What are the conditional assignment operators in PHP?
Free System Design Interview Course
Many candidates are rejected or down-leveled due to poor performance in their System Design Interview. Stand out in System Design Interviews and get hired in 2024 with this popular free course.
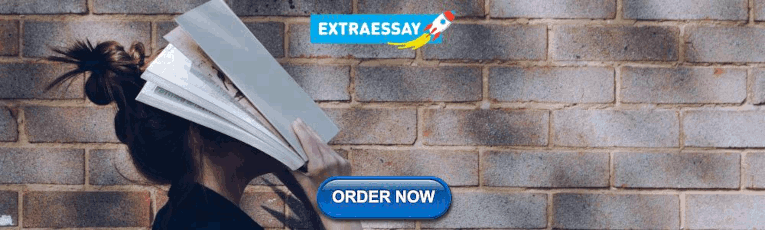
Introduction
Operators in PHP are signs and symbols which are used to indicate that a particular operation should be performed on an operand in an expression. Operands are the operation objects, or targets. An expression contains operands and operators arranged in a logical manner.
In PHP, there are numerous operators, which are grouped into the following categories:
Logical operators
String operators
Array operators
Arithmetic operators
Assignment operators
Comparison operators
Increment/Decrement
Conditional assignment operators
Some examples of these operators are:
- - Subtraction
- * Multiplication
- -- and so much more.
What are conditional assignment operators?
Conditional assignment operators , as the name implies, assign values to operands based on the outcome of a certain condition. If the condition is true , the value is assigned. If the condition is false , the value is not assigned.
There are two types of conditional assignment operators: tenary operators and null coalescing operators .
1. Tenary operators ?:
Basic syntax.
Where expr1 , expr2 , and expr3 are either expressions or variables.
From the syntax, the value of $y will be evaluated. This value of $y is evaluated to expr2 if expr1 = TRUE . The value of $y is expr3 if expr1 = FALSE .
2. Null coalescing operators ??
The ?? operator was introduced in PHP 7 and is basically used to check for a NULL condition before assignment of values.
Where varexp1 and varexp2 are either expressions or variables.
The value of $z is varexp1 if and only if varexp1 exists, and is not NULL. If varexp1 does not exist or is NULL, the value of $z is varexp2 .
Let’s use some code snippets to paint a more vivid description of these operators.
Explanation
In the code above, the new value of $y and $z is set based on the evaluation result of expr1 and varexp1 , respectively.
RELATED TAGS
CONTRIBUTOR
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php operators.
Operators are used to perform operations on variables and values.
PHP divides the operators in the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
PHP Arithmetic Operators
The PHP arithmetic operators are used with numeric values to perform common arithmetical operations, such as addition, subtraction, multiplication etc.
PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Advertisement
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable's value.
The PHP decrement operators are used to decrement a variable's value.
PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
PHP String Operators
PHP has two operators that are specially designed for strings.
PHP Array Operators
The PHP array operators are used to compare arrays.
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value depending on conditions:
PHP Exercises
Test yourself with exercises.
Multiply 10 with 5 , and output the result.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
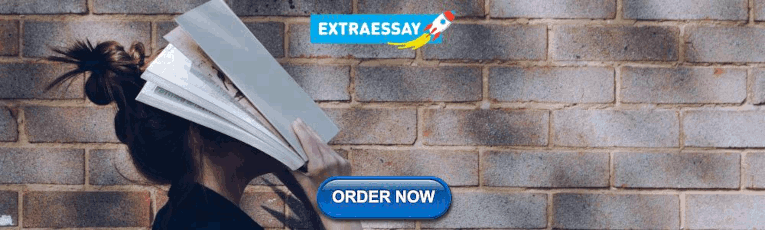
PHP Variable Assignment Within If Statement
The usual practice when checking for the return value of functions is to run the function and store the value in a variable, and then test that variable. Here is an example of that process using the strstr() function.
This code will output "bool(false)" as that was the return value of the strstr() function.
There is another way to write the same code within a single if statement. The following example assigns the variable and checks the return value in a single line of code.
This code will output "bool(false)" again as this has the same function as the previous example.
There is one problem with assigning variables in this way, and this is that you can only do one. If you try to assign more than one variable at a time you will find that everything but the final variable will turn out to be the outcome of the boolean comparison. Take the following example, which uses the same $string variable as the other examples.
The output of this code will be as follows:
The first two comparisons come out as true and so $var1 and $var2 are assigned boolean values of true. The final comparison is the only one that is assigned the expected value. This might be a bug in PHP, but as this is a non-standard way of assigning variables I don't think many people have come across it.
Submitted by James Bower on Mon, 09/07/2009 - 21:17
Submitted by Mirek Suk on Tue, 09/28/2010 - 12:08
Name Philip Norton
Submitted by philipnorton42 on Wed, 09/29/2010 - 08:56
Submitted by Jim on Sun, 11/07/2010 - 23:09
This works too: if( !($val = $arg) ) { $val = 'default'; }
Submitted by Frank on Mon, 05/02/2011 - 17:52
Submitted by Vishal on Sun, 05/17/2015 - 09:24
Submitted by Jesus on Tue, 03/20/2018 - 14:55
Submitted by philipnorton42 on Tue, 03/20/2018 - 15:19
A gotcha worth highlighting, assigning a 0 is of course a falsy, so you can work around it as follows where NULL is returned when we really want to exit, and not on 0.
Submitted by Russ on Fri, 05/31/2019 - 18:18
Hi, I need help with below code
How to create variable with if multiple statement in php and print
and want to print as
Submitted by Sam on Fri, 05/15/2020 - 21:30
For fixed payment types, you may be better off to assign an array with the options, with the code as key, and display name as value. That array could be filled from a database query on payment types allowed, which would then pick up when you add a new option, you don't then have to re-code so much.
That has no input validation etc, but might be a start.
Submitted by Peter H on Fri, 06/05/2020 - 12:24
Add new comment
Related content, recreating spotify wrapped in php.
I quite like the end of the year report from Spotify that they call "Wrapped". This is a little application in which they tell you what your favorite artist was and what sort of genres you listened to the most during the year.
Should A Constructor Throw An Exception?
Let's say you had a class that you wanted to use, but there was some sort of error in creating the object. This might be that the wrong parameters were passed, or the third party service (eg. a database) wasn't available at the time of creation.
PHP Question: Variable Reference
What does the following code print out?
Creating An Authentication System With PHP and MariaDB
Using frameworks to handle the authentication of your PHP application is perfectly fine to do, and normally encouraged. They abstract away all of the complexity of managing users and sessions that need to work in order to allow your application to function.
Creating Sparklines In PHP
A sparkline is a very small line graph that is intended to convey some simple information, usually in terms of a numeric value over time. They tend to lack axes or other labels and are added to information readouts in order to expand on numbers in order to give them more context.
PHP:CSI - Improving Bad PHP Logging Code
I read The Daily WTF every now and then and one story about bad logging code in PHP stood out to me. The post looked at some PHP code that was created to log a string to a file, but would have progressively slowed down the application every time a log was generated.
Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
- Variable Assignment, Expressions, and Operators
Variables are containers for storing information, such as numbers or text so that they can be used multiple times in the code. Variables in PHP are identified by a dollar sign ($) followed by the variable name. A variable name must begin with a letter or the underscore character and only contain alphanumeric characters and underscores. A variable name cannot contain spaces. Finally, variable names in PHP are case-sensitive.
- Post author By BrainBell
- Post date May 12, 2022
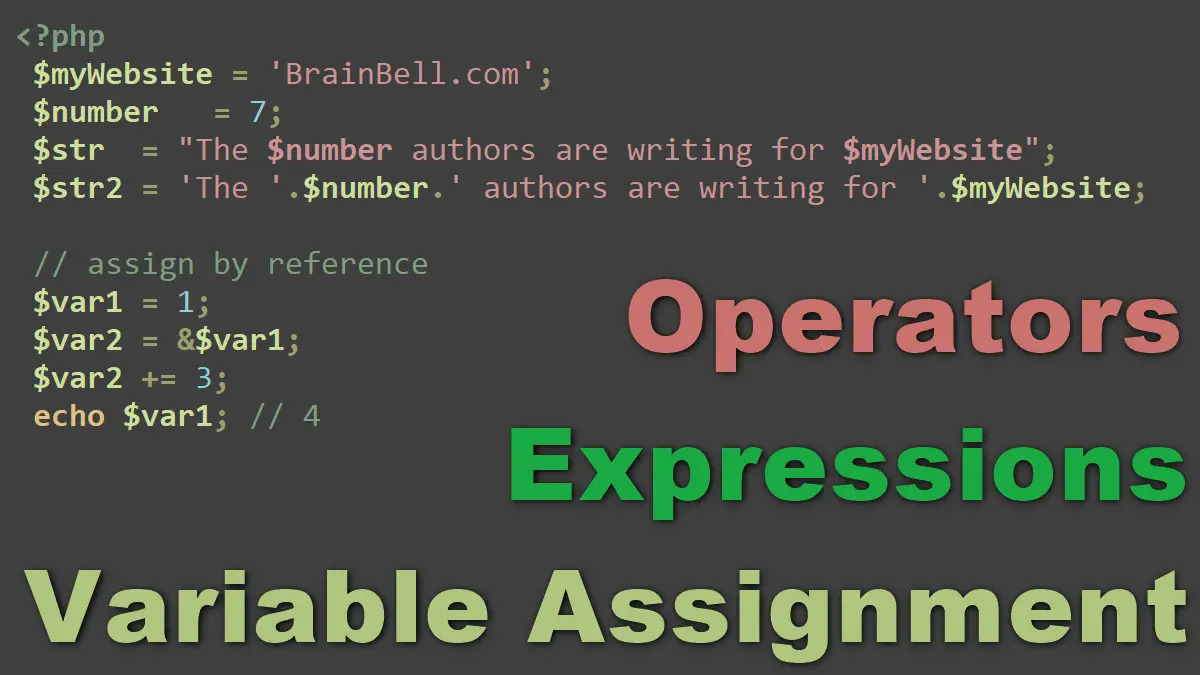
This tutorial covers the following topics:
- Define a variable
- Assign a variable by reference
- Assign a string value to a variable
Assignment Operators
- Arithmetic Operators (See Comparison Operators and Logical Operators on Conditional Expression Tutorial).
Operator precedence
Expressions, define a variable.
PHP uses the = symbol as an assignment operator. The variable goes on the left of the equal sign, and the value goes on the right. Because it assigns a value, the equal sign is called the assignment operator .
$ variableName = 'Assigned Value' ;
You can break the above example into the following parts:
- A dollar sign $ prefix
- Variable name
- The assignment operator (equal sign = )
- Assigned value
- Semicolon to terminate the statement
A PHP variable must be defined before it can be used. Attempting to use an undefined variable will trigger an error exception:
In PHP, you do not need to declare a variable separately before using it. Just assign value to a variable by using the assignment operator (equals sign = ) to make it defined or initialized:
A defined variable can be used by referencing its name, for example, use the print or echo command (followed by the variable’s name) to display the value of the variable on the web page:
Variable names are case-sensitive in PHP, so $Variable , $variable , $VAriable , and $VARIABLE are all different variables.
Text requires quotes
If you look closely at the PHP code block in the above example, you’ll notice that the value assigned to the second variable isn’t enclosed in quotes. It looks like this:
Then the ‘BrainBell.com’ did use quotes, like this:
The simple rules are as follows:
- The text requires quotes (single or double)
- No quotes are required for numbers, True , False and Null
Assign a string value to a variable:
Concatenate two strings together to produce “test string”:
Add a string to the end of another to produce “test string”:
Here is a shortcut to adding a string to the end of another:
Assign by reference
By default, PHP assigns all variables other than objects by value and not by reference. PHP has optimizations to make assignment by value faster than assigning by reference, but if you want to assign by reference you can use the & operator as follows:
The assignment operator (equal = ) can be combined with other operators to make it easier to write certain expressions. See the following table:
These operators assign values to variables. They start with the assignment operator = and move on to += , -= , etc.(see above table). The operator += adds the value on the right side to the variable on the left:
Arithmetic Operators
Using an operator, you can manipulate the contents of one or more variables or constants to produce a new value. For example, this code uses the addition operator ( + ) to add the values of $x and $y together to produce a new value:
So an operator is a symbol that manipulates one or more values, usually producing a new value in the process. The following list describes the types of arithmetic operators:
Sum integers to produce an integer:
The values and variables that are used with an operator are known as operands.
Subtraction, multiplication, and division might have a result that is a float or an integer, depending on the initial value of $var :
Multiply to double a value:
Halve a value:
These work with float types too:
Get the remainder of dividing 5 by 4:
4 exponent (or power) of 2:
These all add 1 to $var:
And these all subtract 1 from $var:
If the -- or ++ operator appears before the variable then the interpreter will first evaluate it and then return the changed variable:
If the -- or ++ operator appears after the variable then the interpreter will return the variable as it was before the statement run and then increment the variable:
There are many mathematical functions available in the math library of PHP for more complex tasks. We introduce some of these in the next pages.
The precedence of operators in an expression is similar to the precedence defined in any other language. Multiplication and division occur before subtraction and addition, and so on. However, reliance on evaluation orders leads to unreadable, confusing code. Rather than memorize the rules, we recommend you construct unambiguous expressions with parentheses because parentheses have the highest precedence in evaluation.
For example, in the following fragment $variable is assigned a value of 32 because of the precedence of multiplication over addition:
The result is much clearer if parentheses are used:
But the following example displays a different result because parentheses have the highest precedence in evaluation.
An expression in PHP is anything that evaluates a value; it is a combination of values, variables, operators, and functions that results in a value. Here are some examples of expressions:
An expression has a value and a type; for example, the expression 4 + 7 has the value 11 and the type integer, and the expression "abcdef" has the value abcdef and the type string . PHP automatically converts types when combining values in an expression. For example, the expression 4 + 7.0 contains an integer and a float; in this case, PHP considers the integer as a floating-point number, and the result is a float. The type conversions are largely straightforward; however, there are some traps, which are discussed later in this section.
Getting Started with PHP:
- Introducing PHP
- PHP Development Environment
- Delimiting Strings
- Variable Substitution
- Language Reference
Expressions
Expressions are the most important building blocks of PHP. In PHP, almost anything you write is an expression. The simplest yet most accurate way to define an expression is "anything that has a value".
The most basic forms of expressions are constants and variables. When you type $a = 5 , you're assigning 5 into $a . 5 , obviously, has the value 5, or in other words 5 is an expression with the value of 5 (in this case, 5 is an integer constant).
After this assignment, you'd expect $a 's value to be 5 as well, so if you wrote $b = $a , you'd expect it to behave just as if you wrote $b = 5 . In other words, $a is an expression with the value of 5 as well. If everything works right, this is exactly what will happen.
Slightly more complex examples for expressions are functions. For instance, consider the following function: <?php function foo () { return 5 ; } ?>
Assuming you're familiar with the concept of functions (if you're not, take a look at the chapter about functions ), you'd assume that typing $c = foo() is essentially just like writing $c = 5 , and you're right. Functions are expressions with the value of their return value. Since foo() returns 5, the value of the expression ' foo() ' is 5. Usually functions don't just return a static value but compute something.
Of course, values in PHP don't have to be integers, and very often they aren't. PHP supports four scalar value types: int values, floating point values ( float ), string values and bool values (scalar values are values that you can't 'break' into smaller pieces, unlike arrays, for instance). PHP also supports two composite (non-scalar) types: arrays and objects. Each of these value types can be assigned into variables or returned from functions.
PHP takes expressions much further, in the same way many other languages do. PHP is an expression-oriented language, in the sense that almost everything is an expression. Consider the example we've already dealt with, $a = 5 . It's easy to see that there are two values involved here, the value of the integer constant 5 , and the value of $a which is being updated to 5 as well. But the truth is that there's one additional value involved here, and that's the value of the assignment itself. The assignment itself evaluates to the assigned value, in this case 5. In practice, it means that $a = 5 , regardless of what it does, is an expression with the value 5. Thus, writing something like $b = ($a = 5) is like writing $a = 5; $b = 5; (a semicolon marks the end of a statement). Since assignments are parsed in a right to left order, you can also write $b = $a = 5 .
Another good example of expression orientation is pre- and post-increment and decrement. Users of PHP and many other languages may be familiar with the notation of variable++ and variable-- . These are increment and decrement operators . In PHP, like in C, there are two types of increment - pre-increment and post-increment. Both pre-increment and post-increment essentially increment the variable, and the effect on the variable is identical. The difference is with the value of the increment expression. Pre-increment, which is written ++$variable , evaluates to the incremented value (PHP increments the variable before reading its value, thus the name 'pre-increment'). Post-increment, which is written $variable++ evaluates to the original value of $variable , before it was incremented (PHP increments the variable after reading its value, thus the name 'post-increment').
A very common type of expressions are comparison expressions. These expressions evaluate to either false or true . PHP supports > (bigger than), >= (bigger than or equal to), == (equal), != (not equal), < (smaller than) and <= (smaller than or equal to). The language also supports a set of strict equivalence operators: === (equal to and same type) and !== (not equal to or not same type). These expressions are most commonly used inside conditional execution, such as if statements.
The last example of expressions we'll deal with here is combined operator-assignment expressions. You already know that if you want to increment $a by 1, you can simply write $a++ or ++$a . But what if you want to add more than one to it, for instance 3? You could write $a++ multiple times, but this is obviously not a very efficient or comfortable way. A much more common practice is to write $a = $a + 3 . $a + 3 evaluates to the value of $a plus 3, and is assigned back into $a , which results in incrementing $a by 3. In PHP, as in several other languages like C, you can write this in a shorter way, which with time would become clearer and quicker to understand as well. Adding 3 to the current value of $a can be written $a += 3 . This means exactly "take the value of $a , add 3 to it, and assign it back into $a ". In addition to being shorter and clearer, this also results in faster execution. The value of $a += 3 , like the value of a regular assignment, is the assigned value. Notice that it is NOT 3, but the combined value of $a plus 3 (this is the value that's assigned into $a ). Any two-place operator can be used in this operator-assignment mode, for example $a -= 5 (subtract 5 from the value of $a ), $b *= 7 (multiply the value of $b by 7), etc.
There is one more expression that may seem odd if you haven't seen it in other languages, the ternary conditional operator:
<?php $first ? $second : $third ?>
If the value of the first subexpression is true (non-zero), then the second subexpression is evaluated, and that is the result of the conditional expression. Otherwise, the third subexpression is evaluated, and that is the value.
The following example should help you understand pre- and post-increment and expressions in general a bit better:
<?php function double ( $i ) { return $i * 2 ; } $b = $a = 5 ; /* assign the value five into the variable $a and $b */ $c = $a ++; /* post-increment, assign original value of $a (5) to $c */ $e = $d = ++ $b ; /* pre-increment, assign the incremented value of $b (6) to $d and $e */ /* at this point, both $d and $e are equal to 6 */ $f = double ( $d ++); /* assign twice the value of $d before the increment, 2*6 = 12 to $f */ $g = double (++ $e ); /* assign twice the value of $e after the increment, 2*7 = 14 to $g */ $h = $g += 10 ; /* first, $g is incremented by 10 and ends with the value of 24. the value of the assignment (24) is then assigned into $h, and $h ends with the value of 24 as well. */ ?>
Some expressions can be considered as statements. In this case, a statement has the form of ' expr ; ' that is, an expression followed by a semicolon. In $b = $a = 5; , $a = 5 is a valid expression, but it's not a statement by itself. $b = $a = 5; , however, is a valid statement.
One last thing worth mentioning is the truth value of expressions. In many events, mainly in conditional execution and loops, you're not interested in the specific value of the expression, but only care about whether it means true or false . The constants true and false (case-insensitive) are the two possible boolean values. When necessary, an expression is automatically converted to boolean. See the section about type-casting for details about how.
PHP provides a full and powerful implementation of expressions, and documenting it entirely goes beyond the scope of this manual. The above examples should give you a good idea about what expressions are and how you can construct useful expressions. Throughout the rest of this manual we'll write expr to indicate any valid PHP expression.
Improve This Page
User contributed notes 12 notes.

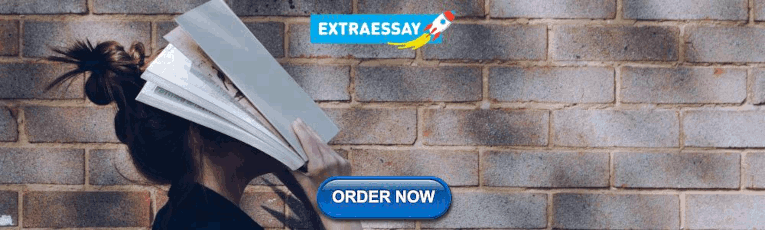
IMAGES
VIDEO
COMMENTS
php variable as conditional assignment. 3. Assign variable whilst checking it. 0. Assign value to variable using multiple conditional statement. 2. Put IF condition inside a variable. 1. One PHP statement to use result of IF statement for assignment. 6. PHP: Assign multiple variables in if statement. 1.
I found this wondering about the rules of declaring a variable then using it immediately in subsequent conditions in the same statement. Thanks to previous answer for the codepad link, I made my own to test the theory.
That's simply not a real question and there is no point in trying to answer it directly, especially if you aren't familiar with PHP syntax.. It's the OP's premises have to be questioned, not the code he's posted.. This code will plainly output the result of getClientAccount method (unless it's 0 or empty array).. So, the OP have to check what "was in the code actually" once more or verify the ...
Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
Conditional Assignment Operator in PHP is a shorthand operator that allow developers to assign values to variables based on certain conditions. In this article, we will explore how the various Conditional Assignment Operators in PHP simplify code and make it more readable. Let's begin with the ternary operator. Ternary Operator Syntax
if. ¶. The if construct is one of the most important features of many languages, PHP included. It allows for conditional execution of code fragments. PHP features an if structure that is similar to that of C: statement. As described in the section about expressions, expression is evaluated to its Boolean value.
When you use an if statement in a variable declaration, it allows you to conditionally assign a value to the variable based on certain conditions. This can be quite useful when you want to set a variable's value dynamically depending on the outcome of certain conditions. For example, let's say you're building a website and you want to display a ...
The if statement allows you to execute a statement if an expression evaluates to true. The following shows the syntax of the if statement: statement; Code language: HTML, XML (xml) In this syntax, PHP evaluates the expression first. If the expression evaluates to true, PHP executes the statement. In case the expression evaluates to false, PHP ...
Decisions written in code are formed using conditionals: "If x, then y.". Even a button click is a form of condition: "If this button is clicked, go to a certain page.". Conditional statements are part of the logic, decision making, or flow control of a computer program. You can compare a conditional statement to a "Choose Your Own ...
The main goal of the ternary operator is to shorten small conditional statements. These are common when you want to assign a value based on a condition. A common conditional variable assignment scenario is assigning a default value. We had that problem several times in our example breaking conditionals into functions.
In addition to what Lawrence said about assigning a default value, one can now use the Null Coalescing Operator (PHP 7). Hence when we want to assign a default value we can write: ... To assign default value in variable assignation, the simpliest solution to me is: <?php
PHP Conditional Statements. Very often when you write code, you want to perform different actions for different conditions. You can use conditional statements in your code to do this. In PHP we have the following conditional statements: if statement - executes some code if one condition is true
Conditional assignment operators, as the name implies, assign values to operands based on the outcome of a certain condition. If the condition is true, the value is assigned. If the condition is false, the value is not assigned. There are two types of conditional assignment operators: tenary operators and null coalescing operators.
The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right. ... The PHP conditional assignment operators are used to set a value depending on conditions: Operator Name Example ...
PHP Variable Assignment Within If Statement. Note: This post is over two years old and so the information contained here might be out of date. If you do spot something please leave a comment and we will endeavour to correct. ... If you try to assign more than one variable at a time you will find that everything but the final variable will turn ...
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
Hmm, after reading what conditional assignment in Ruby is exactly (what the other question was closed as duplicate was about), I guess there is a subtle difference. ... php variable as conditional assignment. 3. nested shorthand if. 3. shorthand for if else statement assigning value to variable. 3.
Assignment operators are essential tools for any PHP developer, facilitating calculations and operations on variables while assigning results to other variables in a single statement. Moreover, leveraging assignment operators allows you to make your code more concise, easier to read, and helps in avoiding common programming errors.
Variable Assignment, Expressions, and Operators. Variables are containers for storing information, such as numbers or text so that they can be used multiple times in the code. Variables in PHP are identified by a dollar sign ($) followed by the variable name. A variable name must begin with a letter or the underscore character and only contain ...
PHP also supports two composite (non-scalar) types: arrays and objects. Each of these value types can be assigned into variables or returned from functions. PHP takes expressions much further, in the same way many other languages do. PHP is an expression-oriented language, in the sense that almost everything is an expression.