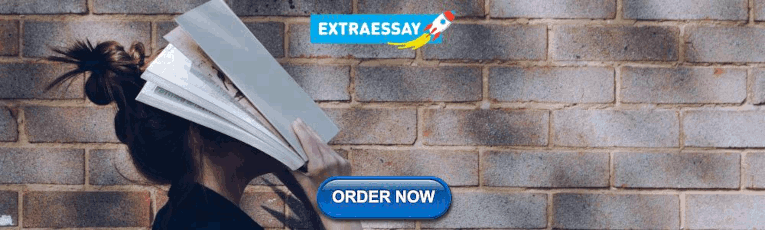
Practice 164 exercises in C#
Learn and practice C# by completing 164 exercises that explore different concepts and ideas.
Explore the C# exercises on Exercism
Unlock more exercises as you progress. They’re great practice and fun to do!
- Skills Directory
Developed around 2000 by Microsoft as part of its .NET initiative, C# is a general-purpose, object-oriented programming language designed for Common Language Infrastructure (CLI), and widely recognized for its structured, strong-typing and lexical scoping abilities.
This competency area includes understanding the structure of C# programs, types, and Variables, basic OOP, Properties and Indexers, Collections, Exception handling, among others.
Key Competencies:
- Program Structure - Understand the physical structure of C# programs and the use of namespaces and types.
- Types and Variables - Know the difference between value types and reference.
- Nullable Types - Understand the differences between nullable and non-nullable types.
- Basic OOP - Use classes and objects to write simple object-oriented code. Understand inheritance facilities and use of interfaces to create compositional object-oriented code.
- Properties and Indexers - Using properties and indexers on classes to provide additional safety and expressivity.
- Collections - Knowledge in trade-offs of the various standard library generic collections like List, Set, Dictionary, etc.
- Exception handling - Use of try/catch/finally for managing exceptions.
Cookie support is required to access HackerRank
Seems like cookies are disabled on this browser, please enable them to open this website
C# Tutorial
C# examples, c# exercises.
You can test your C# skills with W3Schools' Exercises.
We have gathered a variety of C# exercises (with answers) for each C# Chapter.
Try to solve an exercise by editing some code, or show the answer to see what you've done wrong.
Count Your Score
You will get 1 point for each correct answer. Your score and total score will always be displayed.
Start C# Exercises
Start C# Exercises ❯
If you don't know C#, we suggest that you read our C# Tutorial from scratch.
Kickstart your career
Get certified by completing the course

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
Kata Library: C# Practice
- Collections
Collect: kata
Loading collection data...
You have not created any collections yet.
Collections are a way for you to organize kata so that you can create your own training routines. Every collection you create is public and automatically sharable with other warriors. After you have added a few kata to a collection you and others can train on the kata contained within the collection.
Get started now by creating a new collection .
Set the name for your new collection. Remember, this is going to be visible by everyone so think of something that others will understand.
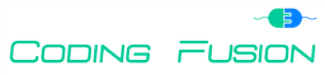
50+ C# Programs, Exercises, Solutions for beginners to practice
Top C# Programs with solutions for beginners to practice. Fibonacci Series, Prime, Palindrome, Armstrong, loops, Swap, Pyramids , Triangles, and many more
C# Program to Check Whether a Number is Prime or Not
In this C# program, we will take input from the user and check whether the number is prime or not. A prime number is a positive integer that is divisible only by 1 and itself.
C# Program to Calculate Sum of first N natural numbers
In this C# program, we will take input from the user and generate the sum of first N natural Numbers. The sum of natural numbers up to 10 is: 1+2+3+4+5+6+7+8+9+10=55
C# Program to swap two numbers without using the third variable
In this C# program, we will swap two numbers without using the third variable. We will use two methods to swap numbers 1) By using Addition and Subtraction 2) By using Multiplication and Division
C# Program to print prime numbers from 1 to N
In this C# program, we will take input from the user and print prime numbers between 1 and the number input by the user. A prime number is a positive integer that is divisible only by 1 and itself.
C# Program to print perfect numbers from 1 to N
In this C# program, we will take input from the user and print perfect numbers between 1 and the number input by the user. A perfect number is a positive integer that is equal to the sum of its positive divisors, excluding the number itself. For instance, 6 has divisors 1, 2 and 3, and 1 + 2 + 3 = 6, so 6 is a perfect number.
C# Program to print even numbers from 1 to N
In this C# program, we will take input from the user and print even numbers between 1 and the number input by the user. A even number is a positive integer that is exactly divisible by 2.
C# Program to print odd numbers from 1 to N
In this C# program, we will take input from the user and print odd numbers between 1 and the number input by the user. An odd number is a positive integer that is not divisible by 2.
C# Program to Check Whether a Number is Even or Odd
In this C# program, we will take input from the user and check whether a number is even or odd. An Even number is a positive integer that is divisible by 2 and An odd number is a positive integer that is not divisible by 2.
C# Program to Check Whether a Number is Positive or Negative
In this C# program, we will take input from the user and check whether a number is Positive or Negative. A Positive number is a number that is greater than 0 and a Negative number is a number that is lesser than 0.
C# Program to generate a sum of digits
In this C# program, we will take input from the user and generate a sum of digits. For example, if the user input 88 then result is 16.
C# Program to Generate a Multiplication Table of a given integer
In this C# program, we will take input from the user and generate a multiplication table.
C# Program to Calculate the Area of a Circle
In this C# program, we will take input (radius) from the user and calculate the area of a circle. Formula to calculate area of circle is A= π*r*r.
C# Program to Calculate the Area of a Square
In this C# program, we will take input (side) from the user and calculate the area of a square. Formula to calculate area of Square is A= (side of square*side of square).
C# Program to Calculate the Area of a Rectangle
In this C# program, we will take input (Length and Width) from the user and calculate the area of a Rectangle. Formula to calculate area of Rectangle is A= Length*Width.
C# Program to Calculate the Area of a Triangle
In this C# program, we will take input (Base and Height) from the user and calculate the area of a Triangle. Formula to calculate area of Triangle is A= (base*height/2).
C# Program to Check Whether a Number is Palindrome or Not
In this C# program, we will take input from the user and Check Whether a Number is Palindrome or Not. A number is a palindrome if the reverse of that number is equal to the original number.
C# Program to Check Whether a String is Palindrome or Not
In this C# program, we will take input from the user and Check Whether a String is Palindrome or Not. A String is a palindrome if the reverse of that String is equal to the original
C# Program to Print Fibonacci Series
The Fibonacci sequence is a sequence where the next number is the sum of the previous two numbers. The first two numbers of fibonacci series are 0 and 1.
C# Program to Find Factorial of a Number
In this C# program, we will take input from the user and Find the Factorial of a Number using 3 different ways using 1) For loop 2) Recursion 3) While loop. The factorial of a positive number n is given by n!. 5!=5*4*3*2*1=120
C# Program to print Alphabet Triangle
In this C# program, we will take input (Number of rows) from the user and print the alphabet triangle based on the number of rows input by the user.
C# Program to print Number Triangle
In this C# program, we will take input (Number of rows) from the user and print the Number triangle based on the number of rows input by the user.
C# Program to print Star Triangle
In this C# program, we will take input (Number of rows) from the user and print the Star (*) triangle based on the Number of rows input by the user.
C# Program to print Diamond Shape
In this C# program, we will take input (Number of rows) from the user and print the diamond shape based on the Number of rows input by the user.
C# program to change case of a string
In this C# program, we will take the input string from the user and change it to 1)Upper Case 2)Lower Case 3)Title Case.
C# program to add and subtract days from the date
In this C# program, we will add and subtract days from the date.
C# Program to Check Leap Year
In this C# program, we will take the input from the user and check whether the input year is a Leap Year or not. Leap year is a year which is divisible by 4.
C# program to compare two dates
In this C# program, we will compare two dates and print the result.
C# program to convert days into years, weeks and days
In this C# program, we will take the input (days) from the user and Convert days into years, weeks and days.
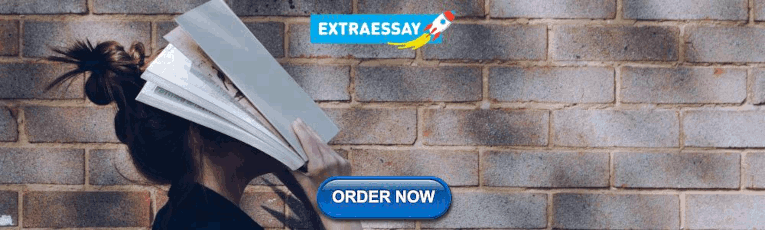
C# Program to Print Day Name of Week
In this C# program, we will take the input from the user and print day name of week.
C# Program to convert Celsius into Fahrenheit
In this C# program, we will take the input Celsius from the user and change it to Fahrenheit.
C# Program to Convert Number into Words
In this C# program, we will take the input number from the user and change it to Words. E.g. 1024 will be converted to One Thousand Twenty Four only.
C# Program to Convert Meters To Kilometers
In this C# program, we will take the input meters from the user and change it Kilometers.
C# program to calculate Simple Interest
In this C# program, we will take the input "Principal Amount", "Interest Rate" and "Time" from the user and calculate the simple interest.
C# program to calculate Compound Interest
In this C# program, we will take the input "Principal Amount", "Interest Rate", "Interest Compound Frequency" and "Time" from the user and calculate the simple interest.
C# Program to Find GCD of two Numbers
In this C# program, we will take the input from the user and find GCD of two numbers.
C# Program to Find LCM of two Numbers
In this C# program, we will take the input from the user and find LCM of two numbers.
C# Program to Find HCF of two Numbers
In this C# program, we will take the input from the user and find HCF of two numbers.
C# program to reverse an array elements
In this C# program, we will reverse an array without using Array.Revers() Method.
C# Program to concatenate two strings
In this C# program, we will take the input strings from the user and print the concatenated string.
C# Program to read the content of a file
In this C# program, we will read the content of the Text file and print the content of the file.
C# Program to Check Whether a Character is a Vowel or Consonant
In this C# program, we will take the input from the user and Check Whether a Character is a Vowel or Consonant.
C# switch example
In this C# program, we will learn how to implement a switch case in C#. We will use a switch statement to display the name of the day based on the input entered by the user.
C# For Loop with examples
In this C# program, we will see For Loop with examples.
C# While Loop with examples
In this C# program, we will see While Loop with examples.
C# Do While Loop with examples
In this C# program, we will see Do While Loop with examples.
C# Remove last specific character in a string
In this C# program, we will see how to Remove last specific character in a string. In this example we will remove last , from the string.
C# Program to Convert a List to a comma separated string
In this C# program, we will see how to Convert a List to a comma separated string.
- Popular Articles
- Recent Articles
Subscribe for Latest Updates.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Introduction
C# tutorial.
- Introduction to .NET Framework
- C# | .NET Framework (Basic Architecture and Component Stack)
- Hello World in C#
- Common Language Runtime (CLR) in C#
Fundamentals
- C# | Identifiers
- C# | Data Types
- C# | Variables
- C# | Literals
- C# | Operators
- C# | Keywords
Control Statements
- C# Decision Making (if, if-else, if-else-if ladder, nested if, switch, nested switch)
- Switch Statement in C#
- Loops in C#
- C# | Jump Statements (Break, Continue, Goto, Return and Throw)
OOP Concepts
- C# | Class and Object
- C# | Constructors
- C# | Inheritance
- C# | Encapsulation
- C# | Abstraction
- C# | Methods
- C# | Method Overloading
- C# | Method Parameters
- C# | Method Overriding
- Anonymous Method in C#
- C# | Arrays
- C# | Jagged Arrays
- C# | Array Class
- How to sort an Array in C# | Array.Sort() Method Set - 1
- How to find the rank of an array in C#
- ArrayList in C#
- C# | ArrayList Class
- C# | Array vs ArrayList
- C# | String
- C# | Verbatim String Literal - @
- C# | String class
- StringBuilder in C#
- C# | String vs StringBuilder
- C# | Tuple Class
- ValueTuple in C#
- ValueTuple Struct in C#
- C# | Indexers
- C# | Multidimensional Indexers
- C# | Overloading of Indexers
- C# | Properties
- C# | Restrictions on Properties
Collections & Generics
- Collections in C#
- C# | Collection Class
- C# | Generics - Introduction
- List Implementation in C#
- C# SortedList with Examples
- HashSet in C# with Examples
- SortedSet in C# with Examples
- C# Dictionary with examples
- SortedDictionary Implementation in C#
- C# Hashtable with Examples
- C# Stack with Examples
- C# Queue with Examples
- Linked List Implementation in C#
In this C# (C Sharp) tutorial, whether you’re beginner or have experience with other programming languages, our free C# tutorials covers the basic and advanced concepts of C# including fundamentals of C#, including syntax, data types, control structures, classes, and objects.
You will also dive into more advanced topics like exception handling, and multithreading. So, whether you are looking to start a career in software development or simply want to expand your programming skills, our C# tutorial is the perfect place to start.
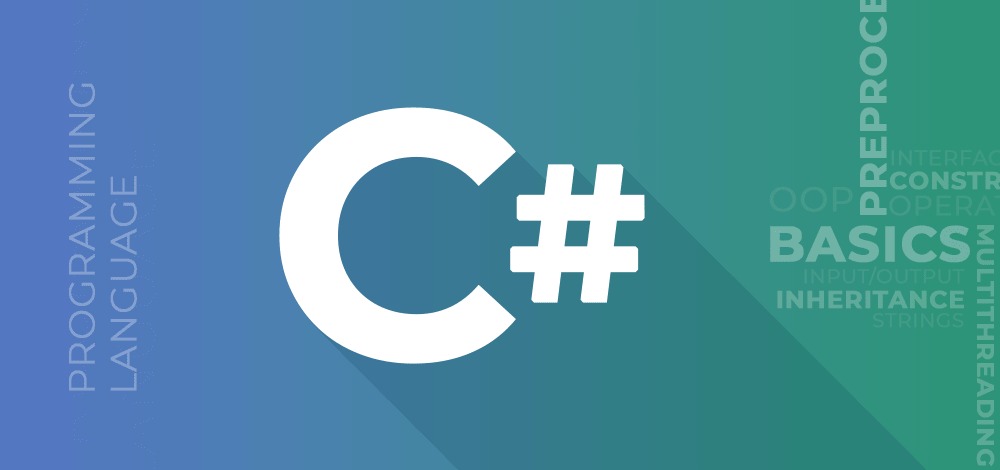
What is C# (C Sharp)
C# is the most common programming language used to develop multiple applications in the.NET framework, and it was introduced by Microsoft in 2000. It was designed to be a simple, object-oriented programming language that can be used to create a wide range of applications and software.
It features a clear syntax, an object-oriented nature, and platform independence, which makes it simpler for developers to organise their code and makes it more legible and manageable.
It is platform-independent in the sense that it may be used to create programmes that operate on different platforms such as Windows, macOS, Linux, and mobile devices. This makes C# a versatile language.
Prerequisite to Learn C#
While there are no strict prerequisites to learning C#, it is a high-level language. So, if you have experience in any programming language like C or C++, then it will significantly enhance your learning experience.
C# Features
C# is a user-friendly language that offers a structured approach to problem-solving. it provides a wide range of library functions and data types to work.
Modern Programming Language
C# programming is a popular and powerful language that is for creating scalable, interoperable, and robust applications.
Object Oriented
C# is an object-oriented programming language, which makes development and maintenance easier. In contrast, with procedure-oriented programming languages, managing code becomes difficult as project size grows.
The code is type safe can only access memory locations that it has permission to execute. This feature significantly enhances program security.
Interoperability
The interoperability process allows C# programs to perform all the tasks that a native C++ application.
Scalable and Updateable
C# is a programming language that is scalable and can be updated automatically. To update our application, we remove the old files and replace them with new ones.
Component Oriented
It is widely used as a software development methodology to create applications that are more strong and can easily scale.
Structured Programming Language
C# is a structured programming language that allows us to divide programs into parts using functions, making it easy to understand and modify.
The compilation and execution time of C# language is fast.
Recent Articles on C# !
Overview , Fundamentals , Important Keywords , Control Statements , OOP Concepts , Methods , Delegates , Constructors , Arrays , ArrayList , String , Tuple , ValueTuple , Indexers , Inheritance , Interfaces , Multithreading , Exception Handling , Collections and Generics , Collections Namespace , Generic Namespace , System Namespace , Specialized Namespace , What’s New in C# 8.0 , Windows Forms
C# Tutorial- C# Index
- .NET Framework (Basic Architecture and Component Stack)
- Managed code and Unmanaged code in .NET
- Managed vs Unmanaged Code
- CIL or MSIL
- .NET Framework Class Library (FCL)
- Introduction to C#
- Setting up the Environment in C#
- How to Install and Setup Visual Studio for C#?
- Evolution of C#
- How to Execute C# Program on cmd (command-line)?
- Main Method
- Getting Familiar With Visual Studio
- Common Language Runtime(CLR)
- Architecture of Common Language Runtime (CLR)
- JIT(Just-In-Time) Compiler
- Garbage Collection
- Windows Form Applications
- Python vs C#
- Interesting Facts about C#
- Type System Unification in C# .NET
- Identifiers
- Types of Variables
- Implicitly Typed Local Variables – var
- Dynamic Type in C#
- var vs dynamic
- Binary Literals and Digit Separators
- Scope of Variables
- Access Modifiers
- Constants or Literals
- Command Line Arguments
- Boxing and Unboxing
- Boxing vs UnBoxing
- Params in C#
- Comments in C#
- Type Casting or Type Conversion
- Enumeration in C#
- Properties in C#
- Nullable Types
Important Keywords
- Is vs As operator keyword
- static keyword
- typeof Keyword
- Difference between readonly and const keyword
- ref keyword
- Decision-Making Statements
- Switch Statement
- Foreach Loop
- Jump Statements(Break, Continue, Goto, Return and Throw)
- Class and Object
- Nested Classes
- Difference between Class and Structure
- Early and Late Binding
- Overloading of Constructors
- Inheritance in C#
- Encapsulation in C#
- Abstraction in C#
- this keyword
- Static Class
- Partial Classes
- Shallow Copy and Deep Copy
- Different ways to create an Object
- Object and Collection Initializer
- Accessing structure’s elements using Pointers
- Method Overloading
- Method Returning an Object
- Method Parameters
- Runtime(Dynamic) Polymorphsim
- Method Overriding
- Method Hiding
- Method Overriding vs Method Hiding
- Optional Parameters
- Different ways to make Method Parameter Optional
- Out Parameters with examples
- Difference between Ref and Out keywords
- Anonymous Method
- Partial Methods
- Extension Method
- Local Function
- Predicate Delegate
- Action Delegate
- Func Delegate
Constructors
- Constructors in C#
- Default Constructor
- Copy Constructor
- Private Constructor
- Constructor Overloading
- Static Constructors vs Non-Static Constructors
- Invoking an overloaded constructor using this keyword
- Destructors
- Jagged Arrays
- Arrays of Strings
- Using foreach loop in arrays
- Array Class
- Sorting an Array
- Length of an Array
- Array.BinarySearch() Method
- Check if two array objects are equal or not
- Number of elements in a specified dimension of an Array
- LongLength property of an Array
- Rank of an Array
- Passing Arrays as Arguments
- Implicitly Typed Arrays
- Object and Dynamic Arrays
- Array IndexOutofRange Exception
- Different ways to sort an array in descending order
- What is ArrayList?
- How to create the ArrayList?
- ArrayList Class
- Array vs ArrayList
- Adding the elements to the end of the ArrayList
- Removing all the elements from the ArrayList
- Removing a range of elements from the ArrayList
- ArrayList to Array Conversion
- Copying the entire ArrayList to a 1-D Array
- Copying the entire ArrayList to 1-D Array starting at the specified index
- Check if two ArrayList objects are equal
- Verbatim String Literal – @
- String Class
- String Class Properties
- How to use strings in switch statement
- String vs StringBuilder
- Length of the StringBuilder
- Remove all characters from StringBuilder
- Check if two StringBuilder objects are Equal
- Capacity of a StringBuilder
- What is Tuple in C#?
- Tuple Class
- Tuple<T1> Class
- Tuple<T1,T2> Class
- Tuple<T1,T2,T3> Class
- Tuple<T1,T2,T3,T4> Class
- Tuple<T1,T2,T3,T4,T5> Class
- Tuple<T1,T2,T3,T4,T5,T6> Class
- Tuple<T1,T2,T3,T4,T5,T6,T7> Class
- Tuple<T1,T2,T3,T4,T5,T6,T7,TRest> Class
- What is ValueTuple in C#?
- ValueTuple Struct
- ValueTuple <T1> Struct
- ValueTuple <T1,T2> Struct
- ValueTuple <T1,T2,T3> Struct
- ValueTuple <T1,T2,T3,T4> Struct
- ValueTuple <T1,T2,T3,T4,T5> Struct
- ValueTuple <T1,T2,T3,T4,T5,T6> Struct
- ValueTuple <T1,T2,T3,T4,T5,T6,T7> Struct
- ValueTuple <T1,T2,T3,T4,T5,T6,T7,TRest> Struct
Indexers & Properties
- Multidimensional Indexers
- Overloading of Indexers
- Restrictions on Properties
Inheritance
- Multilevel Inheritance
- Multiple inheritance using interfaces
- Inheritance in Constructors
- Inheritance in Interfaces
- Abstract Classes
- Using sealed class to Prevent Inheritance
- Object Class
- Interface in C#
- How to use Interface References
- How to Implement Multiple Interfaces Having Same Method Name
- Difference between Abstract Class and Interface
- Delegates vs Interfaces
- Explicit Interface Implementation
Multithreading
- Introduction to Multithreading
- Types of Threads
- How to create Threads
- Main Thread
- Lifecycle and States of a Thread
- Thread Class
- Scheduling a thread for Execution
- Check whether a Thread is Alive or not
- Joining Threads
- Terminating a Thread
- Check whether a thread is a background thread or not
- Naming a thread and fetching name of current thread
- Thread Priority in Multithreading
Exception Handling
- System Level Exception vs Application Level Exception
- How to use Multiple Catch Clause
- Nesting of try and catch blocks
- Using finally
Collections & Generics
- SortedList with Examples
- Dictionary with Examples
- SortedDictionary
- Hashtable with Examples
- Stack with Examples
- Queue with Examples
- Hashtable vs Dictionary
- SortedList vs SortedDictionary
Collections Namespace
- C# | Stack Class
- C# | Queue Class
- C# | Hashtable Class
- C# | BitArray Class
- C# | SortedList Class
Generic Namespace
- C# | HashSet<T> Class
- C# | LinkedList<T> Class
- C# | List<T> Class
- C# | SortedSet<T> Class
- Dictionary Class
- SortedDictionary Class
System Namespace
- BitConverter Class
- Console Class
- Convert Class
- Decimal Struct
- Byte Struct
- Char Struct
- Int16 Struct
- Int32 Struct
- Int64 Struct
- UInt16 Struct
- UInt32 Struct
- UInt64 Struct
Specialized Namespace
- C# | ListDictionary Class
- C# | StringCollection Class
- C# | OrderedDictionary Class
- C# | HybridDictionary Class
- C# | StringDictionary Class
What’s New in C# 8.0
- Static Local Function
- Range and Indices
- Range Structure
- Index Struct
Windows Forms
- What is Windows Forms(WF) in C#?
- Button Control
- Label Control
- RadioButton Control
- CheckBox Control
- TextBox Control
- ComboBox Control
- ToolTip Class
- RichTextBox Class
- MaskedBox Class
- NumericUpDown Class
- DateTimePicker Class
- ListBox Class
- GroupBox Class
- FlowLayoutPanel Class
Why use C#?
C# is a popular and simple-to-learn programming language with a large community and plain syntax. C# is an object-oriented programming language that makes code more structured, reusable, and interoperable with other languages. C# is a versatile programming language that can be used to create a broad variety of applications, including games, apps, websites, and advanced technologies such as AI and machine learning.
C# is used for:
- Mobile applications
- Desktop applications
- Web applications
- Web services
- Database applications
Advantages of C#
- Easy Syntax : C# is a simple syntax that is easy to learn for a beginner.
- Object-oriented Programming language : It is an object-oriented programming language used to make classes and objects.
- Versatile language : It is used to develop software, web applications, mobile apps, and games.
- Platform Independent : C# can be used to make apps that work on Windows, macOS, and mobile devices.
In the above section, we have already said that this free C# programming tutorial is designed to help both beginners and experienced.
Q.1 What is C# programming language used for?
Answer : C# is a versatile programming language primarily used for developing Windows applications, web services, and games within the .NET framework.
Q.2 What is the advantage of ‘using’ statement in C#?
Answer : The ‘using’ statement provides resources for processing before automatically disposing of it when execution is completed.
Q.3 What is serialization?
Answer : To transport an object through a network, we need to convert it into a stream of bytes. It is called Serialization.
Q.4 What are the four steps involved in the C# code compilation?
Answer : Four steps of code compilation in C# include: Source code compilation in managed code. Newly created code is merged with assembly code. The Common Language Runtime (CLR) is loaded. Assembly execution is done through CLR.
Q.5 How can I declare and initialize variables in C#?
Answer: Variables are named containers that store values of different types. To declare a variable in C#, you need to specify its type and name.
Please Login to comment...
- 10 Best Free Social Media Management and Marketing Apps for Android - 2024
- 10 Best Customer Database Software of 2024
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
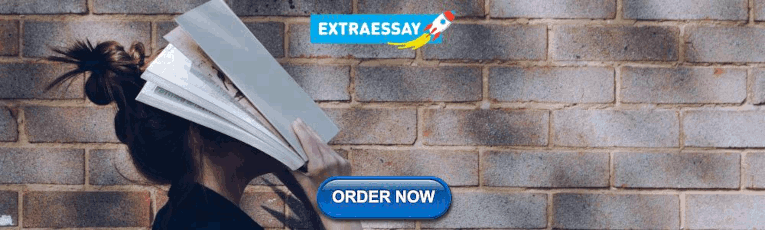
COMMENTS
This is an introduction to how challenges on Edabit work. In the Code tab above you'll see a starter function that looks like this: public class Program { public static bool ReturnTrue () { } } All you have to do is type return true; between the curly braces { } and then click the Check button. If you did this ….
C# is an elegant and type-safe object-oriented language that enables developers to build a variety of secure and robust applications that run on the .NET Framework. You can use C# to create Windows client applications, XML Web services, distributed components, client-server applications, database applications, and much, much more.
Code practice and mentorship for everyone. Develop fluency in 69 programming languages with our unique blend of learning, practice and mentoring. Exercism is fun, effective and 100% free, forever. Explore the 164 C# exercises on Exercism.
C#. Developed around 2000 by Microsoft as part of its .NET initiative, C# is a general-purpose, object-oriented programming language designed for Common Language Infrastructure (CLI), and widely recognized for its structured, strong-typing and lexical scoping abilities. This competency area includes understanding the structure of C# programs ...
We have gathered a variety of C# exercises (with answers) for each C# Chapter. Try to solve an exercise by editing some code, or show the answer to see what you've done wrong. Count Your Score. You will get 1 point for each correct answer. Your score and total score will always be displayed.
1,797 ecolban. Fundamentals. Practice C# coding with code challenges designed to engage your programming skills. Solve coding problems and pick up new techniques from your fellow peers.
C# • Web Development • Code Foundations The template home page contains placeholder text. Replace this with continent and country lists. The student will study the code they already created in previous lessons and reuse that to complete the task. More guidance, 15 min.
1% better everyday can lead to big results. Get hands-on experience with Practice C# programming practice problem course on CodeChef. Solve a wide range of Practice C# coding challenges and boost your confidence in programming.
1) C# Program to Generate a Multiplication Table of a given integer. 2) Try Catch Finally in C# With Real World Examples. 3) C# Program to Check Whether a Number is Palindrome or Not. 4) C# While Loop with examples. 5) C# Program to Print Fibonacci Series. Best quality Asp .Net Ajax Control Toolkit tutorials.
C# is a user-friendly language that offers a structured approach to problem-solving. it provides a wide range of library functions and data types to work. Modern Programming Language. C# programming is a popular and powerful language that is for creating scalable, interoperable, and robust applications. Object Oriented