise and efficient manner. They allow programmers to manipulate data and control the flow of their programs effectively. Understanding and using assignment operators correctly is fundamental to writing clear, efficient, and maintainable code in various programming languages.
Please Login to comment...
Similar reads.
- Programming
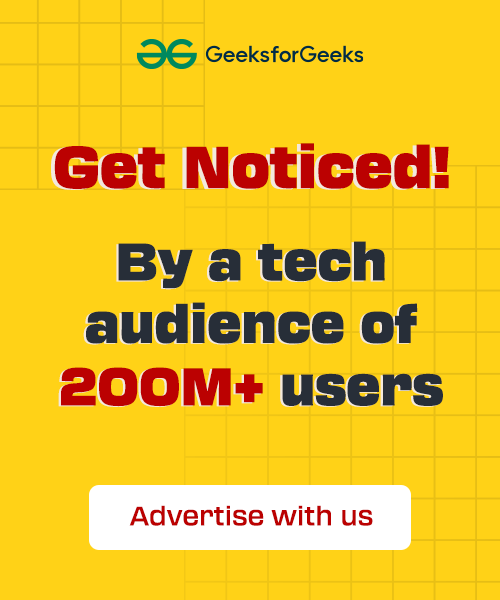
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable or an expression. The value to be assigned forms the right hand operand, whereas the variable to be assigned should be the operand to the left of = symbol, which is defined as a simple assignment operator in C. In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple assignment operator (=)
The = operator is the most frequently used operator in C. As per ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed. You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented assignment operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression a+=b has the same effect of performing a+b first and then assigning the result back to the variable a.
Similarly, the expression a<<=b has the same effect of performing a<<b first and then assigning the result back to the variable a.
Here is a C program that demonstrates the use of assignment operators in C:
When you compile and execute the above program, it produces the following result −
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Assignment Operators
- 6 contributors
An assignment operation assigns the value of the right-hand operand to the storage location named by the left-hand operand. Therefore, the left-hand operand of an assignment operation must be a modifiable l-value. After the assignment, an assignment expression has the value of the left operand but isn't an l-value.
assignment-expression : conditional-expression unary-expression assignment-operator assignment-expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators:
In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place. The left operand must not be an array, a function, or a constant. The specific conversion path, which depends on the two types, is outlined in detail in Type Conversions .
- Assignment Operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
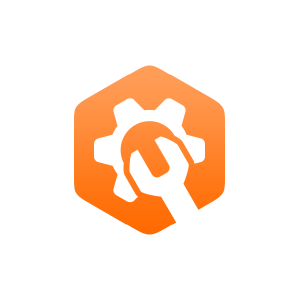
4.6: Assignment Operator
- Last updated
- Save as PDF
- Page ID 29038
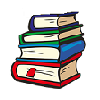
- Patrick McClanahan
- San Joaquin Delta College
Assignment Operator
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would assigned to the variable named: total_cousins.
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
As we have seen, assignment operators are used to assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error. Different types of assignment operators are shown below:
- “=” : This is the simplest assignment operator, which was discussed above. This operator is used to assign the value on the right to the variable on the left. For example: a = 10; b = 20; ch = 'y';
If initially the value 5 is stored in the variable a, then: (a += 6) is equal to 11. (the same as: a = a + 6)
If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
If initially value 5 is stored in the variable a,, then (a *= 6) is equal to 30. (the same as a = a * 6)
If initially value 6 is stored in the variable a, then (a /= 2) is equal to 3. (the same as a = a / 2)
Below example illustrates the various Assignment Operators:
Definitions
Adapted from: "Assignment Operator" by Kenneth Leroy Busbee , (Download for free at http://cnx.org/contents/[email protected] ) is licensed under CC BY 4.0
Home » Learn C Programming from Scratch » C Assignment Operators
C Assignment Operators
Summary : in this tutorial, you’ll learn about the C assignment operators and how to use them effectively.
Introduction to the C assignment operators
An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
After the assignmment, the counter variable holds the number 1.
The following example adds 1 to the counter and assign the result to the counter:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand.
Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
The following example uses a compound-assignment operator (+=):
The expression:
is equivalent to the following expression:
The following table illustrates the compound-assignment operators in C:
- A simple assignment operator assigns the value of the left operand to the right operand.
- A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 08:36.
- This page has been accessed 54,567 times.
- Privacy policy
- About cppreference.com
- Disclaimers

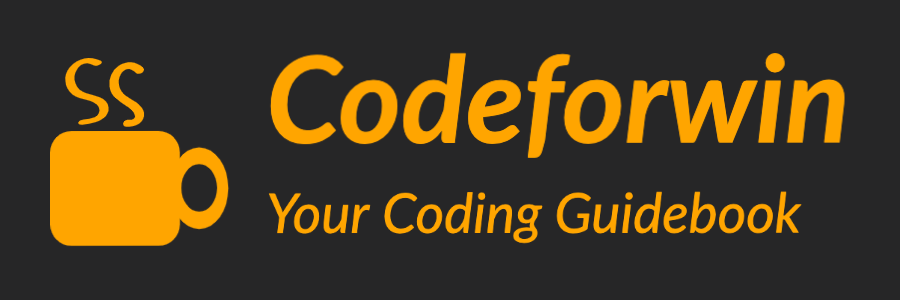
Assignment and shorthand assignment operator in C
Quick links.
- Shorthand assignment
Assignment operator is used to assign value to a variable (memory location). There is a single assignment operator = in C. It evaluates expression on right side of = symbol and assigns evaluated value to left side the variable.
For example consider the below assignment table.
The RHS of assignment operator must be a constant, expression or variable. Whereas LHS must be a variable (valid memory location).
Shorthand assignment operator
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator.
For example, consider following C statements.
The above expression a = a + 2 is equivalent to a += 2 .
Similarly, there are many shorthand assignment operators. Below is a list of shorthand assignment operators in C.
C Programming Tutorial
- Assignment Operator in C
Last updated on July 27, 2020
We have already used the assignment operator ( = ) several times before. Let's discuss it here in detail. The assignment operator ( = ) is used to assign a value to the variable. Its general format is as follows:
The operand on the left side of the assignment operator must be a variable and operand on the right-hand side must be a constant, variable or expression. Here are some examples:
The precedence of the assignment operator is lower than all the operators we have discussed so far and it associates from right to left.
We can also assign the same value to multiple variables at once.
here x , y and z are initialized to 100 .
Since the associativity of the assignment operator ( = ) is from right to left. The above expression is equivalent to the following:
Note that expressions like:
are called assignment expression. If we put a semicolon( ; ) at the end of the expression like this:
then the assignment expression becomes assignment statement.
Compound Assignment Operator #
Assignment operations that use the old value of a variable to compute its new value are called Compound Assignment.
Consider the following two statements:
Here the second statement adds 5 to the existing value of x . This value is then assigned back to x . Now, the new value of x is 105 .
To handle such operations more succinctly, C provides a special operator called Compound Assignment operator.
The general format of compound assignment operator is as follows:
where op can be any of the arithmetic operators ( + , - , * , / , % ). The above statement is functionally equivalent to the following:
Note : In addition to arithmetic operators, op can also be >> (right shift), << (left shift), | (Bitwise OR), & (Bitwise AND), ^ (Bitwise XOR). We haven't discussed these operators yet.
After evaluating the expression, the op operator is then applied to the result of the expression and the current value of the variable (on the RHS). The result of this operation is then assigned back to the variable (on the LHS). Let's take some examples: The statement:
is equivalent to x = x + 5; or x = x + (5); .
Similarly, the statement:
is equivalent to x = x * 2; or x = x * (2); .
Since, expression on the right side of op operator is evaluated first, the statement:
is equivalent to x = x * (y + 1) .
The precedence of compound assignment operators are same and they associate from right to left (see the precedence table ).
The following table lists some Compound assignment operators:
The following program demonstrates Compound assignment operators in action:
Expected Output:
Load Comments
- Intro to C Programming
- Installing Code Blocks
- Creating and Running The First C Program
- Basic Elements of a C Program
- Keywords and Identifiers
- Data Types in C
- Constants in C
- Variables in C
- Input and Output in C
- Formatted Input and Output in C
- Arithmetic Operators in C
- Operator Precedence and Associativity in C
- Increment and Decrement Operators in C
- Relational Operators in C
- Logical Operators in C
- Conditional Operator, Comma operator and sizeof() operator in C
- Implicit Type Conversion in C
- Explicit Type Conversion in C
- if-else statements in C
- The while loop in C
- The do while loop in C
- The for loop in C
- The Infinite Loop in C
- The break and continue statement in C
- The Switch statement in C
- Function basics in C
- The return statement in C
- Actual and Formal arguments in C
- Local, Global and Static variables in C
- Recursive Function in C
- One dimensional Array in C
- One Dimensional Array and Function in C
- Two Dimensional Array in C
- Pointer Basics in C
- Pointer Arithmetic in C
- Pointers and 1-D arrays
- Pointers and 2-D arrays
- Call by Value and Call by Reference in C
- Returning more than one value from function in C
- Returning a Pointer from a Function in C
- Passing 1-D Array to a Function in C
- Passing 2-D Array to a Function in C
- Array of Pointers in C
- Void Pointers in C
- The malloc() Function in C
- The calloc() Function in C
- The realloc() Function in C
- String Basics in C
- The strlen() Function in C
- The strcmp() Function in C
- The strcpy() Function in C
- The strcat() Function in C
- Character Array and Character Pointer in C
- Array of Strings in C
- Array of Pointers to Strings in C
- The sprintf() Function in C
- The sscanf() Function in C
- Structure Basics in C
- Array of Structures in C
- Array as Member of Structure in C
- Nested Structures in C
- Pointer to a Structure in C
- Pointers as Structure Member in C
- Structures and Functions in C
- Union Basics in C
- typedef statement in C
- Basics of File Handling in C
- fputc() Function in C
- fgetc() Function in C
- fputs() Function in C
- fgets() Function in C
- fprintf() Function in C
- fscanf() Function in C
- fwrite() Function in C
- fread() Function in C
Recent Posts
- Machine Learning Experts You Should Be Following Online
- 4 Ways to Prepare for the AP Computer Science A Exam
- Finance Assignment Online Help for the Busy and Tired Students: Get Help from Experts
- Top 9 Machine Learning Algorithms for Data Scientists
- Data Science Learning Path or Steps to become a data scientist Final
- Enable Edit Button in Shutter In Linux Mint 19 and Ubuntu 18.04
- Python 3 time module
- Pygments Tutorial
- How to use Virtualenv?
- Installing MySQL (Windows, Linux and Mac)
- What is if __name__ == '__main__' in Python ?
- Installing GoAccess (A Real-time web log analyzer)
- Installing Isso
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
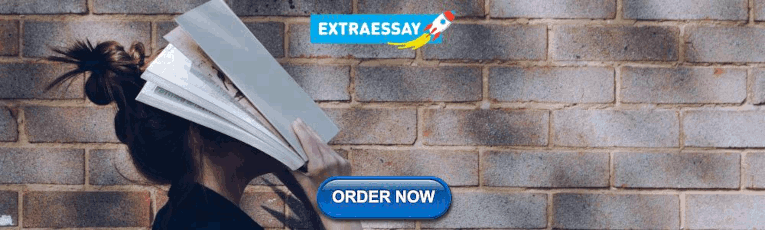
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
Assignment Operators in C
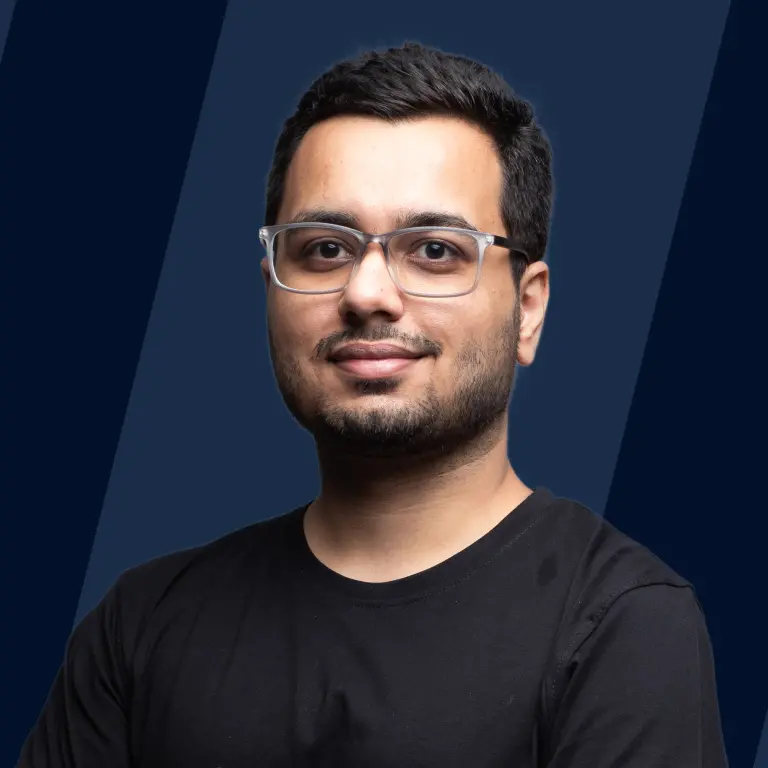
Operators are a fundamental part of all the computations that computers perform. Today we will learn about one of them known as Assignment Operators in C. Assignment Operators are used to assign values to variables. The most common assignment operator is = . Assignment Operators are Binary Operators.
Types of Assignment Operators in C
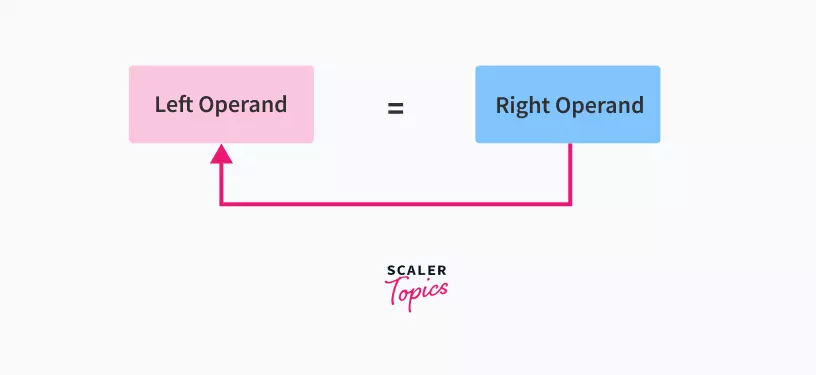
Here is a list of the assignment operators that you can find in the C language:
- basic assignment ( = )
- subtraction assignment ( -= )
- addition assignment ( += )
- division assignment ( /= )
- multiplication assignment ( *= )
- modulo assignment ( %= )
- bitwise XOR assignment ( ^= )
- bitwise OR assignment ( |= )
- bitwise AND assignment ( &= )
- bitwise right shift assignment ( >>= )
- bitwise left shift assignment ( <<= )
Working of Assignment Operators in C
This is the complete list of all assignment operators in C. To read the meaning of operator please keep in mind the above example.
Example for Assignment Operators in C
Basic assignment ( = ) :
Subtraction assignment ( -= ) :
Addition assignment ( += ) :
Division assignment ( /= ) :
Multiplication assignment ( *= ) :
Modulo assignment ( %= ) :
Bitwise XOR assignment ( ^= ) :
Bitwise OR assignment ( |= ) :
Bitwise AND assignment ( &= ) :
Bitwise right shift assignment ( >>= ) :
Bitwise left shift assignment ( <<= ) :
This is the detailed explanation of all the assignment operators in C that we have. Hopefully, This is clear to you.
Practice Problems on Assignment Operators in C
1. what will be the value of a after the following code is executed.
A) 10 B) 11 C) 12 D) 15
Answer – C. 12 Explanation: a starts at 10, increases by 5 to 15, then decreases by 3 to 12. So, a is 12.
2. After executing the following code, what is the value of num ?
A) 4 B) 8 C) 16 D) 32
Answer: C) 16 Explanation: After right-shifting 8 (binary 1000) by one and then left-shifting the result by two, the value becomes 16 (binary 10000).
Q. How does the /= operator function? Is it a combination of two other operators?
A. The /= operator is a compound assignment operator in C++. It divides the left operand by the right operand and assigns the result to the left operand. It is equivalent to using the / operator and then the = operator separately.
Q. What is the most basic operator among all the assignment operators available in the C language?
A. The most basic assignment operator in the C language is the simple = operator, which is used for assigning a value to a variable.
- Assignment operators are used to assign the result of an expression to a variable.
- There are two types of assignment operators in C. Simple assignment operator and compound assignment operator.
- Compound Assignment operators are easy to use and the left operand of expression needs not to write again and again.
- They work the same way in C++ as in C.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
Java Operator Precedence
Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
- Java Math IEEEremainder()
Operators are symbols that perform operations on variables and values. For example, + is an operator used for addition, while * is also an operator used for multiplication.
Operators in Java can be classified into 5 types:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Bitwise Operators
1. Java Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations on variables and data. For example,
Here, the + operator is used to add two variables a and b . Similarly, there are various other arithmetic operators in Java.
Example 1: Arithmetic Operators
In the above example, we have used + , - , and * operators to compute addition, subtraction, and multiplication operations.
/ Division Operator
Note the operation, a / b in our program. The / operator is the division operator.
If we use the division operator with two integers, then the resulting quotient will also be an integer. And, if one of the operands is a floating-point number, we will get the result will also be in floating-point.
% Modulo Operator
The modulo operator % computes the remainder. When a = 7 is divided by b = 4 , the remainder is 3 .
Note : The % operator is mainly used with integers.
2. Java Assignment Operators
Assignment operators are used in Java to assign values to variables. For example,
Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age .
Let's see some more assignment operators available in Java.
Example 2: Assignment Operators
3. java relational operators.
Relational operators are used to check the relationship between two operands. For example,
Here, < operator is the relational operator. It checks if a is less than b or not.
It returns either true or false .
Example 3: Relational Operators
Note : Relational operators are used in decision making and loops.
4. Java Logical Operators
Logical operators are used to check whether an expression is true or false . They are used in decision making.
Example 4: Logical Operators
Working of Program
- (5 > 3) && (8 > 5) returns true because both (5 > 3) and (8 > 5) are true .
- (5 > 3) && (8 < 5) returns false because the expression (8 < 5) is false .
- (5 < 3) || (8 > 5) returns true because the expression (8 > 5) is true .
- (5 > 3) || (8 < 5) returns true because the expression (5 > 3) is true .
- (5 < 3) || (8 < 5) returns false because both (5 < 3) and (8 < 5) are false .
- !(5 == 3) returns true because 5 == 3 is false .
- !(5 > 3) returns false because 5 > 3 is true .
5. Java Unary Operators
Unary operators are used with only one operand. For example, ++ is a unary operator that increases the value of a variable by 1 . That is, ++5 will return 6 .
Different types of unary operators are:
- Increment and Decrement Operators
Java also provides increment and decrement operators: ++ and -- respectively. ++ increases the value of the operand by 1 , while -- decrease it by 1 . For example,
Here, the value of num gets increased to 6 from its initial value of 5 .
Example 5: Increment and Decrement Operators
In the above program, we have used the ++ and -- operator as prefixes (++a, --b) . We can also use these operators as postfix (a++, b++) .
There is a slight difference when these operators are used as prefix versus when they are used as a postfix.
To learn more about these operators, visit increment and decrement operators .
6. Java Bitwise Operators
Bitwise operators in Java are used to perform operations on individual bits. For example,
Here, ~ is a bitwise operator. It inverts the value of each bit ( 0 to 1 and 1 to 0 ).
The various bitwise operators present in Java are:
These operators are not generally used in Java. To learn more, visit Java Bitwise and Bit Shift Operators .
Other operators
Besides these operators, there are other additional operators in Java.
The instanceof operator checks whether an object is an instanceof a particular class. For example,
Here, str is an instance of the String class. Hence, the instanceof operator returns true . To learn more, visit Java instanceof .
The ternary operator (conditional operator) is shorthand for the if-then-else statement. For example,
Here's how it works.
- If the Expression is true , expression1 is assigned to the variable .
- If the Expression is false , expression2 is assigned to the variable .
Let's see an example of a ternary operator.
In the above example, we have used the ternary operator to check if the year is a leap year or not. To learn more, visit the Java ternary operator .
Now that you know about Java operators, it's time to know about the order in which operators are evaluated. To learn more, visit Java Operator Precedence .
Table of Contents
- Introduction
- Java Arithmetic Operators
- Java Assignment Operators
- Java Relational Operators
- Java Logical Operators
- Java Unary Operators
- Java Bitwise Operators
Sorry about that.
Related Tutorials
Java Tutorial

- Design Pattern
- Interview Q
C Control Statements
C functions, c dynamic memory, c structure union, c file handling, c preprocessor, c command line, c programming test, c interview.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

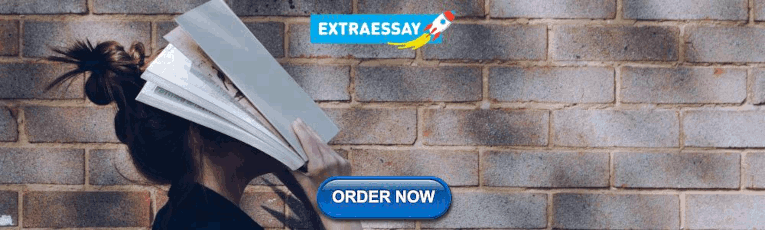
IMAGES
VIDEO
COMMENTS
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
Assignment Statements and the Assignment Operator. One of the most powerful programming language features is the ability to create, access, and mutate variables. In Python, a variable is a name that refers to a concrete value or object, allowing you to reuse that value or object throughout your code. ... In this example, you chain two ...
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: | =. In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place.
Assignment Operator. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Introduction to the C assignment operators. An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable: #include<stdio.h>intmain() { int counter; // assign 1 to counter counter = 1; printf("%d\n", counter); // 1return0; }
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
The += assignment operator is a combination of + arithmetic operator and = simple assignment operator. For example, x += y; is equivalent to x = x+y;. It adds the right side value to the value of left side operand and assign the result back to the left-hand side operand. #include <stdio.h> int main () { int x = 100, y = 20, z = 50 ...
The Assignment operators in C are some of the Programming operators that are useful for assigning the values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: int i = 10; The below table displays all the assignment operators present in C Programming with an example. C Assignment Operators.
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator. For example, consider following C statements. The above expression a = a + 2 is equivalent to a += 2.
Example 2: Assignment Operators # assign 10 to a a = 10 # assign 5 to b b = 5 # assign the sum of a and b to a a += b # a = a + b print(a) # Output: 15. Here, we have used the += operator to assign the sum of a and b to a. Similarly, we can use any other assignment operators as per our needs.
The assignment operator ( = ) is used to assign a value to the variable. Its general format is as follows: variable = right_side. The operand on the left side of the assignment operator must be a variable and operand on the right-hand side must be a constant, variable or expression. Here are some examples:
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
A. The most basic assignment operator in the C language is the simple = operator, which is used for assigning a value to a variable. Conclusion. Assignment operators are used to assign the result of an expression to a variable. There are two types of assignment operators in C. Simple assignment operator and compound assignment operator.
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition. In this tutorial, you will learn about different C operators such as arithmetic, increment, assignment, relational, logical, etc. with the help of examples.
2. Java Assignment Operators. Assignment operators are used in Java to assign values to variables. For example, int age; age = 5; Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age. Let's see some more assignment operators available in Java.
Assignment Operator in C is a tutorial that explains how to use the operator that assigns a value to a variable in C programming language. It covers the syntax, types, and examples of assignment operator in C. It also provides a quiz and interview questions to test your knowledge. Learn assignment operator in C from javatpoint, a leading online platform for learning various technologies.